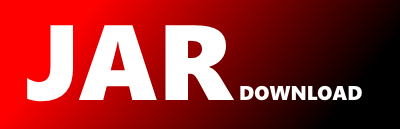
swim.api.policy.AbstractPolicy Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of swim-api Show documentation
Show all versions of swim-api Show documentation
Agent programming interface
// Copyright 2015-2021 Swim Inc.
//
// Licensed under the Apache License, Version 2.0 (the "License");
// you may not use this file except in compliance with the License.
// You may obtain a copy of the License at
//
// http://www.apache.org/licenses/LICENSE-2.0
//
// Unless required by applicable law or agreed to in writing, software
// distributed under the License is distributed on an "AS IS" BASIS,
// WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
// See the License for the specific language governing permissions and
// limitations under the License.
package swim.api.policy;
import swim.api.Downlink;
import swim.api.Lane;
import swim.api.Uplink;
import swim.api.agent.Agent;
import swim.api.agent.AgentRoute;
import swim.api.auth.Identity;
import swim.http.HttpMessage;
import swim.http.HttpRequest;
import swim.http.HttpResponse;
import swim.uri.Uri;
import swim.warp.CommandMessage;
import swim.warp.Envelope;
import swim.warp.EventMessage;
import swim.warp.LinkRequest;
import swim.warp.SyncRequest;
public class AbstractPolicy implements Policy, PlanePolicy, AgentRoutePolicy, AgentPolicy, LanePolicy, UplinkPolicy, DownlinkPolicy {
public AbstractPolicy() {
// nop
}
@Override
public AgentRoutePolicy agentRoutePolicy(AgentRoute> agentRoute) {
return this;
}
@Override
public AgentPolicy agentPolicy(Agent agent) {
return this;
}
@Override
public LanePolicy lanePolicy(Lane lane) {
return this;
}
@Override
public UplinkPolicy uplinkPolicy(Uplink uplink) {
return this;
}
@Override
public DownlinkPolicy downlinkPolicy(Downlink downlink) {
return this;
}
@Override
public PolicyDirective
© 2015 - 2025 Weber Informatics LLC | Privacy Policy