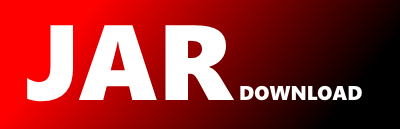
jive3.MultiplePropertySelectionDlg Maven / Gradle / Ivy
The newest version!
package jive3;
import fr.esrf.Tango.DevFailed;
import fr.esrf.Tango.DevVarLongStringArray;
import fr.esrf.TangoApi.Database;
import fr.esrf.TangoApi.DbAttribute;
import fr.esrf.TangoApi.DbDatum;
import fr.esrf.TangoApi.DeviceData;
import fr.esrf.tangoatk.widget.util.ATKConstant;
import jive.JiveUtils;
import jive.MultiLineCellEditor;
import jive.MultiLineCellRenderer;
import javax.swing.*;
import javax.swing.table.DefaultTableModel;
import java.awt.*;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import java.awt.event.WindowAdapter;
import java.awt.event.WindowEvent;
import java.io.File;
import java.io.FileWriter;
import java.io.IOException;
import java.util.ArrayList;
import java.util.Date;
/**
* Multiple selection dialog
*/
class PItem {
String devName;
String attName;
String pName;
boolean updated;
String[] value;
public String toString() {
return JiveUtils.stringArrayToString(value);
}
};
public class MultiplePropertySelectionDlg extends JFrame implements ActionListener {
Database db;
String colName[] = {"Name" , "Value"};
private MultiLineCellEditor editor;
JTextField selectionText;
JLabel selectionLabel;
JButton searchButton;
JButton saveAllButton;
JButton applyAllButton;
JButton applyButton;
JButton dismissButton;
JLabel infoLabel;
DefaultTableModel dm;
JTable theTable;
String selectText;
ArrayList items;
public MultiplePropertySelectionDlg() {
this.db = null;
initComponents();
}
public void setDatabase(Database db) {
this.db = db;
}
public void clear() {
items.clear();
infoLabel.setText(items.size() + " item(s)");
String[][] prop = new String[0][2];
dm.setDataVector(prop, colName);
editor.updateRows();
theTable.getColumnModel().getColumn(1).setPreferredWidth(250);
theTable.validate();
((JPanel)getContentPane()).revalidate();
}
void initComponents() {
getContentPane().setLayout(new BorderLayout());
JPanel innerPanel = new JPanel();
innerPanel.setLayout(new GridBagLayout());
GridBagConstraints gbc = new GridBagConstraints();
gbc.gridx = 0;
gbc.gridy = 0;
gbc.weightx = 0;
gbc.fill = GridBagConstraints.VERTICAL;
JLabel selectionLabel = new JLabel(" Selection ");
selectionLabel.setFont(ATKConstant.labelFont);
innerPanel.add(selectionLabel,gbc);
gbc.gridx = 1;
gbc.gridy = 0;
gbc.weightx = 1;
gbc.fill = GridBagConstraints.BOTH;
selectionText = new JTextField();
selectionText.addActionListener(this);
selectionText.setFont(ATKConstant.labelFont);
innerPanel.add(selectionText,gbc);
gbc.gridx = 2;
gbc.gridy = 0;
gbc.weightx = 0;
gbc.fill = GridBagConstraints.VERTICAL;
searchButton = new JButton("Search");
searchButton.addActionListener(this);
innerPanel.add(searchButton,gbc);
JPanel innerPanel2 = new JPanel();
innerPanel2.setLayout(new FlowLayout(FlowLayout.RIGHT));
infoLabel = new JLabel("0 item(s)");
infoLabel.setFont(ATKConstant.labelFont);
innerPanel2.add(infoLabel);
saveAllButton = new JButton("Save to file ...");
saveAllButton.setToolTipText("Save property selection in a resource file");
saveAllButton.addActionListener(this);
innerPanel2.add(saveAllButton);
applyAllButton = new JButton("Apply to all ...");
applyAllButton.setToolTipText("Ask for a value and apply it to the current selection");
applyAllButton.addActionListener(this);
innerPanel2.add(applyAllButton);
applyButton = new JButton("Apply");
applyButton.setToolTipText("Apply change to the database");
applyButton.addActionListener(this);
applyButton.setEnabled(false);
innerPanel2.add(applyButton);
dismissButton = new JButton("Dismiss");
dismissButton.addActionListener(this);
innerPanel2.add(dismissButton);
getContentPane().add(innerPanel, BorderLayout.NORTH);
getContentPane().add(innerPanel2, BorderLayout.SOUTH);
// Table model
dm = new DefaultTableModel() {
public Class getColumnClass(int columnIndex) {
return String.class;
}
public boolean isCellEditable(int row, int column) {
return column==1;
}
public void setValueAt(Object aValue, int row, int column) {
if(!aValue.equals(getValueAt(row,column))) {
super.setValueAt(aValue, row, column);
items.get(row).value = JiveUtils.makeStringArray((String)aValue);
items.get(row).updated = true;
applyButton.setEnabled(true);
}
}
};
theTable = new JTable(dm);
editor = new MultiLineCellEditor(theTable);
theTable.setDefaultEditor(String.class, editor);
MultiLineCellRenderer renderer = new MultiLineCellRenderer(false,false,false);
theTable.setDefaultRenderer(String.class, renderer);
theTable.setSelectionMode(ListSelectionModel.SINGLE_SELECTION);
JScrollPane textView = new JScrollPane(theTable);
getContentPane().add(textView, BorderLayout.CENTER);
setTitle("Multiple property selection");
setPreferredSize(new Dimension(800,600));
addWindowListener(new WindowAdapter() {
@Override
public void windowClosing(WindowEvent e) {
exitDlg();
super.windowClosing(e);
}
});
items = new ArrayList();
clear();
}
private void selectProperty() {
refresh();
}
private void refresh() {
String selectText = selectionText.getText().trim();
String[] fields = selectText.split("/");
if (fields.length != 4 && fields.length != 5) {
JiveUtils.showJiveError("Invalid selection pattern, 4 or 5 slash separated fields expected");
return;
}
try {
items.clear();
String devName = fields[0] + "/" + fields[1] + "/" + fields[2];
if(fields.length==4) {
// Device properties
String propName = fields[3];
String[] devNames = db.get_device_list(devName);
for( int i=0;i value = new ArrayList();
for(int i=0;i" + pi.pName + ": ", pi.value, resFile);
} else {
JiveUtils.printFormatedRes(pi.devName + "/" + pi.attName + "->" + pi.pName + ": ", pi.value, resFile);
}
}
resFile.close();
} catch (IOException e) {
JiveUtils.showJiveError("Failed to create resource file !\n" + e.getMessage());
}
}
}
private void apply() {
try {
for (int i = 0; i < items.size(); i++) {
PItem pi = items.get(i);
if (pi.updated) {
if( pi.attName==null ) {
// Device property
DbDatum[] ds = new DbDatum[1];
ds[0] = new DbDatum(pi.pName,pi.value);
db.put_device_property(pi.devName, ds);
} else {
// Attribute property
DbAttribute da = new DbAttribute(pi.attName);
da.add(pi.pName, pi.value);
db.put_device_attribute_property(pi.devName,da);
}
}
pi.updated = false;
}
} catch (DevFailed e) {
JiveUtils.showTangoError(e);
}
applyButton.setEnabled(false);
}
public boolean hasChanged() {
boolean hasChanged = false;
int i=0;
while(!hasChanged && i
© 2015 - 2025 Weber Informatics LLC | Privacy Policy