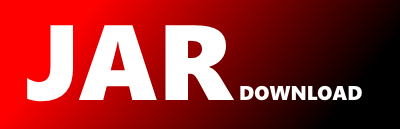
swim.system.lane.ValueLaneModel Maven / Gradle / Ivy
The newest version!
// Copyright 2015-2024 Nstream, inc.
//
// Licensed under the Apache License, Version 2.0 (the "License");
// you may not use this file except in compliance with the License.
// You may obtain a copy of the License at
//
// http://www.apache.org/licenses/LICENSE-2.0
//
// Unless required by applicable law or agreed to in writing, software
// distributed under the License is distributed on an "AS IS" BASIS,
// WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
// See the License for the specific language governing permissions and
// limitations under the License.
package swim.system.lane;
import java.util.Map;
import swim.api.Link;
import swim.api.data.ValueData;
import swim.concurrent.Cont;
import swim.concurrent.Stage;
import swim.structure.Form;
import swim.structure.Value;
import swim.system.LaneModel;
import swim.system.LaneRelay;
import swim.system.LaneView;
import swim.system.Push;
import swim.system.WarpBinding;
import swim.system.warp.WarpLaneModel;
import swim.warp.CommandMessage;
public class ValueLaneModel extends WarpLaneModel, ValueLaneUplink> {
protected int flags;
protected ValueData data;
ValueLaneModel(int flags) {
this.flags = flags;
this.data = null;
}
public ValueLaneModel() {
this(0);
}
@Override
public String laneType() {
return "value";
}
@Override
protected ValueLaneUplink createWarpUplink(WarpBinding link) {
return new ValueLaneUplink(this, link, createUplinkAddress(link));
}
@Override
protected void didOpenLaneView(ValueLaneView> view) {
view.setLaneBinding(this);
}
@Override
public void onCommand(Push push) {
this.awaitStart();
final CommandMessage message = push.message();
final Value value = message.body();
new ValueLaneRelaySet(this, message, push.cont(), value).run();
}
public final boolean isResident() {
return (this.flags & ValueLaneModel.RESIDENT) != 0;
}
public ValueLaneModel isResident(boolean isResident) {
if (this.data != null) {
this.data.isResident(isResident);
}
if (isResident) {
this.flags |= ValueLaneModel.RESIDENT;
} else {
this.flags &= ~ValueLaneModel.RESIDENT;
}
final Object views = LaneModel.VIEWS.get(this);
if (views instanceof ValueLaneView>) {
((ValueLaneView>) views).didSetResident(isResident);
} else if (views instanceof LaneView[]) {
for (LaneView aViewArray : (LaneView[]) views) {
((ValueLaneView>) aViewArray).didSetResident(isResident);
}
}
return this;
}
public final boolean isTransient() {
return (this.flags & ValueLaneModel.TRANSIENT) != 0;
}
public ValueLaneModel isTransient(boolean isTransient) {
if (this.data != null) {
this.data.isTransient(isTransient);
}
if (isTransient) {
this.flags |= ValueLaneModel.TRANSIENT;
} else {
this.flags &= ~ValueLaneModel.TRANSIENT;
}
final Object views = LaneModel.VIEWS.get(this);
if (views instanceof ValueLaneView>) {
((ValueLaneView>) views).didSetTransient(isTransient);
} else if (views instanceof LaneView[]) {
final LaneView[] viewArray = (LaneView[]) views;
for (int i = 0, n = viewArray.length; i < n; i += 1) {
((ValueLaneView>) viewArray[i]).didSetTransient(isTransient);
}
}
return this;
}
public Value get() {
return this.data.get();
}
@SuppressWarnings("unchecked")
public V set(ValueLaneView view, V newObject) {
final Form valueForm = view.valueForm;
final Value newValue = valueForm.mold(newObject).toValue();
final ValueLaneRelaySet relay = new ValueLaneRelaySet(this, this.stage(), newValue);
relay.valueForm = (Form
© 2015 - 2025 Weber Informatics LLC | Privacy Policy