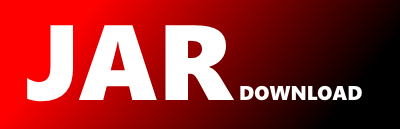
swim.system.lane.DemandMapLaneView Maven / Gradle / Ivy
The newest version!
// Copyright 2015-2024 Nstream, inc.
//
// Licensed under the Apache License, Version 2.0 (the "License");
// you may not use this file except in compliance with the License.
// You may obtain a copy of the License at
//
// http://www.apache.org/licenses/LICENSE-2.0
//
// Unless required by applicable law or agreed to in writing, software
// distributed under the License is distributed on an "AS IS" BASIS,
// WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
// See the License for the specific language governing permissions and
// limitations under the License.
package swim.system.lane;
import java.util.Iterator;
import swim.api.Lane;
import swim.api.Link;
import swim.api.SwimContext;
import swim.api.agent.AgentContext;
import swim.api.lane.DemandMapLane;
import swim.api.lane.function.OnCueKey;
import swim.api.lane.function.OnSyncKeys;
import swim.api.warp.WarpUplink;
import swim.api.warp.function.DidCommand;
import swim.api.warp.function.DidEnter;
import swim.api.warp.function.DidLeave;
import swim.api.warp.function.DidUplink;
import swim.api.warp.function.WillCommand;
import swim.api.warp.function.WillEnter;
import swim.api.warp.function.WillLeave;
import swim.api.warp.function.WillUplink;
import swim.concurrent.Cont;
import swim.structure.Form;
import swim.structure.Value;
import swim.system.warp.WarpLaneView;
public class DemandMapLaneView extends WarpLaneView implements DemandMapLane {
protected final AgentContext agentContext;
protected Form keyForm;
protected Form valueForm;
protected DemandMapLaneModel laneBinding;
public DemandMapLaneView(AgentContext agentContext, Form keyForm, Form valueForm, Object observers) {
super(observers);
this.agentContext = agentContext;
this.keyForm = keyForm;
this.valueForm = valueForm;
this.laneBinding = null;
}
public DemandMapLaneView(AgentContext agentContext, Form keyForm, Form valueForm) {
this(agentContext, keyForm, valueForm, null);
}
@Override
public AgentContext agentContext() {
return this.agentContext;
}
@Override
public DemandMapLaneModel laneBinding() {
return this.laneBinding;
}
void setLaneBinding(DemandMapLaneModel laneBinding) {
this.laneBinding = laneBinding;
}
@Override
public DemandMapLaneModel createLaneBinding() {
return new DemandMapLaneModel();
}
@Override
public final Form keyForm() {
return this.keyForm;
}
@Override
public DemandMapLaneView keyForm(Form keyForm) {
return new DemandMapLaneView(this.agentContext, keyForm, this.valueForm,
this.typesafeObservers(this.observers));
}
@Override
public DemandMapLaneView keyClass(Class keyClass) {
return this.keyForm(Form.forClass(keyClass));
}
public void setKeyForm(Form keyForm) {
this.keyForm = keyForm;
}
@Override
public final Form valueForm() {
return this.valueForm;
}
@Override
public DemandMapLaneView valueForm(Form valueForm) {
return new DemandMapLaneView(this.agentContext, this.keyForm, valueForm,
this.typesafeObservers(this.observers));
}
@Override
public DemandMapLaneView valueClass(Class valueClass) {
return this.valueForm(Form.forClass(valueClass));
}
public void setValueForm(Form valueForm) {
this.valueForm = valueForm;
}
protected Object typesafeObservers(Object observers) {
// TODO: filter out OnCueKey, OnSyncKeys
return observers;
}
@Override
public void close() {
this.laneBinding.closeLaneView(this);
}
@Override
public DemandMapLaneView observe(Object observer) {
super.observe(observer);
return this;
}
@Override
public DemandMapLaneView unobserve(Object observer) {
super.unobserve(observer);
return this;
}
@Override
public DemandMapLaneView onCue(OnCueKey onCue) {
return this.observe(onCue);
}
@Override
public DemandMapLaneView onSync(OnSyncKeys onSync) {
return this.observe(onSync);
}
@Override
public DemandMapLaneView willCommand(WillCommand willCommand) {
return this.observe(willCommand);
}
@Override
public DemandMapLaneView didCommand(DidCommand didCommand) {
return this.observe(didCommand);
}
@Override
public DemandMapLaneView willUplink(WillUplink willUplink) {
return this.observe(willUplink);
}
@Override
public DemandMapLaneView didUplink(DidUplink didUplink) {
return this.observe(didUplink);
}
@Override
public DemandMapLaneView willEnter(WillEnter willEnter) {
return this.observe(willEnter);
}
@Override
public DemandMapLaneView didEnter(DidEnter didEnter) {
return this.observe(didEnter);
}
@Override
public DemandMapLaneView willLeave(WillLeave willLeave) {
return this.observe(willLeave);
}
@Override
public DemandMapLaneView didLeave(DidLeave didLeave) {
return this.observe(didLeave);
}
@SuppressWarnings("unchecked")
public V dispatchOnCue(K key, WarpUplink uplink) {
final Lane lane = SwimContext.getLane();
final Link link = SwimContext.getLink();
SwimContext.setLane(this);
SwimContext.setLink(uplink);
try {
final Object observers = this.observers;
if (observers instanceof OnCueKey, ?>) {
try {
final V value = ((OnCueKey) observers).onCue(key, uplink);
if (value != null) {
return value;
}
} catch (Throwable error) {
if (Cont.isNonFatal(error)) {
this.laneDidFail(error);
}
throw error;
}
} else if (observers instanceof Object[]) {
final Object[] array = (Object[]) observers;
for (int i = 0, n = array.length; i < n; i += 1) {
final Object observer = array[i];
if (observer instanceof OnCueKey, ?>) {
try {
final V value = ((OnCueKey) observer).onCue(key, uplink);
if (value != null) {
return value;
}
} catch (Throwable error) {
if (Cont.isNonFatal(error)) {
this.laneDidFail(error);
}
throw error;
}
}
}
}
return null;
} finally {
SwimContext.setLink(link);
SwimContext.setLane(lane);
}
}
@SuppressWarnings("unchecked")
public Iterator dispatchOnSync(WarpUplink uplink) {
final Lane oldLane = SwimContext.getLane();
final Link oldLink = SwimContext.getLink();
SwimContext.setLane(this);
SwimContext.setLink(uplink);
try {
final Object observers = this.observers;
if (observers instanceof OnSyncKeys>) {
try {
final Iterator iterator = ((OnSyncKeys) observers).onSync(uplink);
if (iterator != null) {
return iterator;
}
} catch (Throwable error) {
if (Cont.isNonFatal(error)) {
this.laneDidFail(error);
}
throw error;
}
} else if (observers instanceof Object[]) {
final Object[] array = (Object[]) observers;
for (int i = 0, n = array.length; i < n; i += 1) {
final Object observer = array[i];
if (observer instanceof OnSyncKeys>) {
try {
final Iterator iterator = ((OnSyncKeys) observer).onSync(uplink);
if (iterator != null) {
return iterator;
}
} catch (Throwable error) {
if (Cont.isNonFatal(error)) {
this.laneDidFail(error);
}
throw error;
}
}
}
}
return null;
} finally {
SwimContext.setLink(oldLink);
SwimContext.setLane(oldLane);
}
}
Value nextDownCue(Value key, WarpUplink uplink) {
final K keyObject = this.keyForm.cast(key);
final V object = this.dispatchOnCue(keyObject, uplink);
if (object != null) {
return this.valueForm.mold(object).toValue();
} else {
return null;
}
}
@SuppressWarnings("unchecked")
Iterator syncKeys(WarpUplink uplink) {
final Iterator keyIterator = this.dispatchOnSync(uplink);
if (keyIterator != null) {
if (this.keyForm == Form.forValue() && this.valueForm == Form.forValue()) {
return (Iterator) (Iterator>) keyIterator;
} else {
return new DemandMapLaneKeyIterator(keyIterator, this.keyForm);
}
}
return null;
}
@Override
public void cue(K key) {
this.laneBinding.cueDownKey(this.keyForm.mold(key).toValue());
}
@Override
public void remove(K key) {
this.laneBinding.remove(this.keyForm.mold(key).toValue());
}
}
final class DemandMapLaneKeyIterator implements Iterator {
final Iterator inner;
final Form keyForm;
DemandMapLaneKeyIterator(Iterator inner, Form keyForm) {
this.inner = inner;
this.keyForm = keyForm;
}
@Override
public boolean hasNext() {
return this.inner.hasNext();
}
@Override
public Value next() {
return this.keyForm.mold(this.inner.next()).toValue();
}
@Override
public void remove() {
throw new UnsupportedOperationException();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy