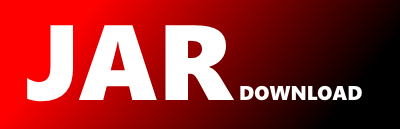
org.ssssssss.magicapi.controller.MagicWebSocketDispatcher Maven / Gradle / Ivy
package org.ssssssss.magicapi.controller;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import org.springframework.web.socket.CloseStatus;
import org.springframework.web.socket.TextMessage;
import org.springframework.web.socket.WebSocketSession;
import org.springframework.web.socket.handler.TextWebSocketHandler;
import org.ssssssss.magicapi.config.Message;
import org.ssssssss.magicapi.config.WebSocketSessionManager;
import org.ssssssss.magicapi.model.Constants;
import org.ssssssss.magicapi.model.MagicConsoleSession;
import org.ssssssss.magicapi.model.MagicNotify;
import org.ssssssss.magicapi.provider.MagicNotifyService;
import org.ssssssss.magicapi.utils.Invoker;
import org.ssssssss.magicapi.utils.JsonUtils;
import org.ssssssss.script.reflection.MethodInvoker;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.stream.Stream;
import static org.ssssssss.magicapi.model.Constants.EMPTY_OBJECT_ARRAY;
public class MagicWebSocketDispatcher extends TextWebSocketHandler {
private static final Logger logger = LoggerFactory.getLogger(MagicWebSocketDispatcher.class);
private static final Map handlers = new HashMap<>();
private final String instanceId;
private final MagicNotifyService magicNotifyService;
public MagicWebSocketDispatcher(String instanceId, MagicNotifyService magicNotifyService, List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy