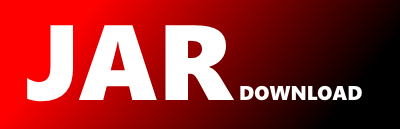
admin.astor.tools.DeviceHierarchyDialog Maven / Gradle / Ivy
//+======================================================================
// $Source: $
//
// Project: Tango
//
// Description: java source code for Tango manager tool..
//
// $Author$
//
// Copyright (C) : 2004,2005,2006,2007,2008,2009,2010,2011,2012,2013,2014,2015,
// European Synchrotron Radiation Facility
// BP 220, Grenoble 38043
// FRANCE
//
// This file is part of Tango.
//
// Tango is free software: you can redistribute it and/or modify
// it under the terms of the GNU General Public License as published by
// the Free Software Foundation, either version 3 of the License, or
// (at your option) any later version.
//
// Tango is distributed in the hope that it will be useful,
// but WITHOUT ANY WARRANTY; without even the implied warranty of
// MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
// GNU General Public License for more details.
//
// You should have received a copy of the GNU General Public License
// along with Tango. If not, see .
//
// $Revision$
//
//-======================================================================
package admin.astor.tools;
import admin.astor.Astor;
import admin.astor.AstorUtil;
import admin.astor.HostInfoDialog;
import fr.esrf.Tango.DevFailed;
import fr.esrf.tangoatk.widget.util.ErrorPane;
import javax.swing.*;
import java.awt.*;
//===============================================================
/**
* JDialog Class to display info
*
* @author Pascal Verdier
*/
//===============================================================
@SuppressWarnings("MagicConstant")
public class DeviceHierarchyDialog extends JDialog {
private Window parent;
private DeviceHierarchy tree = null;
private String name;
//===============================================================
/*
* Creates new form DeviceHierarchyDialog
*/
//===============================================================
public DeviceHierarchyDialog(Window parent, String name) throws DevFailed {
super();
this.parent = parent;
this.name = name;
init();
}
//===============================================================
//===============================================================
private void init() throws DevFailed {
try {
AstorUtil.startSplash("Device Hierarchy");
initComponents();
initOwnComponents();
setTitle("Device Hierarchy");
titleLabel.setText("Device hierarchy for " + name);
pack();
if (parent!=null) {
Point p = parent.getLocation();
p.translate(40, 30);
this.setLocation(p);
}
AstorUtil.stopSplash();
} catch (DevFailed e) {
AstorUtil.stopSplash();
throw e;
}
}
//===============================================================
//===============================================================
private void initOwnComponents() throws DevFailed {
// If it is an update, stop old tree's threads
if (tree != null)
tree.stopThread();
// Build users_tree to display info
Astor astor = null;
if (parent instanceof HostInfoDialog)
astor = ((HostInfoDialog) parent).getAstorObject();
tree = new DeviceHierarchy(this, astor, name);
scrollPane1.setViewportView(tree);
scrollPane1.setPreferredSize(new Dimension(400, 400));
scrollPane2.setPreferredSize(new Dimension(400, 400));
//splitPane.setDividerSize(2);
}
//===============================================================
/**
* This method is called from within the constructor to
* initialize the form.
* WARNING: Do NOT modify this code. The content of this method is
* always regenerated by the Form Editor.
*/
//===============================================================
// //GEN-BEGIN:initComponents
private void initComponents() {
javax.swing.JPanel jPanel1 = new javax.swing.JPanel();
javax.swing.JButton cancelBtn = new javax.swing.JButton();
javax.swing.JPanel jPanel2 = new javax.swing.JPanel();
titleLabel = new javax.swing.JLabel();
javax.swing.JSplitPane splitPane = new javax.swing.JSplitPane();
scrollPane1 = new javax.swing.JScrollPane();
scrollPane2 = new javax.swing.JScrollPane();
textArea = new javax.swing.JTextArea();
addWindowListener(new java.awt.event.WindowAdapter() {
public void windowClosing(java.awt.event.WindowEvent evt) {
closeDialog(evt);
}
});
cancelBtn.setText("Dismiss");
cancelBtn.addActionListener(new java.awt.event.ActionListener() {
public void actionPerformed(java.awt.event.ActionEvent evt) {
cancelBtnActionPerformed(evt);
}
});
jPanel1.add(cancelBtn);
getContentPane().add(jPanel1, java.awt.BorderLayout.SOUTH);
titleLabel.setFont(new java.awt.Font("Dialog", 1, 18));
titleLabel.setText("Dialog Title");
jPanel2.add(titleLabel);
getContentPane().add(jPanel2, java.awt.BorderLayout.NORTH);
splitPane.setLeftComponent(scrollPane1);
textArea.setColumns(20);
textArea.setEditable(false);
textArea.setFont(new java.awt.Font("Monospaced", 1, 12)); // NOI18N
textArea.setRows(5);
scrollPane2.setViewportView(textArea);
splitPane.setRightComponent(scrollPane2);
getContentPane().add(splitPane, java.awt.BorderLayout.CENTER);
pack();
}// //GEN-END:initComponents
//===============================================================
//===============================================================
void setText(String str) {
textArea.setText(str);
}
//===============================================================
//===============================================================
@SuppressWarnings("UnusedParameters")
private void cancelBtnActionPerformed(java.awt.event.ActionEvent evt) {//GEN-FIRST:event_cancelBtnActionPerformed
doClose();
}//GEN-LAST:event_cancelBtnActionPerformed
//===============================================================
//===============================================================
@SuppressWarnings("UnusedParameters")
private void closeDialog(java.awt.event.WindowEvent evt) {//GEN-FIRST:event_closeDialog
doClose();
}//GEN-LAST:event_closeDialog
//===============================================================
//===============================================================
void update() {
try {
AstorUtil.startSplash("Device Hierarchy");
initOwnComponents();
AstorUtil.stopSplash();
} catch (DevFailed e) {
AstorUtil.stopSplash();
ErrorPane.showErrorMessage(this, null, e);
}
}
//===============================================================
/**
* Closes the dialog
*/
//===============================================================
private void doClose() {
if (parent!=null) {
setVisible(false);
tree.stopThread();
dispose();
} else
System.exit(0);
}
//===============================================================
// Variables declaration - do not modify//GEN-BEGIN:variables
private javax.swing.JScrollPane scrollPane1;
private javax.swing.JScrollPane scrollPane2;
private javax.swing.JTextArea textArea;
private javax.swing.JLabel titleLabel;
// End of variables declaration//GEN-END:variables
//===============================================================
//===============================================================
/**
* @param args the command line arguments
*/
//===============================================================
public static void main(String args[]) {
//String serverName = "VacTemperature/c24-wago";
//String serverName = "VacGaugeServer/sr_c27-ip";
//String serverName = "TangoTest/taptap";
//String serverName = "srmag/sqp-sd1/c31-b";
//String serverName = "srdiag/bpm/all";
String serverName = "WhistBunchClock/ctrm";
//String serverName = "PLCmodbus/d23";
//String serverName = "sys/mstatus/rf-cav";
if (args.length > 0)
serverName = args[0];
try {
DeviceHierarchyDialog dlg =
new DeviceHierarchyDialog((JFrame) null, serverName);
dlg.setLocationRelativeTo(null);
dlg.setVisible(true);
} catch (DevFailed e) {
ErrorPane.showErrorMessage(new Frame(), null, e);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy