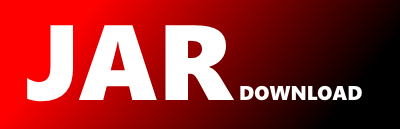
org.ssssssss.magicapi.swagger.SwaggerEntity Maven / Gradle / Ivy
package org.ssssssss.magicapi.swagger;
import java.util.*;
/**
* Swagger接口信息
*/
public class SwaggerEntity {
private String swagger = "2.0";
private String host;
private String basePath;
private Info info;
private Set tags = new TreeSet<>(Comparator.comparing(Tag::getName));
private Map definitions = new HashMap<>();
private Map> paths = new HashMap<>();
private static Map doProcessSchema(Object target) {
Map result = new HashMap<>(3);
result.put("type", getType(target));
if (target instanceof List) {
List targetList = (List) target;
if (targetList.size() > 0) {
result.put("items", doProcessSchema(targetList.get(0)));
} else {
result.put("items", Collections.emptyList());
}
} else if (target instanceof Map) {
Set entries = ((Map) target).entrySet();
Map> properties = new HashMap<>(entries.size());
for (Map.Entry entry : entries) {
properties.put(Objects.toString(entry.getKey()), doProcessSchema(entry.getValue()));
}
result.put("properties", properties);
} else {
result.put("example", target == null ? "" : target);
result.put("description", target == null ? "" : target);
}
return result;
}
private static String getType(Object object) {
if (object instanceof Number) {
return "number";
}
if (object instanceof String) {
return "string";
}
if (object instanceof Boolean) {
return "boolean";
}
if (object instanceof List) {
return "array";
}
if (object instanceof Map) {
return "object";
}
return "string";
}
public Info getInfo() {
return info;
}
public void setInfo(Info info) {
this.info = info;
}
public void addPath(String path, String method, Path pathInfo) {
Map map = paths.computeIfAbsent(path, k -> new HashMap<>());
map.put(method.toLowerCase(), pathInfo);
}
public void addTag(String name, String description) {
this.tags.add(new Tag(name, description));
}
public String getHost() {
return host;
}
public void setHost(String host) {
this.host = host;
}
public String getSwagger() {
return swagger;
}
public void setSwagger(String swagger) {
this.swagger = swagger;
}
public String getBasePath() {
return basePath;
}
public void setBasePath(String basePath) {
this.basePath = basePath;
}
public Map getDefinitions() {
return definitions;
}
public void addDefinitions(String path, Object definition) {
definitions.put(path, definition);
}
public Set getTags() {
return tags;
}
public Map> getPaths() {
return paths;
}
public static class Concat {
private String name;
private String url;
private String email;
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public String getUrl() {
return url;
}
public void setUrl(String url) {
this.url = url;
}
public String getEmail() {
return email;
}
public void setEmail(String email) {
this.email = email;
}
}
public static class Info {
private String description;
private String version;
private String title;
private License license;
private Concat concat;
public Info(String description, String version, String title, License license, Concat concat) {
this.description = description;
this.version = version;
this.title = title;
this.license = license;
this.concat = concat;
}
public String getDescription() {
return description;
}
public void setDescription(String description) {
this.description = description;
}
public String getVersion() {
return version;
}
public void setVersion(String version) {
this.version = version;
}
public String getTitle() {
return title;
}
public void setTitle(String title) {
this.title = title;
}
public License getLicense() {
return license;
}
public void setLicense(License license) {
this.license = license;
}
public Concat getConcat() {
return concat;
}
public void setConcat(Concat concat) {
this.concat = concat;
}
}
public static class Path {
private List tags = new ArrayList<>();
private String summary;
private String description;
private String operationId = UUID.randomUUID().toString().replace("-", "");
private List produces = new ArrayList<>();
private List consumes = new ArrayList<>();
private List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy