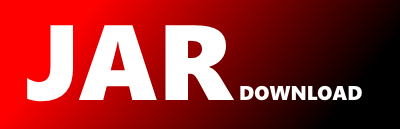
org.ssssssss.magicapi.config.MagicFunctionManager Maven / Gradle / Ivy
package org.ssssssss.magicapi.config;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import org.ssssssss.magicapi.model.FunctionInfo;
import org.ssssssss.magicapi.model.Group;
import org.ssssssss.magicapi.model.Parameter;
import org.ssssssss.magicapi.model.TreeNode;
import org.ssssssss.magicapi.provider.FunctionServiceProvider;
import org.ssssssss.magicapi.provider.GroupServiceProvider;
import org.ssssssss.magicapi.script.ScriptManager;
import org.ssssssss.magicapi.utils.PathUtils;
import org.ssssssss.script.MagicResourceLoader;
import org.ssssssss.script.MagicScriptContext;
import org.ssssssss.script.exception.MagicExitException;
import org.ssssssss.script.runtime.ExitValue;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Set;
import java.util.concurrent.ConcurrentHashMap;
import java.util.function.Function;
import java.util.stream.Collectors;
public class MagicFunctionManager {
private static final Logger logger = LoggerFactory.getLogger(MagicFunctionManager.class);
private static final Map mappings = new ConcurrentHashMap<>();
private final GroupServiceProvider groupServiceProvider;
private final FunctionServiceProvider functionServiceProvider;
private TreeNode groups;
public MagicFunctionManager(GroupServiceProvider groupServiceProvider, FunctionServiceProvider functionServiceProvider) {
this.groupServiceProvider = groupServiceProvider;
this.functionServiceProvider = functionServiceProvider;
}
public void registerFunctionLoader() {
MagicResourceLoader.addFunctionLoader((path) -> {
FunctionInfo info = mappings.get(path);
if (info != null) {
List parameters = info.getParameters();
return (Function
© 2015 - 2025 Weber Informatics LLC | Privacy Policy