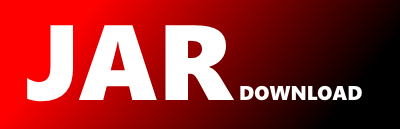
swim.system.downlink.EventDownlinkModel Maven / Gradle / Ivy
The newest version!
// Copyright 2015-2024 Nstream, inc.
//
// Licensed under the Apache License, Version 2.0 (the "License");
// you may not use this file except in compliance with the License.
// You may obtain a copy of the License at
//
// http://www.apache.org/licenses/LICENSE-2.0
//
// Unless required by applicable law or agreed to in writing, software
// distributed under the License is distributed on an "AS IS" BASIS,
// WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
// See the License for the specific language governing permissions and
// limitations under the License.
package swim.system.downlink;
import swim.concurrent.Cont;
import swim.structure.Form;
import swim.structure.Value;
import swim.system.DownlinkRelay;
import swim.system.Push;
import swim.system.warp.SupplyDownlinkModem;
import swim.uri.Uri;
import swim.warp.EventMessage;
public class EventDownlinkModel extends SupplyDownlinkModem> {
public EventDownlinkModel(Uri meshUri, Uri hostUri, Uri nodeUri, Uri laneUri,
float prio, float rate, Value body) {
super(meshUri, hostUri, nodeUri, laneUri, prio, rate, body);
}
@Override
protected void pushDownEvent(Push push) {
final EventMessage message = push.message();
this.onEvent(message);
new EventDownlinkRelayOnEvent(this, message, push.cont()).run();
}
public void command(Value body) {
this.pushUp(body);
}
}
final class EventDownlinkRelayOnEvent extends DownlinkRelay> {
final EventMessage message;
final Cont cont;
Form
© 2015 - 2025 Weber Informatics LLC | Privacy Policy