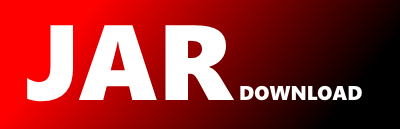
syncloud.storage.StorageUtils Maven / Gradle / Ivy
The newest version!
package syncloud.storage;
import java.util.ArrayList;
import java.util.Iterator;
import java.util.List;
public class StorageUtils {
private IStorage storage;
public StorageUtils(IStorage storage) {
this.storage = storage;
}
private List getChilds(IFolder folder) {
if (folder == null)
return this.storage.getRoots();
return folder.getFolders();
}
private IFolder createFolders(IFolder folder, List pathComponents) {
for (String missingComponent : pathComponents)
folder = folder.createFolder(missingComponent);
return folder;
}
public IFolder getOrCreateFolder(PathKey path) throws StorageException {
IFolder found = null;
List pathComponents = new ArrayList(path.getPathComponents());
Iterator iterator = pathComponents.iterator();
while(iterator.hasNext()) {
List folders = getChilds(found);
IFolder newFound = FolderUtils.find(folders, iterator.next());
if (newFound == null) break;
iterator.remove();
found = newFound;
}
return createFolders(found, pathComponents);
}
public IFolder getFolder(PathKey path) throws StorageException {
IFolder found = null;
for (String component : path.getPathComponents()) {
List folders = getChilds(found);
IFolder newFound = FolderUtils.find(folders, component);
if (newFound == null) return null;
found = newFound;
}
return found;
}
public List getFolders(PathKey path) throws StorageException {
if (path == null) return null;
IFolder folder = getFolder(path);
return getChilds(folder);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy