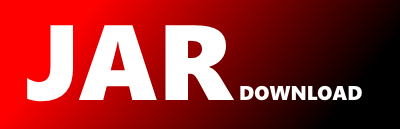
org.structr.websocket.message.MessageBuilder Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of structr-ui Show documentation
Show all versions of structr-ui Show documentation
Structr is an open source framework based on the popular Neo4j graph database.
The newest version!
/**
* Copyright (C) 2010-2016 Structr GmbH
*
* This file is part of Structr .
*
* Structr is free software: you can redistribute it and/or modify
* it under the terms of the GNU Affero General Public License as
* published by the Free Software Foundation, either version 3 of the
* License, or (at your option) any later version.
*
* Structr is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU Affero General Public License for more details.
*
* You should have received a copy of the GNU Affero General Public License
* along with Structr. If not, see .
*/
package org.structr.websocket.message;
import com.google.gson.JsonElement;
import java.util.Map;
import java.util.Map.Entry;
/**
*
*
*/
public class MessageBuilder {
private WebSocketMessage data = null;
public MessageBuilder() {
data = new WebSocketMessage();
}
// ----- static methods -----
public static MessageBuilder progress() {
return builder().command("PROGRESS");
}
public static MessageBuilder finished() {
return builder().command("FINISHED");
}
public static MessageBuilder status() {
return builder().command("STATUS");
}
public static MessageBuilder create() {
return builder().command("CREATE");
}
public static MessageBuilder delete() {
return builder().command("DELETE");
}
public static MessageBuilder update() {
return builder().command("UPDATE");
}
public static MessageBuilder wrappedRest() {
return builder().command("WRAPPED_REST");
}
public static MessageBuilder forName(final String command) {
return builder().command(command);
}
// ----- non-static methods -----
public MessageBuilder command(String command) {
data.setCommand(command);
return this;
}
public MessageBuilder code(int code) {
data.setCode(code);
return this;
}
public MessageBuilder id(String id) {
data.setId(id);
return this;
}
public MessageBuilder message(String message) {
data.setMessage(message);
return this;
}
public MessageBuilder callback(String callback) {
if (callback != null) {
data.setCallback(callback);
}
return this;
}
public MessageBuilder data(String key, Object value) {
data.setNodeData(key, value);
return this;
}
public MessageBuilder data(Map data) {
for(Entry entry : data.entrySet()) {
this.data.setNodeData(entry.getKey(), entry.getValue());
}
return this;
}
public MessageBuilder jsonErrorObject(JsonElement error) {
data.setJsonErrorObject(error);
return this;
}
public WebSocketMessage build() {
return data;
}
// ----- private methods -----
private static MessageBuilder builder() {
return new MessageBuilder();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy