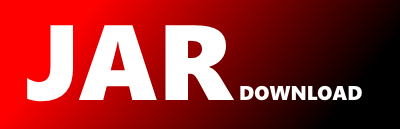
admin.astor.tools.Utils Maven / Gradle / Ivy
//+======================================================================
// $Source: $
//
// Project: Tango
//
// Description: java source code for Tango manager tool..
//
// $Author$
//
// Copyright (C) : 2004,2005,2006,2007,2008,2009,2010,2011,2012,2013,2014,2015,
// European Synchrotron Radiation Facility
// BP 220, Grenoble 38043
// FRANCE
//
// This file is part of Tango.
//
// Tango is free software: you can redistribute it and/or modify
// it under the terms of the GNU General Public License as published by
// the Free Software Foundation, either version 3 of the License, or
// (at your option) any later version.
//
// Tango is distributed in the hope that it will be useful,
// but WITHOUT ANY WARRANTY; without even the implied warranty of
// MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
// GNU General Public License for more details.
//
// You should have received a copy of the GNU General Public License
// along with Tango. If not, see .
//
// $Revision$
//
//-======================================================================
package admin.astor.tools;
import fr.esrf.tangoatk.core.ATKException;
import fr.esrf.tangoatk.widget.util.ErrorPane;
import javax.swing.*;
import java.awt.*;
/**
* This class is a set of tools
*
* @author verdier
*/
public class Utils {
static private Utils instance = null;
static public final String img_path = "/admin/astor/images/";
static private final ImageIcon astorIcon = getIcon("astor.png");
static private final ImageIcon mySqlIcon = getIcon("MySql.png");
static private final ImageIcon tangoIcon = getIcon("TangoLogo.png");
static private final ImageIcon orangeTriangleIcon = getIcon("orangeTriangle.png");
static private final ImageIcon failedIcon = getIcon("failed.gif");
static private final ImageIcon tangoClassIcon = getIcon("TangoClass.png");
static private final ImageIcon serverIcon = getIcon("server.gif");
static private final ImageIcon classIcon = getIcon("class.gif");
static private final ImageIcon deviceIcon = getIcon("device.gif");
static private final ImageIcon tangoTansparIcon = getIcon("TransparentTango.png");
static private final ImageIcon warningIcon = getIcon("warning.gif");
static private final ImageIcon attleafIcon = getIcon("attleaf.gif");
static private final ImageIcon leafIcon = getIcon("leaf.gif");
static private final ImageIcon uleafIcon = getIcon("uleaf.gif");
static private final ImageIcon userIcon = getIcon("user.gif");
static private final ImageIcon clockIcon = getIcon("clock.gif");
static private final ImageIcon upIcon = getIcon("up.gif");
static private final ImageIcon downIcon = getIcon("down.gif");
static private final ImageIcon principleIcon = getIcon("principle.gif");
static private final Cursor drgdrgCursor = getCursor("drg-drp.gif");
//===============================================================
//===============================================================
private Utils() {
}
public static ImageIcon getAstorIcon(){
return astorIcon;
}
public static ImageIcon getMySqlIcon(){
return mySqlIcon;
}
public static ImageIcon getTangoIcon(){
return tangoIcon;
}
public static ImageIcon getOrangeTriangleIcon(){
return orangeTriangleIcon;
}
public static ImageIcon getFailedIcon(){
return failedIcon;
}
public static ImageIcon getTangoClassIcon(){
return tangoClassIcon;
}
public static ImageIcon getServerIcon(){
return serverIcon;
}
public static ImageIcon getClassIcon(){
return classIcon;
}
public static ImageIcon getDeviceIcon(){
return deviceIcon;
}
public static ImageIcon getTangoTansparIcon(){
return tangoTansparIcon;
}
public static ImageIcon getWarningIcon(){
return warningIcon;
}
public static ImageIcon getAttLeafIcon(){
return attleafIcon;
}
public static ImageIcon getLeafIcon(){
return leafIcon;
}
public static ImageIcon getULeafIcon(){
return uleafIcon;
}
public static ImageIcon getUserIcon(){
return userIcon;
}
public static ImageIcon getClockIcon(){
return clockIcon;
}
public static ImageIcon getUpIcon(){
return upIcon;
}
public static ImageIcon getDownIcon(){
return downIcon;
}
public static ImageIcon getPrincipleIcon(){
return principleIcon;
}
public static Cursor getDrgdrgCursor(){
return drgdrgCursor;
}
//===============================================================
//===============================================================
static public Utils getInstance() {
if (instance == null)
instance = new Utils();
return instance;
}
//===============================================================
//===============================================================
private static ImageIcon getIcon(String fileName) {
java.net.URL url = getImageUrl(fileName);
if (url == null) {
System.err.println("WARNING: " + img_path + fileName + " : File not found");
return new ImageIcon();
}
return new ImageIcon(url);
}
//===============================================================
//===============================================================
static private Cursor getCursor(String fileName) {
java.net.URL url = getImageUrl(fileName);
Image image = Toolkit.getDefaultToolkit().getImage(url);
return Toolkit.getDefaultToolkit().createCustomCursor(image, new Point(0, 0), fileName);
}
//===============================================================
//===============================================================
private static java.net.URL getImageUrl(String filename) {
return Utils.class.getResource(img_path + filename);
}
//===============================================================
//===============================================================
public static ImageIcon getResizedIcon(ImageIcon icon, double ratio) {
if (icon != null) {
int width = icon.getIconWidth();
int height = icon.getIconHeight();
width = (int) (ratio * width);
height = (int) (ratio * height);
icon = new ImageIcon(
icon.getImage().getScaledInstance(
width, height, Image.SCALE_SMOOTH));
}
return icon;
}
//===============================================================
//===============================================================
static public void popupMessage(Component c, String message, ImageIcon icon) {
JOptionPane.showMessageDialog(c, message, "Info Window", JOptionPane.INFORMATION_MESSAGE, icon);
}
//===============================================================
//===============================================================
static public void popupMessage(Component c, String message) {
JOptionPane.showMessageDialog(c, message, "Info Window", JOptionPane.INFORMATION_MESSAGE);
}
//===============================================================
//===============================================================
static public void popupError(Component c, String message, Exception e) {
ErrorPane.showErrorMessage(c, message, e);
}
//===============================================================
//===============================================================
static public void popupError(Component c, String message) {
ErrorPane.showErrorMessage(c, null, new ATKException(message));
}
//===============================================================
//===============================================================
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy