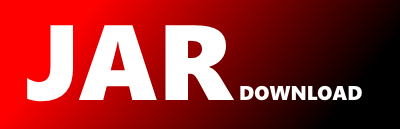
org.tango.utils.ReflectionScanner Maven / Gradle / Ivy
package org.tango.utils;
import java.lang.annotation.Annotation;
import java.lang.reflect.Field;
import java.lang.reflect.Method;
import java.util.HashMap;
import java.util.HashSet;
import java.util.Map;
import java.util.Set;
public class ReflectionScanner {
private final Class> classToScan;
final Map, Set> methods = new HashMap, Set>();
final Map, Set> fields = new HashMap, Set>();
public ReflectionScanner(final Class> classToScan) {
this.classToScan = classToScan;
scanAnnotatedFields();
scanAnnotatedMethods();
}
public Set getMethodsAnnotatedWith(final Class> annototation) {
return methods.get(annototation);
}
public Set getFieldsAnnotatedWith(final Class> annototation) {
return fields.get(annototation);
}
private void scanAnnotatedMethods() {
for (final Method method : classToScan.getDeclaredMethods()) {
final Annotation[] annotations = method.getAnnotations();
for (final Annotation annotation : annotations) {
if (methods.containsKey(annotation.annotationType())) {
methods.get(annotation.annotationType()).add(method);
} else {
final Set list = new HashSet();
list.add(method);
methods.put(annotation.annotationType(), list);
}
}
}
}
private void scanAnnotatedFields() {
for (final Field field : classToScan.getDeclaredFields()) {
final Annotation[] annotations = field.getAnnotations();
for (final Annotation annotation : annotations) {
if (fields.containsKey(annotation.annotationType())) {
fields.get(annotation.annotationType()).add(field);
} else {
final Set list = new HashSet();
list.add(field);
fields.put(annotation.annotationType(), list);
}
}
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy