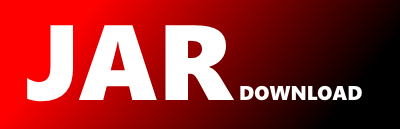
fr.esrf.TangoApi.ApiUtil Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of JTangoCommons Show documentation
Show all versions of JTangoCommons Show documentation
Common classes for tango java API
//+======================================================================
// $Source$
//
// Project: Tango
//
// Description: java source code for the TANGO client/server API.
//
// $Author: pascal_verdier $
//
// Copyright (C) : 2004,2005,2006,2007,2008,2009,2010,2011,2012,2013,2014,
// European Synchrotron Radiation Facility
// BP 220, Grenoble 38043
// FRANCE
//
// This file is part of Tango.
//
// Tango is free software: you can redistribute it and/or modify
// it under the terms of the GNU Lesser General Public License as published by
// the Free Software Foundation, either version 3 of the License, or
// (at your option) any later version.
//
// Tango is distributed in the hope that it will be useful,
// but WITHOUT ANY WARRANTY; without even the implied warranty of
// MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
// GNU Lesser General Public License for more details.
//
// You should have received a copy of the GNU Lesser General Public License
// along with Tango. If not, see .
//
// $Revision: 28157 $
//
//-======================================================================
package fr.esrf.TangoApi;
import fr.esrf.Tango.AttrQuality;
import fr.esrf.Tango.DevFailed;
import fr.esrf.Tango.DevState;
import fr.esrf.Tango.factory.TangoFactory;
import fr.esrf.TangoDs.Except;
import org.omg.CORBA.ORB;
import org.omg.CORBA.Request;
import java.io.FileOutputStream;
import java.util.*;
/**
* Class Description: This class manage a static vector of Database object.
*
*
* Usage example:
*
* Database dbase = ApiUtil.get_db_obj();
*
*
*
* @author verdier
* @version $Revision: 28157 $
*/
public class ApiUtil {
//TODO replace with package.getImplementationVersion
public static String revNumber =
"9.1.0 - Wed Jun 24 14:15:07 CEST 2015";
/**
* ORB object reference for connection.
*/
static protected ORB orb = null;
private static IApiUtilDAO apiutilDAO = TangoFactory.getSingleton().getApiUtilDAO();
private static int hwmValue = 0;
/**
* @return true if use the default factory (new ObjectDAODefaultImpl or
* false if introspection factory (mainly used when from web)
*/
// ===================================================================
public static boolean useDefaultFactory() {
return TangoFactory.getSingleton().isDefaultFactory();
}
/**
* Return the database object created for specified host and port.
*
* @param tango_host
* host and port (hostname:portnumber) where database is running.
*/
// ===================================================================
public static Database get_db_obj(final String tango_host) throws DevFailed {
return apiutilDAO.get_db_obj(tango_host);
}
// ===================================================================
/**
* Return the database object created with TANGO_HOST environment variable .
*/
// ===================================================================
public static Database get_default_db_obj() throws DevFailed {
return apiutilDAO.get_default_db_obj();
}
// ===================================================================
/**
* Return tru if the database object has been created.
*/
// ===================================================================
public static boolean default_db_obj_exists() throws DevFailed {
return apiutilDAO.default_db_obj_exists();
}
// ===================================================================
/**
* Return the database object created with TANGO_HOST environment variable .
*/
// ===================================================================
public static synchronized Database get_db_obj() throws DevFailed {
return apiutilDAO.get_db_obj();
}
// ===================================================================
/**
* Return the database object created for specified host and port.
*
* @param host host where database is running.
* @param port port for database connection.
*/
// ===================================================================
public static Database get_db_obj(final String host, final String port) throws DevFailed {
return apiutilDAO.get_db_obj(host, port);
}
// ===================================================================
/**
* Return the database object created for specified host and port, and set
* this database object for all following uses..
*
* @param host host where database is running.
* @param port port for database connection.
*/
// ===================================================================
public static Database change_db_obj(final String host, final String port) throws DevFailed {
return apiutilDAO.change_db_obj(host, port);
}
// ===================================================================
/**
* Return the database object created for specified host and port, and set
* this database object for all following uses..
*
* @param host host where database is running.
* @param port port for database connection.
*/
// ===================================================================
public static Database set_db_obj(final String host, final String port) throws DevFailed {
return apiutilDAO.set_db_obj(host, port);
}
// ===================================================================
/**
* Return the database object created for specified host and port.
*
* @param tango_host
* host and port (hostname:portnumber) where database is running.
*/
// ===================================================================
public static Database set_db_obj(final String tango_host) throws DevFailed {
return apiutilDAO.set_db_obj(tango_host);
}
// ===================================================================
/**
* Return the orb object
*/
// ===================================================================
public static ORB get_orb() throws DevFailed {
return apiutilDAO.get_orb();
}
// ===================================================================
/**
* Return the host address.
*/
// ===================================================================
public static String getHostAddress() throws DevFailed {
return HostInfo.getAddress();
}
// ===================================================================
// ===================================================================
// ===================================================================
/**
* Return the host addresses.
*/
// ===================================================================
public static Vector getHostAddresses() throws DevFailed {
return HostInfo.getAddresses();
}
// ===================================================================
/**
* Return the host name.
*/
// ===================================================================
public static String getHostName() throws DevFailed {
return HostInfo.getName();
}
// ===================================================================
/**
* Return the orb object
*/
// ===================================================================
public static void set_in_server(final boolean val) {
apiutilDAO.set_in_server(val);
}
// ===================================================================
/**
* Return true if in server code or false if in client code.
*/
// ===================================================================
public static boolean in_server() {
return apiutilDAO.in_server();
}
// ===================================================================
public static int getReconnectionDelay() {
return apiutilDAO.getReconnectionDelay();
}
// ===================================================================
/**
* Add request in hash table and return id
*/
// ==========================================================================
public static int put_async_request(final AsyncCallObject aco) {
return apiutilDAO.put_async_request(aco);
}
/**
* Return the request in hash table for the id
*
* @throws DevFailed
*/
// ==========================================================================
public static Request get_async_request(final int id) throws DevFailed {
return apiutilDAO.get_async_request(id);
}
// ==========================================================================
/*
* Asynchronous request management
*/
// ==========================================================================
// ==========================================================================
/**
* Return the Asynch Object in hash table for the id
*/
// ==========================================================================
public static AsyncCallObject get_async_object(final int id) {
return apiutilDAO.get_async_object(id);
}
// ==========================================================================
/**
* Remove asynchronous call request and id from hashtable.
*/
// ==========================================================================
public static void remove_async_request(final int id) {
apiutilDAO.remove_async_request(id);
}
// ==========================================================================
/**
* Set the reply_model in AsyncCallObject for the id key.
*/
// ==========================================================================
public static void set_async_reply_model(final int id, final int reply_model) {
apiutilDAO.set_async_reply_model(id, reply_model);
}
// ==========================================================================
/**
* Set the Callback object in AsyncCallObject for the id key.
*/
// ==========================================================================
public static void set_async_reply_cb(final int id, final CallBack cb) {
apiutilDAO.set_async_reply_cb(id, cb);
}
// ==========================================================================
/**
* return the still pending asynchronous call for a reply model.
*
* @param dev DeviceProxy object.
* @param reply_model ApiDefs.ALL_ASYNCH, POLLING or CALLBACK.
*/
// ==========================================================================
public static int pending_asynch_call(final DeviceProxy dev, final int reply_model) {
return apiutilDAO.pending_asynch_call(dev, reply_model);
}
// ==========================================================================
/**
* return the still pending asynchronous call for a reply model.
*
* @param reply_model ApiDefs.ALL_ASYNCH, POLLING or CALLBACK.
*/
// ==========================================================================
public static int pending_asynch_call(final int reply_model) {
return apiutilDAO.pending_asynch_call(reply_model);
}
// ==========================================================================
/**
* Set the callback sub model used (ApiDefs.PUSH_CALLBACK or
* ApiDefs.PULL_CALLBACK).
*/
// ==========================================================================
public static int get_asynch_cb_sub_model() {
return apiutilDAO.get_asynch_cb_sub_model();
}
// ==========================================================================
/**
* Return the callback sub model used.
*
* @param model ApiDefs.PUSH_CALLBACK or ApiDefs.PULL_CALLBACK.
*/
// ==========================================================================
public static void set_asynch_cb_sub_model(final int model) {
apiutilDAO.set_asynch_cb_sub_model(model);
}
// ==========================================================================
/**
* Fire callback methods for all (any device) asynchronous requests(cmd and
* attr) with already arrived replies.
*/
// ==========================================================================
public static void get_asynch_replies() {
apiutilDAO.get_asynch_replies();
}
// ==========================================================================
/**
* Fire callback methods for all (any device) asynchronous requests(cmd and
* attr) with already arrived replies.
*/
// ==========================================================================
public static void get_asynch_replies(final int timeout) {
apiutilDAO.get_asynch_replies(timeout);
}
// ==========================================================================
/**
* Fire callback methods for all (any device) asynchronous requests(cmd and
* attr) with already arrived replies.
*/
// ==========================================================================
public static void get_asynch_replies(final DeviceProxy dev) {
apiutilDAO.get_asynch_replies(dev);
}
// ==========================================================================
/**
* Fire callback methods for all (any device) asynchronous requests(cmd and
* attr) with already arrived replies.
*/
// ==========================================================================
public static void get_asynch_replies(final DeviceProxy dev, final int timeout) {
apiutilDAO.get_asynch_replies(dev, timeout);
}
// ==========================================================================
/**
* Convert arguments to one String array
*/
// ==========================================================================
public static String[] toStringArray(final String objname, final String[] argin) {
final String[] array = new String[1 + argin.length];
array[0] = objname;
System.arraycopy(argin, 0, array, 1, argin.length);
return array;
}
// ==========================================================================
/**
* Convert arguments to one String array
*/
// ==========================================================================
public static String[] toStringArray(final String objname, final String argin) {
final String[] array = new String[2];
array[0] = objname;
array[1] = argin;
return array;
}
// ==========================================================================
/*
* Methods to convert data.
*/
// ==========================================================================
// ==========================================================================
/**
* Convert arguments to one String array
*/
// ==========================================================================
public static String[] toStringArray(final String argin) {
final String[] array = new String[1];
array[0] = argin;
return array;
}
// ==========================================================================
/**
* Convert a DbAttribute class array to a StringArray.
*
* @param objname object name (used in first index of output array)..
* @param attributes DbAttribute array to be converted
* @return the String array created from input argument.
*/
// ==========================================================================
public static String[] toStringArray(final String objname, final DbAttribute[] attributes, final int mode) {
final int nb_attr = attributes.length;
// Copy object name and nb attrib to String array
final ArrayList list = new ArrayList();
list.add(objname);
list.add(Integer.toString(nb_attr));
for (DbAttribute attribute : attributes) {
// Copy Attrib name and nb prop to String array
list.add(attribute.name);
list.add("" + attribute.size());
for (int j=0 ; j list = new ArrayList();
list.add(deviceNme);
list.add(Integer.toString(1)); // one pipe
list.add(dbPipe.getName());
list.add(Integer.toString(dbPipe.size())); // property number
// fOR EACH PROPERTY
for (DbDatum datum : dbPipe) {
if (!datum.is_empty()) {
String[] values = datum.extractStringArray();
list.add(datum.name);
list.add(Integer.toString(values.length)); // Property value number (array case)
Collections.addAll(list, values);
}
}
String[] array = new String[list.size()];
for (int i=0 ; i 2) {
Except.throw_non_supported_exception("API_NotSupportedMode", "Mode " + mode
+ " to decode attribute properties is not supported",
"ApiUtil.toDbAttributeArray()");
}
int idx = 1;
final int nb_attr = Integer.parseInt(array[idx++]);
final DbAttribute[] attr = new DbAttribute[nb_attr];
for (int i = 0; i < nb_attr; i++) {
// Create DbAttribute with name and nb properties
// ------------------------------------------------------
attr[i] = new DbAttribute(array[idx++]);
// Get nb properties
// ------------------------------------------------------
final int nb_prop = Integer.parseInt(array[idx++]);
for (int j = 0; j < nb_prop; j++) {
// And copy property name and value in
// DbAttribute's DbDatum array
// ------------------------------------------
final String p_name = array[idx++];
switch (mode) {
case 1:
// Value is just one element
attr[i].add(p_name, array[idx++]);
break;
case 2:
// value is an array
final int p_length = Integer.parseInt(array[idx++]);
final String[] val = new String[p_length];
for (int p = 0; p < p_length; p++) {
val[p] = array[idx++];
}
attr[i].add(p_name, val);
break;
}
}
}
return attr;
}
// ==========================================================================
/**
* Convert a StringArray to a DbPipe
*
* @param pipeName pip name
* @param array String array to be converted
* @return the DbPipe created from input argument.
* @throws DevFailed if array is not coherent.
*/
// ==========================================================================
public static DbPipe toDbPipe(String pipeName, final String[] array) throws DevFailed {
DbPipe dbPipe = new DbPipe(pipeName);
try {
int index = 3;
final int nbProperties = Integer.parseInt(array[index++]);
for (int i=0 ; i
© 2015 - 2025 Weber Informatics LLC | Privacy Policy