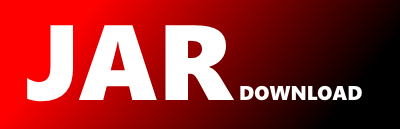
syncloud.google.docs.model.Entry Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of syncloud-google-docs Show documentation
Show all versions of syncloud-google-docs Show documentation
Google Docs Syncloud Starage Adapter
The newest version!
package syncloud.google.docs.model;
import com.google.api.client.util.Key;
import java.util.ArrayList;
import java.util.Date;
import java.util.List;
public class Entry {
private static final String KIND_LABEL_FOLDER = "folder";
@Key("@gd:etag")
public String etag;
@Key("category")
public List categories;
@Key("link")
public List links;
@Key("title")
public String title;
@Key
public String summary;
@Key
public Date updated;
@Key("docs:md5Checksum")
public String mdsChecksum;
@Key("gd:deleted")
public Boolean deleted;
@Key("docs:removed")
public Boolean removed;
@Key("gd:resourceId")
public String resourceId;
private Boolean isCollection;
@Key("gd:quotaBytesUsed")
private Long size;
@Key
private Content content;
public Entry() {
}
public Entry(String id) {
this.resourceId = id;
}
public long getSize() {
return size;
}
public Link getSelf() {
return Link.find(links, LinkRel.SELF);
}
public Link getResumable() {
return Link.find(links, LinkRel.RESUMABLE);
}
public List getLinks() {
return links;
}
public void setLinks(List links) {
this.links = links;
}
public Link getAlternate() {
return Link.find(links, LinkRel.SELF);
}
public String getResourceId() {
return resourceId;
}
public void setResourceId(String resourceId) {
this.resourceId = resourceId;
}
public Category getKind() {
return Category.find(categories, CategoryScheme.KIND);
}
public void setKind(Category category) {
if (categories == null)
categories = new ArrayList();
Category existingCategory = Category.find(categories, CategoryScheme.KIND);
if (existingCategory != null)
categories.remove(existingCategory);
categories.add(category);
}
public boolean isTrashed() {
Category category = Category.find(categories, CategoryScheme.LABELS, CategoryTerm.TRASHED);
return category != null;
}
public Boolean isDeleted() {
return deleted!=null && deleted;
}
public Boolean isRemoved() {
return removed!= null && removed;
}
public Link getParent() {
return Link.find(links, LinkRel.PARENT);
}
public boolean isCollection() {
if (isCollection != null)
return isCollection;
isCollection = getKind().label.equals(KIND_LABEL_FOLDER);
return isCollection;
}
public String getTitle() {
return title;
}
public void setTitle(String title) {
this.title = title;
}
public void setCollection(Boolean collection) {
isCollection = collection;
}
public Content getContent() {
return content;
}
public void setContent(Content content) {
this.content = content;
}
@Override
public String toString() {
return "Entry{" +
"resourceId='" + resourceId + '\'' +
", title='" + title + '\'' +
'}';
}
public Date getUpdated() {
return updated;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy