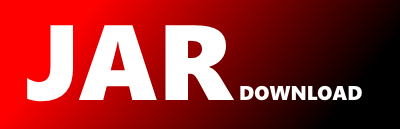
org.ssssssss.magicapi.modules.table.NamedTable Maven / Gradle / Ivy
package org.ssssssss.magicapi.modules.table;
import org.apache.commons.lang3.StringUtils;
import org.ssssssss.magicapi.exception.MagicAPIException;
import org.ssssssss.magicapi.modules.BoundSql;
import org.ssssssss.magicapi.modules.SQLModule;
import org.ssssssss.script.annotation.Comment;
import java.util.*;
import java.util.function.Function;
import java.util.stream.Collectors;
public class NamedTable {
String tableName;
SQLModule sqlModule;
String primary;
String logicDeleteColumn;
String logicDeleteValue;
Map columns = new HashMap<>();
List fields = new ArrayList<>();
List groups = new ArrayList<>();
List orders = new ArrayList<>();
Set excludeColumns = new HashSet<>();
Function rowMapColumnMapper;
Object defaultPrimaryValue;
boolean useLogic = false;
boolean withBlank = false;
Where where = new Where(this);
public NamedTable(String tableName, SQLModule sqlModule, Function rowMapColumnMapper) {
this.tableName = tableName;
this.sqlModule = sqlModule;
this.rowMapColumnMapper = rowMapColumnMapper;
this.logicDeleteColumn = sqlModule.getLogicDeleteColumn();
this.logicDeleteValue = sqlModule.getLogicDeleteValue();
}
@Comment("使用逻辑删除")
public NamedTable logic(){
this.useLogic = true;
return this;
}
@Comment("更新空值")
public NamedTable withBlank(){
this.withBlank = true;
return this;
}
@Comment("设置主键名,update时使用")
public NamedTable primary(String primary) {
return primary(primary, null);
}
@Comment("设置主键名,并设置默认主键值(主要用于insert)")
public NamedTable primary(String primary, Object defaultPrimaryValue) {
this.primary = rowMapColumnMapper.apply(primary);
this.defaultPrimaryValue = defaultPrimaryValue;
return this;
}
@Comment("拼接where")
public Where where() {
return where;
}
@Comment("设置单列的值")
public NamedTable column(@Comment("列名") String key, @Comment("值") Object value) {
this.columns.put(rowMapColumnMapper.apply(key), value);
return this;
}
@Comment("设置查询的列,如`columns('a','b','c')`")
public NamedTable columns(@Comment("各项列") String... columns) {
if (columns != null) {
for (String column : columns) {
column(column);
}
}
return this;
}
@Comment("设置要排除的列")
public NamedTable exclude(String column){
if(column != null){
excludeColumns.add(column);
}
return this;
}
@Comment("设置要排除的列")
public NamedTable excludes(String ... columns){
if(columns != null){
excludeColumns.addAll(Arrays.asList(columns));
}
return this;
}
@Comment("设置要排除的列")
public NamedTable excludes(List columns){
if(columns != null){
excludeColumns.addAll(columns);
}
return this;
}
@Comment("设置查询的列,如`columns(['a','b','c'])`")
public NamedTable columns(Collection columns) {
if (columns != null) {
columns.stream().filter(StringUtils::isNotBlank).map(rowMapColumnMapper).forEach(this.fields::add);
}
return this;
}
@Comment("设置查询的列,如`column('a')`")
public NamedTable column(String column) {
if (StringUtils.isNotBlank(column)) {
this.fields.add(this.rowMapColumnMapper.apply(column));
}
return this;
}
@Comment("拼接`order by xxx asc/desc`")
public NamedTable orderBy(@Comment("要排序的列") String column, @Comment("`asc`或`desc`") String sort) {
this.orders.add(column + " " + sort);
return this;
}
@Comment("拼接`order by xxx asc`")
public NamedTable orderBy(@Comment("要排序的列") String column) {
return orderBy(column, "asc");
}
@Comment("拼接`order by xxx desc`")
public NamedTable orderByDesc(@Comment("要排序的列") String column) {
return orderBy(column, "desc");
}
@Comment("拼接`group by`")
public NamedTable groupBy(@Comment("要分组的列") String... columns) {
this.groups.addAll(Arrays.asList(columns));
return this;
}
private Collection> filterNotBlanks() {
if(this.withBlank){
return this.columns.entrySet()
.stream()
.filter(it -> !excludeColumns.contains(it.getKey()))
.collect(Collectors.toList());
}
return this.columns.entrySet()
.stream()
.filter(it -> StringUtils.isNotBlank(Objects.toString(it.getValue(), "")))
.filter(it -> !excludeColumns.contains(it.getKey()))
.collect(Collectors.toList());
}
@Comment("执行插入,返回主键")
public Object insert() {
return insert(null);
}
@Comment("执行插入,返回主键")
public Object insert(@Comment("各项列和值") Map data) {
if (data != null) {
data.forEach((key, value) -> this.columns.put(rowMapColumnMapper.apply(key), value));
}
if (this.defaultPrimaryValue != null && StringUtils.isBlank(Objects.toString(this.columns.getOrDefault(this.primary, "")))) {
this.columns.put(this.primary, this.defaultPrimaryValue);
}
Collection> entries = filterNotBlanks();
if (entries.isEmpty()) {
throw new MagicAPIException("参数不能为空");
}
StringBuilder builder = new StringBuilder();
builder.append("insert into ");
builder.append(tableName);
builder.append("(");
builder.append(StringUtils.join(entries.stream().map(Map.Entry::getKey).toArray(), ","));
builder.append(") values (");
builder.append(StringUtils.join(Collections.nCopies(entries.size(), "?"), ","));
builder.append(")");
return sqlModule.insert(new BoundSql(builder.toString(), entries.stream().map(Map.Entry::getValue).collect(Collectors.toList()), sqlModule), this.primary);
}
@Comment("执行delete语句")
public int delete() {
if(useLogic){
Map dataMap = new HashMap<>();
dataMap.put(logicDeleteColumn, logicDeleteValue);
return update(dataMap);
}
if (where.isEmpty()) {
throw new MagicAPIException("delete语句不能没有条件");
}
StringBuilder builder = new StringBuilder();
builder.append("delete from ");
builder.append(tableName);
builder.append(where.getSql());
return sqlModule.update(new BoundSql(builder.toString(), where.getParams(), sqlModule));
}
@Comment("保存到表中,当主键有值时则修改,否则插入")
public Object save() {
return this.save(null, false);
}
@Comment("保存到表中,当主键有值时则修改,否则插入")
public Object save(@Comment("各项列和值") Map data, @Comment("是否根据id查询有没有数据") boolean beforeQuery) {
if (StringUtils.isBlank(this.primary)) {
throw new MagicAPIException("请设置主键");
}
String primaryValue = Objects.toString(this.columns.get(this.primary), "");
if (StringUtils.isBlank(primaryValue) && data != null) {
primaryValue = Objects.toString(data.get(this.primary), "");
}
if (beforeQuery) {
if (StringUtils.isNotBlank(primaryValue)) {
List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy