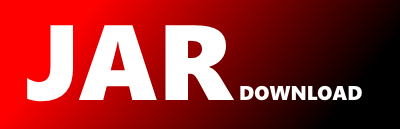
org.swisspush.mirror.ZipFileEntryIterator Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of rest-mirror Show documentation
Show all versions of rest-mirror Show documentation
A verticle that mirrors resources, which are provided as zip into a rest storage.
package org.swisspush.mirror;
import io.vertx.core.logging.Logger;
import java.io.*;
import java.net.URL;
import java.util.*;
import java.util.zip.ZipEntry;
import java.util.zip.ZipInputStream;
/**
* Iterates over the content of a zip.
* The zip can be provided as InputStream or as URL.
* Only files are considered as valid zip entries.
*
* @author: Florian Kammermann
*/
public class ZipFileEntryIterator implements Iterator
© 2015 - 2025 Weber Informatics LLC | Privacy Policy