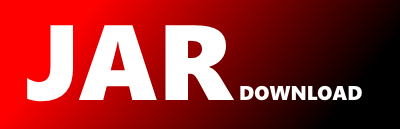
org.structr.web.entity.VideoFile Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of structr-ui Show documentation
Show all versions of structr-ui Show documentation
Structr is an open source framework based on the popular Neo4j graph database.
The newest version!
/**
* Copyright (C) 2010-2016 Structr GmbH
*
* This file is part of Structr .
*
* Structr is free software: you can redistribute it and/or modify
* it under the terms of the GNU Affero General Public License as
* published by the Free Software Foundation, either version 3 of the
* License, or (at your option) any later version.
*
* Structr is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU Affero General Public License for more details.
*
* You should have received a copy of the GNU Affero General Public License
* along with Structr. If not, see .
*/
package org.structr.web.entity;
import java.util.LinkedHashMap;
import java.util.List;
import java.util.Map;
import java.util.Map.Entry;
import java.util.logging.Level;
import java.util.logging.Logger;
import org.structr.common.PropertyView;
import org.structr.common.SecurityContext;
import org.structr.common.error.ErrorBuffer;
import org.structr.common.error.FrameworkException;
import org.structr.core.Export;
import static org.structr.core.GraphObject.type;
import org.structr.core.GraphObjectMap;
import org.structr.core.JsonInput;
import org.structr.core.app.StructrApp;
import static org.structr.core.graph.NodeInterface.name;
import static org.structr.core.graph.NodeInterface.owner;
import org.structr.core.graph.Tx;
import org.structr.core.property.ConstantBooleanProperty;
import org.structr.core.property.DoubleProperty;
import org.structr.core.property.EndNode;
import org.structr.core.property.EndNodes;
import org.structr.core.property.IntProperty;
import org.structr.core.property.Property;
import org.structr.core.property.StartNode;
import org.structr.core.property.StringProperty;
import org.structr.dynamic.File;
import org.structr.media.AVConv;
import org.structr.rest.RestMethodResult;
import org.structr.schema.SchemaService;
import org.structr.web.common.FileHelper;
import static org.structr.web.entity.FileBase.relativeFilePath;
import static org.structr.web.entity.FileBase.size;
import org.structr.web.entity.relation.VideoFileHasConvertedVideoFile;
import org.structr.web.entity.relation.VideoFileHasPosterImage;
//~--- classes ----------------------------------------------------------------
/**
* A video whose binary data will be stored on disk.
*
*
*
*/
public class VideoFile extends File {
private static final Logger logger = Logger.getLogger(VideoFile.class.getName());
// register this type as an overridden builtin type
static {
SchemaService.registerBuiltinTypeOverride("VideoFile", VideoFile.class.getName());
}
public static final Property> convertedVideos = new EndNodes<>("convertedVideos", VideoFileHasConvertedVideoFile.class);
public static final Property originalVideo = new StartNode<>("originalVideo", VideoFileHasConvertedVideoFile.class);
public static final Property posterImage = new EndNode<>("posterImage", VideoFileHasPosterImage.class);
public static final Property isVideo = new ConstantBooleanProperty("isVideo", true);
public static final Property videoCodecName = new StringProperty("videoCodecName").cmis();
public static final Property videoCodec = new StringProperty("videoCodec").cmis();
public static final Property pixelFormat = new StringProperty("pixelFormat").cmis();
public static final Property audioCodecName = new StringProperty("audioCodecName").cmis();
public static final Property audioCodec = new StringProperty("audioCodec").cmis();
public static final Property audioChannels = new IntProperty("audioChannels").cmis();
public static final Property sampleRate = new DoubleProperty("sampleRate").cmis().indexed();
public static final Property duration = new DoubleProperty("duration").cmis().indexed();
public static final Property width = new IntProperty("width").cmis().indexed();
public static final Property height = new IntProperty("height").cmis().indexed();
public static final org.structr.common.View uiView = new org.structr.common.View(VideoFile.class, PropertyView.Ui,
type, name, contentType, size, relativeFilePath, owner, parent, path, isVideo, videoCodecName, videoCodec, pixelFormat,
audioCodecName, audioCodec, audioChannels, sampleRate, duration, width, height, originalVideo, convertedVideos,
posterImage
);
public static final org.structr.common.View publicView = new org.structr.common.View(VideoFile.class, PropertyView.Public,
type, name, owner, parent, path, isVideo, videoCodecName, videoCodec, pixelFormat,
audioCodecName, audioCodec, audioChannels, sampleRate, duration, width, height,
convertedVideos, posterImage
);
@Override
public boolean onCreation(SecurityContext securityContext, ErrorBuffer errorBuffer) throws FrameworkException {
updateVideoInfo();
return super.onCreation(securityContext, errorBuffer);
}
@Override
public boolean onModification(SecurityContext securityContext, ErrorBuffer errorBuffer) throws FrameworkException {
updateVideoInfo();
return super.onModification(securityContext, errorBuffer);
}
public String getDiskFilePath(final SecurityContext securityContext) {
try (final Tx tx = StructrApp.getInstance(securityContext).tx()) {
final String path = getRelativeFilePath();
tx.success();
if (path != null) {
return new java.io.File(FileHelper.getFilePath(path)).getAbsolutePath();
}
} catch (FrameworkException fex) {
logger.log(Level.WARNING, "", fex);
}
return null;
}
@Export
public void convert(final String scriptName, final String newFileName) throws FrameworkException {
AVConv.newInstance(securityContext, this, newFileName).doConversion(scriptName);
}
@Export
public void grab(final String scriptName, final String imageName, final long timeIndex) throws FrameworkException {
AVConv.newInstance(securityContext, this, imageName).grabFrame(scriptName, imageName, timeIndex);
}
@Export
public RestMethodResult getMetadata() throws FrameworkException {
final Map metadata = AVConv.newInstance(securityContext, this).getMetadata();
final RestMethodResult result = new RestMethodResult(200);
final GraphObjectMap map = new GraphObjectMap();
for (final Entry entry : metadata.entrySet()) {
map.setProperty(new StringProperty(entry.getKey()), entry.getValue());
}
result.addContent(map);
return result;
}
@Export
public void setMetadata(final String key, final String value) throws FrameworkException {
AVConv.newInstance(securityContext, this).setMetadata(key, value);
}
@Export
public void setMetadata(final JsonInput metadata) throws FrameworkException {
final Map map = new LinkedHashMap<>();
for (final Entry entry : metadata.entrySet()) {
map.put(entry.getKey(), entry.getValue().toString());
}
AVConv.newInstance(securityContext, this).setMetadata(map);
}
@Export
public void updateVideoInfo() {
try (final Tx tx = StructrApp.getInstance(securityContext).tx()) {
final Map info = AVConv.newInstance(securityContext, this).getVideoInfo();
if (info != null && info.containsKey("streams")) {
final List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy