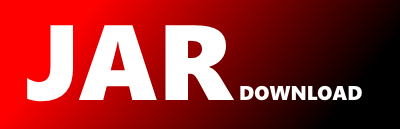
swim.spatial.QTreePage Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of swim-spatial Show documentation
Show all versions of swim-spatial Show documentation
Uploads all artifacts belonging to configuration ':swim-spatial:archives'
// Copyright 2015-2019 SWIM.AI inc.
//
// Licensed under the Apache License, Version 2.0 (the "License");
// you may not use this file except in compliance with the License.
// You may obtain a copy of the License at
//
// http://www.apache.org/licenses/LICENSE-2.0
//
// Unless required by applicable law or agreed to in writing, software
// distributed under the License is distributed on an "AS IS" BASIS,
// WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
// See the License for the specific language governing permissions and
// limitations under the License.
package swim.spatial;
import java.util.ArrayList;
import java.util.Collection;
import java.util.Comparator;
import swim.util.Cursor;
public abstract class QTreePage {
QTreePage() {
// stub
}
public abstract boolean isEmpty();
public abstract long span();
public abstract int arity();
public abstract QTreePage getPage(int index);
public abstract int slotCount();
public abstract QTreeEntry getSlot(int index);
public abstract long x();
public abstract int xRank();
public abstract long xBase();
public abstract long xMask();
public abstract long xSplit();
public abstract long y();
public abstract int yRank();
public abstract long yBase();
public abstract long yMask();
public abstract long ySplit();
public abstract boolean containsKey(K key, long xk, long yk, QTreeContext tree);
public boolean containsKey(K key, int xkRank, long xkBase, int ykRank, long ykBase, QTreeContext tree) {
final long x = BitInterval.from(xkRank, xkBase);
final long y = BitInterval.from(ykRank, ykBase);
return containsKey(key, x, y, tree);
}
public abstract V get(K key, long xk, long yk, QTreeContext tree);
public V get(K key, int xkRank, long xkBase, int ykRank, long ykBase, QTreeContext tree) {
final long x = BitInterval.from(xkRank, xkBase);
final long y = BitInterval.from(ykRank, ykBase);
return get(key, x, y, tree);
}
public Collection> getAll(long x, long y) {
final Collection> slots = new ArrayList>();
final Cursor> cursor = cursor(x, y);
while (cursor.hasNext()) {
slots.add(cursor.next());
}
return slots;
}
public Collection> getAll(long x0, long y0, long x1, long y1) {
final long x = BitInterval.span(x0, x1);
final long y = BitInterval.span(y0, y1);
return getAll(x, y);
}
abstract QTreePage updated(K key, S shape, long xk, long yk, V newValue,
QTreeContext tree, boolean canSplit);
public QTreePage updated(K key, S shape, long xk, long yk, V newValue, QTreeContext tree) {
return updated(key, shape, xk, yk, newValue, tree, true);
}
public QTreePage updated(K key, S shape, int xkRank, long xkBase,
int ykRank, long ykBase, V newValue, QTreeContext tree) {
final long xk = BitInterval.from(xkRank, xkBase);
final long yk = BitInterval.from(ykRank, ykBase);
return updated(key, shape, xk, yk, newValue, tree);
}
abstract QTreePage insertedPage(QTreePage newPage, QTreeContext tree);
abstract QTreePage mergedPage(QTreePage newPage, QTreeContext tree);
abstract QTreePage mergedSlots(QTreeEntry[] midSlots, QTreeContext tree);
abstract QTreePage updatedSlot(QTreeEntry newSlot, QTreeContext tree);
public abstract QTreePage removed(K key, long xk, long yk, QTreeContext tree);
public QTreePage removed(K key, int xkRank, long xkBase, int ykRank, long ykBase, QTreeContext tree) {
final long xk = BitInterval.from(xkRank, xkBase);
final long yk = BitInterval.from(ykRank, ykBase);
return removed(key, xk, yk, tree);
}
public abstract QTreePage flattened(QTreeContext tree);
public abstract QTreePage balanced(QTreeContext tree);
// Always returns QTreeNode, but we don't want to publicly expose it.
public abstract QTreePage split(QTreeContext tree);
public abstract Cursor> cursor(long x, long y);
public Cursor> cursor(long x0, long y0, long x1, long y1) {
final long x = BitInterval.span(x0, x1);
final long y = BitInterval.span(y0, y1);
return cursor(x, y);
}
public Cursor> cursor() {
return cursor(-1L, -1L);
}
static final QTreePage, ?, ?>[] EMPTY_PAGES = new QTreePage, ?, ?>[0];
static final QTreeEntry, ?, ?>[] EMPTY_SLOTS = new QTreeEntry, ?, ?>[0];
static final Comparator> PAGE_ORDERING = new QTreePageOrdering();
public static QTreePage empty() {
return QTreeLeaf.empty();
}
}
final class QTreePageOrdering implements Comparator> {
@Override
public int compare(QTreePage, ?, ?> a, QTreePage, ?, ?> b) {
return BitInterval.compare(a.x(), a.y(), b.x(), b.y());
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy