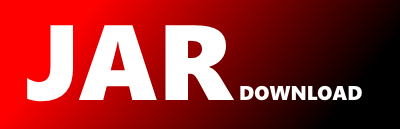
org.jdesktop.swingbinding.SwingBindings Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of swixml Show documentation
Show all versions of swixml Show documentation
GUI generating engine for Java applications
The newest version!
/*
* Copyright (C) 2007 Sun Microsystems, Inc. All rights reserved. Use is
* subject to license terms.
*/
package org.jdesktop.swingbinding;
import java.util.*;
import javax.swing.*;
import org.jdesktop.beansbinding.AutoBinding;
import org.jdesktop.beansbinding.ObjectProperty;
import org.jdesktop.beansbinding.Property;
/**
* A factory class for creating instances of the custom Swing {@code Binding}
* implementations provided by this package. See the
* package summary for full details on
* binding to Swing components.
*
* @author Shannon Hickey
*/
public class SwingBindings {
private SwingBindings() {}
/**
* Creates a {@code JListBinding} from direct references to a {@code List} and {@code JList}.
*
* @param strategy the update strategy
* @param sourceList the source {@code List}
* @param targetJList the target {@code JList}
* @return the {@code JTableBinding}
*/
public static JListBinding, JList> createJListBinding(AutoBinding.UpdateStrategy strategy, List sourceList, JList targetJList) {
return new JListBinding, JList>(strategy, sourceList, ObjectProperty.>create(), targetJList, ObjectProperty.create(), null);
}
/**
* Creates a named {@code JListBinding} from direct references to a {@code List} and {@code JList}.
*
* @param strategy the update strategy
* @param sourceList the source {@code List}
* @param targetJList the target {@code JList}
* @return the {@code JListBinding}
*/
public static JListBinding, JList> createJListBinding(AutoBinding.UpdateStrategy strategy, List sourceList, JList targetJList, String name) {
return new JListBinding, JList>(strategy, sourceList, ObjectProperty.>create(), targetJList, ObjectProperty.create(), name);
}
/**
* Creates a {@code JListBinding} from an object and property that resolves to a {@code List} and a direct reference to a {@code JList}.
*
* @param strategy the update strategy
* @param sourceObject the source object
* @param sourceListProperty a property on the source object that resolves to a {@code List}
* @param targetJList the target {@code JList}
* @return the {@code JListBinding}
* @throws IllegalArgumentException if {@code sourceListProperty} is {@code null}
*/
public static JListBinding createJListBinding(AutoBinding.UpdateStrategy strategy, SS sourceObject, Property> sourceListProperty, JList targetJList) {
return new JListBinding(strategy, sourceObject, sourceListProperty, targetJList, ObjectProperty.create(), null);
}
/**
* Creates a named {@code JListBinding} from an object and property that resolves to a {@code List} and a direct reference to a {@code JList}.
*
* @param strategy the update strategy
* @param sourceObject the source object
* @param sourceListProperty a property on the source object that resolves to a {@code List}
* @param targetJList the target {@code JList}
* @return the {@code JListBinding}
* @throws IllegalArgumentException if {@code sourceListProperty} is {@code null}
*/
public static JListBinding createJListBinding(AutoBinding.UpdateStrategy strategy, SS sourceObject, Property> sourceListProperty, JList targetJList, String name) {
return new JListBinding(strategy, sourceObject, sourceListProperty, targetJList, ObjectProperty.create(), name);
}
/**
* Creates a {@code JListBinding} from a direct reference to a {@code List} and an object and property that resolves to a {@code JList}.
*
* @param strategy the update strategy
* @param sourceList the source {@code List}
* @param targetObject the target object
* @param targetJListProperty a property on the target object that resolves to a {@code JList}
* @return the {@code JListBinding}
* @throws IllegalArgumentException if {@code targetJListProperty} is {@code null}
*/
public static JListBinding, TS> createJListBinding(AutoBinding.UpdateStrategy strategy, List sourceList, TS targetObject, Property targetJListProperty) {
return new JListBinding, TS>(strategy, sourceList, ObjectProperty.>create(), targetObject, targetJListProperty, null);
}
/**
* Creates a named {@code JListBinding} from a direct reference to a {@code List} and an object and property that resolves to a {@code JList}.
*
* @param strategy the update strategy
* @param sourceList the source {@code List}
* @param targetObject the target object
* @param targetJListProperty a property on the target object that resolves to a {@code JList}
* @return the {@code JListBinding}
* @throws IllegalArgumentException if {@code targetJListProperty} is {@code null}
*/
public static JListBinding, TS> createJListBinding(AutoBinding.UpdateStrategy strategy, List sourceList, TS targetObject, Property targetJListProperty, String name) {
return new JListBinding, TS>(strategy, sourceList, ObjectProperty.>create(), targetObject, targetJListProperty, name);
}
/**
* Creates a {@code JListBinding} from an object and property that resolves to a {@code List} and an object and property that resolves to a {@code JList}.
*
* @param strategy the update strategy
* @param sourceObject the source object
* @param sourceListProperty a property on the source object that resolves to a {@code List}
* @param targetObject the target object
* @param targetJListProperty a property on the target object that resolves to a {@code JList}
* @return the {@code JListBinding}
* @throws IllegalArgumentException if {@code sourceListProperty} or {@code targetJListProperty} is {@code null}
*/
public static JListBinding createJListBinding(AutoBinding.UpdateStrategy strategy, SS sourceObject, Property> sourceListProperty, TS targetObject, Property targetJListProperty) {
return new JListBinding(strategy, sourceObject, sourceListProperty, targetObject, targetJListProperty, null);
}
/**
* Creates a named {@code JListBinding} from an object and property that resolves to a {@code List} and an object and property that resolves to a {@code JList}.
*
* @param strategy the update strategy
* @param sourceObject the source object
* @param sourceListProperty a property on the source object that resolves to a {@code List}
* @param targetObject the target object
* @param targetJListProperty a property on the target object that resolves to a {@code JList}
* @return the {@code JListBinding}
* @throws IllegalArgumentException if {@code sourceListProperty} or {@code targetJListProperty} is {@code null}
*/
public static JListBinding createJListBinding(AutoBinding.UpdateStrategy strategy, SS sourceObject, Property> sourceListProperty, TS targetObject, Property targetJListProperty, String name) {
return new JListBinding(strategy, sourceObject, sourceListProperty, targetObject, targetJListProperty, name);
}
/**
* Creates a {@code JTableBinding} from direct references to a {@code List} and {@code JTable}.
*
* @param strategy the update strategy
* @param sourceList the source {@code List}
* @param targetJTable the target {@code JTable}
* @return the {@code JTableBinding}
*/
public static JTableBinding, JTable> createJTableBinding(AutoBinding.UpdateStrategy strategy, List sourceList, JTable targetJTable) {
return new JTableBinding, JTable>(strategy, sourceList, ObjectProperty.>create(), targetJTable, ObjectProperty.create(), null);
}
/**
* Creates a named {@code JTableBinding} from direct references to a {@code List} and {@code JTable}.
*
* @param strategy the update strategy
* @param sourceList the source {@code List}
* @param targetJTable the target {@code JTable}
* @return the {@code JTableBinding}
*/
public static JTableBinding, JTable> createJTableBinding(AutoBinding.UpdateStrategy strategy, List sourceList, JTable targetJTable, String name) {
return new JTableBinding, JTable>(strategy, sourceList, ObjectProperty.>create(), targetJTable, ObjectProperty.create(), name);
}
/**
* Creates a {@code JTableBinding} from an object and property that resolves to a {@code List} and a direct reference to a {@code JTable}.
*
* @param strategy the update strategy
* @param sourceObject the source object
* @param sourceListProperty a property on the source object that resolves to a {@code List}
* @param targetJTable the target {@code JTable}
* @return the {@code JTableBinding}
* @throws IllegalArgumentException if {@code sourceListProperty} is {@code null}
*/
public static JTableBinding createJTableBinding(AutoBinding.UpdateStrategy strategy, SS sourceObject, Property> sourceListProperty, JTable targetJTable) {
return new JTableBinding(strategy, sourceObject, sourceListProperty, targetJTable, ObjectProperty.create(), null);
}
/**
* Creates a named {@code JTableBinding} from an object and property that resolves to a {@code List} and a direct reference to a {@code JTable}.
*
* @param strategy the update strategy
* @param sourceObject the source object
* @param sourceListProperty a property on the source object that resolves to a {@code List}
* @param targetJTable the target {@code JTable}
* @return the {@code JTableBinding}
* @throws IllegalArgumentException if {@code sourceListProperty} is {@code null}
*/
public static JTableBinding createJTableBinding(AutoBinding.UpdateStrategy strategy, SS sourceObject, Property> sourceListProperty, JTable targetJTable, String name) {
return new JTableBinding(strategy, sourceObject, sourceListProperty, targetJTable, ObjectProperty.create(), name);
}
/**
* Creates a {@code JTableBinding} from a direct reference to a {@code List} and an object and property that resolves to a {@code JTable}.
*
* @param strategy the update strategy
* @param sourceList the source {@code List}
* @param targetObject the target object
* @param targetJTableProperty a property on the target object that resolves to a {@code JTable}
* @return the {@code JTableBinding}
* @throws IllegalArgumentException if {@code targetJTableProperty} is {@code null}
*/
public static JTableBinding, TS> createJTableBinding(AutoBinding.UpdateStrategy strategy, List sourceList, TS targetObject, Property targetJTableProperty) {
return new JTableBinding, TS>(strategy, sourceList, ObjectProperty.>create(), targetObject, targetJTableProperty, null);
}
/**
* Creates a named {@code JTableBinding} from a direct reference to a {@code List} and an object and property that resolves to a {@code JTable}.
*
* @param strategy the update strategy
* @param sourceList the source {@code List}
* @param targetObject the target object
* @param targetJTableProperty a property on the target object that resolves to a {@code JTable}
* @return the {@code JTableBinding}
* @throws IllegalArgumentException if {@code targetJTableProperty} is {@code null}
*/
public static JTableBinding, TS> createJTableBinding(AutoBinding.UpdateStrategy strategy, List sourceList, TS targetObject, Property targetJTableProperty, String name) {
return new JTableBinding, TS>(strategy, sourceList, ObjectProperty.>create(), targetObject, targetJTableProperty, name);
}
/**
* Creates a {@code JTableBinding} from an object and property that resolves to a {@code List} and an object and property that resolves to a {@code JTable}.
*
* @param strategy the update strategy
* @param sourceObject the source object
* @param sourceListProperty a property on the source object that resolves to a {@code List}
* @param targetObject the target object
* @param targetJTableProperty a property on the target object that resolves to a {@code JTable}
* @return the {@code JTableBinding}
* @throws IllegalArgumentException if {@code sourceListProperty} or {@code targetJTableProperty} is {@code null}
*/
public static JTableBinding createJTableBinding(AutoBinding.UpdateStrategy strategy, SS sourceObject, Property> sourceListProperty, TS targetObject, Property targetJTableProperty) {
return new JTableBinding(strategy, sourceObject, sourceListProperty, targetObject, targetJTableProperty, null);
}
/**
* Creates a named {@code JTableBinding} from an object and property that resolves to a {@code List} and an object and property that resolves to a {@code JTable}.
*
* @param strategy the update strategy
* @param sourceObject the source object
* @param sourceListProperty a property on the source object that resolves to a {@code List}
* @param targetObject the target object
* @param targetJTableProperty a property on the target object that resolves to a {@code JTable}
* @return the {@code JTableBinding}
* @throws IllegalArgumentException if {@code sourceListProperty} or {@code targetJTableProperty} is {@code null}
*/
public static JTableBinding createJTableBinding(AutoBinding.UpdateStrategy strategy, SS sourceObject, Property> sourceListProperty, TS targetObject, Property targetJTableProperty, String name) {
return new JTableBinding(strategy, sourceObject, sourceListProperty, targetObject, targetJTableProperty, name);
}
/**
* Creates a {@code JComboBoxBinding} from direct references to a {@code List} and {@code JComboBox}.
*
* @param strategy the update strategy
* @param sourceList the source {@code List}
* @param targetJComboBox the target {@code JComboBox}
* @return the {@code JComboBoxBinding}
*/
public static JComboBoxBinding, JComboBox> createJComboBoxBinding(AutoBinding.UpdateStrategy strategy, List sourceList, JComboBox targetJComboBox) {
return new JComboBoxBinding, JComboBox>(strategy, sourceList, ObjectProperty.>create(), targetJComboBox, ObjectProperty.create(), null);
}
/**
* Creates a named {@code JComboBoxBinding} from direct references to a {@code List} and {@code JComboBox}.
*
* @param strategy the update strategy
* @param sourceList the source {@code List}
* @param targetJComboBox the target {@code JComboBox}
* @return the {@code JComboBoxBinding}
*/
public static JComboBoxBinding, JComboBox> createJComboBoxBinding(AutoBinding.UpdateStrategy strategy, List sourceList, JComboBox targetJComboBox, String name) {
return new JComboBoxBinding, JComboBox>(strategy, sourceList, ObjectProperty.>create(), targetJComboBox, ObjectProperty.create(), name);
}
/**
* Creates a {@code JComboBoxBinding} from an object and property that resolves to a {@code List} and a direct reference to a {@code JComboBox}.
*
* @param strategy the update strategy
* @param sourceObject the source object
* @param sourceListProperty a property on the source object that resolves to a {@code List}
* @param targetJComboBox the target {@code JComboBox}
* @return the {@code JComboBoxBinding}
* @throws IllegalArgumentException if {@code sourceListProperty} is {@code null}
*/
public static JComboBoxBinding createJComboBoxBinding(AutoBinding.UpdateStrategy strategy, SS sourceObject, Property> sourceListProperty, JComboBox targetJComboBox) {
return new JComboBoxBinding(strategy, sourceObject, sourceListProperty, targetJComboBox, ObjectProperty.create(), null);
}
/**
* Creates a named {@code JComboBoxBinding} from an object and property that resolves to a {@code List} and a direct reference to a {@code JComboBox}.
*
* @param strategy the update strategy
* @param sourceObject the source object
* @param sourceListProperty a property on the source object that resolves to a {@code List}
* @param targetJComboBox the target {@code JComboBox}
* @return the {@code JComboBoxBinding}
* @throws IllegalArgumentException if {@code sourceListProperty} is {@code null}
*/
public static JComboBoxBinding createJComboBoxBinding(AutoBinding.UpdateStrategy strategy, SS sourceObject, Property> sourceListProperty, JComboBox targetJComboBox, String name) {
return new JComboBoxBinding(strategy, sourceObject, sourceListProperty, targetJComboBox, ObjectProperty.create(), name);
}
/**
* Creates a {@code JComboBoxBinding} from a direct reference to a {@code List} and an object and property that resolves to a {@code JComboBox}.
*
* @param strategy the update strategy
* @param sourceList the source {@code List}
* @param targetObject the target object
* @param targetJComboBoxProperty a property on the target object that resolves to a {@code JComboBox}
* @return the {@code JComboBoxBinding}
* @throws IllegalArgumentException if {@code targetJComboBoxProperty} is {@code null}
*/
public static JComboBoxBinding, TS> createJComboBoxBinding(AutoBinding.UpdateStrategy strategy, List sourceList, TS targetObject, Property targetJComboBoxProperty) {
return new JComboBoxBinding, TS>(strategy, sourceList, ObjectProperty.>create(), targetObject, targetJComboBoxProperty, null);
}
/**
* Creates a named {@code JComboBoxBinding} from a direct reference to a {@code List} and an object and property that resolves to a {@code JComboBox}.
*
* @param strategy the update strategy
* @param sourceList the source {@code List}
* @param targetObject the target object
* @param targetJComboBoxProperty a property on the target object that resolves to a {@code JComboBox}
* @return the {@code JComboBoxBinding}
* @throws IllegalArgumentException if {@code targetJComboBoxProperty} is {@code null}
*/
public static JComboBoxBinding, TS> createJComboBoxBinding(AutoBinding.UpdateStrategy strategy, List sourceList, TS targetObject, Property targetJComboBoxProperty, String name) {
return new JComboBoxBinding, TS>(strategy, sourceList, ObjectProperty.>create(), targetObject, targetJComboBoxProperty, name);
}
/**
* Creates a {@code JComboBoxBinding} from an object and property that resolves to a {@code List} and an object and property that resolves to a {@code JComboBox}.
*
* @param strategy the update strategy
* @param sourceObject the source object
* @param sourceListProperty a property on the source object that resolves to a {@code List}
* @param targetObject the target object
* @param targetJComboBoxProperty a property on the target object that resolves to a {@code JComboBox}
* @return the {@code JComboBoxBinding}
* @throws IllegalArgumentException if {@code sourceListProperty} or {@code targetJComboBoxProperty} is {@code null}
*/
public static JComboBoxBinding createJComboBoxBinding(AutoBinding.UpdateStrategy strategy, SS sourceObject, Property> sourceListProperty, TS targetObject, Property targetJComboBoxProperty) {
return new JComboBoxBinding(strategy, sourceObject, sourceListProperty, targetObject, targetJComboBoxProperty, null);
}
/**
* Creates a named {@code JComboBoxBinding} from an object and property that resolves to a {@code List} and an object and property that resolves to a {@code JComboBox}.
*
* @param strategy the update strategy
* @param sourceObject the source object
* @param sourceListProperty a property on the source object that resolves to a {@code List}
* @param targetObject the target object
* @param targetJComboBoxProperty a property on the target object that resolves to a {@code JComboBox}
* @return the {@code JComboBoxBinding}
* @throws IllegalArgumentException if {@code sourceListProperty} or {@code targetJComboBoxProperty} is {@code null}
*/
public static JComboBoxBinding createJComboBoxBinding(AutoBinding.UpdateStrategy strategy, SS sourceObject, Property> sourceListProperty, TS targetObject, Property targetJComboBoxProperty, String name) {
return new JComboBoxBinding(strategy, sourceObject, sourceListProperty, targetObject, targetJComboBoxProperty, name);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy