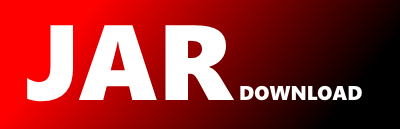
org.structr.websocket.command.ClonePageCommand Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of structr-ui Show documentation
Show all versions of structr-ui Show documentation
Structr is an open source framework based on the popular Neo4j graph database.
The newest version!
/**
* Copyright (C) 2010-2016 Structr GmbH
*
* This file is part of Structr .
*
* Structr is free software: you can redistribute it and/or modify
* it under the terms of the GNU Affero General Public License as
* published by the Free Software Foundation, either version 3 of the
* License, or (at your option) any later version.
*
* Structr is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU Affero General Public License for more details.
*
* You should have received a copy of the GNU Affero General Public License
* along with Structr. If not, see .
*/
package org.structr.websocket.command;
import java.util.Map;
import java.util.logging.Level;
import java.util.logging.Logger;
import org.structr.common.SecurityContext;
import org.structr.common.error.FrameworkException;
import org.structr.core.entity.AbstractNode;
import org.structr.web.entity.dom.DOMNode;
import org.structr.web.entity.dom.Page;
import org.structr.websocket.StructrWebSocket;
import org.structr.websocket.message.MessageBuilder;
import org.structr.websocket.message.WebSocketMessage;
import org.w3c.dom.DOMException;
//~--- classes ----------------------------------------------------------------
/**
* Websocket command to clone a page
*
*
*/
public class ClonePageCommand extends AbstractCommand {
private static final Logger logger = Logger.getLogger(ClonePageCommand.class.getName());
static {
StructrWebSocket.addCommand(ClonePageCommand.class);
}
//~--- methods --------------------------------------------------------
@Override
public void processMessage(final WebSocketMessage webSocketData) {
final SecurityContext securityContext = getWebSocket().getSecurityContext();
// Node to clone
String nodeId = webSocketData.getId();
final AbstractNode nodeToClone = getNode(nodeId);
final Map nodeData = webSocketData.getNodeData();
final String newName;
if (nodeData.containsKey(AbstractNode.name.dbName())) {
newName = (String) nodeData.get(AbstractNode.name.dbName());
} else {
newName = "unknown";
}
if (nodeToClone != null) {
try {
final Page pageToClone = nodeToClone instanceof Page ? (Page) nodeToClone : null;
if (pageToClone != null) {
//final List elements = pageToClone.getProperty(Page.elements);
DOMNode firstChild = (DOMNode) pageToClone.getFirstChild().getNextSibling();
if (firstChild == null) {
firstChild = (DOMNode) pageToClone.treeGetFirstChild();
}
final DOMNode newHtmlNode = DOMNode.cloneAndAppendChildren(securityContext, firstChild);
final Page newPage = (Page) pageToClone.cloneNode(false);
newPage.setProperty(Page.name, pageToClone.getProperty(Page.name) + "-" + newPage.getIdString());
newPage.appendChild(newHtmlNode);
}
} catch (FrameworkException fex) {
logger.log(Level.WARNING, "Could not create node.", fex);
getWebSocket().send(MessageBuilder.status().code(fex.getStatus()).message(fex.getMessage()).build(), true);
} catch (DOMException dex) {
logger.log(Level.WARNING, "Could not create node.", dex);
getWebSocket().send(MessageBuilder.status().code(422).message(dex.getMessage()).build(), true);
}
} else {
logger.log(Level.WARNING, "Node with uuid {0} not found.", webSocketData.getId());
getWebSocket().send(MessageBuilder.status().code(404).build(), true);
}
}
//~--- get methods ----------------------------------------------------
@Override
public String getCommand() {
return "CLONE_PAGE";
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy