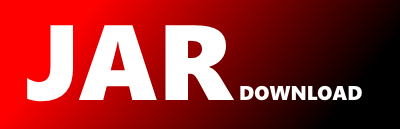
swim.mqtt.MqttDecoder Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of swim-mqtt Show documentation
Show all versions of swim-mqtt Show documentation
MQTT packet model and wire protocol codec that incrementally decodes and encodes MQTT streams without intermediate buffering
The newest version!
// Copyright 2015-2024 Nstream, inc.
//
// Licensed under the Apache License, Version 2.0 (the "License");
// you may not use this file except in compliance with the License.
// You may obtain a copy of the License at
//
// http://www.apache.org/licenses/LICENSE-2.0
//
// Unless required by applicable law or agreed to in writing, software
// distributed under the License is distributed on an "AS IS" BASIS,
// WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
// See the License for the specific language governing permissions and
// limitations under the License.
package swim.mqtt;
import swim.codec.Decoder;
import swim.codec.Encoder;
import swim.codec.InputBuffer;
import swim.collections.FingerTrieSeq;
import swim.structure.Data;
public class MqttDecoder {
public MqttDecoder() {
// nop
}
public MqttConnectPacket connectPacket(int packetFlags, String protocolName, int protocolLevel,
int connectFlags, int keepAlive, String clientId, String willTopic,
Data willMessage, String username, Data password) {
return MqttConnectPacket.create(packetFlags, protocolName, protocolLevel,
connectFlags, keepAlive, clientId, willTopic,
willMessage, username, password);
}
public MqttConnAckPacket connAckPacket(int packetFlags, int connectFlags, int connectCode) {
return MqttConnAckPacket.create(packetFlags, connectFlags, connectCode);
}
public MqttPublishPacket publishPacket(int packetFlags, String topicName, int packetId, T payloadValue) {
return MqttPublishPacket.create(packetFlags, topicName, packetId, payloadValue, Encoder.done(), 0);
}
public MqttPubAckPacket pubAckPacket(int packetFlags, int packetId) {
return MqttPubAckPacket.create(packetFlags, packetId);
}
public MqttPubRecPacket pubRecPacket(int packetFlags, int packetId) {
return MqttPubRecPacket.create(packetFlags, packetId);
}
public MqttPubRelPacket pubRelPacket(int packetFlags, int packetId) {
return MqttPubRelPacket.create(packetFlags, packetId);
}
public MqttPubCompPacket pubCompPacket(int packetFlags, int packetId) {
return MqttPubCompPacket.create(packetFlags, packetId);
}
public MqttSubscribePacket subscribePacket(int packetFlags, int packetId,
FingerTrieSeq subscriptions) {
return MqttSubscribePacket.create(packetFlags, packetId, subscriptions);
}
public MqttSubAckPacket subAckPacket(int packetFlags, int packetId,
FingerTrieSeq subscriptions) {
return MqttSubAckPacket.create(packetFlags, packetId, subscriptions);
}
public MqttUnsubscribePacket unsubscribePacket(int packetFlags, int packetId,
FingerTrieSeq topicNames) {
return MqttUnsubscribePacket.create(packetFlags, packetId, topicNames);
}
public MqttUnsubAckPacket unsubAckPacket(int packetFlags, int packetId) {
return MqttUnsubAckPacket.create(packetFlags, packetId);
}
public MqttPingReqPacket pingReqPacket(int packetFlags) {
return MqttPingReqPacket.create(packetFlags);
}
public MqttPingRespPacket pingRespPacket(int packetFlags) {
return MqttPingRespPacket.create(packetFlags);
}
public MqttDisconnectPacket disconnectPacket(int packetFlags) {
return MqttDisconnectPacket.create(packetFlags);
}
public MqttSubscription subscription(String topicName, int flags) {
return MqttSubscription.create(topicName, flags);
}
public MqttSubStatus subStatus(int code) {
return MqttSubStatus.create(code);
}
public Decoder> packetDecoder(Decoder payloadDecoder) {
return new MqttPacketDecoder(this, payloadDecoder);
}
public Decoder> decodePacket(InputBuffer input, Decoder payloadDecoder) {
return MqttPacketDecoder.decode(input, this, payloadDecoder);
}
@SuppressWarnings("unchecked")
public Decoder> decodePacketType(InputBuffer input, int packetType, Decoder payloadDecoder) {
final Decoder> decoder;
switch (packetType) {
case 1: decoder = this.decodeConnectPacket(input); break;
case 2: decoder = this.decodeConnAckPacket(input); break;
case 3: decoder = this.decodePublishPacket(input, payloadDecoder); break;
case 4: decoder = this.decodePubAckPacket(input); break;
case 5: decoder = this.decodePubRecPacket(input); break;
case 6: decoder = this.decodePubRelPacket(input); break;
case 7: decoder = this.decodePubCompPacket(input); break;
case 8: decoder = this.decodeSubscribePacket(input); break;
case 9: decoder = this.decodeSubAckPacket(input); break;
case 10: decoder = this.decodeUnsubscribePacket(input); break;
case 11: decoder = this.decodeUnsubAckPacket(input); break;
case 12: decoder = this.decodePingReqPacket(input); break;
case 13: decoder = this.decodePingRespPacket(input); break;
case 14: decoder = this.decodeDisconnectPacket(input); break;
default: return Decoder.error(new MqttException("reserved packet type: " + packetType));
}
return (Decoder>) decoder;
}
public Decoder decodeConnectPacket(InputBuffer input) {
return MqttConnectPacketDecoder.decode(input, this);
}
public Decoder decodeConnAckPacket(InputBuffer input) {
return MqttConnAckPacketDecoder.decode(input, this);
}
public Decoder> decodePublishPacket(InputBuffer input, Decoder payloadDecoder) {
return MqttPublishPacketDecoder.decode(input, this, payloadDecoder);
}
public Decoder decodePubAckPacket(InputBuffer input) {
return MqttPubAckPacketDecoder.decode(input, this);
}
public Decoder decodePubRecPacket(InputBuffer input) {
return MqttPubRecPacketDecoder.decode(input, this);
}
public Decoder decodePubRelPacket(InputBuffer input) {
return MqttPubRelPacketDecoder.decode(input, this);
}
public Decoder decodePubCompPacket(InputBuffer input) {
return MqttPubCompPacketDecoder.decode(input, this);
}
public Decoder decodeSubscribePacket(InputBuffer input) {
return MqttSubscribePacketDecoder.decode(input, this);
}
public Decoder decodeSubAckPacket(InputBuffer input) {
return MqttSubAckPacketDecoder.decode(input, this);
}
public Decoder decodeUnsubscribePacket(InputBuffer input) {
return MqttUnsubscribePacketDecoder.decode(input, this);
}
public Decoder decodeUnsubAckPacket(InputBuffer input) {
return MqttUnsubAckPacketDecoder.decode(input, this);
}
public Decoder decodePingReqPacket(InputBuffer input) {
return MqttPingReqPacketDecoder.decode(input, this);
}
public Decoder decodePingRespPacket(InputBuffer input) {
return MqttPingRespPacketDecoder.decode(input, this);
}
public Decoder decodeDisconnectPacket(InputBuffer input) {
return MqttDisconnectPacketDecoder.decode(input, this);
}
public Decoder subscriptionDecoder() {
return new MqttSubscriptionDecoder(this);
}
public Decoder decodeSubscription(InputBuffer input) {
return MqttSubscriptionDecoder.decode(input, this);
}
public Decoder stringDecoder() {
return new MqttStringDecoder();
}
public Decoder decodeString(InputBuffer input) {
return MqttStringDecoder.decode(input);
}
public Decoder dataDecoder() {
return new MqttDataDecoder();
}
public Decoder decodeData(InputBuffer input) {
return MqttDataDecoder.decode(input);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy