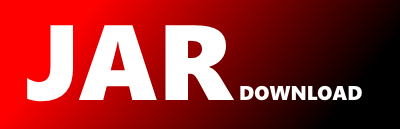
admin.astor.tools.PopupText Maven / Gradle / Ivy
//+======================================================================
// $Source: $
//
// Project: Tango
//
// Description: java source code for Tango manager tool..
//
// $Author$
//
// Copyright (C) : 2004,2005,2006,2007,2008,2009,2010,2011,2012,2013,2014,2015,
// European Synchrotron Radiation Facility
// BP 220, Grenoble 38043
// FRANCE
//
// This file is part of Tango.
//
// Tango is free software: you can redistribute it and/or modify
// it under the terms of the GNU General Public License as published by
// the Free Software Foundation, either version 3 of the License, or
// (at your option) any later version.
//
// Tango is distributed in the hope that it will be useful,
// but WITHOUT ANY WARRANTY; without even the implied warranty of
// MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
// GNU General Public License for more details.
//
// You should have received a copy of the GNU General Public License
// along with Tango. If not, see .
//
// $Revision$
//
//-======================================================================
package admin.astor.tools;
import java.awt.Color;
import java.awt.Dimension;
import java.awt.Font;
import java.awt.Window;
import javax.swing.JFrame;
import javax.swing.text.BadLocationException;
import javax.swing.text.Document;
import javax.swing.text.SimpleAttributeSet;
import javax.swing.text.StyleConstants;
/**
* Display a message in a scrolled JText
*
* @author tappret
*/
public class PopupText extends javax.swing.JFrame {
Window parent = null;
//======================================================
public PopupText(Window parent,String titre) {
this(parent);
setTitle(titre);
}
public PopupText(Window parent) {
super();
this.parent = parent;
initComponents();
setIconImage(Utils.getAstorIcon().getImage());
jLabelTitle.setVisible(false);
setLocationRelativeTo(parent);
}
//======================================================
//======================================================
@SuppressWarnings("unchecked")
// //GEN-BEGIN:initComponents
private void initComponents() {
jScrollPane = new javax.swing.JScrollPane();
jTextArea = new javax.swing.JTextArea();
jButtonDismiss = new javax.swing.JButton();
jLabelTitle = new javax.swing.JLabel();
setDefaultCloseOperation(javax.swing.WindowConstants.DISPOSE_ON_CLOSE);
jTextArea.setEditable(false);
jTextArea.setColumns(20);
jTextArea.setRows(5);
jScrollPane.setViewportView(jTextArea);
jButtonDismiss.setText("Dismiss");
jButtonDismiss.addActionListener(new java.awt.event.ActionListener() {
public void actionPerformed(java.awt.event.ActionEvent evt) {
jButtonDismissActionPerformed(evt);
}
});
jLabelTitle.setText("jLabel1");
javax.swing.GroupLayout layout = new javax.swing.GroupLayout(getContentPane());
getContentPane().setLayout(layout);
layout.setHorizontalGroup(
layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addComponent(jScrollPane, javax.swing.GroupLayout.DEFAULT_SIZE, 413, Short.MAX_VALUE)
.addGroup(javax.swing.GroupLayout.Alignment.TRAILING, layout.createSequentialGroup()
.addContainerGap(javax.swing.GroupLayout.DEFAULT_SIZE, Short.MAX_VALUE)
.addComponent(jButtonDismiss)
.addContainerGap())
.addGroup(layout.createSequentialGroup()
.addContainerGap()
.addComponent(jLabelTitle)
.addContainerGap(javax.swing.GroupLayout.DEFAULT_SIZE, Short.MAX_VALUE))
);
layout.setVerticalGroup(
layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addGroup(layout.createSequentialGroup()
.addContainerGap()
.addComponent(jLabelTitle)
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED)
.addComponent(jScrollPane, javax.swing.GroupLayout.DEFAULT_SIZE, 293, Short.MAX_VALUE)
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED)
.addComponent(jButtonDismiss)
.addContainerGap())
);
pack();
}// //GEN-END:initComponents
private void jButtonDismissActionPerformed(java.awt.event.ActionEvent evt) {//GEN-FIRST:event_jButtonDismissActionPerformed
doClose();
}//GEN-LAST:event_jButtonDismissActionPerformed
//============================================================
//============================================================
public void setFont(Font font) {
jTextArea.setFont(font);
}
//============================================================
//============================================================
@SuppressWarnings("UnusedParameters")
private void closeDialog(java.awt.event.WindowEvent evt) {//GEN-FIRST:event_closeDialog
doClose();
}//GEN-LAST:event_closeDialog
//============================================================
//============================================================
private void doClose() {
setVisible(false);
dispose();
}
//============================================================
/**
* Insert the message in the TextPane with its attributes
*/
//============================================================
private void showMsg(String text, SimpleAttributeSet attrs) {
Document doc = jTextArea.getDocument();
try {
doc.insertString(doc.getLength(), text, attrs);
} catch (BadLocationException ex) {
ex.printStackTrace();
}
}
//============================================================
/**
* format the text and Popup the window.
*/
//============================================================
@SuppressWarnings("unused")
public void showFormatted(String text) {
int nbLines = 0;
int start = 0;
int end;
while ((end = text.indexOf('\n', start)) >= 0) {
// Get line after line
end++;
String line = text.substring(start, end);
SimpleAttributeSet attrs = new SimpleAttributeSet();
// Check if title
if (line.indexOf(':') < 0)
StyleConstants.setBold(attrs, false);
else
StyleConstants.setBold(attrs, true);
// Check if state
int start1;
if ((start1 = line.indexOf("Running")) > 0) {
showMsg(line.substring(0, start1), attrs);
StyleConstants.setForeground(attrs, Color.green);
showMsg(line.substring(start1), attrs);
} else if ((start1 = line.indexOf("Stopped")) > 0) {
showMsg(line.substring(0, start1), attrs);
StyleConstants.setForeground(attrs, Color.red);
showMsg(line.substring(start1), attrs);
} else {
StyleConstants.setForeground(attrs, Color.black);
showMsg(line, attrs);
}
start = end;
nbLines++;
}
jTextArea.setEditable(false);
jScrollPane.setPreferredSize(new Dimension(450, 350));
pack();
setVisible(true);
}
//============================================================
//============================================================
@Override
public void setTitle(String title) {
super.setTitle(title);
jLabelTitle.setText(title);
jLabelTitle.setVisible(true);
}
//============================================================
/**
* Display a message in a scrolled JText dialog.
*/
//============================================================
public void show(String text) {
jTextArea.setText(text);
jScrollPane.setPreferredSize(new Dimension(450, 350));
pack();
setVisible(true);
}
//============================================================
/**
* Display a message in a scrolled JText dialog.
*/
//============================================================
public void show(String title, String[] array, int width, int height) {
// Display title
SimpleAttributeSet attrs = new SimpleAttributeSet();
int fs = StyleConstants.getFontSize(attrs);
StyleConstants.setBold(attrs, true);
StyleConstants.setUnderline(attrs, true);
StyleConstants.setFontSize(attrs, fs + 4);
showMsg(title + "\n\n", attrs);
StyleConstants.setBold(attrs, false);
StyleConstants.setUnderline(attrs, false);
StyleConstants.setFontSize(attrs, fs);
// Display lines
for (String str : array)
showMsg(str + "\n", attrs);
jScrollPane.setPreferredSize(new Dimension(width, height));
pack();
setVisible(true);
}
//============================================================
/**
* Display a message in a scrolled JText dialog.
*/
//============================================================
public void show(String title, String[] array) {
show(title, array, 800, 600);
}
//============================================================
/**
* Display a message in a scrolled JText dialog and set dimentions.
*/
//============================================================
public void show(String text, int sizeX, int sizeY) {
jTextArea.setText(text);
setPreferredSize(new Dimension(sizeX, sizeY));
pack();
setLocationRelativeTo(parent);
setVisible(true);
}
//============================================================
//============================================================
public void setSize(int width, int height) {
jScrollPane.setPreferredSize(new Dimension(width, height));
pack();
}
//============================================================
//============================================================
public void addText(String text) {
SimpleAttributeSet attrs = new SimpleAttributeSet();
int fs = StyleConstants.getFontSize(attrs);
StyleConstants.setBold(attrs, false);
StyleConstants.setUnderline(attrs, false);
StyleConstants.setFontSize(attrs, fs);
showMsg(text + "\n", attrs);
}
//============================================================
/**
* @param args the command line arguments
*/
//============================================================
public static void main(String args[]) {
/* Create and display the form */
java.awt.EventQueue.invokeLater(new Runnable() {
@Override
public void run() {
PopupText pt = new PopupText(new JFrame());
//String text = ""
//pt.show(text);
pt.show("My Title", new String[]{"Line #1", "line #2", "Bla bla bla !"});
}
});
}
// Variables declaration - do not modify//GEN-BEGIN:variables
private javax.swing.JButton jButtonDismiss;
private javax.swing.JLabel jLabelTitle;
private javax.swing.JScrollPane jScrollPane;
private javax.swing.JTextArea jTextArea;
// End of variables declaration//GEN-END:variables
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy