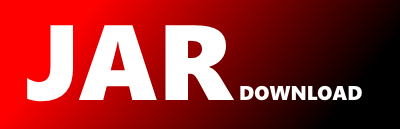
admin.astor.HostList Maven / Gradle / Ivy
//+======================================================================
// $Source: $
//
// Project: Tango
//
// Description: Basic Dialog Class to display info
//
// $Author: pascal_verdier $
//
// Copyright (C) : 2004,2005,2006,2007,2008,2009,2009
// European Synchrotron Radiation Facility
// BP 220, Grenoble 38043
// FRANCE
//
// This file is part of Tango.
//
// Tango is free software: you can redistribute it and/or modify
// it under the terms of the GNU General Public License as published by
// the Free Software Foundation, either version 3 of the License, or
// (at your option) any later version.
//
// Tango is distributed in the hope that it will be useful,
// but WITHOUT ANY WARRANTY; without even the implied warranty of
// MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
// GNU General Public License for more details.
//
// You should have received a copy of the GNU General Public License
// along with Tango. If not, see .
//
// $Revision: $
//
// $Log: $
//
//-======================================================================
package admin.astor;
import fr.esrf.Tango.DevFailed;
import fr.esrf.TangoApi.ApiUtil;
import fr.esrf.TangoApi.DeviceData;
import fr.esrf.tangoatk.widget.util.ATKGraphicsUtils;
import javax.swing.*;
import java.awt.*;
import java.awt.event.MouseAdapter;
import java.awt.event.MouseEvent;
import java.util.ArrayList;
import java.util.List;
/**
* JDialog Class to display a host list, to select one of them
* and to return the list of servers registered for this host.
*
* @author Pascal Verdier
*/
@SuppressWarnings("MagicConstant")
public class HostList extends JDialog {
private JDialog parent;
private TangoHost[] hosts;
private String selectedHostName;
private int retVal = JOptionPane.OK_OPTION;
//===============================================================
/**
* Creates new form HostList
*/
//===============================================================
public HostList(JDialog parent, String theHost) throws DevFailed {
super(parent, true);
this.parent = parent;
initComponents();
hosts = AstorUtil.getInstance().getTangoHostList();
List hostNames = new ArrayList<>();
int i=0;
for (TangoHost host : hosts) {
int start = host.name().lastIndexOf('/');
if (start>0)
hostNames.add(host.name().substring(start+1));
else
hostNames.add(host.name());
}
// Remove theHost
for (String hostName : hostNames) {
if (hostName.equals(theHost)) {
hostNames.remove(hostName);
break;
}
}
hostList.setListData(hostNames.toArray(new String[hostNames.size()]));
hostList.addMouseListener(new MouseAdapter() {
public void mouseClicked(MouseEvent e) {
listSelectionPerformed(e);
}
});
titleLabel.setText("Host List ");
int h = 20*hostNames.size();
if (h>600) h = 600;
jScrollPane1.setPreferredSize(new Dimension(200, h));
pack();
ATKGraphicsUtils.centerDialog(this);
}
//===============================================================
/** This method is called from within the constructor to
* initialize the form.
* WARNING: Do NOT modify this code. The content of this method is
* always regenerated by the Form Editor.
*/
//===============================================================
// //GEN-BEGIN:initComponents
private void initComponents() {
javax.swing.JPanel topPanel = new javax.swing.JPanel();
titleLabel = new javax.swing.JLabel();
javax.swing.JPanel bottomPanel = new javax.swing.JPanel();
javax.swing.JButton okBtn = new javax.swing.JButton();
javax.swing.JButton cancelBtn = new javax.swing.JButton();
jScrollPane1 = new javax.swing.JScrollPane();
hostList = new javax.swing.JList<>();
addWindowListener(new java.awt.event.WindowAdapter() {
public void windowClosing(java.awt.event.WindowEvent evt) {
closeDialog(evt);
}
});
titleLabel.setFont(new java.awt.Font("Dialog", 1, 18)); // NOI18N
titleLabel.setText("Hosts in control system");
topPanel.add(titleLabel);
getContentPane().add(topPanel, java.awt.BorderLayout.NORTH);
okBtn.setText("OK");
okBtn.addActionListener(new java.awt.event.ActionListener() {
public void actionPerformed(java.awt.event.ActionEvent evt) {
okBtnActionPerformed(evt);
}
});
bottomPanel.add(okBtn);
cancelBtn.setText("Cancel");
cancelBtn.addActionListener(new java.awt.event.ActionListener() {
public void actionPerformed(java.awt.event.ActionEvent evt) {
cancelBtnActionPerformed(evt);
}
});
bottomPanel.add(cancelBtn);
getContentPane().add(bottomPanel, java.awt.BorderLayout.SOUTH);
jScrollPane1.setViewportView(hostList);
getContentPane().add(jScrollPane1, java.awt.BorderLayout.CENTER);
pack();
}// //GEN-END:initComponents
//===============================================================
//===============================================================
@SuppressWarnings("unused")
private TangoHost getHost(String name) {
String deviceName = AstorUtil.getStarterDeviceHeader()+name.toLowerCase();
for (TangoHost host : hosts)
if (host.name().toLowerCase().equals(deviceName))
return host;
return null;
}
//===============================================================
//===============================================================
public List getServerList() throws DevFailed {
DeviceData argIn = new DeviceData();
argIn.insert(selectedHostName);
//cout << "DbGetHostServerList for " << hostnames[i] << endl;
DeviceData argOut = ApiUtil.get_db_obj().command_inout("DbGetHostServerList", argIn);
String[] serverList = argOut.extractStringArray();
List list = new ArrayList<>();
for (String server : serverList) {
if (!server.startsWith("Starter/"))
list.add(server);
}
return list;
}
//===============================================================
//===============================================================
public String getSelectedHostName() {
return selectedHostName;
}
//===============================================================
//===============================================================
private void listSelectionPerformed(MouseEvent evt) {
System.out.println("In listSelectionPerformed() " + evt.getClickCount());
// Check if double click
if (evt.getClickCount() == 2) {
selectedHostName = hostList.getSelectedValue();
retVal = JOptionPane.OK_OPTION;
doClose();
}
}
//===============================================================
//===============================================================
@SuppressWarnings("UnusedParameters")
private void okBtnActionPerformed(java.awt.event.ActionEvent evt) {//GEN-FIRST:event_okBtnActionPerformed
selectedHostName = hostList.getSelectedValue();
retVal = JOptionPane.OK_OPTION;
doClose();
}//GEN-LAST:event_okBtnActionPerformed
//===============================================================
//===============================================================
@SuppressWarnings("UnusedParameters")
private void cancelBtnActionPerformed(java.awt.event.ActionEvent evt) {//GEN-FIRST:event_cancelBtnActionPerformed
retVal = JOptionPane.CANCEL_OPTION;
doClose();
}//GEN-LAST:event_cancelBtnActionPerformed
//===============================================================
//===============================================================
@SuppressWarnings("UnusedParameters")
private void closeDialog(java.awt.event.WindowEvent evt) {//GEN-FIRST:event_closeDialog
retVal = JOptionPane.CANCEL_OPTION;
doClose();
}//GEN-LAST:event_closeDialog
//===============================================================
/**
* Closes the dialog
*/
//===============================================================
private void doClose() {
if (parent==null)
System.exit(0);
else {
setVisible(false);
dispose();
}
}
//===============================================================
//===============================================================
public int showDialog() {
setVisible(true);
return retVal;
}
//===============================================================
// Variables declaration - do not modify//GEN-BEGIN:variables
private javax.swing.JList hostList;
private javax.swing.JScrollPane jScrollPane1;
private javax.swing.JLabel titleLabel;
// End of variables declaration//GEN-END:variables
//===============================================================
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy