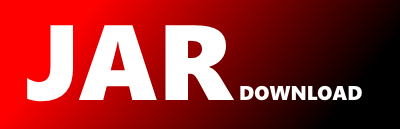
admin.astor.ServerCmdThread Maven / Gradle / Ivy
//+======================================================================
// $Source: $
//
// Project: Tango
//
// Description: java source code for Tango manager tool..
//
// $Author$
//
// Copyright (C) : 2004,2005,2006,2007,2008,2009,2010,2011,2012,2013,2014,2015,
// European Synchrotron Radiation Facility
// BP 220, Grenoble 38043
// FRANCE
//
// This file is part of Tango.
//
// Tango is free software: you can redistribute it and/or modify
// it under the terms of the GNU General Public License as published by
// the Free Software Foundation, either version 3 of the License, or
// (at your option) any later version.
//
// Tango is distributed in the hope that it will be useful,
// but WITHOUT ANY WARRANTY; without even the implied warranty of
// MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
// GNU General Public License for more details.
//
// You should have received a copy of the GNU General Public License
// along with Tango. If not, see .
//
// $Revision$
//
//-======================================================================
package admin.astor;
import admin.astor.tools.Utils;
import fr.esrf.Tango.DevFailed;
import fr.esrf.tangoatk.widget.util.ATKGraphicsUtils;
import javax.swing.*;
import java.awt.*;
import java.util.List;
/**
* This class is a thread to send command to all servers.
*
* @author verdier
*/
public class ServerCmdThread extends Thread implements AstorDefs {
private Window parent;
private TangoHost[] hosts;
private int cmd;
private Monitor monitor;
private boolean[] levelUsed;
private short nbStartupLevels;
private String monitor_title;
private boolean forAll = true;
private boolean fromList;
private List levels = null;
private TangoServer[] servers = null;
private enum ServerCmdMode {MULTI_HOST,SERVERS_LIST,LEVELS_LIST_SINGLE_HOST}
private ServerCmdMode mode;
//=======================================================
/**
* Thread Constructor for many hosts.
*
* @param parent The application parent used as parent
* for ProgressMonitor.
* @param hosts The controlled hosts.
* @param cmd command to be executed on all hosts.
*/
//=======================================================
public ServerCmdThread(Window parent, TangoHost[] hosts, int cmd) {
this.mode = ServerCmdMode.MULTI_HOST;
this.parent = parent;
this.hosts = hosts;
this.cmd = cmd;
monitor_title = " on all controlled hosts ";
nbStartupLevels = AstorUtil.getStarterNbStartupLevels();
levelUsed = new boolean[nbStartupLevels];
for (int i = 0; i < nbStartupLevels; i++)
levelUsed[i] = true;
fromList = false;
}
//=======================================================
/**
* Thread Constructor for one host.
*
* @param parent The application parent used as parent
* for ProgressMonitor.
* @param host The controlled host.
* @param cmd command to be executed on all hosts.
* @param levels list of levels
*/
//=======================================================
public ServerCmdThread(Window parent, TangoHost host, int cmd, List levels) {
this(parent, host, cmd, levels, true);
}
//=======================================================
/**
* Thread Constructor for one host.
*
* @param parent The application parent used as parent
* for ProgressMonitor.
* @param host The controlled host.
* @param cmd command to be executed on all hosts.
* @param levels list of levels
* @param forAll preSelection for checkbox do it for all Level
*/
//=======================================================
public ServerCmdThread(Window parent, TangoHost host, int cmd, List levels, boolean forAll) {
this.mode = ServerCmdMode.LEVELS_LIST_SINGLE_HOST;
this.parent = parent;
this.hosts = new TangoHost[1];
this.hosts[0] = host;
this.cmd = cmd;
this.levels = levels;
this.forAll = forAll;
monitor_title = " on " + host + " ";
nbStartupLevels = AstorUtil.getStarterNbStartupLevels();
fromList = true;
}
//=======================================================
/**
* Thread Constructor for one host.
*
* @param parent The application parent used as parent
* for ProgressMonitor.
* @param host The controlled host.
* @param cmd command to be executed on all hosts.
* @param servers list of servers
*/
//=======================================================
public ServerCmdThread(Window parent, TangoHost host, int cmd, TangoServer[] servers) {
this.mode = ServerCmdMode.SERVERS_LIST;
this.parent = parent;
this.hosts = new TangoHost[1];
this.hosts[0] = host;
this.cmd = cmd;
this.servers = servers;
this.forAll = true;
monitor_title = " on " + host + " ";
nbStartupLevels = AstorUtil.getStarterNbStartupLevels();
fromList = true;
}
//=======================================================
/*
* Update the ProgressMonitor
*/
//=======================================================
private void updateProgressMonitor(int level, int hostIndex, double ratio) {
String message;
if (monitor == null) {
message = cmdStr[cmd] + monitor_title;
if (parent instanceof JDialog)
monitor = new Monitor((JDialog) parent, message, cmdStr[cmd]);
else if (parent instanceof JFrame)
monitor = new Monitor((JFrame) parent, message, cmdStr[cmd]);
monitor.setLocationRelativeTo(parent);
}
message = cmdStr[cmd] + "Servers on " +
hosts[hostIndex].getName() + " for level " + level;
//System.out.println(hostIndex + " -> " + ratio);
monitor.setProgressValue(ratio, message);
}
//=======================================================
/*
* Update the ProgressMonitor
*/
//=======================================================
private void updateProgressMonitor(String serverName, int hostIndex, double ratio) {
String message;
if (monitor == null) {
message = cmdStr[cmd] + monitor_title;
if (parent instanceof JDialog)
monitor = new Monitor((JDialog) parent, message, cmdStr[cmd]);
else if (parent instanceof JFrame)
monitor = new Monitor((JFrame) parent, message, cmdStr[cmd]);
}
message = cmdStr[cmd] + serverName + " on " + hosts[hostIndex].getName();
//System.out.println(hostIndex + " -> " + ratio);
monitor.setProgressValue(ratio, message);
}
//=======================================================
/**
* Execute the servers commands.
*/
//=======================================================
public void run() {
// Initialize from properties
AstorUtil.getStarterNbStartupLevels();
// Start progress monitor
updateProgressMonitor(0, 0, 0.05);
if(mode == ServerCmdMode.MULTI_HOST || mode == ServerCmdMode.LEVELS_LIST_SINGLE_HOST){
// Build the confirm dialog
StartStopDialog startStopDialog;
if (parent instanceof JDialog)
startStopDialog = new StartStopDialog((JDialog)parent, levels==null || levels.size()>1);
else
startStopDialog = new StartStopDialog((JFrame) parent, levels==null || levels.size()>1);
startStopDialog.setSelectedForAll(!forAll);
// For each startup level
// (Increase for start or decrease for stop)
if (fromList) {
for (int level : levels) {
if (startStopDialog.doItForAll()) {
executeCommand(hosts, level);
} else {
switch (startStopDialog.showDialog(cmdStr[cmd] + " for level " + level + " ? ")) {
case JOptionPane.CANCEL_OPTION:
monitor.setProgressValue(100.0);
return;
case JOptionPane.OK_OPTION:
executeCommand(hosts, level);
break;
case JOptionPane.NO_OPTION:
break;
}
}
}
}
else { // For all levels
switch (cmd) {
case StartAllServers:
for (int level=1 ; !monitor.isCanceled() && level<=nbStartupLevels; level++) {
if (levelUsed[level - 1]) {
if (startStopDialog.doItForAll()) {
executeCommand(hosts, level);
}
else {
switch (startStopDialog.showDialog(cmdStr[cmd] + " for level " + level + " ? ")) {
case JOptionPane.CANCEL_OPTION :
level = nbStartupLevels;
break;
case JOptionPane.OK_OPTION:
executeCommand(hosts, level);
break;
case JOptionPane.NO_OPTION:
break;
}
}
}
}
break;
case StopAllServers:
for (int level = nbStartupLevels; !monitor.isCanceled() && level>0 ; level--) {
if (levelUsed[level - 1]) {
if (startStopDialog.doItForAll()) {
executeCommand(hosts, level);
}
else {
switch (startStopDialog.showDialog(cmdStr[cmd] + " for level " + level)) {
case JOptionPane.CANCEL_OPTION:
level = 0;
break;
case JOptionPane.OK_OPTION:
executeCommand(hosts, level);
break;
case JOptionPane.NO_OPTION:
break;
}
}
}
}
break;
}
}
} else if (this.mode == ServerCmdMode.SERVERS_LIST){
// Build the confirm dialog
StartStopDialog startStopDialog;
if (parent instanceof JDialog)
startStopDialog = new StartStopDialog((JDialog)parent, levels==null || levels.size()>1);
else
startStopDialog = new StartStopDialog((JFrame) parent, levels==null || levels.size()>1);
//button No is not a option
startStopDialog.setNoOption(false);
startStopDialog.setSelectedForAll(false);
if (cmd == StartAllServers) {
executeCommand(hosts[0], servers);
} else {
String serversList = AstorUtil.buildServerList(servers);
startStopDialog.setVisibleForAll(false);
switch (startStopDialog.showDialog("" + cmdStr[cmd] + " for "+ servers.length +" Servers :
" + serversList + "
Are you sure ? ")) {
case JOptionPane.CANCEL_OPTION:
monitor.setProgressValue(100.0);
return;
case JOptionPane.OK_OPTION:
executeCommand(hosts[0], servers);
break;
case JOptionPane.NO_OPTION:
break;
}
}
}
monitor.setProgressValue(100.0);
}
//============================================================
//============================================================
@SuppressWarnings({"NestedTryStatement"})
private void executeCommand(TangoHost[] hosts, int level) {
// For each host
for (int i=0 ; !monitor.isCanceled() && i
© 2015 - 2025 Weber Informatics LLC | Privacy Policy