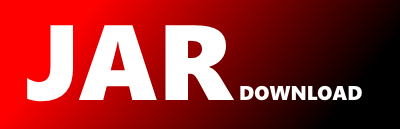
org.jdesktop.beans.editors.EnumerationValuePropertyEditor Maven / Gradle / Ivy
/*
* EnumerationValuePropertyEditor.java
*
* Created on March 28, 2006, 3:49 PM
*
* To change this template, choose Tools | Template Manager
* and open the template in the editor.
*/
package org.jdesktop.beans.editors;
import java.beans.PropertyEditorSupport;
import java.util.HashMap;
import java.util.Map;
import org.jdesktop.beans.EnumerationValue;
/**
*
* @author Richard
*/
public abstract class EnumerationValuePropertyEditor extends PropertyEditorSupport {
private String[] tags;
private Map
© 2015 - 2025 Weber Informatics LLC | Privacy Policy