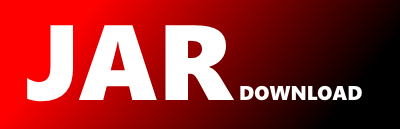
org.switchyard.rhq.plugin.SwitchYardResourceComponent Maven / Gradle / Ivy
The newest version!
/*
* Copyright 2013 Red Hat Inc. and/or its affiliates and other contributors.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
* http://www.apache.org/licenses/LICENSE-2.0
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.switchyard.rhq.plugin;
import java.util.Map;
import java.util.Set;
import java.util.concurrent.atomic.AtomicLong;
import java.util.concurrent.atomic.AtomicReference;
import org.apache.commons.logging.Log;
import org.apache.commons.logging.LogFactory;
import org.rhq.core.domain.configuration.Configuration;
import org.rhq.core.domain.measurement.AvailabilityType;
import org.rhq.core.domain.measurement.MeasurementDataNumeric;
import org.rhq.core.domain.measurement.MeasurementReport;
import org.rhq.core.domain.measurement.MeasurementScheduleRequest;
import org.rhq.core.pluginapi.measurement.MeasurementFacet;
import org.rhq.core.pluginapi.operation.OperationFacet;
import org.rhq.core.pluginapi.operation.OperationResult;
import org.rhq.modules.plugins.jbossas7.json.Address;
import org.rhq.modules.plugins.jbossas7.BaseComponent;
import org.rhq.modules.plugins.jbossas7.BaseServerComponent;
import org.rhq.modules.plugins.jbossas7.ManagedASComponent;
import org.rhq.modules.plugins.jbossas7.json.Operation;
import org.switchyard.rhq.plugin.model.Application;
import org.switchyard.rhq.plugin.model.ComponentServiceMetrics;
import org.switchyard.rhq.plugin.model.ModelUtil;
import org.switchyard.rhq.plugin.model.ReferenceMetrics;
import org.switchyard.rhq.plugin.model.ServiceMetrics;
import org.switchyard.rhq.plugin.model.SwitchYardMetrics;
import org.switchyard.rhq.plugin.operations.ReadApplication;
import org.switchyard.rhq.plugin.operations.ReadComponentServiceMetrics;
import org.switchyard.rhq.plugin.operations.ReadReferenceMetrics;
import org.switchyard.rhq.plugin.operations.ReadServiceMetrics;
import org.switchyard.rhq.plugin.operations.ReadSwitchYardMetrics;
import org.switchyard.rhq.plugin.operations.ResetMetrics;
import static org.switchyard.rhq.plugin.SwitchYardConstants.DMR_GET_VERSION;
import static org.switchyard.rhq.plugin.SwitchYardConstants.OPERATION_RESET;
import static org.switchyard.rhq.plugin.SwitchYardConstants.DMR_READ_APPLICATION;
import static org.switchyard.rhq.plugin.SwitchYardConstants.DMR_READ_REFERENCE;
import static org.switchyard.rhq.plugin.SwitchYardConstants.DMR_READ_SERVICE;
import static org.switchyard.rhq.plugin.SwitchYardConstants.DMR_SHOW_METRICS;
import static org.switchyard.rhq.plugin.SwitchYardConstants.PARAM_SERVICE_NAME;
import static org.switchyard.rhq.plugin.SwitchYardConstants.PARAM_TYPE;
/**
* SwitchYard Component
*/
public class SwitchYardResourceComponent extends BaseSwitchYardResourceComponent> implements MeasurementFacet, OperationFacet {
/**
* The logger instance.
*/
private static Log LOG = LogFactory.getLog(SwitchYardResourceComponent.class);
// TODO, make configurable
private static long REFRESH = 30*1000;
/**
* The current application map
*/
private AtomicReference
© 2015 - 2025 Weber Informatics LLC | Privacy Policy