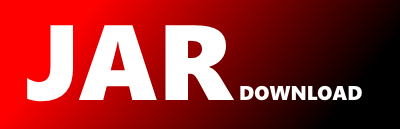
swim.system.reflect.HostPulse Maven / Gradle / Ivy
The newest version!
// Copyright 2015-2024 Nstream, inc.
//
// Licensed under the Apache License, Version 2.0 (the "License");
// you may not use this file except in compliance with the License.
// You may obtain a copy of the License at
//
// http://www.apache.org/licenses/LICENSE-2.0
//
// Unless required by applicable law or agreed to in writing, software
// distributed under the License is distributed on an "AS IS" BASIS,
// WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
// See the License for the specific language governing permissions and
// limitations under the License.
package swim.system.reflect;
import swim.structure.Form;
import swim.structure.Item;
import swim.structure.Kind;
import swim.structure.Record;
import swim.structure.Value;
public class HostPulse extends Pulse {
protected final long nodeCount;
protected final AgentPulse agents;
protected final WarpDownlinkPulse downlinks;
protected final WarpUplinkPulse uplinks;
protected final SystemPulse system;
public HostPulse(long nodeCount, AgentPulse agents,
WarpDownlinkPulse downlinks, WarpUplinkPulse uplinks, SystemPulse system) {
this.nodeCount = nodeCount;
this.agents = agents;
this.downlinks = downlinks;
this.uplinks = uplinks;
this.system = system;
}
@Override
public boolean isDefined() {
return this.nodeCount != 0L || this.agents.isDefined()
|| this.downlinks.isDefined() || this.uplinks.isDefined() || this.system.isDefined();
}
public final long nodeCount() {
return this.nodeCount;
}
public final AgentPulse agents() {
return this.agents;
}
public final WarpDownlinkPulse downlinks() {
return this.downlinks;
}
public final WarpUplinkPulse uplinks() {
return this.uplinks;
}
public SystemPulse system() {
return this.system;
}
@Override
public Value toValue() {
return HostPulse.form().mold(this).toValue();
}
private static Form form;
@Kind
public static Form form() {
if (HostPulse.form == null) {
HostPulse.form = new HostPulseForm();
}
return HostPulse.form;
}
}
final class HostPulseForm extends Form {
@Override
public Class> type() {
return HostPulse.class;
}
@Override
public Item mold(HostPulse pulse) {
if (pulse != null) {
final Record record = Record.create(4);
if (pulse.nodeCount > 0L) {
record.slot("nodeCount", pulse.nodeCount);
}
if (pulse.agents.isDefined()) {
record.slot("agents", pulse.agents.toValue());
}
if (pulse.downlinks.isDefined()) {
record.slot("downlinks", pulse.downlinks.toValue());
}
if (pulse.uplinks.isDefined()) {
record.slot("uplinks", pulse.uplinks.toValue());
}
if (pulse.system != null && pulse.system.isDefined()) {
record.slot("system", pulse.system.toValue());
}
return record;
} else {
return Item.extant();
}
}
@Override
public HostPulse cast(Item item) {
final Value value = item.toValue();
final long nodeCount = value.get("nodeCount").longValue(0L);
final AgentPulse agents = value.get("agents").coerce(AgentPulse.form());
final WarpDownlinkPulse downlinks = value.get("downlinks").coerce(WarpDownlinkPulse.form());
final WarpUplinkPulse uplinks = value.get("uplinks").coerce(WarpUplinkPulse.form());
final SystemPulse system = value.get("system").coerce(SystemPulse.form());
return new HostPulse(nodeCount, agents, downlinks, uplinks, system);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy