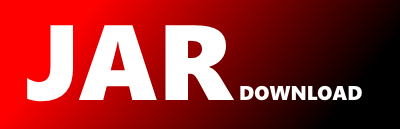
syncloud.storage.PathKey Maven / Gradle / Ivy
The newest version!
package syncloud.storage;
import org.apache.commons.io.FilenameUtils;
import org.apache.commons.lang.StringUtils;
import java.io.File;
import java.util.*;
public class PathKey {
private static String slash = "/";
private static String nativeSeparator = File.separator;
private List pathComponents = new ArrayList();
public PathKey(List pathComponents) {
this.pathComponents = pathComponents;
}
public PathKey(String... components) {
if (components != null)
this.pathComponents = Arrays.asList(components);
}
public static PathKey create(String path) throws StorageException {
String normilized = FilenameUtils.normalize(path);
normilized = FilenameUtils.separatorsToUnix(normilized);
ArrayList components = new ArrayList();
if (!normilized.isEmpty()) {
if (normilized.equals(slash)) {
components.add("");
} else {
String[] names = normilized.split(slash);
components.addAll(Arrays.asList(names));
}
}
return new PathKey(components);
}
public String getNativePath() {
return getPath(nativeSeparator);
}
public String getPath(String separator) {
String path = StringUtils.join(pathComponents, separator);
if (pathComponents.size() == 1) path += separator;
return path;
}
public PathKey child(String name) {
ArrayList components = new ArrayList(pathComponents);
components.add(name);
return new PathKey(components);
}
public List getPathComponents() {
return Collections.unmodifiableList(pathComponents);
}
@Override
public boolean equals(Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
PathKey pathKey = (PathKey) o;
if (pathComponents != null ? !pathComponents.equals(pathKey.pathComponents) : pathKey.pathComponents != null)
return false;
return true;
}
@Override
public int hashCode() {
int result = pathComponents != null ? pathComponents.hashCode() : 0;
return result;
}
@Override
public String toString() {
return "PathKey{" + "pathComponents=" + pathComponents + '}';
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy