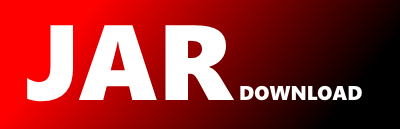
swim.csv.parser.CsvParser Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of swim-csv Show documentation
Show all versions of swim-csv Show documentation
Comma-Separated Values (CSV) codec that incrementally parses and writes swim-structure values
The newest version!
// Copyright 2015-2024 Nstream, inc.
//
// Licensed under the Apache License, Version 2.0 (the "License");
// you may not use this file except in compliance with the License.
// You may obtain a copy of the License at
//
// http://www.apache.org/licenses/LICENSE-2.0
//
// Unless required by applicable law or agreed to in writing, software
// distributed under the License is distributed on an "AS IS" BASIS,
// WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
// See the License for the specific language governing permissions and
// limitations under the License.
package swim.csv.parser;
import java.nio.ByteBuffer;
import swim.codec.Binary;
import swim.codec.Diagnostic;
import swim.codec.Input;
import swim.codec.Parser;
import swim.codec.Unicode;
import swim.codec.Utf8;
import swim.csv.schema.CsvCol;
import swim.csv.schema.CsvHeader;
public class CsvParser {
protected final int delimiter;
public CsvParser(int delimiter) {
this.delimiter = delimiter;
}
public final int delimiter() {
return this.delimiter;
}
public boolean isDelimiter(int c) {
return c == this.delimiter;
}
public Parser parseTable(Input input, CsvHeader header) {
return TableParser.parse(input, this, header);
}
public Parser> parseHeader(Input input, CsvHeader header) {
return HeaderParser.parse(input, this, header);
}
public Parser parseBody(Input input, CsvHeader header) {
return BodyParser.parse(input, this, header);
}
public Parser parseRow(Input input, CsvHeader header) {
return RowParser.parse(input, this, header);
}
public Parser parseCell(Input input, CsvCol col) {
return col.parseCell(input);
}
public Parser tableParser(CsvHeader header) {
return new TableParser(this, header);
}
public Parser> headerParser(CsvHeader header) {
return new HeaderParser(this, header);
}
public Parser bodyParser(CsvHeader header) {
return new BodyParser(this, header);
}
public Parser rowParser(CsvHeader header) {
return new RowParser(this, header);
}
public T parseTableString(CsvHeader header, String string) {
final Input input = Unicode.stringInput(string);
Parser parser = this.parseTable(input, header);
if (input.isCont() && !parser.isError()) {
parser = Parser.error(Diagnostic.unexpected(input));
} else if (input.isError()) {
parser = Parser.error(input.trap());
}
return parser.bind();
}
public T parseTableData(CsvHeader header, byte[] data) {
final Input input = Utf8.decodedInput(Binary.inputBuffer(data));
Parser parser = this.parseTable(input, header);
if (input.isCont() && !parser.isError()) {
parser = Parser.error(Diagnostic.unexpected(input));
} else if (input.isError()) {
parser = Parser.error(input.trap());
}
return parser.bind();
}
public T parseTableBuffer(CsvHeader header, ByteBuffer buffer) {
final Input input = Utf8.decodedInput(Binary.inputBuffer(buffer));
Parser parser = this.parseTable(input, header);
if (input.isCont() && !parser.isError()) {
parser = Parser.error(Diagnostic.unexpected(input));
} else if (input.isError()) {
parser = Parser.error(input.trap());
}
return parser.bind();
}
public T parseBodyString(CsvHeader header, String string) {
final Input input = Unicode.stringInput(string);
Parser parser = this.parseBody(input, header);
if (input.isCont() && !parser.isError()) {
parser = Parser.error(Diagnostic.unexpected(input));
} else if (input.isError()) {
parser = Parser.error(input.trap());
}
return parser.bind();
}
public T parseBodyData(CsvHeader header, byte[] data) {
final Input input = Utf8.decodedInput(Binary.inputBuffer(data));
Parser parser = this.parseBody(input, header);
if (input.isCont() && !parser.isError()) {
parser = Parser.error(Diagnostic.unexpected(input));
} else if (input.isError()) {
parser = Parser.error(input.trap());
}
return parser.bind();
}
public T parseBodyBuffer(CsvHeader header, ByteBuffer buffer) {
final Input input = Utf8.decodedInput(Binary.inputBuffer(buffer));
Parser parser = this.parseBody(input, header);
if (input.isCont() && !parser.isError()) {
parser = Parser.error(Diagnostic.unexpected(input));
} else if (input.isError()) {
parser = Parser.error(input.trap());
}
return parser.bind();
}
public R parseRowString(CsvHeader header, String string) {
Input input = Unicode.stringInput(string);
Parser parser = this.parseRow(input, header);
if (parser.isDone()) {
while (input.isCont()) {
final int c = input.head();
if (c == '\r' || c == '\n') {
input = input.step();
}
}
}
if (input.isCont() && !parser.isError()) {
parser = Parser.error(Diagnostic.unexpected(input));
} else if (input.isError()) {
parser = Parser.error(input.trap());
}
return parser.bind();
}
public R parseRowData(CsvHeader header, byte[] data) {
Input input = Utf8.decodedInput(Binary.inputBuffer(data));
Parser parser = this.parseRow(input, header);
if (parser.isDone()) {
while (input.isCont()) {
final int c = input.head();
if (c == '\r' || c == '\n') {
input = input.step();
}
}
}
if (input.isCont() && !parser.isError()) {
parser = Parser.error(Diagnostic.unexpected(input));
} else if (input.isError()) {
parser = Parser.error(input.trap());
}
return parser.bind();
}
public R parseRowBuffer(CsvHeader header, ByteBuffer buffer) {
Input input = Utf8.decodedInput(Binary.inputBuffer(buffer));
Parser parser = this.parseRow(input, header);
if (parser.isDone()) {
while (input.isCont()) {
final int c = input.head();
if (c == '\r' || c == '\n') {
input = input.step();
}
}
}
if (input.isCont() && !parser.isError()) {
parser = Parser.error(Diagnostic.unexpected(input));
} else if (input.isError()) {
parser = Parser.error(input.trap());
}
return parser.bind();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy