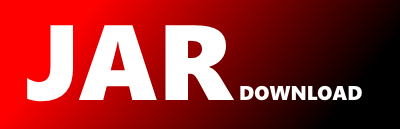
org.talend.sdk.component.runtime.manager.ComponentFamilyMeta Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of component-runtime-manager Show documentation
Show all versions of component-runtime-manager Show documentation
Core of the framework, the manager allows to access components and instantiate them.
/**
* Copyright (C) 2006-2024 Talend Inc. - www.talend.com
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.talend.sdk.component.runtime.manager;
import static java.util.Optional.empty;
import static java.util.Optional.ofNullable;
import java.lang.reflect.Method;
import java.util.Collection;
import java.util.HashMap;
import java.util.List;
import java.util.Locale;
import java.util.Map;
import java.util.MissingResourceException;
import java.util.Optional;
import java.util.ResourceBundle;
import java.util.concurrent.ConcurrentHashMap;
import java.util.concurrent.ConcurrentMap;
import java.util.function.Function;
import java.util.function.Supplier;
import java.util.stream.Stream;
import org.talend.sdk.component.api.component.MigrationHandler;
import org.talend.sdk.component.api.processor.ElementListener;
import org.talend.sdk.component.runtime.base.Lifecycle;
import org.talend.sdk.component.runtime.input.Mapper;
import org.talend.sdk.component.runtime.internationalization.ComponentBundle;
import org.talend.sdk.component.runtime.internationalization.FamilyBundle;
import org.talend.sdk.component.runtime.manager.util.IdGenerator;
import org.talend.sdk.component.runtime.output.Processor;
import org.talend.sdk.component.runtime.standalone.DriverRunner;
import lombok.AccessLevel;
import lombok.Data;
import lombok.EqualsAndHashCode;
import lombok.Getter;
import lombok.ToString;
import lombok.extern.slf4j.Slf4j;
@Data
@Slf4j
public class ComponentFamilyMeta {
private static final FamilyBundle NO_COMPONENT_BUNDLE = new FamilyBundle(null, null) {
@Override
public Optional actionDisplayName(final String type, final String name) {
return empty();
}
@Override
public Optional category(final String value) {
return empty();
}
@Override
public Optional configurationDisplayName(final String type, final String name) {
return empty();
}
@Override
public Optional displayName() {
return empty();
}
};
private final String id;
private final String plugin;
private final Collection categories;
private final String icon;
private final String name;
private final String packageName;
private final Map partitionMappers = new HashMap<>();
private final Map processors = new HashMap<>();
private final Map driverRunners = new HashMap<>();
private final ConcurrentMap bundles = new ConcurrentHashMap<>();
public ComponentFamilyMeta(final String plugin, final Collection categories, final String icon,
final String name, final String packageName) {
this.id = IdGenerator.get(plugin, name);
this.plugin = plugin;
this.categories = categories;
this.icon = icon;
this.name = name;
this.packageName = packageName;
}
public FamilyBundle findBundle(final ClassLoader loader, final Locale locale) {
return bundles.computeIfAbsent(locale, l -> {
try {
final ResourceBundle bundle = ResourceBundle
.getBundle((packageName.isEmpty() ? packageName : (packageName + '.')) + "Messages", locale,
loader);
return new FamilyBundle(bundle, name + '.');
} catch (final MissingResourceException mre) {
log
.warn("No bundle for " + packageName + " (" + name
+ "), means the display names will be the technical names");
log.debug(mre.getMessage(), mre);
return NO_COMPONENT_BUNDLE;
}
});
}
@Data
public static class BaseMeta {
private static final ComponentBundle NO_COMPONENT_BUNDLE = new ComponentBundle(null, null) {
@Override
public Optional displayName() {
return empty();
}
};
private final ComponentFamilyMeta parent;
private final String name;
private final String icon;
private final int version;
private final String packageName;
private final Supplier migrationHandler;
private final Supplier> parameterMetas;
private final ConcurrentMap bundles = new ConcurrentHashMap<>();
/**
* Stores data provided by extensions like ContainerListenerExtension
*/
@Getter(AccessLevel.NONE)
private final ConcurrentMap, Object> extensionsData = new ConcurrentHashMap<>();
private final String id;
private final Class> type;
private final Function
© 2015 - 2025 Weber Informatics LLC | Privacy Policy