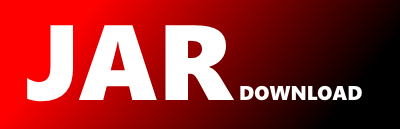
fr.esrf.Tango._DeviceStub Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of JavaTangoIDL Show documentation
Show all versions of JavaTangoIDL Show documentation
Java Tango IDL (compiled with jacorb IDL compiler, http://www.jacorb.org)
The newest version!
package fr.esrf.Tango;
/**
* Generated from IDL interface "Device".
*
* @author JacORB IDL compiler V 3.8
* @version generated at Oct 21, 2017 6:12:33 PM
*/
public class _DeviceStub
extends org.omg.CORBA.portable.ObjectImpl
implements fr.esrf.Tango.Device
{
/** Serial version UID. */
private static final long serialVersionUID = 1L;
private String[] ids = {"IDL:Tango/Device:1.0"};
public String[] _ids()
{
return ids;
}
public final static java.lang.Class _opsClass = fr.esrf.Tango.DeviceOperations.class;
public void write_attributes(fr.esrf.Tango.AttributeValue[] values) throws fr.esrf.Tango.DevFailed
{
while(true)
{
if(! this._is_local())
{
org.omg.CORBA.portable.InputStream _is = null;
org.omg.CORBA.portable.OutputStream _os = null;
try
{
_os = _request( "write_attributes", true);
fr.esrf.Tango.AttributeValueListHelper.write(_os,values);
_is = _invoke(_os);
return;
}
catch( org.omg.CORBA.portable.RemarshalException _rx )
{
continue;
}
catch( org.omg.CORBA.portable.ApplicationException _ax )
{
String _id = _ax.getId();
try
{
if( _id.equals("IDL:Tango/DevFailed:1.0"))
{
throw fr.esrf.Tango.DevFailedHelper.read(_ax.getInputStream());
}
else
{
throw new RuntimeException("Unexpected exception " + _id );
}
}
finally
{
try
{
_ax.getInputStream().close();
}
catch (java.io.IOException e)
{
throw new RuntimeException("Unexpected exception " + e.toString() );
}
}
}
finally
{
if (_os != null)
{
try
{
_os.close();
}
catch (java.io.IOException e)
{
throw new RuntimeException("Unexpected exception " + e.toString() );
}
}
this._releaseReply(_is);
}
}
else
{
org.omg.CORBA.portable.ServantObject _so = _servant_preinvoke( "write_attributes", _opsClass );
if( _so == null )
continue;
DeviceOperations _localServant = (DeviceOperations)_so.servant;
try
{
_localServant.write_attributes(values);
if ( _so instanceof org.omg.CORBA.portable.ServantObjectExt)
((org.omg.CORBA.portable.ServantObjectExt)_so).normalCompletion();
return;
}
catch (fr.esrf.Tango.DevFailed ex)
{
if ( _so instanceof org.omg.CORBA.portable.ServantObjectExt)
((org.omg.CORBA.portable.ServantObjectExt)_so).exceptionalCompletion(ex);
throw ex;
}
catch (RuntimeException re)
{
if ( _so instanceof org.omg.CORBA.portable.ServantObjectExt)
((org.omg.CORBA.portable.ServantObjectExt)_so).exceptionalCompletion(re);
throw re;
}
catch (java.lang.Error err)
{
if ( _so instanceof org.omg.CORBA.portable.ServantObjectExt)
((org.omg.CORBA.portable.ServantObjectExt)_so).exceptionalCompletion(err);
throw err;
}
finally
{
_servant_postinvoke(_so);
}
}
}
}
public java.lang.String name()
{
while(true)
{
if(! this._is_local())
{
org.omg.CORBA.portable.InputStream _is = null;
org.omg.CORBA.portable.OutputStream _os = null;
try
{
_os = _request("_get_name",true);
_is = _invoke(_os);
return _is.read_string();
}
catch( org.omg.CORBA.portable.RemarshalException _rx )
{
continue;
}
catch( org.omg.CORBA.portable.ApplicationException _ax )
{
String _id = _ax.getId();
try
{
_ax.getInputStream().close();
}
catch (java.io.IOException e)
{
throw new RuntimeException("Unexpected exception " + e.toString() );
}
throw new RuntimeException("Unexpected exception " + _id );
}
finally
{
if (_os != null)
{
try
{
_os.close();
}
catch (java.io.IOException e)
{
throw new RuntimeException("Unexpected exception " + e.toString() );
}
}
this._releaseReply(_is);
}
}
else
{
org.omg.CORBA.portable.ServantObject _so = _servant_preinvoke( "_get_name", _opsClass);
if( _so == null )
continue;
DeviceOperations _localServant = (DeviceOperations)_so.servant;
java.lang.String _result;
try
{
_result = _localServant.name();
if ( _so instanceof org.omg.CORBA.portable.ServantObjectExt)
((org.omg.CORBA.portable.ServantObjectExt)_so).normalCompletion();
return _result;
}
catch (RuntimeException re)
{
if ( _so instanceof org.omg.CORBA.portable.ServantObjectExt)
((org.omg.CORBA.portable.ServantObjectExt)_so).exceptionalCompletion(re);
throw re;
}
catch (java.lang.Error err)
{
if ( _so instanceof org.omg.CORBA.portable.ServantObjectExt)
((org.omg.CORBA.portable.ServantObjectExt)_so).exceptionalCompletion(err);
throw err;
}
finally
{
_servant_postinvoke(_so);
}
}
}
}
public void ping() throws fr.esrf.Tango.DevFailed
{
while(true)
{
if(! this._is_local())
{
org.omg.CORBA.portable.InputStream _is = null;
org.omg.CORBA.portable.OutputStream _os = null;
try
{
_os = _request( "ping", true);
_is = _invoke(_os);
return;
}
catch( org.omg.CORBA.portable.RemarshalException _rx )
{
continue;
}
catch( org.omg.CORBA.portable.ApplicationException _ax )
{
String _id = _ax.getId();
try
{
if( _id.equals("IDL:Tango/DevFailed:1.0"))
{
throw fr.esrf.Tango.DevFailedHelper.read(_ax.getInputStream());
}
else
{
throw new RuntimeException("Unexpected exception " + _id );
}
}
finally
{
try
{
_ax.getInputStream().close();
}
catch (java.io.IOException e)
{
throw new RuntimeException("Unexpected exception " + e.toString() );
}
}
}
finally
{
if (_os != null)
{
try
{
_os.close();
}
catch (java.io.IOException e)
{
throw new RuntimeException("Unexpected exception " + e.toString() );
}
}
this._releaseReply(_is);
}
}
else
{
org.omg.CORBA.portable.ServantObject _so = _servant_preinvoke( "ping", _opsClass );
if( _so == null )
continue;
DeviceOperations _localServant = (DeviceOperations)_so.servant;
try
{
_localServant.ping();
if ( _so instanceof org.omg.CORBA.portable.ServantObjectExt)
((org.omg.CORBA.portable.ServantObjectExt)_so).normalCompletion();
return;
}
catch (fr.esrf.Tango.DevFailed ex)
{
if ( _so instanceof org.omg.CORBA.portable.ServantObjectExt)
((org.omg.CORBA.portable.ServantObjectExt)_so).exceptionalCompletion(ex);
throw ex;
}
catch (RuntimeException re)
{
if ( _so instanceof org.omg.CORBA.portable.ServantObjectExt)
((org.omg.CORBA.portable.ServantObjectExt)_so).exceptionalCompletion(re);
throw re;
}
catch (java.lang.Error err)
{
if ( _so instanceof org.omg.CORBA.portable.ServantObjectExt)
((org.omg.CORBA.portable.ServantObjectExt)_so).exceptionalCompletion(err);
throw err;
}
finally
{
_servant_postinvoke(_so);
}
}
}
}
public java.lang.String description()
{
while(true)
{
if(! this._is_local())
{
org.omg.CORBA.portable.InputStream _is = null;
org.omg.CORBA.portable.OutputStream _os = null;
try
{
_os = _request("_get_description",true);
_is = _invoke(_os);
return _is.read_string();
}
catch( org.omg.CORBA.portable.RemarshalException _rx )
{
continue;
}
catch( org.omg.CORBA.portable.ApplicationException _ax )
{
String _id = _ax.getId();
try
{
_ax.getInputStream().close();
}
catch (java.io.IOException e)
{
throw new RuntimeException("Unexpected exception " + e.toString() );
}
throw new RuntimeException("Unexpected exception " + _id );
}
finally
{
if (_os != null)
{
try
{
_os.close();
}
catch (java.io.IOException e)
{
throw new RuntimeException("Unexpected exception " + e.toString() );
}
}
this._releaseReply(_is);
}
}
else
{
org.omg.CORBA.portable.ServantObject _so = _servant_preinvoke( "_get_description", _opsClass);
if( _so == null )
continue;
DeviceOperations _localServant = (DeviceOperations)_so.servant;
java.lang.String _result;
try
{
_result = _localServant.description();
if ( _so instanceof org.omg.CORBA.portable.ServantObjectExt)
((org.omg.CORBA.portable.ServantObjectExt)_so).normalCompletion();
return _result;
}
catch (RuntimeException re)
{
if ( _so instanceof org.omg.CORBA.portable.ServantObjectExt)
((org.omg.CORBA.portable.ServantObjectExt)_so).exceptionalCompletion(re);
throw re;
}
catch (java.lang.Error err)
{
if ( _so instanceof org.omg.CORBA.portable.ServantObjectExt)
((org.omg.CORBA.portable.ServantObjectExt)_so).exceptionalCompletion(err);
throw err;
}
finally
{
_servant_postinvoke(_so);
}
}
}
}
public fr.esrf.Tango.AttributeValue[] read_attributes(java.lang.String[] names) throws fr.esrf.Tango.DevFailed
{
while(true)
{
if(! this._is_local())
{
org.omg.CORBA.portable.InputStream _is = null;
org.omg.CORBA.portable.OutputStream _os = null;
try
{
_os = _request( "read_attributes", true);
fr.esrf.Tango.DevVarStringArrayHelper.write(_os,names);
_is = _invoke(_os);
fr.esrf.Tango.AttributeValue[] _result = fr.esrf.Tango.AttributeValueListHelper.read(_is);
return _result;
}
catch( org.omg.CORBA.portable.RemarshalException _rx )
{
continue;
}
catch( org.omg.CORBA.portable.ApplicationException _ax )
{
String _id = _ax.getId();
try
{
if( _id.equals("IDL:Tango/DevFailed:1.0"))
{
throw fr.esrf.Tango.DevFailedHelper.read(_ax.getInputStream());
}
else
{
throw new RuntimeException("Unexpected exception " + _id );
}
}
finally
{
try
{
_ax.getInputStream().close();
}
catch (java.io.IOException e)
{
throw new RuntimeException("Unexpected exception " + e.toString() );
}
}
}
finally
{
if (_os != null)
{
try
{
_os.close();
}
catch (java.io.IOException e)
{
throw new RuntimeException("Unexpected exception " + e.toString() );
}
}
this._releaseReply(_is);
}
}
else
{
org.omg.CORBA.portable.ServantObject _so = _servant_preinvoke( "read_attributes", _opsClass );
if( _so == null )
continue;
DeviceOperations _localServant = (DeviceOperations)_so.servant;
fr.esrf.Tango.AttributeValue[] _result;
try
{
_result = _localServant.read_attributes(names);
if ( _so instanceof org.omg.CORBA.portable.ServantObjectExt)
((org.omg.CORBA.portable.ServantObjectExt)_so).normalCompletion();
return _result;
}
catch (fr.esrf.Tango.DevFailed ex)
{
if ( _so instanceof org.omg.CORBA.portable.ServantObjectExt)
((org.omg.CORBA.portable.ServantObjectExt)_so).exceptionalCompletion(ex);
throw ex;
}
catch (RuntimeException re)
{
if ( _so instanceof org.omg.CORBA.portable.ServantObjectExt)
((org.omg.CORBA.portable.ServantObjectExt)_so).exceptionalCompletion(re);
throw re;
}
catch (java.lang.Error err)
{
if ( _so instanceof org.omg.CORBA.portable.ServantObjectExt)
((org.omg.CORBA.portable.ServantObjectExt)_so).exceptionalCompletion(err);
throw err;
}
finally
{
_servant_postinvoke(_so);
}
}
}
}
public java.lang.String adm_name()
{
while(true)
{
if(! this._is_local())
{
org.omg.CORBA.portable.InputStream _is = null;
org.omg.CORBA.portable.OutputStream _os = null;
try
{
_os = _request("_get_adm_name",true);
_is = _invoke(_os);
return _is.read_string();
}
catch( org.omg.CORBA.portable.RemarshalException _rx )
{
continue;
}
catch( org.omg.CORBA.portable.ApplicationException _ax )
{
String _id = _ax.getId();
try
{
_ax.getInputStream().close();
}
catch (java.io.IOException e)
{
throw new RuntimeException("Unexpected exception " + e.toString() );
}
throw new RuntimeException("Unexpected exception " + _id );
}
finally
{
if (_os != null)
{
try
{
_os.close();
}
catch (java.io.IOException e)
{
throw new RuntimeException("Unexpected exception " + e.toString() );
}
}
this._releaseReply(_is);
}
}
else
{
org.omg.CORBA.portable.ServantObject _so = _servant_preinvoke( "_get_adm_name", _opsClass);
if( _so == null )
continue;
DeviceOperations _localServant = (DeviceOperations)_so.servant;
java.lang.String _result;
try
{
_result = _localServant.adm_name();
if ( _so instanceof org.omg.CORBA.portable.ServantObjectExt)
((org.omg.CORBA.portable.ServantObjectExt)_so).normalCompletion();
return _result;
}
catch (RuntimeException re)
{
if ( _so instanceof org.omg.CORBA.portable.ServantObjectExt)
((org.omg.CORBA.portable.ServantObjectExt)_so).exceptionalCompletion(re);
throw re;
}
catch (java.lang.Error err)
{
if ( _so instanceof org.omg.CORBA.portable.ServantObjectExt)
((org.omg.CORBA.portable.ServantObjectExt)_so).exceptionalCompletion(err);
throw err;
}
finally
{
_servant_postinvoke(_so);
}
}
}
}
public fr.esrf.Tango.DevCmdInfo[] command_list_query() throws fr.esrf.Tango.DevFailed
{
while(true)
{
if(! this._is_local())
{
org.omg.CORBA.portable.InputStream _is = null;
org.omg.CORBA.portable.OutputStream _os = null;
try
{
_os = _request( "command_list_query", true);
_is = _invoke(_os);
fr.esrf.Tango.DevCmdInfo[] _result = fr.esrf.Tango.DevCmdInfoListHelper.read(_is);
return _result;
}
catch( org.omg.CORBA.portable.RemarshalException _rx )
{
continue;
}
catch( org.omg.CORBA.portable.ApplicationException _ax )
{
String _id = _ax.getId();
try
{
if( _id.equals("IDL:Tango/DevFailed:1.0"))
{
throw fr.esrf.Tango.DevFailedHelper.read(_ax.getInputStream());
}
else
{
throw new RuntimeException("Unexpected exception " + _id );
}
}
finally
{
try
{
_ax.getInputStream().close();
}
catch (java.io.IOException e)
{
throw new RuntimeException("Unexpected exception " + e.toString() );
}
}
}
finally
{
if (_os != null)
{
try
{
_os.close();
}
catch (java.io.IOException e)
{
throw new RuntimeException("Unexpected exception " + e.toString() );
}
}
this._releaseReply(_is);
}
}
else
{
org.omg.CORBA.portable.ServantObject _so = _servant_preinvoke( "command_list_query", _opsClass );
if( _so == null )
continue;
DeviceOperations _localServant = (DeviceOperations)_so.servant;
fr.esrf.Tango.DevCmdInfo[] _result;
try
{
_result = _localServant.command_list_query();
if ( _so instanceof org.omg.CORBA.portable.ServantObjectExt)
((org.omg.CORBA.portable.ServantObjectExt)_so).normalCompletion();
return _result;
}
catch (fr.esrf.Tango.DevFailed ex)
{
if ( _so instanceof org.omg.CORBA.portable.ServantObjectExt)
((org.omg.CORBA.portable.ServantObjectExt)_so).exceptionalCompletion(ex);
throw ex;
}
catch (RuntimeException re)
{
if ( _so instanceof org.omg.CORBA.portable.ServantObjectExt)
((org.omg.CORBA.portable.ServantObjectExt)_so).exceptionalCompletion(re);
throw re;
}
catch (java.lang.Error err)
{
if ( _so instanceof org.omg.CORBA.portable.ServantObjectExt)
((org.omg.CORBA.portable.ServantObjectExt)_so).exceptionalCompletion(err);
throw err;
}
finally
{
_servant_postinvoke(_so);
}
}
}
}
public fr.esrf.Tango.DevInfo info() throws fr.esrf.Tango.DevFailed
{
while(true)
{
if(! this._is_local())
{
org.omg.CORBA.portable.InputStream _is = null;
org.omg.CORBA.portable.OutputStream _os = null;
try
{
_os = _request( "info", true);
_is = _invoke(_os);
fr.esrf.Tango.DevInfo _result = fr.esrf.Tango.DevInfoHelper.read(_is);
return _result;
}
catch( org.omg.CORBA.portable.RemarshalException _rx )
{
continue;
}
catch( org.omg.CORBA.portable.ApplicationException _ax )
{
String _id = _ax.getId();
try
{
if( _id.equals("IDL:Tango/DevFailed:1.0"))
{
throw fr.esrf.Tango.DevFailedHelper.read(_ax.getInputStream());
}
else
{
throw new RuntimeException("Unexpected exception " + _id );
}
}
finally
{
try
{
_ax.getInputStream().close();
}
catch (java.io.IOException e)
{
throw new RuntimeException("Unexpected exception " + e.toString() );
}
}
}
finally
{
if (_os != null)
{
try
{
_os.close();
}
catch (java.io.IOException e)
{
throw new RuntimeException("Unexpected exception " + e.toString() );
}
}
this._releaseReply(_is);
}
}
else
{
org.omg.CORBA.portable.ServantObject _so = _servant_preinvoke( "info", _opsClass );
if( _so == null )
continue;
DeviceOperations _localServant = (DeviceOperations)_so.servant;
fr.esrf.Tango.DevInfo _result;
try
{
_result = _localServant.info();
if ( _so instanceof org.omg.CORBA.portable.ServantObjectExt)
((org.omg.CORBA.portable.ServantObjectExt)_so).normalCompletion();
return _result;
}
catch (fr.esrf.Tango.DevFailed ex)
{
if ( _so instanceof org.omg.CORBA.portable.ServantObjectExt)
((org.omg.CORBA.portable.ServantObjectExt)_so).exceptionalCompletion(ex);
throw ex;
}
catch (RuntimeException re)
{
if ( _so instanceof org.omg.CORBA.portable.ServantObjectExt)
((org.omg.CORBA.portable.ServantObjectExt)_so).exceptionalCompletion(re);
throw re;
}
catch (java.lang.Error err)
{
if ( _so instanceof org.omg.CORBA.portable.ServantObjectExt)
((org.omg.CORBA.portable.ServantObjectExt)_so).exceptionalCompletion(err);
throw err;
}
finally
{
_servant_postinvoke(_so);
}
}
}
}
public org.omg.CORBA.Any command_inout(java.lang.String command, org.omg.CORBA.Any argin) throws fr.esrf.Tango.DevFailed
{
while(true)
{
if(! this._is_local())
{
org.omg.CORBA.portable.InputStream _is = null;
org.omg.CORBA.portable.OutputStream _os = null;
try
{
_os = _request( "command_inout", true);
java.lang.String tmpResult95 = command;
_os.write_string( tmpResult95 );
_os.write_any(argin);
_is = _invoke(_os);
org.omg.CORBA.Any _result = _is.read_any();
return _result;
}
catch( org.omg.CORBA.portable.RemarshalException _rx )
{
continue;
}
catch( org.omg.CORBA.portable.ApplicationException _ax )
{
String _id = _ax.getId();
try
{
if( _id.equals("IDL:Tango/DevFailed:1.0"))
{
throw fr.esrf.Tango.DevFailedHelper.read(_ax.getInputStream());
}
else
{
throw new RuntimeException("Unexpected exception " + _id );
}
}
finally
{
try
{
_ax.getInputStream().close();
}
catch (java.io.IOException e)
{
throw new RuntimeException("Unexpected exception " + e.toString() );
}
}
}
finally
{
if (_os != null)
{
try
{
_os.close();
}
catch (java.io.IOException e)
{
throw new RuntimeException("Unexpected exception " + e.toString() );
}
}
this._releaseReply(_is);
}
}
else
{
org.omg.CORBA.portable.ServantObject _so = _servant_preinvoke( "command_inout", _opsClass );
if( _so == null )
continue;
DeviceOperations _localServant = (DeviceOperations)_so.servant;
org.omg.CORBA.Any _result;
try
{
_result = _localServant.command_inout(command,argin);
if ( _so instanceof org.omg.CORBA.portable.ServantObjectExt)
((org.omg.CORBA.portable.ServantObjectExt)_so).normalCompletion();
return _result;
}
catch (fr.esrf.Tango.DevFailed ex)
{
if ( _so instanceof org.omg.CORBA.portable.ServantObjectExt)
((org.omg.CORBA.portable.ServantObjectExt)_so).exceptionalCompletion(ex);
throw ex;
}
catch (RuntimeException re)
{
if ( _so instanceof org.omg.CORBA.portable.ServantObjectExt)
((org.omg.CORBA.portable.ServantObjectExt)_so).exceptionalCompletion(re);
throw re;
}
catch (java.lang.Error err)
{
if ( _so instanceof org.omg.CORBA.portable.ServantObjectExt)
((org.omg.CORBA.portable.ServantObjectExt)_so).exceptionalCompletion(err);
throw err;
}
finally
{
_servant_postinvoke(_so);
}
}
}
}
public fr.esrf.Tango.DevState state()
{
while(true)
{
if(! this._is_local())
{
org.omg.CORBA.portable.InputStream _is = null;
org.omg.CORBA.portable.OutputStream _os = null;
try
{
_os = _request("_get_state",true);
_is = _invoke(_os);
return fr.esrf.Tango.DevStateHelper.read(_is);
}
catch( org.omg.CORBA.portable.RemarshalException _rx )
{
continue;
}
catch( org.omg.CORBA.portable.ApplicationException _ax )
{
String _id = _ax.getId();
try
{
_ax.getInputStream().close();
}
catch (java.io.IOException e)
{
throw new RuntimeException("Unexpected exception " + e.toString() );
}
throw new RuntimeException("Unexpected exception " + _id );
}
finally
{
if (_os != null)
{
try
{
_os.close();
}
catch (java.io.IOException e)
{
throw new RuntimeException("Unexpected exception " + e.toString() );
}
}
this._releaseReply(_is);
}
}
else
{
org.omg.CORBA.portable.ServantObject _so = _servant_preinvoke( "_get_state", _opsClass);
if( _so == null )
continue;
DeviceOperations _localServant = (DeviceOperations)_so.servant;
fr.esrf.Tango.DevState _result;
try
{
_result = _localServant.state();
if ( _so instanceof org.omg.CORBA.portable.ServantObjectExt)
((org.omg.CORBA.portable.ServantObjectExt)_so).normalCompletion();
return _result;
}
catch (RuntimeException re)
{
if ( _so instanceof org.omg.CORBA.portable.ServantObjectExt)
((org.omg.CORBA.portable.ServantObjectExt)_so).exceptionalCompletion(re);
throw re;
}
catch (java.lang.Error err)
{
if ( _so instanceof org.omg.CORBA.portable.ServantObjectExt)
((org.omg.CORBA.portable.ServantObjectExt)_so).exceptionalCompletion(err);
throw err;
}
finally
{
_servant_postinvoke(_so);
}
}
}
}
public java.lang.String status()
{
while(true)
{
if(! this._is_local())
{
org.omg.CORBA.portable.InputStream _is = null;
org.omg.CORBA.portable.OutputStream _os = null;
try
{
_os = _request("_get_status",true);
_is = _invoke(_os);
return _is.read_string();
}
catch( org.omg.CORBA.portable.RemarshalException _rx )
{
continue;
}
catch( org.omg.CORBA.portable.ApplicationException _ax )
{
String _id = _ax.getId();
try
{
_ax.getInputStream().close();
}
catch (java.io.IOException e)
{
throw new RuntimeException("Unexpected exception " + e.toString() );
}
throw new RuntimeException("Unexpected exception " + _id );
}
finally
{
if (_os != null)
{
try
{
_os.close();
}
catch (java.io.IOException e)
{
throw new RuntimeException("Unexpected exception " + e.toString() );
}
}
this._releaseReply(_is);
}
}
else
{
org.omg.CORBA.portable.ServantObject _so = _servant_preinvoke( "_get_status", _opsClass);
if( _so == null )
continue;
DeviceOperations _localServant = (DeviceOperations)_so.servant;
java.lang.String _result;
try
{
_result = _localServant.status();
if ( _so instanceof org.omg.CORBA.portable.ServantObjectExt)
((org.omg.CORBA.portable.ServantObjectExt)_so).normalCompletion();
return _result;
}
catch (RuntimeException re)
{
if ( _so instanceof org.omg.CORBA.portable.ServantObjectExt)
((org.omg.CORBA.portable.ServantObjectExt)_so).exceptionalCompletion(re);
throw re;
}
catch (java.lang.Error err)
{
if ( _so instanceof org.omg.CORBA.portable.ServantObjectExt)
((org.omg.CORBA.portable.ServantObjectExt)_so).exceptionalCompletion(err);
throw err;
}
finally
{
_servant_postinvoke(_so);
}
}
}
}
public void set_attribute_config(fr.esrf.Tango.AttributeConfig[] new_conf) throws fr.esrf.Tango.DevFailed
{
while(true)
{
if(! this._is_local())
{
org.omg.CORBA.portable.InputStream _is = null;
org.omg.CORBA.portable.OutputStream _os = null;
try
{
_os = _request( "set_attribute_config", true);
fr.esrf.Tango.AttributeConfigListHelper.write(_os,new_conf);
_is = _invoke(_os);
return;
}
catch( org.omg.CORBA.portable.RemarshalException _rx )
{
continue;
}
catch( org.omg.CORBA.portable.ApplicationException _ax )
{
String _id = _ax.getId();
try
{
if( _id.equals("IDL:Tango/DevFailed:1.0"))
{
throw fr.esrf.Tango.DevFailedHelper.read(_ax.getInputStream());
}
else
{
throw new RuntimeException("Unexpected exception " + _id );
}
}
finally
{
try
{
_ax.getInputStream().close();
}
catch (java.io.IOException e)
{
throw new RuntimeException("Unexpected exception " + e.toString() );
}
}
}
finally
{
if (_os != null)
{
try
{
_os.close();
}
catch (java.io.IOException e)
{
throw new RuntimeException("Unexpected exception " + e.toString() );
}
}
this._releaseReply(_is);
}
}
else
{
org.omg.CORBA.portable.ServantObject _so = _servant_preinvoke( "set_attribute_config", _opsClass );
if( _so == null )
continue;
DeviceOperations _localServant = (DeviceOperations)_so.servant;
try
{
_localServant.set_attribute_config(new_conf);
if ( _so instanceof org.omg.CORBA.portable.ServantObjectExt)
((org.omg.CORBA.portable.ServantObjectExt)_so).normalCompletion();
return;
}
catch (fr.esrf.Tango.DevFailed ex)
{
if ( _so instanceof org.omg.CORBA.portable.ServantObjectExt)
((org.omg.CORBA.portable.ServantObjectExt)_so).exceptionalCompletion(ex);
throw ex;
}
catch (RuntimeException re)
{
if ( _so instanceof org.omg.CORBA.portable.ServantObjectExt)
((org.omg.CORBA.portable.ServantObjectExt)_so).exceptionalCompletion(re);
throw re;
}
catch (java.lang.Error err)
{
if ( _so instanceof org.omg.CORBA.portable.ServantObjectExt)
((org.omg.CORBA.portable.ServantObjectExt)_so).exceptionalCompletion(err);
throw err;
}
finally
{
_servant_postinvoke(_so);
}
}
}
}
public java.lang.String[] black_box(int n) throws fr.esrf.Tango.DevFailed
{
while(true)
{
if(! this._is_local())
{
org.omg.CORBA.portable.InputStream _is = null;
org.omg.CORBA.portable.OutputStream _os = null;
try
{
_os = _request( "black_box", true);
_os.write_long(n);
_is = _invoke(_os);
java.lang.String[] _result = fr.esrf.Tango.DevVarStringArrayHelper.read(_is);
return _result;
}
catch( org.omg.CORBA.portable.RemarshalException _rx )
{
continue;
}
catch( org.omg.CORBA.portable.ApplicationException _ax )
{
String _id = _ax.getId();
try
{
if( _id.equals("IDL:Tango/DevFailed:1.0"))
{
throw fr.esrf.Tango.DevFailedHelper.read(_ax.getInputStream());
}
else
{
throw new RuntimeException("Unexpected exception " + _id );
}
}
finally
{
try
{
_ax.getInputStream().close();
}
catch (java.io.IOException e)
{
throw new RuntimeException("Unexpected exception " + e.toString() );
}
}
}
finally
{
if (_os != null)
{
try
{
_os.close();
}
catch (java.io.IOException e)
{
throw new RuntimeException("Unexpected exception " + e.toString() );
}
}
this._releaseReply(_is);
}
}
else
{
org.omg.CORBA.portable.ServantObject _so = _servant_preinvoke( "black_box", _opsClass );
if( _so == null )
continue;
DeviceOperations _localServant = (DeviceOperations)_so.servant;
java.lang.String[] _result;
try
{
_result = _localServant.black_box(n);
if ( _so instanceof org.omg.CORBA.portable.ServantObjectExt)
((org.omg.CORBA.portable.ServantObjectExt)_so).normalCompletion();
return _result;
}
catch (fr.esrf.Tango.DevFailed ex)
{
if ( _so instanceof org.omg.CORBA.portable.ServantObjectExt)
((org.omg.CORBA.portable.ServantObjectExt)_so).exceptionalCompletion(ex);
throw ex;
}
catch (RuntimeException re)
{
if ( _so instanceof org.omg.CORBA.portable.ServantObjectExt)
((org.omg.CORBA.portable.ServantObjectExt)_so).exceptionalCompletion(re);
throw re;
}
catch (java.lang.Error err)
{
if ( _so instanceof org.omg.CORBA.portable.ServantObjectExt)
((org.omg.CORBA.portable.ServantObjectExt)_so).exceptionalCompletion(err);
throw err;
}
finally
{
_servant_postinvoke(_so);
}
}
}
}
public fr.esrf.Tango.AttributeConfig[] get_attribute_config(java.lang.String[] names) throws fr.esrf.Tango.DevFailed
{
while(true)
{
if(! this._is_local())
{
org.omg.CORBA.portable.InputStream _is = null;
org.omg.CORBA.portable.OutputStream _os = null;
try
{
_os = _request( "get_attribute_config", true);
fr.esrf.Tango.DevVarStringArrayHelper.write(_os,names);
_is = _invoke(_os);
fr.esrf.Tango.AttributeConfig[] _result = fr.esrf.Tango.AttributeConfigListHelper.read(_is);
return _result;
}
catch( org.omg.CORBA.portable.RemarshalException _rx )
{
continue;
}
catch( org.omg.CORBA.portable.ApplicationException _ax )
{
String _id = _ax.getId();
try
{
if( _id.equals("IDL:Tango/DevFailed:1.0"))
{
throw fr.esrf.Tango.DevFailedHelper.read(_ax.getInputStream());
}
else
{
throw new RuntimeException("Unexpected exception " + _id );
}
}
finally
{
try
{
_ax.getInputStream().close();
}
catch (java.io.IOException e)
{
throw new RuntimeException("Unexpected exception " + e.toString() );
}
}
}
finally
{
if (_os != null)
{
try
{
_os.close();
}
catch (java.io.IOException e)
{
throw new RuntimeException("Unexpected exception " + e.toString() );
}
}
this._releaseReply(_is);
}
}
else
{
org.omg.CORBA.portable.ServantObject _so = _servant_preinvoke( "get_attribute_config", _opsClass );
if( _so == null )
continue;
DeviceOperations _localServant = (DeviceOperations)_so.servant;
fr.esrf.Tango.AttributeConfig[] _result;
try
{
_result = _localServant.get_attribute_config(names);
if ( _so instanceof org.omg.CORBA.portable.ServantObjectExt)
((org.omg.CORBA.portable.ServantObjectExt)_so).normalCompletion();
return _result;
}
catch (fr.esrf.Tango.DevFailed ex)
{
if ( _so instanceof org.omg.CORBA.portable.ServantObjectExt)
((org.omg.CORBA.portable.ServantObjectExt)_so).exceptionalCompletion(ex);
throw ex;
}
catch (RuntimeException re)
{
if ( _so instanceof org.omg.CORBA.portable.ServantObjectExt)
((org.omg.CORBA.portable.ServantObjectExt)_so).exceptionalCompletion(re);
throw re;
}
catch (java.lang.Error err)
{
if ( _so instanceof org.omg.CORBA.portable.ServantObjectExt)
((org.omg.CORBA.portable.ServantObjectExt)_so).exceptionalCompletion(err);
throw err;
}
finally
{
_servant_postinvoke(_so);
}
}
}
}
public fr.esrf.Tango.DevCmdInfo command_query(java.lang.String command) throws fr.esrf.Tango.DevFailed
{
while(true)
{
if(! this._is_local())
{
org.omg.CORBA.portable.InputStream _is = null;
org.omg.CORBA.portable.OutputStream _os = null;
try
{
_os = _request( "command_query", true);
java.lang.String tmpResult96 = command;
_os.write_string( tmpResult96 );
_is = _invoke(_os);
fr.esrf.Tango.DevCmdInfo _result = fr.esrf.Tango.DevCmdInfoHelper.read(_is);
return _result;
}
catch( org.omg.CORBA.portable.RemarshalException _rx )
{
continue;
}
catch( org.omg.CORBA.portable.ApplicationException _ax )
{
String _id = _ax.getId();
try
{
if( _id.equals("IDL:Tango/DevFailed:1.0"))
{
throw fr.esrf.Tango.DevFailedHelper.read(_ax.getInputStream());
}
else
{
throw new RuntimeException("Unexpected exception " + _id );
}
}
finally
{
try
{
_ax.getInputStream().close();
}
catch (java.io.IOException e)
{
throw new RuntimeException("Unexpected exception " + e.toString() );
}
}
}
finally
{
if (_os != null)
{
try
{
_os.close();
}
catch (java.io.IOException e)
{
throw new RuntimeException("Unexpected exception " + e.toString() );
}
}
this._releaseReply(_is);
}
}
else
{
org.omg.CORBA.portable.ServantObject _so = _servant_preinvoke( "command_query", _opsClass );
if( _so == null )
continue;
DeviceOperations _localServant = (DeviceOperations)_so.servant;
fr.esrf.Tango.DevCmdInfo _result;
try
{
_result = _localServant.command_query(command);
if ( _so instanceof org.omg.CORBA.portable.ServantObjectExt)
((org.omg.CORBA.portable.ServantObjectExt)_so).normalCompletion();
return _result;
}
catch (fr.esrf.Tango.DevFailed ex)
{
if ( _so instanceof org.omg.CORBA.portable.ServantObjectExt)
((org.omg.CORBA.portable.ServantObjectExt)_so).exceptionalCompletion(ex);
throw ex;
}
catch (RuntimeException re)
{
if ( _so instanceof org.omg.CORBA.portable.ServantObjectExt)
((org.omg.CORBA.portable.ServantObjectExt)_so).exceptionalCompletion(re);
throw re;
}
catch (java.lang.Error err)
{
if ( _so instanceof org.omg.CORBA.portable.ServantObjectExt)
((org.omg.CORBA.portable.ServantObjectExt)_so).exceptionalCompletion(err);
throw err;
}
finally
{
_servant_postinvoke(_so);
}
}
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy