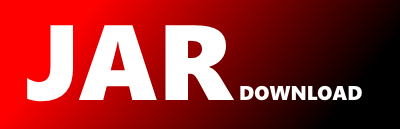
swim.api.lane.JoinValueLane Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of swim-api Show documentation
Show all versions of swim-api Show documentation
Agent programming interface
// Copyright 2015-2021 Swim Inc.
//
// Licensed under the Apache License, Version 2.0 (the "License");
// you may not use this file except in compliance with the License.
// You may obtain a copy of the License at
//
// http://www.apache.org/licenses/LICENSE-2.0
//
// Unless required by applicable law or agreed to in writing, software
// distributed under the License is distributed on an "AS IS" BASIS,
// WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
// See the License for the specific language governing permissions and
// limitations under the License.
package swim.api.lane;
import java.util.Iterator;
import java.util.Map;
import swim.api.downlink.ValueDownlink;
import swim.api.lane.function.DidDownlinkValue;
import swim.api.lane.function.WillDownlinkValue;
import swim.api.warp.WarpLane;
import swim.api.warp.function.DidCommand;
import swim.api.warp.function.DidEnter;
import swim.api.warp.function.DidLeave;
import swim.api.warp.function.DidUplink;
import swim.api.warp.function.WillCommand;
import swim.api.warp.function.WillEnter;
import swim.api.warp.function.WillLeave;
import swim.api.warp.function.WillUplink;
import swim.observable.ObservableMap;
import swim.observable.function.DidClear;
import swim.observable.function.DidRemoveKey;
import swim.observable.function.DidUpdateKey;
import swim.observable.function.WillClear;
import swim.observable.function.WillRemoveKey;
import swim.observable.function.WillUpdateKey;
import swim.structure.Form;
public interface JoinValueLane extends WarpLane, Iterable>, ObservableMap {
Form keyForm();
JoinValueLane keyForm(Form keyForm);
JoinValueLane keyClass(Class keyClass);
Form valueForm();
JoinValueLane valueForm(Form valueForm);
JoinValueLane valueClass(Class valueClass);
boolean isResident();
JoinValueLane isResident(boolean isResident);
boolean isTransient();
JoinValueLane isTransient(boolean isTransient);
@Override
JoinValueLane observe(Object observer);
@Override
JoinValueLane unobserve(Object observer);
JoinValueLane willDownlink(WillDownlinkValue willDownlink);
JoinValueLane didDownlink(DidDownlinkValue didDownlink);
@Override
JoinValueLane willUpdate(WillUpdateKey willUpdate);
@Override
JoinValueLane didUpdate(DidUpdateKey didUpdate);
@Override
JoinValueLane willRemove(WillRemoveKey willRemove);
@Override
JoinValueLane didRemove(DidRemoveKey didRemove);
@Override
JoinValueLane willClear(WillClear willClear);
@Override
JoinValueLane didClear(DidClear didClear);
@Override
JoinValueLane willCommand(WillCommand willCommand);
@Override
JoinValueLane didCommand(DidCommand didCommand);
@Override
JoinValueLane willUplink(WillUplink willUplink);
@Override
JoinValueLane didUplink(DidUplink didUplink);
@Override
JoinValueLane willEnter(WillEnter willEnter);
@Override
JoinValueLane didEnter(DidEnter didEnter);
@Override
JoinValueLane willLeave(WillLeave willLeave);
@Override
JoinValueLane didLeave(DidLeave didLeave);
ValueDownlink downlink(K key);
ValueDownlink> getDownlink(Object key);
Iterator keyIterator();
Iterator valueIterator();
Iterator>> downlinkIterator();
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy