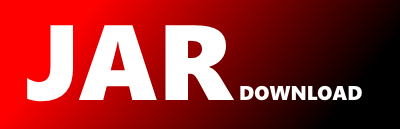
jive3.MainPanel Maven / Gradle / Ivy
The newest version!
package jive3;
import jive.*;
import javax.swing.*;
import javax.swing.event.ChangeListener;
import javax.swing.event.ChangeEvent;
import javax.swing.tree.TreePath;
import java.awt.*;
import java.awt.event.*;
import java.io.File;
import java.util.ArrayList;
import java.util.Vector;
import java.util.prefs.Preferences;
import fr.esrf.TangoApi.ApiUtil;
import fr.esrf.TangoApi.Database;
import fr.esrf.Tango.DevFailed;
import fr.esrf.tangoatk.widget.util.ATKConstant;
import fr.esrf.tangoatk.widget.util.ATKGraphicsUtils;
public class MainPanel extends JFrame implements ChangeListener,NavigationListener,IServerAction {
private Database db;
JTabbedPane treePane;
JSplitPane splitPane;
JPanel lockPanel;
int panelMask;
// Trees
class TreePanelRecord {
String name;
String tabName;
TreePanel treePanel;
boolean display;
TreePanelRecord(TreePanel t,Database db,String tabName,String name,String treeName) {
t.setDatabase(db);
this.treePanel = t;
this.tabName = tabName;
this.name = name;
if(this.treePanel.tree!=null)
this.treePanel.tree.setName(treeName);
this.display = true;
}
}
ArrayList treePanels;
int nbPanels;
// Right panels
DefaultPanel defaultPanel;
PropertyPanel propertyPanel;
DevicePollingPanel devicePollingPanel;
DeviceEventPanel deviceEventPanel;
DeviceAttributePanel deviceAttributePanel;
DevicePipePanel devicePipePanel;
DeviceLoggingPanel deviceLoggingPanel;
SingleAttributePanel singleAttributePanel;
// History panel
PropertyHistoryDlg historyDlg;
// Multiple property selection panel
MultiplePropertySelectionDlg propertySelectionDlg;
// Multiple device selection panel
MultipleTestDeviceDlg deviceSelectionDlg;
// Filter dialog
FilterDlg filterDlg=null;
// Navigation stuff
private NavManager navManager;
private NavigationBar navBar;
private boolean recordPos;
//Search stuff
SearchEngine searchEngine;
// Inner panel
JPanel innerPanel;
private String lastResOpenedDir = ".";
private boolean running_from_shell;
// User settings
Preferences prefs;
private String[] knownTangoHost;
private String THID = "TangoHost";
// Relase number
public static final String DEFAULT_VERSION = "-.-";
public static final String VERSION = getVersion();
// General constructor
public MainPanel() {
this(false,false);
}
/**
* Construct a Jive application.
* @param runningFromShell True if running from shell. If true , Jive calls System.exit().
* @param readOnly Read only flag.
*/
public MainPanel(boolean runningFromShell,boolean readOnly) {
this(runningFromShell, readOnly, 126, null);
}
public MainPanel(boolean runningFromShell,boolean readOnly,int panelMask) {
this(runningFromShell, readOnly, panelMask, null);
}
public MainPanel(boolean runningFromShell,boolean readOnly,int panelMask,String tangoHost) {
this.panelMask = panelMask;
// Get user settings
prefs = Preferences.userRoot().node(this.getClass().getName());
knownTangoHost = JiveUtils.makeStringArray(prefs.get(THID,""));
if(knownTangoHost.length==1)
if(knownTangoHost[0].equals(""))
knownTangoHost = new String[0];
running_from_shell = runningFromShell;
JiveUtils.readOnly = readOnly;
initComponents(tangoHost);
centerWindow();
setVisible(true);
JiveUtils.parent = this;
navManager = new NavManager(this);
searchEngine = new SearchEngine(this);
recordPos = true;
}
// Init componenet
private void initComponents(String tangoHost) {
innerPanel = new JPanel();
innerPanel.setLayout(new BorderLayout());
MultiLineToolTipUI.initialize();
// *************************************************************
// Initialise the Tango database
// *************************************************************
if( tangoHost==null ) {
try {
tangoHost = ApiUtil.getTangoHost();
} catch (DevFailed e) {
System.out.println("TANGO_HOST no defined, exiting...");
exitForm();
}
try {
db = ApiUtil.get_db_obj();
} catch (DevFailed e) {
JiveUtils.showTangoError(e);
db = null;
}
} else {
try {
String[] tgh_arr = ApiUtil.parseTangoHost(tangoHost);
if (tgh_arr.length != 2) {
JiveUtils.showJiveError( "Input syntax error \n" + tangoHost + "\n is not a valid TANGO_HOST");
db = null;
} else {
db = ApiUtil.get_db_obj(tgh_arr[0], tgh_arr[1]);
}
} catch (DevFailed e) {
JiveUtils.showTangoError(e);
db = null;
}
}
updateTitle(tangoHost);
// *************************************************************
// Create widget
// *************************************************************
defaultPanel = new DefaultPanel();
propertyPanel = new PropertyPanel();
propertyPanel.setParent(this);
devicePollingPanel = new DevicePollingPanel();
deviceEventPanel = new DeviceEventPanel();
deviceAttributePanel = new DeviceAttributePanel();
devicePipePanel = new DevicePipePanel();
deviceLoggingPanel = new DeviceLoggingPanel();
singleAttributePanel = new SingleAttributePanel();
splitPane = new JSplitPane();
splitPane.setOrientation(JSplitPane.HORIZONTAL_SPLIT);
treePanels = new ArrayList();
TreePanel.invoker = this;
if(isCollectionPanelVisible())
treePanels.add( new TreePanelRecord(new TreePanelHostCollection(),db,"Collection","Collection:","COLLECTION") );
if(isServerPanelVisible())
treePanels.add( new TreePanelRecord(new TreePanelServer(),db,"Server","Server:","SERVER") );
if(isDevicePanelVisible())
treePanels.add( new TreePanelRecord(new TreePanelDevice(),db,"Device","Device:","DEVICE") );
if(isClassPanelVisible())
treePanels.add( new TreePanelRecord(new TreePanelClass(),db,"Class","Class:","CLASS") );
if(isDevAliasPanelVisible())
treePanels.add( new TreePanelRecord(new TreePanelAlias(),db,"Alias","Alias:","DEV-ALIAS") );
if(isAttAliasPanelVisible())
treePanels.add( new TreePanelRecord(new TreePanelAttributeAlias(),db,"Att. Alias","AttAlias:","ATT-ALIAS") );
if(isFreePropertyPanelVisible())
treePanels.add( new TreePanelRecord(new TreePanelFreeProperty(),db,"Property","FreeProperty:","PROPERTY") );
treePane = new JTabbedPane();
treePane.setFont(ATKConstant.labelFont);
treePane.addChangeListener(this);
splitPane.setLeftComponent(treePane);
nbPanels = treePanels.size();
for(int i=0;i0) {
String message = "Warning, following device(s) already declared:\n";
for(int i=0;i 0) JiveUtils.showJiveError(err);
if (f != null) lastResOpenedDir = f.getAbsolutePath();
}
}
}
// Load a property file in the database
private void checkPropFile() {
String err = "";
TangoFileReader fr = new TangoFileReader(db);
JFileChooser chooser = new JFileChooser(lastResOpenedDir);
JCheckBox ignoreSubDevices = new JCheckBox("Ignore __SubDevices");
ignoreSubDevices.setSelected(true);
chooser.setAccessory(ignoreSubDevices);
chooser.setMultiSelectionEnabled(true);
chooser.setApproveButtonText("Check");
int returnVal = chooser.showOpenDialog(this);
if (returnVal == JFileChooser.APPROVE_OPTION) {
File[] f = chooser.getSelectedFiles();
if( f!=null && f.length>0 ) {
Vector> diff = new Vector>();
lastResOpenedDir = f[0].getAbsolutePath();
for (File file : f) {
Vector fdiff = new Vector<>();
err = fr.check_res_file(file.getAbsolutePath(), fdiff, ignoreSubDevices.isSelected());
if (err.length() > 0)
fdiff.add(err);
else if(fdiff.size()==0)
fdiff.add("No difference");
diff.add(fdiff);
}
DiffDlg dlg = new DiffDlg(diff, f);
ATKGraphicsUtils.centerFrameOnScreen(dlg);
dlg.setVisible(true);
}
}
}
// Show database info
private void showDatabaseInfo() {
if(db==null) return;
try {
String result = db.get_info();
JOptionPane.showMessageDialog(this,result,"Database Info",JOptionPane.INFORMATION_MESSAGE);
} catch (DevFailed e) {
JiveUtils.showTangoError(e);
}
}
// Show Multiple property selection dialog
private void showMultiplePropertySelection() {
if(!propertySelectionDlg.isVisible())
ATKGraphicsUtils.centerFrameOnScreen(propertySelectionDlg);
propertySelectionDlg.clear();
propertySelectionDlg.setVisible(true);
}
// Show Multiple device selection dialog
private void showMultipleDeviceSelection() {
if(!deviceSelectionDlg.isVisible())
ATKGraphicsUtils.centerFrameOnScreen(deviceSelectionDlg);
deviceSelectionDlg.clear();
deviceSelectionDlg.setVisible(true);
}
// Display the history window
public void showHistory() {
if(!historyDlg.isVisible())
ATKGraphicsUtils.centerFrameOnScreen(historyDlg);
historyDlg.setVisible(true);
}
// Select a device and show the device tree panel
public void goToClassNode(String className) {
if(isClassPanelVisible()) {
TreePanelClass classTreePanel = getClassTreePanel();
classTreePanel.selectClass(className);
treePane.setSelectedComponent(classTreePanel);
// Work around X11 bug
treePane.getSelectedComponent().setVisible(true);
}
}
// Select a device and show the device tree panel
public void goToDeviceNode(String devName) {
if(isDevicePanelVisible()) {
TreePanelDevice deviceTreePanel = getDeviceTreePanel();
deviceTreePanel.selectDevice(devName);
treePane.setSelectedComponent(deviceTreePanel);
// Work around X11 bug
treePane.getSelectedComponent().setVisible(true);
}
}
private TangoNode goToDeviceFullNode(String devName) {
if (isDevicePanelVisible()) {
TreePanelDevice deviceTreePanel = getDeviceTreePanel();
TangoNode selected = deviceTreePanel.selectDevice(devName);
if (selected != null) {
treePane.setSelectedComponent(deviceTreePanel);
// Work around X11 bug
treePane.getSelectedComponent().setVisible(true);
}
return selected;
} else {
return null;
}
}
// Select a server and show the server tree panel
public void goToServerNode(String srvName) {
goToServerFullNode(srvName);
}
// Select a server and show the server tree panel
public TangoNode goToServerFullNode(String srvName) {
if (isServerPanelVisible()) {
TreePanelServer serverTreePanel = getServerTreePanel();
TangoNode selected = serverTreePanel.selectFullServer(srvName);
if (selected != null) {
treePane.setSelectedComponent(serverTreePanel);
// Work around X11 bug
treePane.getSelectedComponent().setVisible(true);
}
return selected;
} else {
return null;
}
}
// Select a server and show the server tree panel
public TangoNode goToServerRootNode(String srvName) {
if (isServerPanelVisible()) {
TreePanelServer serverTreePanel = getServerTreePanel();
TangoNode selected = serverTreePanel.selectServerRoot(srvName);
if (selected != null) {
treePane.setSelectedComponent(serverTreePanel);
// Work around X11 bug
treePane.getSelectedComponent().setVisible(true);
}
return selected;
} else {
return null;
}
}
// Tabbed pane listener
public void stateChanged(ChangeEvent e) {
updateTabbedPane();
}
private void updateTabbedPane() {
TreePanel p = (TreePanel)(treePane.getSelectedComponent());
p.refreshValues();
}
// TreeListener
void updatePanel(TangoNode[] source) {
int i;
// Check if there is some unsaved change
try {
Component panel = splitPane.getRightComponent();
if( panel instanceof PropertyPanel) {
PropertyPanel propertyPanel = (PropertyPanel)panel;
if( propertyPanel.hasChanged() ) {
if( JOptionPane.showConfirmDialog(this,"Some properties has been updated and not saved.\nWould you like to save change ?","Confirmation",JOptionPane.YES_NO_OPTION)==JOptionPane.YES_OPTION )
propertyPanel.saveChange();
}
}
if( panel instanceof SingleAttributePanel) {
SingleAttributePanel attPanel = (SingleAttributePanel)panel;
if( attPanel.hasChanged() ) {
if( JOptionPane.showConfirmDialog(this,"Some attribute properties has been updated and not saved.\nWould you like to save change ?","Confirmation",JOptionPane.YES_NO_OPTION)==JOptionPane.YES_OPTION )
attPanel.saveChange();
}
}
} catch(Exception e) {}
// No selection
if(source==null || source.length==0) {
defaultPanel.setSource(null,0);
splitPane.setRightComponent(defaultPanel);
return;
}
TreePanel p = (TreePanel)treePane.getSelectedComponent();
if(recordPos) navManager.recordPath(p.tree);
navBar.addLink(p.tree.getSelectionPath());
navBar.enableBack(navManager.canGoBackward());
navBar.enableForward(navManager.canGoForward());
// Check node class
boolean sameClass = true;
Class nodeClass = source[0].getClass();
i=1;
while(sameClass && i=args.length)
printUsage();
server = args[i];
} else if(args[i].equalsIgnoreCase("-d")) {
i++;
if(i>=args.length)
printUsage();
device = args[i];
} else if(args[i].equalsIgnoreCase("-c")) {
i++;
if(i>=args.length)
printUsage();
className = args[i];
} else if(args[i].equalsIgnoreCase("-fs")) {
i++;
if(i>=args.length)
printUsage();
fs = args[i];
} else if(args[i].equalsIgnoreCase("-fd")) {
i++;
if(i>=args.length)
printUsage();
fd = args[i];
} else if(args[i].equalsIgnoreCase("-fc")) {
i++;
if(i>=args.length)
printUsage();
fc = args[i];
} else if(args[i].equalsIgnoreCase("-fa")) {
i++;
if(i>=args.length)
printUsage();
fa = args[i];
} else if(args[i].equalsIgnoreCase("-faa")) {
i++;
if(i>=args.length)
printUsage();
faa = args[i];
} else if(args[i].equalsIgnoreCase("-fp")) {
i++;
if(i>=args.length)
printUsage();
fp = args[i];
} else if(args[i].equalsIgnoreCase("-p")) {
i++;
if(i>=args.length)
printUsage();
pmask = Integer.parseInt(args[i]);
} else {
System.out.println("Invalid option " + args[i]);
printUsage();
}
i++;
}
MainPanel p = new MainPanel(true,readOnly,pmask);
if(server!=null)
p.goToServerFullNode(server);
if(device!=null)
p.goToDeviceNode(device);
if(className!=null)
p.goToClassNode(className);
if(fs!=null)
p.filterServer(fs);
if(fd!=null)
p.filterDevice(fd);
if(fc!=null)
p.filterClass(fc);
if(fa!=null)
p.filterAlias(fa);
if(faa!=null)
p.filterAttributeAlias(faa);
if(fp!=null)
p.filterProperty(fp);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy