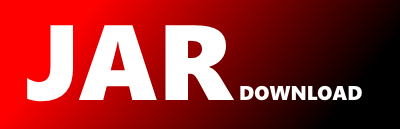
jive3.TreePanel Maven / Gradle / Ivy
The newest version!
package jive3;
import fr.esrf.TangoApi.*;
import fr.esrf.Tango.DevFailed;
import fr.esrf.Tango.DevVarLongStringArray;
import fr.esrf.tangoatk.widget.util.ATKGraphicsUtils;
import javax.swing.*;
import javax.swing.event.TreeExpansionEvent;
import javax.swing.event.TreeExpansionListener;
import javax.swing.event.TreeSelectionListener;
import javax.swing.event.TreeSelectionEvent;
import javax.swing.tree.DefaultTreeModel;
import javax.swing.tree.TreePath;
import javax.swing.tree.TreeSelectionModel;
import javax.swing.tree.TreeNode;
import java.awt.event.*;
import java.awt.*;
import java.io.File;
import java.io.FileWriter;
import java.io.IOException;
import java.util.Date;
import java.util.Vector;
import jive.JiveUtils;
import jive.ExecDev;
import static java.awt.event.InputEvent.CTRL_DOWN_MASK;
class Action {
static TangoNode[] selectedNodes = null;
Action(int a,boolean aM,String n) {
this(a,aM,n,-1,0);
}
Action(int a,boolean aM,String n,int keyCode,int modifier) {
action = a;
allowMultiple = aM;
name = n;
menuItem = new JMenuItem(name);
this.keyStroke = null;
if( keyCode>=0 ) {
keyStroke = KeyStroke.getKeyStroke(keyCode, modifier);
menuItem.setAccelerator(keyStroke);
}
menuItem.addActionListener(new ActionListener(){
public void actionPerformed(ActionEvent e) {
execAction();
}
});
}
boolean isShortcut(KeyStroke ks) {
if (keyStroke==null) return false;
return keyStroke.getKeyCode() == ks.getKeyCode() && keyStroke.getModifiers()==ks.getModifiers();
}
void execAction() {
if(selectedNodes!=null) {
if(allowMultiple && selectedNodes.length>1) {
Vector errors = new Vector();
switch(action) {
case TreePanel.ACTION_DELETE:
int ok = JOptionPane.showConfirmDialog(TreePanel.invoker, "Delete " + selectedNodes.length + " items ?",
"Confirm delete", JOptionPane.YES_NO_OPTION);
if (ok == JOptionPane.YES_OPTION) {
for(int i=0;i0)
JiveUtils.showJiveErrors(errors);
}
TreePanel.panelInvoker.refresh();
break;
case TreePanel.ACTION_SAVESERVER:
File file = TreePanel.getSaveFile(TreePanel.invoker);
if( file!=null ) {
try {
TreePanel.globalResFile = new FileWriter(file.getAbsolutePath());
Date date = new Date(System.currentTimeMillis());
TreePanel.globalResFile.write("#\n# Resource backup , created " + date + "\n#\n\n");
for(int i=0;i0)
selectedNodes[0].execAction(action,false);
} catch (IOException e) {
JiveUtils.showJiveError("Error while performing " + name + " !\n");
}
}
}
}
String name;
int action;
boolean allowMultiple;
JMenuItem menuItem;
KeyStroke keyStroke;
}
/**
* An abstract class for tree panel.
*/
public abstract class TreePanel extends JPanel implements TreeSelectionListener,MouseListener,TreeExpansionListener {
protected JTree tree;
protected JScrollPane treeView = null;
protected DefaultTreeModel treeModel;
protected TangoNode root;
protected Database db;
TreePanel self;
private boolean updateOnChange;
// Actions
public final static int ACTION_COPY = 0;
public final static int ACTION_PASTE = 1;
public final static int ACTION_RENAME = 2;
public final static int ACTION_DELETE = 3;
public final static int ACTION_ADDCLASS = 4;
public final static int ACTION_TESTADMIN = 5;
public final static int ACTION_SAVESERVER = 6;
public final static int ACTION_CLASSWIZ = 7;
public final static int ACTION_ADDDEVICE = 8;
public final static int ACTION_DEVICESWIZ = 9;
public final static int ACTION_MONITORDEV = 10;
public final static int ACTION_TESTDEV = 11;
public final static int ACTION_DEFALIAS = 12;
public final static int ACTION_GOTODEVNODE = 13;
public final static int ACTION_RESTART = 14;
public final static int ACTION_DEVICEWIZ = 15;
public final static int ACTION_GOTOSERVNODE = 16;
public final static int ACTION_GOTOADMINNODE = 17;
public final static int ACTION_ADDCLASSATT = 18;
public final static int ACTION_UNEXPORT = 19;
public final static int ACTION_SELECT_PROP = 20;
public final static int ACTION_SELECT_POLLING = 21;
public final static int ACTION_SELECT_EVENT = 22;
public final static int ACTION_SELECT_ATTCONF = 23;
public final static int ACTION_SELECT_LOGGING = 24;
public final static int ACTION_LOG_VIEWER = 25;
public final static int ACTION_DEV_DEPEND = 26;
public final static int ACTION_THREAD_POLL = 27;
public final static int ACTION_VIEW_HISTORY = 28;
public final static int ACTION_MOVE_SERVER = 29;
public final static int ACTION_CREATE_ATTPROP = 30;
public final static int ACTION_START_SERVER = 31;
public final static int ACTION_STOP_SERVER = 32;
public final static int ACTION_RESTART_SERVER = 33;
public final static int ACTION_START_LEVEL = 34;
public final static int ACTION_STOP_LEVEL = 35;
public final static int ACTION_START_HOST = 36;
public final static int ACTION_STOP_HOST = 37;
public final static int ACTION_CH_HOST_USAGE = 38;
public final static int ACTION_GO_TO_STATER = 39;
public final static int ACTION_CH_LEVEL = 40;
public final static int ACTION_TERMINAL = 41;
public final static int ACTION_NEW_SERVERS = 42;
public final static int ACTION_COPY_ATT_SET = 43;
public final static int ACTION_SAVE_PROP = 44;
public final static int ACTION_FIND_USAGE = 45;
public final static int ACTION_UNEXPORT_HOST = 46;
public final static Action[] actions = {
new Action(ACTION_COPY,false,"Copy",KeyEvent.VK_C,InputEvent.CTRL_DOWN_MASK),
new Action(ACTION_PASTE,false,"Paste",KeyEvent.VK_V,InputEvent.CTRL_DOWN_MASK),
new Action(ACTION_RENAME,false,"Rename",KeyEvent.VK_F2,0),
new Action(ACTION_DELETE,true,"Delete",KeyEvent.VK_DELETE,0),
new Action(ACTION_ADDCLASS,false,"Add class"),
new Action(ACTION_TESTADMIN,false,"Test admin server"),
new Action(ACTION_SAVESERVER,true,"Save server data"),
new Action(ACTION_CLASSWIZ,false,"Class(es) wizard"),
new Action(ACTION_ADDDEVICE,false,"Add device"),
new Action(ACTION_DEVICESWIZ,false,"Device(s) wizard"),
new Action(ACTION_MONITORDEV,true,"Monitor device"),
new Action(ACTION_TESTDEV,true,"Test device"),
new Action(ACTION_DEFALIAS,false,"Define device alias"),
new Action(ACTION_GOTODEVNODE,false,"Go to device node"),
new Action(ACTION_RESTART,false,"Restart device"),
new Action(ACTION_DEVICEWIZ,false,"Device wizard"),
new Action(ACTION_GOTOSERVNODE,false,"Go to server node"),
new Action(ACTION_GOTOADMINNODE,false,"Go to device admin node"),
new Action(ACTION_ADDCLASSATT,false,"Add attribute"),
new Action(ACTION_UNEXPORT,false,"Unexport devices"),
new Action(ACTION_SELECT_PROP,false,"Select 'property node'"),
new Action(ACTION_SELECT_POLLING,false,"Select 'polling' nodes"),
new Action(ACTION_SELECT_EVENT,false,"Select 'event' nodes"),
new Action(ACTION_SELECT_ATTCONF,false,"Select 'attribute config' nodes"),
new Action(ACTION_SELECT_LOGGING,false,"Select 'logging' nodes"),
new Action(ACTION_LOG_VIEWER,false,"Log Viewer"),
new Action(ACTION_DEV_DEPEND,false,"Devices dependencies"),
new Action(ACTION_THREAD_POLL,false,"Polling threads manager"),
new Action(ACTION_VIEW_HISTORY,false,"View history"),
new Action(ACTION_MOVE_SERVER,false,"Move server"),
new Action(ACTION_CREATE_ATTPROP,false,"Create attribute property"),
new Action(ACTION_START_SERVER,false,"Start Server"),
new Action(ACTION_STOP_SERVER,false,"Stop Server"),
new Action(ACTION_RESTART_SERVER,false,"Restart Server"),
new Action(ACTION_START_LEVEL,false,"Start all servers (Level)"),
new Action(ACTION_STOP_LEVEL,false,"Stop all servers (Level)"),
new Action(ACTION_START_HOST,false,"Start all servers (Host)"),
new Action(ACTION_STOP_HOST,false,"Stop all servers (Host)"),
new Action(ACTION_CH_HOST_USAGE,false,"Edit Host Usage"),
new Action(ACTION_GO_TO_STATER,false,"Go to Starter Node"),
new Action(ACTION_CH_LEVEL,false,"Change Level"),
new Action(ACTION_TERMINAL,false,"Open terminal"),
new Action(ACTION_NEW_SERVERS,false,"Start new servers"),
new Action(ACTION_COPY_ATT_SET,false,"Copy Setpoints"),
new Action(ACTION_SAVE_PROP,false,"Save properties",KeyEvent.VK_S,InputEvent.CTRL_DOWN_MASK),
new Action(ACTION_FIND_USAGE,false,"Find usage"),
new Action(ACTION_UNEXPORT_HOST,false,"Unexport devices"),
};
static File lastFile = null;
static FileWriter globalResFile;
static MainPanel invoker;
static TreePanel panelInvoker;
private static JPopupMenu actionMenu;
static {
actionMenu = new JPopupMenu();
for(int i=0;iPolling Status\n
\n";
String[] pi = ds.polling_status();
for (i = 0; i < pi.length; i++) result += (pi[i] + "\n\n");
} catch (DevFailed e) {
for (i = 0; i < e.errors.length; i++) {
result += "Desc -> " + e.errors[i].desc + "\n";
result += "Reason -> " + e.errors[i].reason + "\n";
result += "Origin -> " + e.errors[i].origin + "\n";
}
}
return result;
}
// ---------------------------------------------------------------
public void findUsage(String devName) {
try {
DeviceData argin = new DeviceData();
String request = "select distinct device from property_device where name=\"__SubDevices\" and value=\"" + devName.toLowerCase() + "\";";
argin.insert(request);
DeviceData argout = db.command_inout("DbMySqlSelect",argin);
DevVarLongStringArray arg = argout.extractLongStringArray();
StringBuffer str = new StringBuffer();
for(int i=0;i1) {
int hasMultiple[] = new int[actions.length];
for(int i=0;i 0) {
for(int i = 0; i < actions.length; i++)
actionMenu.getComponent(i).setVisible(false);
for(int i = 0; i < actionsNode.length;i ++)
actionsNode[i].menuItem.setVisible(true);
actionMenu.show(tree, e.getX(), e.getY());
}
}
// Launch ATK panel on double click (when possible)
if(e.getClickCount() == 2 && e.getButton() == MouseEvent.BUTTON1 ) {
// Force single selection on double click
createSelectedNodes(new TreePath[]{selPath});
tree.setSelectionPath(selPath);
Action a = nodeAction(Action.selectedNodes[0],ACTION_MONITORDEV);
if(a!=null) a.execAction();
}
}
}
public void mouseClicked(MouseEvent e) {}
public void mouseReleased(MouseEvent e) {}
public void mouseEntered(MouseEvent e) {}
public void mouseExited(MouseEvent e) {}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy