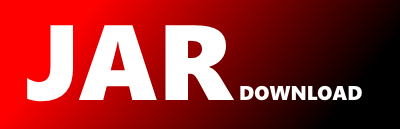
jive3.PropertyPanel Maven / Gradle / Ivy
The newest version!
package jive3;
import jive.ArgParser;
import jive.MultiLineCellEditor;
import jive.JiveUtils;
import jive.MultiLineCellRenderer;
import javax.swing.*;
import javax.swing.table.DefaultTableModel;
import javax.swing.border.Border;
import java.awt.*;
import java.awt.event.*;
import java.util.Vector;
public class PropertyPanel extends JPanel implements ActionListener,MouseListener {
private JScrollPane textView;
private JiveTable theTable;
private JButton refreshButton;
private JButton applyButton;
private JButton deleteButton;
private JButton copyButton;
private JButton newButton;
private MainPanel parent;
private PropertyEditorDlg propertyEditor = null;
private PropertyNode[] source = null;
private DefaultTableModel dm;
private boolean[] updatedProp;
private JPopupMenu tableMenu;
private JMenuItem historyMenuItem;
private JMenuItem renameMenuItem;
private JMenuItem deleteMenuItem;
private JMenuItem copyMenuItem;
private JMenuItem editMenuItem;
private JMenuItem toHexMenuItem;
private JMenuItem toDecMenuItem;
private JMenuItem descMenuItem;
PropertyPanel() {
setLayout(new BorderLayout());
// Table model
dm = new DefaultTableModel() {
public Class getColumnClass(int columnIndex) {
return String.class;
}
public boolean isCellEditable(int row, int column) {
return (column==1) && (!JiveUtils.readOnly);
}
public void setValueAt(Object aValue, int row, int column) {
if(!aValue.equals(getValueAt(row,column))) {
super.setValueAt(aValue,row,column);
applyButton.setEnabled(true);
updatedProp[row] = true;
}
}
};
// Table initialisation
theTable = new JiveTable(dm);
theTable.addMouseListener(this);
theTable.getEditor().getTextArea().addMouseListener(this);
textView = new JScrollPane(theTable);
add(textView, BorderLayout.CENTER);
Border b = BorderFactory.createTitledBorder(BorderFactory.createEtchedBorder(),"...");
textView.setBorder(b);
add(textView,BorderLayout.CENTER);
// Bottom panel
JPanel btnPanel = new JPanel(new FlowLayout(FlowLayout.LEFT));
refreshButton = new JButton("Refresh");
refreshButton.addActionListener(this);
applyButton = new JButton("Apply");
applyButton.addActionListener(this);
deleteButton = new JButton("Delete");
deleteButton.setEnabled(!JiveUtils.readOnly);
deleteButton.addActionListener(this);
copyButton = new JButton("Copy");
copyButton.setEnabled(!JiveUtils.readOnly);
copyButton.addActionListener(this);
newButton = new JButton("New property");
newButton.setEnabled(!JiveUtils.readOnly);
newButton.addActionListener(this);
btnPanel.add(refreshButton);
btnPanel.add(applyButton);
btnPanel.add(newButton);
btnPanel.add(copyButton);
btnPanel.add(deleteButton);
add(btnPanel,BorderLayout.SOUTH);
// Contextual menu
tableMenu = new JPopupMenu();
copyMenuItem = new JMenuItem("Copy");
copyMenuItem.addActionListener(this);
copyMenuItem.setAccelerator(KeyStroke.getKeyStroke(KeyEvent.VK_C, InputEvent.CTRL_DOWN_MASK));
theTable.addKeyPressedEvent(KeyEvent.VK_C, InputEvent.CTRL_DOWN_MASK, new KeyAdapter() {
public void keyPressed(KeyEvent e) {
copy();
}
});
renameMenuItem = new JMenuItem("Rename");
renameMenuItem.addActionListener(this);
renameMenuItem.setAccelerator(KeyStroke.getKeyStroke(KeyEvent.VK_F2, 0));
theTable.addKeyPressedEvent(KeyEvent.VK_F2, 0, new KeyAdapter() {
public void keyPressed(KeyEvent e) {
rename();
}
});
historyMenuItem = new JMenuItem("View history");
historyMenuItem.addActionListener(this);
deleteMenuItem = new JMenuItem("Delete");
deleteMenuItem.addActionListener(this);
deleteMenuItem.setAccelerator(KeyStroke.getKeyStroke(KeyEvent.VK_DELETE, 0));
theTable.addKeyPressedEvent(KeyEvent.VK_DELETE, 0, new KeyAdapter() {
public void keyPressed(KeyEvent e) {
delete();
}
});
editMenuItem = new JMenuItem("Edit");
editMenuItem.addActionListener(this);
toHexMenuItem = new JMenuItem("Convert to Hexa");
toHexMenuItem.addActionListener(this);
toDecMenuItem = new JMenuItem("Convert to Decimal");
toDecMenuItem.addActionListener(this);
descMenuItem = new JMenuItem("Show description");
descMenuItem.addActionListener(this);
tableMenu.add(copyMenuItem);
tableMenu.add(deleteMenuItem);
tableMenu.add(renameMenuItem);
tableMenu.add(editMenuItem);
tableMenu.add(historyMenuItem);
tableMenu.add(descMenuItem);
tableMenu.add(new JSeparator());
tableMenu.add(toHexMenuItem);
tableMenu.add(toDecMenuItem);
setMinimumSize(new Dimension(0,0));
}
// Mouse listener -------------------------------------------
public void mousePressed(MouseEvent e) {}
public void mouseClicked(MouseEvent e) {
Object src = e.getSource();
if(src==theTable && e.getButton() == MouseEvent.BUTTON3 && e.getClickCount()==1 && !JiveUtils.readOnly) {
int row = theTable.getRowForLocation(e.getY());
if(row!=-1) {
theTable.addRowSelectionInterval(row,row);
theTable.setColumnSelectionInterval(0,1);
int[] rows = theTable.getSelectedRows();
renameMenuItem.setEnabled(rows.length==1);
historyMenuItem.setEnabled(rows.length==1);
tableMenu.show(theTable, e.getX(), e.getY());
}
}
if(src==theTable.getEditor().getTextArea() && e.getButton() == MouseEvent.BUTTON3 && e.getClickCount()==1) {
String selText = theTable.getEditor().getTextArea().getSelectedText();
if( selText!=null ) {
// Basic device name check
int slashCount=0;
for(int i=0;i1) {
Vector propChange = new Vector();
for(int i=0;i1) ProgressFrame.displayProgress("Updating properties");
for(int i=0;i1) ProgressFrame.setProgress("Applying " + source[j].getName() + "/" + dm.getValueAt(i,0),
(k*100)/nb );
source[j].setProperty((String)dm.getValueAt(i,0),(String)dm.getValueAt(i,1));
}
updatedProp[i] = false;
}
}
applyButton.setEnabled(false);
ProgressFrame.hideProgress();
}
private String convertToHex(String s) {
ArgParser p = new ArgParser(s);
return p.convert_to_hex();
}
private String convertToDec(String s) {
ArgParser p = new ArgParser(s);
return p.convert_to_dec();
}
// Action listener -------------------------------------------
private void copy() {
int[] rows = theTable.getSelectedRows();
if(rows.length==0) {
JiveUtils.showJiveError("Nothing to copy.");
return;
}
JiveUtils.the_clipboard.clear();
for(int i=0;i1) ProgressFrame.displayProgress("Renaming properties");
for(int j=0;j1) ProgressFrame.displayProgress("Deleting properties");
for(int i=0;i1) ProgressFrame.displayProgress("Creating properties");
for(int j=0;j
© 2015 - 2025 Weber Informatics LLC | Privacy Policy