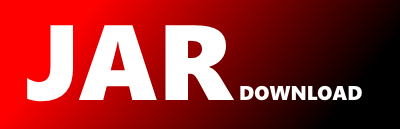
syncloud.storage.NodeKey Maven / Gradle / Ivy
The newest version!
package syncloud.storage;
public class NodeKey {
public static final String STORAGE_KEY_CANNOT_BE_EMPTY = "storage key cannot be empty";
public static final String PATH_KEY_CANNOT_BE_EMPTY = "path key cannot be empty";
private StorageKey storageKey;
private PathKey pathKey;
public NodeKey(StorageKey storageKey, PathKey pathKey) {
if (storageKey == null)
throw new StorageException(STORAGE_KEY_CANNOT_BE_EMPTY);
if (pathKey == null)
throw new StorageException(PATH_KEY_CANNOT_BE_EMPTY);
this.storageKey = storageKey;
this.pathKey = pathKey;
}
public static NodeKey create(StorageKey storageKey, String path) {
return new NodeKey(storageKey, PathKey.create(path));
}
public static NodeKey create(StorageKey storageKey, PathKey pathKey) {
return new NodeKey(storageKey, pathKey);
}
public NodeKey child(String name) {
return create(storageKey, pathKey.child(name));
}
public StorageKey getStorageKey() {
return storageKey;
}
public PathKey getPathKey() {
return pathKey;
}
public String getNativePath() {
return pathKey.getNativePath();
}
@Override
public boolean equals(Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
NodeKey nodeKey = (NodeKey) o;
if (!pathKey.equals(nodeKey.pathKey)) return false;
if (!storageKey.equals(nodeKey.storageKey)) return false;
return true;
}
@Override
public int hashCode() {
int result = storageKey.hashCode();
result = 31 * result + pathKey.hashCode();
return result;
}
@Override
public String toString() {
return "NodeKey{" + "storageKey=" + storageKey + ", path='" + pathKey + '\'' + '}';
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy