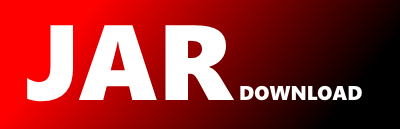
swim.system.lane.SpatialLaneView Maven / Gradle / Ivy
The newest version!
// Copyright 2015-2024 Nstream, inc.
//
// Licensed under the Apache License, Version 2.0 (the "License");
// you may not use this file except in compliance with the License.
// You may obtain a copy of the License at
//
// http://www.apache.org/licenses/LICENSE-2.0
//
// Unless required by applicable law or agreed to in writing, software
// distributed under the License is distributed on an "AS IS" BASIS,
// WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
// See the License for the specific language governing permissions and
// limitations under the License.
package swim.system.lane;
import java.util.AbstractMap;
import java.util.Iterator;
import java.util.Map;
import swim.api.Lane;
import swim.api.Link;
import swim.api.SwimContext;
import swim.api.agent.AgentContext;
import swim.api.data.SpatialData;
import swim.api.lane.SpatialLane;
import swim.api.warp.function.DidCommand;
import swim.api.warp.function.DidEnter;
import swim.api.warp.function.DidLeave;
import swim.api.warp.function.DidUplink;
import swim.api.warp.function.WillCommand;
import swim.api.warp.function.WillEnter;
import swim.api.warp.function.WillLeave;
import swim.api.warp.function.WillUplink;
import swim.concurrent.Cont;
import swim.math.Z2Form;
import swim.observable.function.DidClear;
import swim.observable.function.DidMoveShape;
import swim.observable.function.DidRemoveShape;
import swim.observable.function.DidUpdateShape;
import swim.observable.function.WillClear;
import swim.observable.function.WillMoveShape;
import swim.observable.function.WillRemoveShape;
import swim.observable.function.WillUpdateShape;
import swim.spatial.SpatialMap;
import swim.structure.Form;
import swim.system.LaneBinding;
import swim.system.warp.WarpLaneView;
public class SpatialLaneView extends WarpLaneView implements SpatialLane {
protected final AgentContext agentContext;
protected Form keyForm;
protected Z2Form shapeForm;
protected Form valueForm;
protected SpatialLaneModel laneBinding;
protected SpatialData dataView;
protected int flags;
SpatialLaneView(AgentContext agentContext, Form keyForm, Z2Form shapeForm, Form valueForm,
int flags, Object observers) {
super(observers);
this.agentContext = agentContext;
this.keyForm = keyForm;
this.shapeForm = shapeForm;
this.valueForm = valueForm;
this.laneBinding = null;
this.dataView = null;
this.flags = flags;
}
public SpatialLaneView(AgentContext agentContext, Form keyForm, Z2Form shapeForm, Form valueForm) {
this(agentContext, keyForm, shapeForm, valueForm, 0, null);
}
@Override
public AgentContext agentContext() {
return this.agentContext;
}
@Override
public SpatialLaneModel laneBinding() {
return this.laneBinding;
}
public void setLaneBinding(SpatialLaneModel laneBinding) {
this.laneBinding = laneBinding;
}
@Override
public LaneBinding createLaneBinding() {
return new SpatialLaneModel(this.shapeForm, this.flags);
}
@Override
public Form keyForm() {
return this.keyForm;
}
@Override
public SpatialLane keyForm(Form keyForm) {
return new SpatialLaneView(this.agentContext, keyForm, this.shapeForm, this.valueForm,
this.flags, this.typesafeObservers(this.observers));
}
@Override
public SpatialLane keyClass(Class keyClass) {
return this.keyForm(Form.forClass(keyClass));
}
public void setKeyForm(Form keyForm) {
this.keyForm = keyForm;
}
@Override
public Form valueForm() {
return this.valueForm;
}
@Override
public SpatialLane valueForm(Form valueForm) {
return new SpatialLaneView(this.agentContext, this.keyForm, this.shapeForm, valueForm,
this.flags, this.typesafeObservers(this.observers));
}
@Override
public SpatialLane valueClass(Class valueClass) {
return this.valueForm(Form.forClass(valueClass));
}
public void setValueForm(Form valueForm) {
this.valueForm = valueForm;
}
protected Object typesafeObservers(Object observers) {
// TODO: filter out WillUpdateKey, DidUpdateKey, WillRemoveKey, DidRemoveKey,
// WillDrop, DidDrop, WillTake, DidTake, WillClear, DidClear
return observers;
}
public final boolean isResident() {
return (this.flags & SpatialLaneView.RESIDENT) != 0;
}
@Override
public SpatialLane isResident(boolean isResident) {
if (isResident) {
this.flags |= SpatialLaneView.RESIDENT;
} else {
this.flags &= ~SpatialLaneView.RESIDENT;
}
final SpatialLaneModel laneBinding = this.laneBinding;
if (laneBinding != null) {
laneBinding.isResident(isResident);
}
return this;
}
void didSetResident(boolean isResident) {
if (isResident) {
this.flags |= SpatialLaneView.RESIDENT;
} else {
this.flags &= ~SpatialLaneView.RESIDENT;
}
}
public final boolean isTransient() {
return (this.flags & SpatialLaneView.TRANSIENT) != 0;
}
@Override
public SpatialLane isTransient(boolean isTransient) {
if (isTransient) {
this.flags |= SpatialLaneView.TRANSIENT;
} else {
this.flags &= ~SpatialLaneView.TRANSIENT;
}
final SpatialLaneModel laneBinding = this.laneBinding;
if (laneBinding != null) {
laneBinding.isTransient(isTransient);
}
return this;
}
void didSetTransient(boolean isTransient) {
if (isTransient) {
this.flags |= SpatialLaneView.TRANSIENT;
} else {
this.flags &= ~SpatialLaneView.TRANSIENT;
}
}
@Override
protected void willLoad() {
this.dataView = this.laneBinding.data.keyForm(this.keyForm).valueForm(this.valueForm);
super.willLoad();
}
@Override
public void close() {
this.laneBinding.closeLaneView(this);
}
@Override
public SpatialLaneView observe(Object observer) {
super.observe(observer);
return this;
}
@Override
public SpatialLaneView unobserve(Object observer) {
super.unobserve(observer);
return this;
}
@Override
public SpatialLane willUpdate(WillUpdateShape willUpdate) {
return this.observe(willUpdate);
}
@Override
public SpatialLane didUpdate(DidUpdateShape didUpdate) {
return this.observe(didUpdate);
}
@Override
public SpatialLane willMove(WillMoveShape willMove) {
return this.observe(willMove);
}
@Override
public SpatialLane didMove(DidMoveShape didMove) {
return this.observe(didMove);
}
@Override
public SpatialLane willRemove(WillRemoveShape willRemove) {
return this.observe(willRemove);
}
@Override
public SpatialLane didRemove(DidRemoveShape didRemove) {
return this.observe(didRemove);
}
@Override
public SpatialLane willClear(WillClear willClear) {
return this.observe(willClear);
}
@Override
public SpatialLane didClear(DidClear didClear) {
return this.observe(didClear);
}
@Override
public SpatialLaneView willCommand(WillCommand willCommand) {
return this.observe(willCommand);
}
@Override
public SpatialLaneView didCommand(DidCommand didCommand) {
return this.observe(didCommand);
}
@Override
public SpatialLaneView willUplink(WillUplink willUplink) {
return this.observe(willUplink);
}
@Override
public SpatialLaneView didUplink(DidUplink didUplink) {
return this.observe(didUplink);
}
@Override
public SpatialLaneView willEnter(WillEnter willEnter) {
return this.observe(willEnter);
}
@Override
public SpatialLaneView didEnter(DidEnter didEnter) {
return this.observe(didEnter);
}
@Override
public SpatialLaneView willLeave(WillLeave willLeave) {
return this.observe(willLeave);
}
@Override
public SpatialLaneView didLeave(DidLeave didLeave) {
return this.observe(didLeave);
}
@SuppressWarnings("unchecked")
public Map.Entry dispatchWillUpdate(Link link, K key, S shape, V newValue, boolean preemptive) {
final Lane oldLane = SwimContext.getLane();
final Link oldLink = SwimContext.getLink();
try {
SwimContext.setLane(this);
SwimContext.setLink(link);
final Object observers = this.observers;
boolean complete = true;
if (observers instanceof WillUpdateShape, ?, ?>) {
if (((WillUpdateShape, ?, ?>) observers).isPreemptive() == preemptive) {
try {
newValue = ((WillUpdateShape) observers).willUpdate(key, shape, newValue);
} catch (Throwable error) {
if (Cont.isNonFatal(error)) {
this.laneDidFail(error);
}
throw error;
}
} else if (preemptive) {
complete = false;
}
} else if (observers instanceof Object[]) {
final Object[] array = (Object[]) observers;
for (int i = 0, n = array.length; i < n; i += 1) {
final Object observer = array[i];
if (observer instanceof WillUpdateShape, ?, ?>) {
if (((WillUpdateShape, ?, ?>) observer).isPreemptive() == preemptive) {
try {
newValue = ((WillUpdateShape) observer).willUpdate(key, shape, newValue);
} catch (Throwable error) {
if (Cont.isNonFatal(error)) {
this.laneDidFail(error);
}
throw error;
}
} else if (preemptive) {
complete = false;
}
}
}
}
return new AbstractMap.SimpleImmutableEntry(complete, newValue);
} finally {
SwimContext.setLink(oldLink);
SwimContext.setLane(oldLane);
}
}
@SuppressWarnings("unchecked")
public boolean dispatchDidUpdate(Link link, K key, S shape, V newValue, V oldValue, boolean preemptive) {
final Lane oldLane = SwimContext.getLane();
final Link oldLink = SwimContext.getLink();
try {
SwimContext.setLane(this);
SwimContext.setLink(link);
final Object observers = this.observers;
boolean complete = true;
if (observers instanceof DidUpdateShape, ?, ?>) {
if (((DidUpdateShape, ?, ?>) observers).isPreemptive() == preemptive) {
try {
((DidUpdateShape) observers).didUpdate(key, shape, newValue, oldValue);
} catch (Throwable error) {
if (Cont.isNonFatal(error)) {
this.laneDidFail(error);
}
throw error;
}
} else if (preemptive) {
complete = false;
}
} else if (observers instanceof Object[]) {
final Object[] array = (Object[]) observers;
for (int i = 0, n = array.length; i < n; i += 1) {
final Object observer = array[i];
if (observer instanceof DidUpdateShape, ?, ?>) {
if (((DidUpdateShape, ?, ?>) observer).isPreemptive() == preemptive) {
try {
((DidUpdateShape) observer).didUpdate(key, shape, newValue, oldValue);
} catch (Throwable error) {
if (Cont.isNonFatal(error)) {
this.laneDidFail(error);
}
throw error;
}
} else if (preemptive) {
complete = false;
}
}
}
}
return complete;
} finally {
SwimContext.setLink(oldLink);
SwimContext.setLane(oldLane);
}
}
@SuppressWarnings("unchecked")
public Map.Entry dispatchWillMove(Link link, K key, S newShape, V newValue, S oldShape,
boolean preemptive) {
final Lane oldLane = SwimContext.getLane();
final Link oldLink = SwimContext.getLink();
try {
SwimContext.setLane(this);
SwimContext.setLink(link);
final Object observers = this.observers;
boolean complete = true;
if (observers instanceof WillMoveShape, ?, ?>) {
if (((WillMoveShape, ?, ?>) observers).isPreemptive() == preemptive) {
try {
newValue = ((WillMoveShape) observers).willMove(key, newShape, newValue, oldShape);
} catch (Throwable error) {
if (Cont.isNonFatal(error)) {
this.laneDidFail(error);
}
throw error;
}
} else if (preemptive) {
complete = false;
}
} else if (observers instanceof Object[]) {
final Object[] array = (Object[]) observers;
for (int i = 0, n = array.length; i < n; i += 1) {
final Object observer = array[i];
if (observer instanceof WillMoveShape, ?, ?>) {
if (((WillMoveShape, ?, ?>) observer).isPreemptive() == preemptive) {
try {
newValue = ((WillMoveShape) observer).willMove(key, newShape, newValue, oldShape);
} catch (Throwable error) {
if (Cont.isNonFatal(error)) {
this.laneDidFail(error);
}
throw error;
}
} else if (preemptive) {
complete = false;
}
}
}
}
return new AbstractMap.SimpleImmutableEntry(complete, newValue);
} finally {
SwimContext.setLink(oldLink);
SwimContext.setLane(oldLane);
}
}
@SuppressWarnings("unchecked")
public boolean dispatchDidMove(Link link, K key, S newShape, V newValue, S oldShape, V oldValue,
boolean preemptive) {
final Lane oldLane = SwimContext.getLane();
final Link oldLink = SwimContext.getLink();
try {
SwimContext.setLane(this);
SwimContext.setLink(link);
final Object observers = this.observers;
boolean complete = true;
if (observers instanceof WillMoveShape, ?, ?>) {
if (((DidMoveShape, ?, ?>) observers).isPreemptive() == preemptive) {
try {
((DidMoveShape) observers).didMove(key, newShape, newValue, oldShape, oldValue);
} catch (Throwable error) {
if (Cont.isNonFatal(error)) {
this.laneDidFail(error);
}
throw error;
}
} else if (preemptive) {
complete = false;
}
} else if (observers instanceof Object[]) {
final Object[] array = (Object[]) observers;
for (int i = 0, n = array.length; i < n; i += 1) {
final Object observer = array[i];
if (observer instanceof WillMoveShape, ?, ?>) {
if (((DidMoveShape, ?, ?>) observer).isPreemptive() == preemptive) {
try {
((DidMoveShape) observer).didMove(key, newShape, newValue, oldShape, oldValue);
} catch (Throwable error) {
if (Cont.isNonFatal(error)) {
this.laneDidFail(error);
}
throw error;
}
} else if (preemptive) {
complete = false;
}
}
}
}
return complete;
} finally {
SwimContext.setLink(oldLink);
SwimContext.setLane(oldLane);
}
}
@SuppressWarnings("unchecked")
public boolean dispatchWillRemove(Link link, K key, S shape, boolean preemptive) {
final Lane oldLane = SwimContext.getLane();
final Link oldLink = SwimContext.getLink();
try {
SwimContext.setLane(this);
SwimContext.setLink(link);
final Object observers = this.observers;
boolean complete = true;
if (observers instanceof WillMoveShape, ?, ?>) {
if (((WillRemoveShape, ?>) observers).isPreemptive() == preemptive) {
try {
((WillRemoveShape) observers).willRemove(key, shape);
} catch (Throwable error) {
if (Cont.isNonFatal(error)) {
this.laneDidFail(error);
}
throw error;
}
} else if (preemptive) {
complete = false;
}
} else if (observers instanceof Object[]) {
final Object[] array = (Object[]) observers;
for (int i = 0, n = array.length; i < n; i += 1) {
final Object observer = array[i];
if (observer instanceof WillMoveShape, ?, ?>) {
if (((WillRemoveShape, ?>) observers).isPreemptive() == preemptive) {
try {
((WillRemoveShape) observers).willRemove(key, shape);
} catch (Throwable error) {
if (Cont.isNonFatal(error)) {
this.laneDidFail(error);
}
throw error;
}
} else if (preemptive) {
complete = false;
}
}
}
}
return complete;
} finally {
SwimContext.setLink(oldLink);
SwimContext.setLane(oldLane);
}
}
@SuppressWarnings("unchecked")
public boolean dispatchDidRemove(Link link, K key, S shape, V oldValue, boolean preemptive) {
final Lane oldLane = SwimContext.getLane();
final Link oldLink = SwimContext.getLink();
try {
SwimContext.setLane(this);
SwimContext.setLink(link);
final Object observers = this.observers;
boolean complete = true;
if (observers instanceof WillMoveShape, ?, ?>) {
if (((DidRemoveShape, ?, ?>) observers).isPreemptive() == preemptive) {
try {
((DidRemoveShape) observers).didRemove(key, shape, oldValue);
} catch (Throwable error) {
if (Cont.isNonFatal(error)) {
this.laneDidFail(error);
}
throw error;
}
} else if (preemptive) {
complete = false;
}
} else if (observers instanceof Object[]) {
final Object[] array = (Object[]) observers;
for (int i = 0, n = array.length; i < n; i += 1) {
final Object observer = array[i];
if (observer instanceof WillMoveShape, ?, ?>) {
if (((DidRemoveShape, ?, ?>) observers).isPreemptive() == preemptive) {
try {
((DidRemoveShape) observers).didRemove(key, shape, oldValue);
} catch (Throwable error) {
if (Cont.isNonFatal(error)) {
this.laneDidFail(error);
}
throw error;
}
} else if (preemptive) {
complete = false;
}
}
}
}
return complete;
} finally {
SwimContext.setLink(oldLink);
SwimContext.setLane(oldLane);
}
}
public boolean dispatchWillClear(Link link, boolean preemptive) {
final Lane oldLane = SwimContext.getLane();
final Link oldLink = SwimContext.getLink();
try {
SwimContext.setLane(this);
SwimContext.setLink(link);
final Object observers = this.observers;
boolean complete = true;
if (observers instanceof WillClear) {
if (((WillClear) observers).isPreemptive() == preemptive) {
try {
((WillClear) observers).willClear();
} catch (Throwable error) {
if (Cont.isNonFatal(error)) {
this.laneDidFail(error);
}
throw error;
}
} else if (preemptive) {
complete = false;
}
} else if (observers instanceof Object[]) {
final Object[] array = (Object[]) observers;
for (int i = 0, n = array.length; i < n; i += 1) {
final Object observer = array[i];
if (observer instanceof WillClear) {
if (((WillClear) observer).isPreemptive() == preemptive) {
try {
((WillClear) observer).willClear();
} catch (Throwable error) {
if (Cont.isNonFatal(error)) {
this.laneDidFail(error);
}
throw error;
}
} else if (preemptive) {
complete = false;
}
}
}
}
return complete;
} finally {
SwimContext.setLink(oldLink);
SwimContext.setLane(oldLane);
}
}
public boolean dispatchDidClear(Link link, boolean preemptive) {
final Lane oldLane = SwimContext.getLane();
final Link oldLink = SwimContext.getLink();
try {
SwimContext.setLane(this);
SwimContext.setLink(link);
final Object observers = this.observers;
boolean complete = true;
if (observers instanceof DidClear) {
if (((DidClear) observers).isPreemptive() == preemptive) {
try {
((DidClear) observers).didClear();
} catch (Throwable error) {
if (Cont.isNonFatal(error)) {
this.laneDidFail(error);
}
throw error;
}
} else if (preemptive) {
complete = false;
}
} else if (observers instanceof Object[]) {
final Object[] array = (Object[]) observers;
for (int i = 0, n = array.length; i < n; i += 1) {
final Object observer = array[i];
if (observer instanceof DidClear) {
if (((DidClear) observer).isPreemptive() == preemptive) {
try {
((DidClear) observer).didClear();
} catch (Throwable error) {
if (Cont.isNonFatal(error)) {
this.laneDidFail(error);
}
throw error;
}
} else if (preemptive) {
complete = false;
}
}
}
}
return complete;
} finally {
SwimContext.setLink(oldLink);
SwimContext.setLane(oldLane);
}
}
public V laneWillUpdate(K key, S shape, V newValue) {
return newValue;
}
public void laneDidUpdate(K key, S shape, V newValue, V oldValue) {
// hook
}
public V laneWillMove(K key, S newShape, V newValue, S oldShape) {
return newValue;
}
public V laneDidMove(K key, S newShape, V newValue, S oldShape, V oldValue) {
return newValue;
}
public void laneWillRemove(K key, S shape) {
// hook
}
public void laneDidRemove(K key, S shape) {
// hook
}
public void laneWillClear() {
// hook
}
public void laneDidClear() {
// hook
}
@Override
public SpatialMap snapshot() {
return this.dataView.snapshot();
}
@Override
public Iterator> iterator() {
return this.dataView.iterator();
}
@Override
public boolean isEmpty() {
return this.dataView.isEmpty();
}
@Override
public int size() {
return this.dataView.size();
}
@Override
public boolean containsKey(K key, S shape) {
return this.dataView.containsKey(key, shape);
}
@Override
public boolean containsKey(Object key) {
return this.dataView.containsKey(key);
}
@Override
public boolean containsValue(Object value) {
return this.dataView.containsValue(value);
}
@Override
public V get(K key, S shape) {
return this.dataView.get(key, shape);
}
@Override
public V get(Object key) {
return this.dataView.get(key);
}
@Override
public V put(K key, S shape, V newValue) {
return this.dataView.put(key, shape, newValue);
}
@Override
public V move(K key, S oldShape, S newShape, V newValue) {
return this.dataView.move(key, oldShape, newShape, newValue);
}
@Override
public V remove(K key, S shape) {
return this.dataView.remove(key, shape);
}
@Override
public void clear() {
this.dataView.clear();
}
@Override
public Iterator> iterator(S shape) {
return this.dataView.iterator(shape);
}
@Override
public Iterator keyIterator() {
return this.dataView.keyIterator();
}
@Override
public Iterator valueIterator() {
return this.dataView.valueIterator();
}
static final int RESIDENT = 1 << 0;
static final int TRANSIENT = 1 << 1;
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy