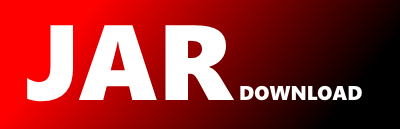
jive3.SingleAttributePanel Maven / Gradle / Ivy
The newest version!
package jive3;
import jive.JiveUtils;
import jive.MultiLineCellEditor;
import jive.MultiLineCellRenderer;
import javax.swing.*;
import javax.swing.border.Border;
import javax.swing.table.DefaultTableModel;
import java.awt.*;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
public class SingleAttributePanel extends JPanel implements ActionListener {
private final static int NB_ROW = 31;
private TaskSingleAttributeNode[] source = null;
private JTable attTable;
private DefaultTableModel attModel;
MultiLineCellEditor editor;
private JButton refreshBtn;
private JButton applyBtn;
private boolean[] updatedProp;
public SingleAttributePanel() {
updatedProp = new boolean[NB_ROW];
setLayout(new BorderLayout());
// -- Attribute table -------------------------------
attModel = new DefaultTableModel() {
public Class getColumnClass(int columnIndex) {
return String.class;
}
public boolean isCellEditable(int row, int column) {
return (column!=0) && (!JiveUtils.readOnly)
&& (row!=0) && (row!=3) && (row!=6) && (row!=10) && (row!=12) && (row!=15)
&& (row!=19) && (row!=22) && (row!=29);
}
public void setValueAt(Object aValue, int row, int column) {
if(!aValue.equals(getValueAt(row,column))) {
super.setValueAt(aValue,row,column);
applyBtn.setEnabled(true);
updatedProp[row] = true;
}
}
};
attTable = new JTable(attModel);
editor = new MultiLineCellEditor(attTable);
attTable.setDefaultEditor(String.class, editor);
MultiLineCellRenderer renderer = new MultiLineCellRenderer(true,true,false);
attTable.setDefaultRenderer(String.class, renderer);
JScrollPane attView = new JScrollPane(attTable);
add(attView,BorderLayout.CENTER);
// Button
JPanel btnPanel = new JPanel();
btnPanel.setLayout(new FlowLayout(FlowLayout.LEFT));
add(btnPanel,BorderLayout.SOUTH);
refreshBtn = new JButton("Refresh");
refreshBtn.addActionListener(this);
btnPanel.add(refreshBtn);
applyBtn = new JButton("Apply");
applyBtn.addActionListener(this);
btnPanel.add(applyBtn);
Border b = BorderFactory.createTitledBorder(BorderFactory.createEtchedBorder(),"...");
setBorder(b);
}
public void actionPerformed(ActionEvent e) {
Object src = e.getSource();
if( src==refreshBtn ) {
refreshValue();
} else if ( src==applyBtn ) {
saveChange();
}
}
public void setSource(TaskSingleAttributeNode[] src) {
this.source = src;
refreshValue();
}
public boolean hasChanged() {
return applyBtn.isEnabled();
}
public void saveChange() {
String newVal;
// Update property
int nb = getNbUpdated() * source.length;
int k = 0;
if (nb > 1) ProgressFrame.displayProgress("Updating properties");
for (int i = 0; i < updatedProp.length; i++) {
if (updatedProp[i]) {
for (int j = 0; j < source.length; j++) {
k++;
ProgressFrame.setProgress("Applying " + source[j].getTitle(),
(k * 100) / nb);
switch (i) {
case 1:
case 2:
// Polling
boolean polled = ((String) (attModel.getValueAt(1, 1))).equalsIgnoreCase("true");
String period = (String) attModel.getValueAt(2, 1);
source[j].setAttributePolling(polled, period);
break;
case 4:
// Absolute change event
newVal = (String) attModel.getValueAt(4, 1);
source[j].setAbsoluteChange(newVal);
break;
case 5:
// Relative change event
newVal = (String) attModel.getValueAt(5, 1);
source[j].setRelativeChange(newVal);
break;
case 7:
// Archive Absolute change event
newVal = (String) attModel.getValueAt(7, 1);
source[j].setArchAbsoluteChange(newVal);
break;
case 8:
// Archive Relative change event
newVal = (String) attModel.getValueAt(8, 1);
source[j].setArchRelativeChange(newVal);
break;
case 9:
// Archive Period event
newVal = (String) attModel.getValueAt(9, 1);
source[j].setArchPeriod(newVal);
break;
case 11:
// Periodic event
newVal = (String) attModel.getValueAt(11, 1);
source[j].setPeriod(newVal);
break;
case 13:
// Label
newVal = (String) attModel.getValueAt(13, 1);
source[j].setLabel(newVal);
break;
case 14:
// Format
newVal = (String) attModel.getValueAt(14, 1);
source[j].setFormat(newVal);
break;
case 16:
// Unit
newVal = (String) attModel.getValueAt(16, 1);
source[j].setUnit(newVal);
break;
case 17:
// Display Unit
newVal = (String) attModel.getValueAt(17, 1);
source[j].setDisplayUnit(newVal);
break;
case 18:
// Standard Unit
newVal = (String) attModel.getValueAt(18, 1);
source[j].setStandardUnit(newVal);
break;
case 20:
// Min
newVal = (String) attModel.getValueAt(20, 1);
source[j].setMin(newVal);
break;
case 21:
// Max
newVal = (String) attModel.getValueAt(21, 1);
source[j].setMax(newVal);
break;
case 23:
// Min alarm
newVal = (String) attModel.getValueAt(23, 1);
source[j].setMinAlarm(newVal);
break;
case 24:
// Max alarm
newVal = (String) attModel.getValueAt(24, 1);
source[j].setMaxAlarm(newVal);
break;
case 25:
// Min warning
newVal = (String) attModel.getValueAt(25, 1);
source[j].setMinWarning(newVal);
break;
case 26:
// Max warning
newVal = (String) attModel.getValueAt(26, 1);
source[j].setMaxWarning(newVal);
break;
case 27:
// Delta T
newVal = (String) attModel.getValueAt(27, 1);
source[j].setDeltaT(newVal);
break;
case 28:
// Delta Val
newVal = (String) attModel.getValueAt(28, 1);
source[j].setDeltaVal(newVal);
break;
case 30:
// Description
newVal = (String) attModel.getValueAt(30, 1);
source[j].setDescr(newVal);
break;
}
}
}
}
refreshValue();
ProgressFrame.hideProgress();
}
private int getNbUpdated() {
int k = 0;
for(int i=0;i
© 2015 - 2025 Weber Informatics LLC | Privacy Policy