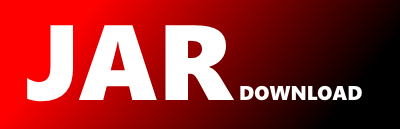
jive.ArgParser Maven / Gradle / Ivy
The newest version!
package jive;
import fr.esrf.Tango.DevState;
import fr.esrf.TangoApi.PipeBlob;
import fr.esrf.TangoApi.PipeDataElement;
import fr.esrf.TangoDs.TangoConst;
import java.io.*;
import java.util.Vector;
import java.util.Properties;
/** A class for parsing input arguments of tango command and attribute. */
public class ArgParser {
/**
* Construct an parser for the given string.
* @param s String to be parsed.
*/
public ArgParser(String s) throws NumberFormatException {
if(s==null || s.length()==0)
throw new NumberFormatException("Empty argument, you must specify a value");
if(s.startsWith("file:/")) {
// We have a file
try {
theStream = new FileReader(s.substring(6));
} catch( IOException e) {
throw new NumberFormatException(e.getMessage());
}
} else {
theStream = new StringReader(s);
}
width=0;
height=0;
// Done 3 times to initialise nextchars and currentChar
read_char();
read_char();
read_char();
}
public String convert_to_hex() throws NumberFormatException {
StringBuffer ret_string = new StringBuffer();
String word = "";
while(word!=null) {
word = get_space();
ret_string.append(word);
word =read_word();
if(word!=null) {
if(is_integer(word)) {
long l = get_long64(word);
ret_string.append("0x"+String.format("%X",l));
} else {
ret_string.append(word);
}
}
}
return ret_string.toString();
}
public String convert_to_dec() throws NumberFormatException {
StringBuffer ret_string = new StringBuffer();
String word = "";
while(word!=null) {
word = get_space();
ret_string.append(word);
word =read_word();
if(word!=null) {
if(is_integer(word)) {
long l = get_long64(word);
ret_string.append(String.format("%d",l));
} else {
ret_string.append(word);
}
}
}
return ret_string.toString();
}
public PipeBlob parse_pipe() throws NumberFormatException {
String blobName = read_word();
jump_space();
PipeBlob pb = new PipeBlob(blobName);
do {
jump_sep(",");
jump_space();
jump_sep("[");
jump_space();
String itemName = read_word();
jump_space();
jump_sep(",");
jump_space();
PipeDataElement de;
if (currentChar == '[') {
// An inner blob
jump_sep("[");
jump_space();
PipeBlob ipb = parse_pipe();
jump_space();
jump_sep("]");
de = new PipeDataElement(itemName, ipb);
} else {
Vector tmp = parse_array();
int vSize = tmp.size();
if (vSize < 2) {
throw new NumberFormatException("Typed value expected: typeStr,value");
}
// Get type STR
String typeStr = (String) tmp.get(0);
tmp.remove(0);
vSize -= 1;
if (typeStr.equalsIgnoreCase("D")) {
// Double array
double[] da = new double[vSize];
for (int i = 0; i < vSize; i++) da[i] = Double.parseDouble((String) tmp.get(i));
de = new PipeDataElement(itemName, da);
} else if (typeStr.equalsIgnoreCase("F")) {
// Float array
float[] fa = new float[vSize];
for (int i = 0; i < vSize; i++) fa[i] = Float.parseFloat((String) tmp.get(i));
de = new PipeDataElement(itemName, fa);
} else if (typeStr.equalsIgnoreCase("UC")) {
// UChar array
short[] ca = new short[vSize];
for (int i = 0; i < vSize; i++) ca[i] = get_uchar((String) tmp.get(i));
de = new PipeDataElement(itemName, ca, true);
} else if (typeStr.equalsIgnoreCase("S")) {
// Short array
short[] sa = new short[vSize];
for (int i = 0; i < vSize; i++) sa[i] = get_short((String) tmp.get(i));
de = new PipeDataElement(itemName, sa);
} else if (typeStr.equalsIgnoreCase("US")) {
// UShort array
int[] usa = new int[vSize];
for (int i = 0; i < vSize; i++) usa[i] = get_ushort((String) tmp.get(i));
de = new PipeDataElement(itemName, usa, true);
} else if (typeStr.equalsIgnoreCase("L")) {
// Long array
int[] la = new int[vSize];
for (int i = 0; i < vSize; i++) la[i] = get_long((String) tmp.get(i));
de = new PipeDataElement(itemName, la);
} else if (typeStr.equalsIgnoreCase("UL")) {
// ULong array
long[] ula = new long[vSize];
for (int i = 0; i < vSize; i++) ula[i] = get_long((String) tmp.get(i));
de = new PipeDataElement(itemName, ula, true);
} else if (typeStr.equalsIgnoreCase("LL")) {
// Long array
long[] lla = new long[vSize];
for (int i = 0; i < vSize; i++) lla[i] = get_long64((String) tmp.get(i));
de = new PipeDataElement(itemName, lla);
} else if (typeStr.equalsIgnoreCase("ST")) {
// State array
DevState[] sa = new DevState[vSize];
for (int i = 0; i < vSize; i++) sa[i] = get_state((String) tmp.get(i));
de = new PipeDataElement(itemName, sa);
} else if (typeStr.equalsIgnoreCase("STR")) {
// Default as string array
String[] ss = new String[vSize];
for (int i = 0; i < vSize; i++) ss[i] = (String) tmp.get(i);
de = new PipeDataElement(itemName, ss);
} else {
throw new NumberFormatException("Unknown type specified " + typeStr);
}
}
pb.add(de);
jump_space();
jump_sep("]");
jump_space();
} while (currentChar == ',');
return pb;
}
/**
* Parse a boolean value.
* @return The boolean.
* @throws NumberFormatException In case of failure
*/
public boolean parse_boolean() throws NumberFormatException {
String w = read_word();
if(w!=null) {
return get_boolean(w);
} else {
throw new NumberFormatException("boolean value expected.");
}
}
/**
* Parse a boolean array.
* @return The boolean array.
* @throws NumberFormatException In case of failure
*/
public boolean[] parse_boolean_array() throws NumberFormatException {
Vector tmp = parse_array();
boolean[] ret = new boolean[tmp.size()];
for(int l=0;l 0) read_char();
}
private String get_space() {
StringBuffer ret_word = new StringBuffer();
while (currentChar <= 32 && currentChar > 0) {
ret_word.append(currentChar);
read_char();
}
return ret_word.toString();
}
private boolean is_special_char(char c) {
return c == ',' || c == '[' || c == ']' || c == '(' || c == ')';
}
// ****************************************************
private String read_word() throws NumberFormatException {
StringBuffer ret_word = new StringBuffer();
/* Jump space and comments */
jump_space();
/* Treat special character */
if (is_special_char(currentChar)) {
ret_word.append(currentChar);
read_char();
return ret_word.toString();
}
/* Treat char */
if(currentChar=='\'' && nextNextChar=='\'') {
ret_word.append(currentChar);
read_char();
ret_word.append(currentChar);
read_char();
ret_word.append(currentChar);
read_char();
return ret_word.toString();
}
/* Treat string */
if (currentChar=='"' && !backSlashed) {
read_char();
while ((currentChar != '"' || backSlashed) && currentChar != 0 && currentChar != '\n') {
ret_word.append(currentChar);
read_char();
}
if (currentChar == 0 || currentChar == '\n') {
NumberFormatException e = new NumberFormatException("Unterminated string.");
throw e;
}
read_char();
return ret_word.toString();
}
/* Treat other word */
while (currentChar > 32 && !is_special_char(currentChar)) {
ret_word.append(currentChar);
read_char();
}
if (ret_word.length() == 0) {
return null;
}
return ret_word.toString();
}
// ****************************************************
private void jump_sep(String sep) throws NumberFormatException {
String w = read_word();
if(w==null)
throw new NumberFormatException("Separator " + sep + " expected.");
if(!w.equals(sep))
throw new NumberFormatException("Separator " + sep + " expected.");
}
// ****************************************************
private boolean is_array_end() {
if(currentChar==0) return true;
if(currentChar==']') return true;
return false;
}
// ****************************************************
private Vector parse_array() throws NumberFormatException {
Vector ret = new Vector();
boolean isClosed=false;
jump_space();
if(currentChar=='[') {
isClosed = true;
jump_sep("[");
jump_space();
}
while(!is_array_end()) {
ret.add(read_word());
jump_space();
if(!is_array_end()) {
jump_sep(",");
jump_space();
}
}
if(isClosed) jump_sep("]");
return ret;
}
// ****************************************************
private Vector parse_image() throws NumberFormatException {
// Read the fist line
Vector ret = parse_array();
jump_space();
width = ret.size();
height = 1;
while(currentChar=='[') {
Vector tmp = parse_array();
if(tmp.size()!=width)
throw new NumberFormatException("All lines in an image must have the same size.");
ret.addAll(tmp);
height++;
jump_space();
}
return ret;
}
// ****************************************************
private boolean get_boolean(String w) throws NumberFormatException {
if(w.equalsIgnoreCase("true") || w.equalsIgnoreCase("1"))
return true;
if(w.equalsIgnoreCase("false") || w.equalsIgnoreCase("0"))
return false;
throw new NumberFormatException("invalid boolean value "+w+" [true,false or 0,1].");
}
// ****************************************************
private long get_number(String w,String type,long min,long max) throws NumberFormatException {
int conv_base=10;
if (w.startsWith("0x") || w.startsWith("0X")) {
w = w.substring(2);
conv_base=16;
}
long ret = Long.parseLong(w,conv_base);
if(retmax)
throw new NumberFormatException(type+" value "+ret+" out of range ["+min+","+max+"].");
return ret;
}
// ****************************************************
private byte get_char(String w) throws NumberFormatException {
if(w.startsWith("'")) {
if(w.endsWith("'") && w.length()==3) {
return (byte)w.charAt(1);
} else {
throw new NumberFormatException("Invalid char value for input string " + w);
}
} else {
return (byte)get_number(w,"char",-128L,127L);
}
}
// ****************************************************
private short get_uchar(String w) throws NumberFormatException {
return (short)get_number(w,"unsigned char",0L,255L);
}
// ****************************************************
private short get_short(String w) throws NumberFormatException {
return (short)get_number(w,"short",-32768L,32767L);
}
// ****************************************************
private int get_ushort(String w) throws NumberFormatException {
return (int)get_number(w,"unsigned short",0L,65535L);
}
// ****************************************************
private int get_long(String w) throws NumberFormatException {
return (int)get_number(w,"long",-2147483648L,2147483647L);
}
// ****************************************************
private long get_ulong(String w) throws NumberFormatException {
return get_number(w,"unsigned long",0,4294967295L);
}
// ****************************************************
private long get_long64(String w) throws NumberFormatException {
return get_number(w,"long64",-9223372036854775808L,9223372036854775807L);
}
private boolean is_native_number(String s) {
int length = s.length();
int i=0;
boolean ret = true;
while( ret && i='0' && c<='9');
i++;
}
return ret;
}
private boolean is_native_hex_number(String s) {
int length = s.length();
int i=0;
boolean ret = true;
while( ret && i='0' && c<='9') || (c>='a' && c<='f') || (c>='A' && c<='F');
i++;
}
return ret;
}
private boolean is_integer(String s) {
if(s.contains("."))
return false;
if (s.startsWith("0x") || s.startsWith("0X")) {
return is_native_hex_number(s.substring(2));
}
if(s.startsWith("-"))
return is_native_number(s.substring(1));
return is_native_number(s);
}
private DevState get_state(String s) throws NumberFormatException {
boolean found = false;
int i=0;
while(!found && i< TangoConst.Tango_DevStateName.length) {
found = s.equalsIgnoreCase(TangoConst.Tango_DevStateName[i]);
if(!found) i++;
}
if( found ) {
return DevState.from_int(i);
} else {
throw new NumberFormatException("Invalid state name " + s);
}
}
private boolean is_state_array(Vector v) {
try {
for(int i=0;i
© 2015 - 2025 Weber Informatics LLC | Privacy Policy