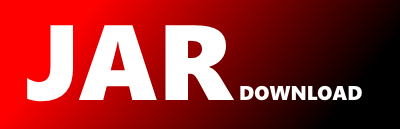
admin.astor.tools.TopDialog Maven / Gradle / Ivy
//+======================================================================
// $Source: $
//
// Project: Tango
//
// Description: java source code for Tango manager tool..
//
// $Author$
//
// Copyright (C) : 2004,2005,2006,2007,2008,2009,2010,2011,2012,2013,2014,2015,
// European Synchrotron Radiation Facility
// BP 220, Grenoble 38043
// FRANCE
//
// This file is part of Tango.
//
// Tango is free software: you can redistribute it and/or modify
// it under the terms of the GNU General Public License as published by
// the Free Software Foundation, either version 3 of the License, or
// (at your option) any later version.
//
// Tango is distributed in the hope that it will be useful,
// but WITHOUT ANY WARRANTY; without even the implied warranty of
// MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
// GNU General Public License for more details.
//
// You should have received a copy of the GNU General Public License
// along with Tango. If not, see .
//
// $Revision$
//
//-======================================================================
package admin.astor.tools;
import fr.esrf.tangoatk.widget.util.ATKGraphicsUtils;
import javax.swing.*;
import java.awt.*;
import java.util.Collections;
import java.util.Comparator;
import java.util.ArrayList;
import java.util.List;
/**
* JDialog Class to display info
*
* @author Pascal Verdier
*/
@SuppressWarnings("MagicConstant")
public class TopDialog extends JDialog {
private BlackBoxTable.BlackBox blackBox;
private List textAreaList;
private String deviceName;
//===============================================================
/**
* Creates new form TopDialog
*/
//===============================================================
public TopDialog(JDialog parent, String deviceName, BlackBoxTable.BlackBox blackBox) {
super(parent, false);
this.blackBox = blackBox;
this.deviceName = deviceName;
initComponents();
textAreaList = new ArrayList<>();
textAreaList.add(null); // DATE is not used
textAreaList.add(operationTxt);
textAreaList.add(nameTxt);
textAreaList.add(sourceTxt);
textAreaList.add(hostTxt);
textAreaList.add(processTxt);
for (int i = BlackBoxTable.OPERATION; i <= BlackBoxTable.PROCESS; i++) {
textAreaList.get(i).getParent().setPreferredSize(new Dimension(500, 350));
textAreaList.get(i).setFont(new Font("dialog", Font.BOLD, 12));
}
tabbedPane.setSelectedIndex(BlackBoxTable.PROCESS - 1); // -1 for DATES not used
pack();
ATKGraphicsUtils.centerDialog(this);
}
//===============================================================
//===============================================================
void displayTop() {
if (blackBox== null)
return;
for (int i = BlackBoxTable.OPERATION; i <= BlackBoxTable.PROCESS; i++)
textAreaList.get(i).setText(computeTop(i).toString());
titleLabel.setText("Top on " + deviceName + " during " + blackBox.getDeltaTimeStr());
}
//===============================================================
//===============================================================
private TopObject computeTop(int index) {
TopObject topObject = new TopObject();
for (int i = 0; i < blackBox.nbRecords(); i++) {
ArrayList line = blackBox.getLine(i);
String item = line.get(index);
if (index==BlackBoxTable.PROCESS) {
if (item.isEmpty())
item = "? ?";
item += " (" + line.get(BlackBoxTable.HOST) + ")";
}
// Check if already in list
boolean found = false;
for (ArrayList lineItems : topObject) {
if (lineItems.get(0).equals(item)) {
lineItems.add(item);
found = true;
}
}
// If not -> add it in object
if (!found) {
ArrayList lineItems = new ArrayList<>();
lineItems.add(item);
topObject.add(lineItems);
}
}
// Sort for size order
Collections.sort(topObject, new StringListComparator());
return topObject;
}
//===============================================================
/**
* This method is called from within the constructor to
* initialize the form.
* WARNING: Do NOT modify this code. The content of this method is
* always regenerated by the Form Editor.
*/
//===============================================================
// //GEN-BEGIN:initComponents
private void initComponents() {
javax.swing.JPanel jPanel1 = new javax.swing.JPanel();
javax.swing.JButton cancelBtn = new javax.swing.JButton();
javax.swing.JPanel jPanel2 = new javax.swing.JPanel();
titleLabel = new javax.swing.JLabel();
tabbedPane = new javax.swing.JTabbedPane();
javax.swing.JPanel operationPanel = new javax.swing.JPanel();
javax.swing.JScrollPane jScrollPane1 = new javax.swing.JScrollPane();
operationTxt = new javax.swing.JTextArea();
javax.swing.JPanel namePanel = new javax.swing.JPanel();
javax.swing.JScrollPane jScrollPane2 = new javax.swing.JScrollPane();
nameTxt = new javax.swing.JTextArea();
javax.swing.JPanel sourcePanel = new javax.swing.JPanel();
javax.swing.JScrollPane jScrollPane3 = new javax.swing.JScrollPane();
sourceTxt = new javax.swing.JTextArea();
javax.swing.JPanel hostPanel = new javax.swing.JPanel();
javax.swing.JScrollPane jScrollPane4 = new javax.swing.JScrollPane();
hostTxt = new javax.swing.JTextArea();
javax.swing.JPanel pocessPanel = new javax.swing.JPanel();
javax.swing.JScrollPane jScrollPane5 = new javax.swing.JScrollPane();
processTxt = new javax.swing.JTextArea();
addWindowListener(new java.awt.event.WindowAdapter() {
public void windowClosing(java.awt.event.WindowEvent evt) {
closeDialog(evt);
}
});
cancelBtn.setText("Dismiss");
cancelBtn.addActionListener(new java.awt.event.ActionListener() {
public void actionPerformed(java.awt.event.ActionEvent evt) {
cancelBtnActionPerformed(evt);
}
});
jPanel1.add(cancelBtn);
getContentPane().add(jPanel1, java.awt.BorderLayout.SOUTH);
titleLabel.setFont(new java.awt.Font("Dialog", 1, 18));
titleLabel.setText("Dialog Title");
jPanel2.add(titleLabel);
getContentPane().add(jPanel2, java.awt.BorderLayout.NORTH);
operationPanel.setLayout(new java.awt.BorderLayout());
operationTxt.setColumns(20);
operationTxt.setEditable(false);
operationTxt.setRows(5);
jScrollPane1.setViewportView(operationTxt);
operationPanel.add(jScrollPane1, java.awt.BorderLayout.CENTER);
tabbedPane.addTab("Operation", operationPanel);
namePanel.setLayout(new java.awt.BorderLayout());
nameTxt.setColumns(20);
nameTxt.setEditable(false);
nameTxt.setRows(5);
jScrollPane2.setViewportView(nameTxt);
namePanel.add(jScrollPane2, java.awt.BorderLayout.CENTER);
tabbedPane.addTab("Name", namePanel);
sourcePanel.setLayout(new java.awt.BorderLayout());
sourceTxt.setColumns(20);
sourceTxt.setEditable(false);
sourceTxt.setRows(5);
jScrollPane3.setViewportView(sourceTxt);
sourcePanel.add(jScrollPane3, java.awt.BorderLayout.CENTER);
tabbedPane.addTab("Source", sourcePanel);
hostPanel.setLayout(new java.awt.BorderLayout());
hostTxt.setColumns(20);
hostTxt.setEditable(false);
hostTxt.setRows(5);
jScrollPane4.setViewportView(hostTxt);
hostPanel.add(jScrollPane4, java.awt.BorderLayout.CENTER);
tabbedPane.addTab("Host", hostPanel);
pocessPanel.setLayout(new java.awt.BorderLayout());
processTxt.setColumns(20);
processTxt.setEditable(false);
processTxt.setRows(5);
jScrollPane5.setViewportView(processTxt);
pocessPanel.add(jScrollPane5, java.awt.BorderLayout.CENTER);
tabbedPane.addTab("Process", pocessPanel);
getContentPane().add(tabbedPane, java.awt.BorderLayout.CENTER);
pack();
}// //GEN-END:initComponents
//===============================================================
//===============================================================
@SuppressWarnings({"UnusedParameters"})
private void cancelBtnActionPerformed(java.awt.event.ActionEvent evt) {//GEN-FIRST:event_cancelBtnActionPerformed
doClose();
}//GEN-LAST:event_cancelBtnActionPerformed
//===============================================================
//===============================================================
@SuppressWarnings({"UnusedParameters"})
private void closeDialog(java.awt.event.WindowEvent evt) {//GEN-FIRST:event_closeDialog
doClose();
}//GEN-LAST:event_closeDialog
//===============================================================
/**
* Closes the dialog
*/
//===============================================================
private void doClose() {
setVisible(false);
dispose();
}
//===============================================================
//===============================================================
public void showDialog() {
displayTop();
setVisible(true);
}
//===============================================================
// Variables declaration - do not modify//GEN-BEGIN:variables
private javax.swing.JTextArea hostTxt;
private javax.swing.JTextArea nameTxt;
private javax.swing.JTextArea operationTxt;
private javax.swing.JTextArea processTxt;
private javax.swing.JTextArea sourceTxt;
private javax.swing.JTabbedPane tabbedPane;
private javax.swing.JLabel titleLabel;
// End of variables declaration//GEN-END:variables
//===============================================================
//======================================================
//======================================================
private class TopObject extends ArrayList> {
//======================================================
private double[] computeRatios() {
int sum = 0;
double [] ratios = new double[size()];
for (List line : this) {
sum += line.size();
}
int i = 0;
for (List line : this) {
ratios[i++] = 100.0*line.size()/sum;
}
return ratios;
}
//======================================================
public String toString() {
double [] ratios = computeRatios();
int i=0;
StringBuilder sb = new StringBuilder();
for (List line : this)
sb.append(String.format("%3d", line.size()))
.append(" calls(").append(String.format("%5.1f", ratios[i++]))
.append(" %):\t").append(line.get(0)).append('\n');
return sb.toString();
}
}
//======================================================
//======================================================
//======================================================
/**
* StringListComparator class to sort by size
*/
//======================================================
class StringListComparator implements Comparator> {
public int compare(ArrayList list1, ArrayList list2) {
if (list1.size() == list2.size()) return 0;
return ((list1.size() < list2.size())? 1 : -1);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy