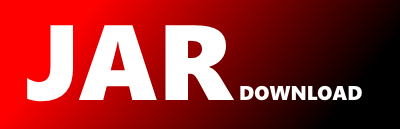
org.jdesktop.el.impl.parser.AstIdentifier Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of swixml Show documentation
Show all versions of swixml Show documentation
GUI generating engine for Java applications
/*
* Copyright (C) 2007 Sun Microsystems, Inc. All rights reserved. Use is
* subject to license terms.
*
*//* Generated By:JJTree: Do not edit this line. AstIdentifier.java */
package org.jdesktop.el.impl.parser;
import org.jdesktop.el.ELContext;
import org.jdesktop.el.ELException;
import org.jdesktop.el.Expression;
import org.jdesktop.el.MethodExpression;
import org.jdesktop.el.MethodInfo;
import org.jdesktop.el.MethodNotFoundException;
import org.jdesktop.el.ValueExpression;
import org.jdesktop.el.VariableMapper;
import org.jdesktop.el.impl.lang.EvaluationContext;
/**
* @author Jacob Hookom [[email protected]]
* @version $Change: 181177 $$DateTime: 2001/06/26 08:45:09 $$Author: kchung $
*/
public final class AstIdentifier extends SimpleNode {
public AstIdentifier(int id) {
super(id);
}
public Class getType(EvaluationContext ctx) throws ELException {
VariableMapper varMapper = ctx.getVariableMapper();
if (varMapper != null) {
ValueExpression expr = varMapper.resolveVariable(this.image);
if (expr != null) {
return expr.getType(ctx.getELContext());
}
}
ctx.setPropertyResolved(false);
return ctx.getELResolver().getType(ctx, getSource(ctx), this.image);
}
public Object getValue(EvaluationContext ctx) throws ELException {
VariableMapper varMapper = ctx.getVariableMapper();
if (varMapper != null) {
ValueExpression expr = varMapper.resolveVariable(this.image);
if (expr != null) {
return expr.getValue(ctx.getELContext());
}
}
ctx.setPropertyResolved(false);
Object source = getSource(ctx);
Object retVal = ctx.getELResolver().getValue(ctx, source, this.image);
if (retVal != ELContext.UNRESOLVABLE_RESULT) {
ctx.resolvedIdentifier(source, this.image);
}
return retVal;
}
public boolean isReadOnly(EvaluationContext ctx) throws ELException {
VariableMapper varMapper = ctx.getVariableMapper();
if (varMapper != null) {
ValueExpression expr = varMapper.resolveVariable(this.image);
if (expr != null) {
return expr.isReadOnly(ctx.getELContext());
}
}
ctx.setPropertyResolved(false);
return ctx.getELResolver().isReadOnly(ctx, getSource(ctx), this.image);
}
public void setValue(EvaluationContext ctx, Object value)
throws ELException {
VariableMapper varMapper = ctx.getVariableMapper();
if (varMapper != null) {
ValueExpression expr = varMapper.resolveVariable(this.image);
if (expr != null) {
expr.setValue(ctx.getELContext(), value);
return;
}
}
ctx.setPropertyResolved(false);
ctx.getELResolver().setValue(ctx, getSource(ctx), this.image, value);
}
private final Object invokeTarget(EvaluationContext ctx, Object target,
Object[] paramValues) throws ELException {
if (target instanceof MethodExpression) {
MethodExpression me = (MethodExpression) target;
return me.invoke(ctx.getELContext(), paramValues);
} else if (target == null) {
throw new MethodNotFoundException("Identity '" + this.image
+ "' was null and was unable to invoke");
} else {
throw new ELException(
"Identity '"
+ this.image
+ "' does not reference a MethodExpression instance, returned type: "
+ target.getClass().getName());
}
}
public Object invoke(EvaluationContext ctx, Class[] paramTypes,
Object[] paramValues) throws ELException {
return this.getMethodExpression(ctx).invoke(ctx.getELContext(), paramValues);
}
public MethodInfo getMethodInfo(EvaluationContext ctx, Class[] paramTypes)
throws ELException {
return this.getMethodExpression(ctx).getMethodInfo(ctx.getELContext());
}
private final MethodExpression getMethodExpression(EvaluationContext ctx)
throws ELException {
Object obj = null;
// case A: ValueExpression exists, getValue which must
// be a MethodExpression
VariableMapper varMapper = ctx.getVariableMapper();
ValueExpression ve = null;
if (varMapper != null) {
ve = varMapper.resolveVariable(this.image);
if (ve != null) {
obj = ve.getValue(ctx);
}
}
// case B: evaluate the identity against the ELResolver, again, must be
// a MethodExpression to be able to invoke
if (ve == null) {
ctx.setPropertyResolved(false);
obj = ctx.getELResolver().getValue(ctx, null, this.image);
}
// finally provide helpful hints
if (obj instanceof MethodExpression) {
return (MethodExpression) obj;
} else if (obj == null) {
throw new MethodNotFoundException("Identity '" + this.image
+ "' was null and was unable to invoke");
} else {
throw new ELException(
"Identity '"
+ this.image
+ "' does not reference a MethodExpression instance, returned type: "
+ obj.getClass().getName());
}
}
private Object getSource(EvaluationContext ctx) {
Expression expression = ctx.getExpression();
if (expression instanceof ValueExpression) {
return ((ValueExpression)expression).getSource();
}
return null;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy