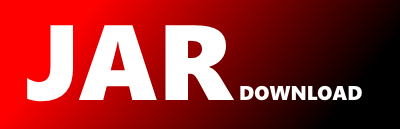
syncloud.google.docs.ChunkUploader Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of syncloud-google-docs Show documentation
Show all versions of syncloud-google-docs Show documentation
Google Docs Syncloud Starage Adapter
The newest version!
package syncloud.google.docs;
import syncloud.core.CoreException;
import syncloud.core.log.Logger;
import java.io.IOException;
import java.io.InputStream;
import java.util.Arrays;
public abstract class ChunkUploader {
private static Logger logger = Logger.getLogger(ChunkUploader.class);
private long size;
private TParameter parameter;
public ChunkUploader(long size, TParameter parameter) {
this.size = size;
this.parameter = parameter;
}
public TResult upload(InputStream is, int chunkSize) {
TResult result = null;
try {
byte[] chunk = new byte[chunkSize];
int bytesRed;
int currentChuck = 0;
logger.debug("will start reading bytes from input stream");
while ((bytesRed = is.read(chunk, 0, chunkSize)) != -1) {
int position = currentChuck * chunkSize;
byte[] bytes = chunk;
if (bytesRed != chunkSize)
bytes = Arrays.copyOfRange(chunk, 0, bytesRed);
logger.debug("processing bytes: " + bytes.length);
if ((position + bytesRed) < size) {
parameter = onChunk(bytes, position, bytesRed, parameter);
logger.debug("chunk uploaded");
} else {
result = onLastChunk(bytes, position, bytesRed, parameter);
logger.debug("last chunk uploaded");
}
currentChuck++;
}
logger.debug("no more bytes left in input stream");
} catch (IOException e) {
String message = "unable to read from input stream, " + e.getMessage();
logger.error(message);
throw new CoreException(message);
} finally {
try {
is.close();
} catch (IOException e) {
String message = "unable to close input stream";
logger.error(message);
throw new CoreException(message);
}
}
return result;
}
protected abstract TParameter onChunk(byte[] bytes, int position, int length, TParameter parameter);
protected abstract TResult onLastChunk(byte[] bytes, int position, int length, TParameter parameter);
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy