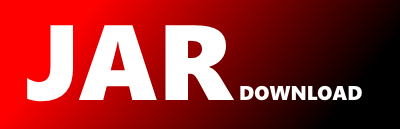
jive3.TreePanelServer Maven / Gradle / Ivy
The newest version!
package jive3;
import javax.swing.*;
import javax.swing.tree.TreePath;
import fr.esrf.Tango.DevFailed;
import fr.esrf.TangoApi.*;
import java.awt.*;
import java.io.File;
import java.io.FileWriter;
import java.io.IOException;
import java.util.Date;
import java.util.regex.Matcher;
import java.util.regex.Pattern;
import java.util.regex.PatternSyntaxException;
import fr.esrf.tangoatk.widget.util.ATKGraphicsUtils;
import jive.JiveUtils;
import jive.ServerDlg;
import jive.DevWizard;
/**
* A panel for selecting tango servers
*/
public class TreePanelServer extends TreePanel {
// Filtering stuff
String serverFilterString="*";
Pattern serverPattern=null;
String[] serverList;
public TreePanelServer() {
this.self = this;
setLayout(new BorderLayout());
}
public TangoNode createRoot() {
return new RootNode();
}
public void selectDevice(String className,String serverName,String devName) {
int slash = serverName.indexOf('/');
String server = serverName.substring(0,slash);
String instance = serverName.substring(slash+1);
// Search server
TangoNode srvNode = searchNode(root,server);
if(srvNode==null) return;
TangoNode instNode = searchNode(srvNode,instance);
if(instNode==null) return;
TangoNode classNode = searchNode(instNode,className);
if(classNode==null) return;
TangoNode devNode = searchNode(classNode,devName);
if(devNode==null) return;
TreePath selPath = new TreePath(root);
selPath = selPath.pathByAddingChild(srvNode);
selPath = selPath.pathByAddingChild(instNode);
selPath = selPath.pathByAddingChild(classNode);
selPath = selPath.pathByAddingChild(devNode);
tree.setSelectionPath(selPath);
}
public void selectClass(String className,String serverName) {
int slash = serverName.indexOf('/');
String server = serverName.substring(0,slash);
String instance = serverName.substring(slash+1);
// Search server
TangoNode srvNode = searchNode(root,server);
if(srvNode==null) return;
TangoNode instNode = searchNode(srvNode,instance);
if(instNode==null) return;
TangoNode classNode = searchNode(instNode,className);
if(classNode==null) return;
TreePath selPath = new TreePath(root);
selPath = selPath.pathByAddingChild(srvNode);
selPath = selPath.pathByAddingChild(instNode);
selPath = selPath.pathByAddingChild(classNode);
tree.setSelectionPath(selPath);
}
public boolean isServer(String exeName) {
TangoNode srvNode = searchNode(root,exeName);
return srvNode!=null;
}
public TangoNode selectFullServer(String serverName) {
int slash = serverName.indexOf('/');
String server = serverName.substring(0,slash);
String instance = serverName.substring(slash+1);
// Search server
TangoNode srvNode = searchNode(root,server);
if(srvNode==null) return null;
TangoNode instNode = searchNode(srvNode,instance);
if(instNode==null) return null;
TreePath selPath = new TreePath(root);
selPath = selPath.pathByAddingChild(srvNode);
selPath = selPath.pathByAddingChild(instNode);
tree.setSelectionPath(selPath);
return instNode;
}
public TangoNode selectServerRoot(String serverName) {
// Search server
TangoNode srvNode = searchNode(root,serverName);
if(srvNode==null) return null;
TreePath selPath = new TreePath(root);
selPath = selPath.pathByAddingChild(srvNode);
tree.setSelectionPath(selPath);
return srvNode;
}
public void applyFilter(String filter) {
serverFilterString = filter;
if( filter.equals("*") ) {
serverPattern = null;
} else if (filter.length()==0) {
serverPattern = null;
} else {
try {
String f = filterToRegExp(serverFilterString);
serverPattern = Pattern.compile(f);
} catch (PatternSyntaxException e) {
JOptionPane.showMessageDialog(invoker,e.getMessage());
}
}
}
public String getFilter() {
return serverFilterString;
}
public String[] getServerList() {
return serverList;
}
// ---------------------------------------------------------------
class RootNode extends TangoNode {
void populateNode() throws DevFailed {
serverList = db.get_server_name_list();
for (int i = 0; i < serverList.length; i++) {
if( serverPattern!=null ) {
Matcher matcher = serverPattern.matcher(serverList[i].toLowerCase());
if( matcher.find() && matcher.start()==0 && matcher.end()==serverList[i].length() ) {
add(new ServerNode(serverList[i]));
}
} else {
add(new ServerNode(serverList[i]));
}
}
}
public String toString() {
return "Server:";
}
void execAction(int number,boolean multipleCall) {
}
}
// ---------------------------------------------------------------
class ServerNode extends TangoNode {
private String server;
ServerNode(String server) {
this.server = server;
}
void populateNode() throws DevFailed {
String[] list = db.get_instance_name_list(server);
for (int i = 0; i < list.length; i++)
add(new InstanceNode(server, list[i]));
}
public String toString() {
return server;
}
ImageIcon getIcon() {
return TangoNodeRenderer.srvicon;
}
Action[] getAction() {
if (JiveUtils.readOnly)
return new Action[0];
else
return new Action[]{TreePanel.getAction(ACTION_RENAME)};
}
void execAction(int action,boolean multipleCall) {
switch(action) {
// ---------------------------------------------------------------------------
case ACTION_RENAME:
// Rename a server
String newName = JOptionPane.showInputDialog(null,"Rename server",server);
if(newName==null) return;
if(searchNodeCaseSensitive(root,newName)!=null) {
JiveUtils.showJiveError("Name already exists.");
return;
}
// Clone instances,classes and devices
for(int i=0;i " + e.errors[i].desc + "\n";
result += "Reason -> " + e.errors[i].reason + "\n";
result += "Origin -> " + e.errors[i].origin + "\n";
}
}
return result;
}
String getTitle() {
return "Server Info";
}
Action[] getAction() {
if (JiveUtils.readOnly) {
return new Action[]{
TreePanel.getAction(ACTION_TESTADMIN),
TreePanel.getAction(ACTION_SAVESERVER)
};
} else {
return new Action[]{
TreePanel.getAction(ACTION_RENAME),
TreePanel.getAction(ACTION_DELETE),
TreePanel.getAction(ACTION_ADDCLASS),
TreePanel.getAction(ACTION_TESTADMIN),
TreePanel.getAction(ACTION_SAVESERVER),
TreePanel.getAction(ACTION_CLASSWIZ),
TreePanel.getAction(ACTION_UNEXPORT),
TreePanel.getAction(ACTION_DEV_DEPEND),
TreePanel.getAction(ACTION_THREAD_POLL),
TreePanel.getAction(ACTION_MOVE_SERVER)
};
}
}
void execAction(int action,boolean multipleCall) throws IOException {
int ok;
switch(action) {
// ----------------------------------------------------------------------------
case ACTION_RENAME:
// Rename an instance
String newName = JOptionPane.showInputDialog(null,"Rename instance",instance);
if(newName==null) return;
if(searchNode((TangoNode)getParent(),newName)!=null) {
JiveUtils.showJiveError("Name already exists.");
return;
}
// Clone classes and devices
for(int i=0;i 0) {
DbDatum[] data = db.get_device_property(devName, propList);
db.put_device_property(nDevName, data);
}
// Clone attributes properties
try {
String[] attList = db.get_device_attribute_list(devName);
if (attList.length > 0) {
DbAttribute[] adata = db.get_device_attribute_property(devName, attList);
db.put_device_attribute_property(nDevName, adata);
}
} catch (DevFailed e3) {
JiveUtils.showJiveError("Failed to copy attribute properties of " + devName + "\n" + e3.errors[0].desc);
}
}
// Remove the old device
if(isAlive)
JiveUtils.showJiveWarning("The old device " + devName + " is still alive and should be removed by hand.");
else
db.delete_device(devName);
} catch (DevFailed e4) {
JiveUtils.showTangoError(e4);
}
}
return success;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy