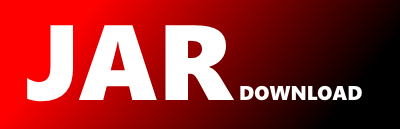
admin.astor.MultiServerCommand Maven / Gradle / Ivy
//+======================================================================
// $Source: $
//
// Project: Tango
//
// Description: java source code for Tango manager tool..
//
// $Author$
//
// Copyright (C) : 2004,2005,2006,2007,2008,2009,2010,2011,2012,2013,2014,2015,
// European Synchrotron Radiation Facility
// BP 220, Grenoble 38043
// FRANCE
//
// This file is part of Tango.
//
// Tango is free software: you can redistribute it and/or modify
// it under the terms of the GNU General Public License as published by
// the Free Software Foundation, either version 3 of the License, or
// (at your option) any later version.
//
// Tango is distributed in the hope that it will be useful,
// but WITHOUT ANY WARRANTY; without even the implied warranty of
// MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
// GNU General Public License for more details.
//
// You should have received a copy of the GNU General Public License
// along with Tango. If not, see .
//
// $Revision$
//
//-======================================================================
package admin.astor;
import admin.astor.tools.PopupTable;
import fr.esrf.Tango.DevFailed;
import fr.esrf.TangoApi.*;
import fr.esrf.TangoDs.Except;
import fr.esrf.tangoatk.widget.util.ATKGraphicsUtils;
import fr.esrf.tangoatk.widget.util.ErrorPane;
import javax.swing.*;
import java.awt.*;
import java.awt.event.MouseAdapter;
import java.awt.event.MouseEvent;
import java.awt.event.MouseListener;
import java.util.StringTokenizer;
import java.util.ArrayList;
import java.util.List;
/**
*
* @author verdier
*/
public class MultiServerCommand extends JDialog {
private JFrame parent;
private static String strFilter = "*";
private static String[] commands = {
"",
"Start",
"Stop",
"Status",
"Uptime",
};
//======================================================
/**
* Creates new form MultiServerCommand
*
* @param parent the parent instance
* @throws DevFailed in case of database connection failed
*/
//======================================================
public MultiServerCommand(JFrame parent) throws DevFailed {
super(parent, false);
this.parent = parent;
initComponents();
setTitle("Multi Servers Start/Stop");
// fix str filter and add a mouse listener on list
filterTxt.setText(strFilter);
MouseListener mouseListener = new MouseAdapter() {
public void mouseClicked(MouseEvent e) {
listSelectionPerformed(e);
}
};
jList.addMouseListener(mouseListener);
for (String cmd : commands)
comboBox.addItem(cmd);
setList();
pack();
ATKGraphicsUtils.centerDialog(this);
}
//======================================================
//======================================================
private void setList() throws DevFailed {
strFilter = filterTxt.getText();
String[] serverList = ApiUtil.get_db_obj().get_server_list(strFilter);
jList.setListData(serverList);
}
//======================================================
//======================================================
//======================================================
/**
* This method is called from within the constructor to
* initialize the form.
* WARNING: Do NOT modify this code. The content of this method is
* always regenerated by the FormEditor.
*/
//======================================================
// //GEN-BEGIN:initComponents
private void initComponents() {
java.awt.GridBagConstraints gridBagConstraints;
javax.swing.JPanel topPanel = new javax.swing.JPanel();
javax.swing.JLabel filterLabel = new javax.swing.JLabel();
filterTxt = new javax.swing.JTextField();
javax.swing.JScrollPane scrollPane = new javax.swing.JScrollPane();
jList = new javax.swing.JList<>();
javax.swing.JPanel bottomPanel = new javax.swing.JPanel();
javax.swing.JPanel jPanel1 = new javax.swing.JPanel();
javax.swing.JLabel jLabel1 = new javax.swing.JLabel();
comboBox = new javax.swing.JComboBox<>();
javax.swing.JButton sendCmdBtn = new javax.swing.JButton();
javax.swing.JButton cancelBtn = new javax.swing.JButton();
addWindowListener(new java.awt.event.WindowAdapter() {
public void windowClosing(java.awt.event.WindowEvent evt) {
closeDialog(evt);
}
});
filterLabel.setText("Filter : ");
topPanel.add(filterLabel);
filterTxt.setColumns(20);
filterTxt.addActionListener(new java.awt.event.ActionListener() {
public void actionPerformed(java.awt.event.ActionEvent evt) {
filterTxtActionPerformed(evt);
}
});
topPanel.add(filterTxt);
getContentPane().add(topPanel, java.awt.BorderLayout.NORTH);
scrollPane.setPreferredSize(new java.awt.Dimension(200, 300));
scrollPane.setViewportView(jList);
getContentPane().add(scrollPane, java.awt.BorderLayout.CENTER);
bottomPanel.setLayout(new java.awt.GridBagLayout());
jLabel1.setText("Command:");
jPanel1.add(jLabel1);
jPanel1.add(comboBox);
sendCmdBtn.setText("Send");
sendCmdBtn.addActionListener(new java.awt.event.ActionListener() {
public void actionPerformed(java.awt.event.ActionEvent evt) {
sendCmdBtnActionPerformed(evt);
}
});
jPanel1.add(sendCmdBtn);
gridBagConstraints = new java.awt.GridBagConstraints();
gridBagConstraints.gridx = 0;
gridBagConstraints.gridy = 0;
gridBagConstraints.insets = new java.awt.Insets(0, 0, 0, 50);
bottomPanel.add(jPanel1, gridBagConstraints);
cancelBtn.setText("Dismiss");
cancelBtn.addActionListener(new java.awt.event.ActionListener() {
public void actionPerformed(java.awt.event.ActionEvent evt) {
cancelBtnActionPerformed(evt);
}
});
gridBagConstraints = new java.awt.GridBagConstraints();
gridBagConstraints.gridx = 1;
gridBagConstraints.gridy = 0;
gridBagConstraints.insets = new java.awt.Insets(0, 50, 0, 0);
bottomPanel.add(cancelBtn, gridBagConstraints);
getContentPane().add(bottomPanel, java.awt.BorderLayout.SOUTH);
}// //GEN-END:initComponents
//======================================================
//======================================================
@SuppressWarnings({"UnusedDeclaration"})
private void listSelectionPerformed(MouseEvent evt) {
}
//======================================================
//======================================================
@SuppressWarnings({"UnusedDeclaration"})
private void filterTxtActionPerformed(java.awt.event.ActionEvent evt) {//GEN-FIRST:event_filterTxtActionPerformed
try {
setList();
} catch (DevFailed e) {
ErrorPane.showErrorMessage(this, "", e);
}
}//GEN-LAST:event_filterTxtActionPerformed
//======================================================
//======================================================
@SuppressWarnings({"UnusedDeclaration"})
private void cancelBtnActionPerformed(java.awt.event.ActionEvent evt) {//GEN-FIRST:event_cancelBtnActionPerformed
if (parent==null)
System.exit(0);
setVisible(false);
dispose();
}//GEN-LAST:event_cancelBtnActionPerformed
//======================================================
//======================================================
@SuppressWarnings({"UnusedDeclaration"})
private void closeDialog(java.awt.event.WindowEvent evt) {//GEN-FIRST:event_closeDialog
if (parent==null)
System.exit(0);
setVisible(false);
dispose();
}//GEN-LAST:event_closeDialog
//======================================================
//======================================================
@SuppressWarnings({"UnusedDeclaration"})
private void sendCmdBtnActionPerformed(java.awt.event.ActionEvent evt) {//GEN-FIRST:event_sendCmdBtnActionPerformed
// Get and check selection
List selections = jList.getSelectedValuesList();
if (selections.size() == 0) {
displayError(this, "No server selected !",
"MultiServerCommand.sendCmdBtnActionPerformed()");
return;
}
// Get command to be executed
String command = (String) comboBox.getSelectedItem();
if (command==null)
return;
if (command.length() == 0) {
displayError(this, "No command selected !",
"MultiServerCommand.sendCmdBtnActionPerformed()");
return;
}
// Dispatch for command
switch (command.toLowerCase()) {
case "uptime":
displayServerUpTimes(selections);
break;
case "status":
displayServerStatus(selections);
break;
default:
starterCommandForServers(command, selections);
break;
}
}//GEN-LAST:event_sendCmdBtnActionPerformed
//======================================================
//======================================================
public static void displayError(Component component, String desc, String orig) {
try {
Except.throw_exception("ERROR", desc, orig);
} catch (DevFailed e) {
ErrorPane.showErrorMessage(component, "", e);
}
}
//======================================================
//======================================================
//======================================================
/**
* @param args the command line arguments
*/
//======================================================
public static void main(String[] args) {
try {
MultiServerCommand msc = new MultiServerCommand(null);
msc.setVisible(true);
} catch (DevFailed e) {
ErrorPane.showErrorMessage(new JFrame(), "", e);
}
}
//======================================================
//======================================================
// Variables declaration - do not modify//GEN-BEGIN:variables
private javax.swing.JComboBox comboBox;
private javax.swing.JTextField filterTxt;
private javax.swing.JList jList;
// End of variables declaration//GEN-END:variables
//======================================================
//======================================================
//======================================================
//======================================================
private static String getHostnameWithoutFQDN(String hostname) {
int pos = hostname.indexOf('.');
if (pos < 0)
return hostname;
else
return hostname.substring(0, pos);
}
//======================================================
//======================================================
private DeviceProxy getStarterInstanceForServer(String serverName) throws DevFailed {
String devName = "dserver/" + serverName;
DeviceProxy dev = new DeviceProxy(devName);
DeviceInfo info = dev.get_info();
String hostname = getHostnameWithoutFQDN(info.hostname);
String starterDeviceName = AstorUtil.getStarterDeviceHeader() + hostname;
return new DeviceProxy(starterDeviceName);
}
//======================================================
//======================================================
private long getDelayBetweenServers(String message, long defaultValue) {
long t = -1;
while (t < 0) {
String strVal = (String) JOptionPane.showInputDialog(this,
message + "\nDelay between servers (ms) ?",
"Input Dialog",
JOptionPane.INFORMATION_MESSAGE,
null, null, defaultValue);
if (strVal == null)
return -1;
try {
t = Long.parseLong(strVal);
} catch (NumberFormatException e) {
ErrorPane.showErrorMessage(this, null, e);
}
}
if (t == 0)
t = 10; // ms
return t;
}
//======================================================
//======================================================
private void displayServerUpTimes(List serverNames) {
List lines = new ArrayList<>();
try {
for (String serverName : serverNames) {
String[] exportedStr = new TangoServer("dserver/" + serverName).getServerUptime();
lines.add(new String[]{
serverName, exportedStr[0], exportedStr[1]});
}
String[] columns = new String[]{"Server", "Last exported", "Last unexported"};
PopupTable ppt = new PopupTable(this, "",
columns, lines, new Dimension(650, 250));
ppt.setVisible(true);
} catch (DevFailed e) {
ErrorPane.showErrorMessage(this, null, e);
}
}
//======================================================
//======================================================
private static String convertStatus(String status) {
switch (status) {
case "ON":
return "Running";
case "FAULT":
return "Stopped";
case "MOVING":
return "Running but NOT responding";
default:
return status;
}
}
//======================================================
//======================================================
private void displayServerStatus(List serverNames) {
List statusList = new ArrayList<>();
// For each starter, get server status
for (String serverName : serverNames) {
try {
DeviceProxy starter = getStarterInstanceForServer(serverName);
DeviceAttribute argOut = starter.read_attribute("Servers");
String[] serverStates = argOut.extractStringArray();
for (String line : serverStates) {
StringTokenizer stk = new StringTokenizer(line);
String server = stk.nextToken();
String status = convertStatus(stk.nextToken());
if (server.toLowerCase().equals(serverName.toLowerCase()))
statusList.add(new String[]{server, status});
}
} catch (DevFailed e) {
if (e.errors[0].desc.contains("/nada not defined in the database"))
statusList.add(new String[]{serverName, "The server has never been running"});
else
statusList.add(new String[]{serverName, e.errors[0].desc});
}
}
if (statusList.size() == 0) {
System.err.println("No data found !");
return;
}
String[] columns = new String[]{"Servers", "Status"};
try {
int height = 30 + statusList.size()*16;
if (height>800) height = 800;
PopupTable ppt = new PopupTable(this, "",
columns, statusList, new Dimension(700, height));
ppt.setVisible(true);
} catch (DevFailed e) {
ErrorPane.showErrorMessage(this, null, e);
}
}
//======================================================
//======================================================
private long delayBetweenServers = 1000;
private void starterCommandForServers(String command, List servNames) {
// Check for command.
if (command.equals("Start"))
command = "DevStart";
else if (command.equals("Stop"))
command = "DevStop";
// Ask to confirm
long tmpDelay = getDelayBetweenServers(
"Send " + command + " on all selected servers:",
delayBetweenServers);
if (tmpDelay > 0) {
delayBetweenServers = tmpDelay;
new CommandThread(this, command, servNames, delayBetweenServers).start();
}
}
//======================================================
//======================================================
//======================================================
//======================================================
private class CommandThread extends Thread {
private Component component;
private String command;
private List servNames;
private long delay;
//======================================================
private CommandThread(Component component,
String command,
List servNames,
long delay) {
this.component = component;
this.command = command;
this.servNames = servNames;
this.delay = delay;
}
//======================================================
public void run() {
component.setCursor(new Cursor(Cursor.WAIT_CURSOR));
StringBuilder sb = new StringBuilder();
for (String servName : servNames) {
try {
DeviceProxy starter = getStarterInstanceForServer(servName);
DeviceData argin = new DeviceData();
argin.insert(servName);
starter.command_inout(command, argin);
Thread.sleep(delay);
} catch (DevFailed e) {
sb.append(e.errors[0].desc).append(" for ").append(servName).append('\n');
} catch (InterruptedException e) {
sb.append(e).append(" for ").append(servName).append('\n');
}
}
component.setCursor(new Cursor(Cursor.DEFAULT_CURSOR));
if (sb.length() > 0)
displayError(component, sb.toString(),
"MultiServerCommand.starterCommandForServers()");
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy