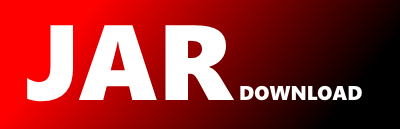
fr.esrf.TangoApi.DeviceProxyDAODefaultImpl Maven / Gradle / Ivy
//+======================================================================
// $Source$
//
// Project: Tango
//
// Description: java source code for the TANGO client/server API.
//
// $Author: pascal_verdier $
//
// Copyright (C) : 2004,2005,2006,2007,2008,2009,2010,2011,2012,2013,2014,
// European Synchrotron Radiation Facility
// BP 220, Grenoble 38043
// FRANCE
//
// This file is part of Tango.
//
// Tango is free software: you can redistribute it and/or modify
// it under the terms of the GNU Lesser General Public License as published by
// the Free Software Foundation, either version 3 of the License, or
// (at your option) any later version.
//
// Tango is distributed in the hope that it will be useful,
// but WITHOUT ANY WARRANTY; without even the implied warranty of
// MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
// GNU Lesser General Public License for more details.
//
// You should have received a copy of the GNU Lesser General Public License
// along with Tango. If not, see .
//
// $Revision: 30265 $
//
//-======================================================================
package fr.esrf.TangoApi;
import fr.esrf.Tango.*;
import fr.esrf.TangoApi.events.EventConsumerUtil;
import fr.esrf.TangoDs.Except;
import fr.esrf.TangoDs.NamedDevFailed;
import fr.esrf.TangoDs.NamedDevFailedList;
import fr.esrf.TangoDs.TangoConst;
import org.omg.CORBA.*;
import java.util.ArrayList;
import java.util.List;
/**
* Class Description: This class manage device connection for Tango objects. It
* is an api between user and IDL Device object.
*
* @author verdier
* @version $Revision: 30265 $
*/
public class DeviceProxyDAODefaultImpl extends ConnectionDAODefaultImpl implements ApiDefs,
IDeviceProxyDAO {
public DeviceProxyDAODefaultImpl() {
// super();
}
// ===================================================================
/**
* Default DeviceProxy constructor. It will do nothing
*/
// ===================================================================
public void init(final DeviceProxy deviceProxy) throws DevFailed {
// super.init(deviceProxy);
}
// ===================================================================
/**
* DeviceProxy constructor. It will import the device.
*
* @param devname name of the device to be imported.
*/
// ===================================================================
public void init(final DeviceProxy deviceProxy, final String devname) throws DevFailed {
// super.init(deviceProxy, devname);
deviceProxy.setFull_class_name("DeviceProxy(" + name(deviceProxy) + ")");
}
// ===================================================================
/**
* DeviceProxy constructor. It will import the device.
*
* @param devname name of the device to be imported.
* @param check_access set check_access value
*/
// ===================================================================
public void init(final DeviceProxy deviceProxy, final String devname, final boolean check_access)
throws DevFailed {
// super.init(deviceProxy, devname, check_access);
deviceProxy.setFull_class_name("DeviceProxy(" + name(deviceProxy) + ")");
}
// ===================================================================
/**
* TangoDevice constructor. It will import the device.
*
* @param devname name of the device to be imported.
* @param ior ior string used to import device
*/
// ===================================================================
public void init(final DeviceProxy deviceProxy, final String devname, final String ior)
throws DevFailed {
// super.init(deviceProxy, devname, ior, FROM_IOR);
deviceProxy.setFull_class_name("DeviceProxy(" + name(deviceProxy) + ")");
}
// ===================================================================
/**
* TangoDevice constructor. It will import the device.
*
* @param devname name of the device to be imported.
* @param host host where database is running.
* @param port port for database connection.
*/
// ===================================================================
public void init(final DeviceProxy deviceProxy, final String devname, final String host,
final String port) throws DevFailed {
// super.init(deviceProxy, devname, host, port);
deviceProxy.setFull_class_name("DeviceProxy(" + name(deviceProxy) + ")");
}
// ==========================================================================
// ==========================================================================
public boolean use_db(final DeviceProxy deviceProxy) {
return deviceProxy.url.use_db;
}
// ==========================================================================
// ==========================================================================
private void checkIfUseDb(final DeviceProxy deviceProxy, final String origin) throws DevFailed {
if (!deviceProxy.url.use_db) {
Except.throw_non_db_exception("Api_NonDatabaseDevice", "Device " + name(deviceProxy)
+ " do not use database", "DeviceProxy(" + name(deviceProxy) + ")." + origin);
}
}
// ==========================================================================
// ==========================================================================
public Database get_db_obj(final DeviceProxy deviceProxy) throws DevFailed {
checkIfUseDb(deviceProxy, "get_db_obj()");
return ApiUtil.get_db_obj(deviceProxy.url.host, deviceProxy.url.strPort);
}
// ===================================================================
/**
* Get connection on administration device.
*/
// ===================================================================
public void import_admin_device(final DeviceProxy deviceProxy, final DbDevImportInfo info)
throws DevFailed {
checkIfTango(deviceProxy, "import_admin_device()");
/*
* if (deviceProxy.device==null && deviceProxy.devname!=null)
* build_connection(deviceProxy);
*/
// Get connection on administration device
if (deviceProxy.getAdm_dev() == null) {
if (DeviceProxyFactory.exists(info.name)) {
deviceProxy.setAdm_dev(DeviceProxyFactory.get(info.name));
} else {
// If not exists, create it with info
deviceProxy.setAdm_dev(new DeviceProxy(info));
}
}
}
// ===================================================================
/**
* Get connection on administration device.
*/
// ===================================================================
public void import_admin_device(final DeviceProxy deviceProxy, final String origin)
throws DevFailed {
checkIfTango(deviceProxy, origin);
build_connection(deviceProxy);
// Get connection on administration device
if (deviceProxy.getAdm_dev() == null) {
deviceProxy.setAdm_dev(DeviceProxyFactory.get(
adm_name(deviceProxy), deviceProxy.getUrl().getTangoHost()));
}
}
// ===========================================================
/**
* return the device name.
*/
// ===========================================================
public String name(final DeviceProxy deviceProxy) {
return get_name(deviceProxy);
}
// ===========================================================
/**
* return the device status read from CORBA attribute.
*/
// ===========================================================
public String status(final DeviceProxy deviceProxy) throws DevFailed {
return status(deviceProxy, ApiDefs.FROM_ATTR);
}
// ===========================================================
/**
* return the device status.
*
* @param src Source to read status. It could be ApiDefs.FROM_CMD to read it
* from a command_inout or ApiDefs.FROM_ATTR to read it from
* CORBA attribute.
*/
// ===========================================================
public String status(final DeviceProxy deviceProxy, final boolean src) throws DevFailed {
build_connection(deviceProxy);
if (deviceProxy.url.protocol == TANGO) {
String status = "Unknown";
final int retries = deviceProxy.transparent_reconnection ? 2 : 1;
boolean done = false;
for (int i = 0; i < retries && !done; i++) {
try {
//noinspection PointlessBooleanExpression
if (src == ApiDefs.FROM_ATTR) {
status = deviceProxy.device.status();
} else {
final DeviceData argout = deviceProxy.command_inout("Status");
status = argout.extractString();
}
done = true;
} catch (final Exception e) {
manageExceptionReconnection(deviceProxy, retries, i, e, this.getClass()
+ ".status");
}
}
return status;
} else {
return command_inout(deviceProxy, "DevStatus").extractString();
}
}
// ===========================================================
/**
* return the device state read from CORBA attribute.
*/
// ===========================================================
public DevState state(final DeviceProxy deviceProxy) throws DevFailed {
return state(deviceProxy, ApiDefs.FROM_ATTR);
}
// ===========================================================
/**
* return the device state.
*
* @param src Source to read state. It could be ApiDefs.FROM_CMD to read it
* from a command_inout or ApiDefs.FROM_ATTR to read it from
* CORBA attribute.
*/
// ===========================================================
public DevState state(final DeviceProxy deviceProxy, final boolean src) throws DevFailed {
build_connection(deviceProxy);
if (deviceProxy.url.protocol == TANGO) {
DevState state = DevState.UNKNOWN;
final int retries = deviceProxy.transparent_reconnection ? 2 : 1;
boolean done = false;
for (int i = 0; i < retries && !done; i++) {
try {
//noinspection PointlessBooleanExpression
if (src == ApiDefs.FROM_ATTR) {
state = deviceProxy.device.state();
} else {
final DeviceData argout = deviceProxy.command_inout("State");
state = argout.extractDevState();
}
done = true;
} catch (final Exception e) {
manageExceptionReconnection(deviceProxy,
retries, i, e, this.getClass() + ".state");
}
}
return state;
} else {
final DeviceData argout = command_inout(deviceProxy, "DevState");
final short state = argout.extractShort();
return T2Ttypes.tangoState(state);
}
}
// ===========================================================
/**
* return the IDL device command_query for the specified command.
*
* @param commandName command name to be used for the command_query
* @return the command information..
*/
// ===========================================================
public CommandInfo command_query(final DeviceProxy deviceProxy,
final String commandName) throws DevFailed {
build_connection(deviceProxy);
CommandInfo info = null;
if (deviceProxy.url.protocol == TANGO) {
// try 2 times for reconnection if requested
final int retries = deviceProxy.transparent_reconnection ? 2 : 1;
for (int i=0 ; i
* [0] Device Class
* [1] Class from the device class is inherited.
* .....And so on
*
*/
// ==========================================================================
public String[] get_class_inheritance(final DeviceProxy deviceProxy) throws DevFailed {
checkIfUseDb(deviceProxy, "get_class_inheritance()");
if (deviceProxy.getDb_dev() == null) {
deviceProxy.setDb_dev(new DbDevice(deviceProxy.devname, deviceProxy.url.host,
deviceProxy.url.strPort));
}
checkIfTango(deviceProxy, "get_class_inheritance");
return deviceProxy.getDb_dev().get_class_inheritance();
}
// ==========================================================================
/**
* Set an alias for a device name
*
* @param aliasName alias name.
*/
// ==========================================================================
public void put_alias(final DeviceProxy deviceProxy, final String aliasName) throws DevFailed {
checkIfUseDb(deviceProxy, "put_alias()");
if (deviceProxy.getDb_dev() == null) {
deviceProxy.setDb_dev(new DbDevice(deviceProxy.devname, deviceProxy.url.host,
deviceProxy.url.strPort));
}
checkIfTango(deviceProxy, "put_alias");
deviceProxy.getDb_dev().put_alias(aliasName);
}
// ==========================================================================
/**
* Get alias for the device
*
* @return the alias name if found.
*/
// ==========================================================================
public String get_alias(final DeviceProxy deviceProxy) throws DevFailed {
checkIfUseDb(deviceProxy, "get_alias()");
if (deviceProxy.getDb_dev() == null) {
deviceProxy.setDb_dev(new DbDevice(deviceProxy.devname, deviceProxy.url.host,
deviceProxy.url.strPort));
}
checkIfTango(deviceProxy, "get_alias");
return deviceProxy.getDb_dev().get_alias();
}
// ==========================================================================
/**
* Query the database for the info of this device.
*
* @return the information in a DeviceInfo.
*/
// ==========================================================================
public DeviceInfo get_info(final DeviceProxy deviceProxy) throws DevFailed {
checkIfUseDb(deviceProxy, "get_info()");
if (deviceProxy.getDb_dev() == null) {
deviceProxy.setDb_dev(new DbDevice(deviceProxy.devname, deviceProxy.url.host,
deviceProxy.url.strPort));
}
// checkIfTango("import_device");
if (deviceProxy.url.protocol == TANGO) {
return deviceProxy.getDb_dev().get_info();
} else {
return null;
}
}
// ==========================================================================
/**
* Query the database for the export info of this device.
*
* @return the information in a DbDevImportInfo.
*/
// ==========================================================================
public DbDevImportInfo import_device(final DeviceProxy deviceProxy) throws DevFailed {
checkIfUseDb(deviceProxy, "import_device()");
if (deviceProxy.getDb_dev() == null) {
deviceProxy.setDb_dev(new DbDevice(deviceProxy.devname, deviceProxy.url.host,
deviceProxy.url.strPort));
}
// checkIfTango("import_device");
if (deviceProxy.url.protocol == TANGO) {
return deviceProxy.getDb_dev().import_device();
} else {
return new DbDevImportInfo(dev_inform(deviceProxy));
}
}
// ==========================================================================
/**
* Update the export info for this device in the database.
*
* @param devinfo Device information to export.
*/
// ==========================================================================
public void export_device(final DeviceProxy deviceProxy,
final DbDevExportInfo devinfo) throws DevFailed {
checkIfTango(deviceProxy, "export_device");
checkIfUseDb(deviceProxy, "export_device()");
if (deviceProxy.getDb_dev() == null) {
deviceProxy.setDb_dev(new DbDevice(deviceProxy.devname, deviceProxy.url.host,
deviceProxy.url.strPort));
}
deviceProxy.getDb_dev().export_device(devinfo);
}
// ==========================================================================
/**
* Unexport the device in database.
*/
// ==========================================================================
public void unexport_device(final DeviceProxy deviceProxy) throws DevFailed {
checkIfTango(deviceProxy, "unexport_device");
checkIfUseDb(deviceProxy, "unexport_device()");
if (deviceProxy.getDb_dev() == null) {
deviceProxy.setDb_dev(new DbDevice(deviceProxy.devname, deviceProxy.url.host,
deviceProxy.url.strPort));
}
deviceProxy.getDb_dev().unexport_device();
}
// ==========================================================================
/**
* Add/update this device to the database
*
* @param devinfo
* The device name, class and server specified in object.
*/
// ==========================================================================
public void add_device(final DeviceProxy deviceProxy, final DbDevInfo devinfo) throws DevFailed {
checkIfTango(deviceProxy, "add_device");
checkIfUseDb(deviceProxy, "add_device()");
if (deviceProxy.getDb_dev() == null) {
deviceProxy.setDb_dev(new DbDevice(deviceProxy.devname, deviceProxy.url.host,
deviceProxy.url.strPort));
}
deviceProxy.getDb_dev().add_device(devinfo);
}
// ==========================================================================
/**
* Delete this device from the database
*/
// ==========================================================================
public void delete_device(final DeviceProxy deviceProxy) throws DevFailed {
checkIfTango(deviceProxy, "delete_device");
checkIfUseDb(deviceProxy, "delete_device()");
if (deviceProxy.getDb_dev() == null) {
deviceProxy.setDb_dev(new DbDevice(deviceProxy.devname, deviceProxy.url.host,
deviceProxy.url.strPort));
}
deviceProxy.getDb_dev().delete_device();
}
// ==========================================================================
/**
* Query the database for a list of device properties for the pecified
* object.
*
* @param wildcard propertie's wildcard (* matches any charactere).
* @return properties in DbDatum objects.
*/
// ==========================================================================
public String[] get_property_list(final DeviceProxy deviceProxy,
final String wildcard) throws DevFailed {
checkIfTango(deviceProxy, "get_property_list");
checkIfUseDb(deviceProxy, "get_property_list()");
if (deviceProxy.getDb_dev() == null) {
deviceProxy.setDb_dev(new DbDevice(deviceProxy.devname, deviceProxy.url.host,
deviceProxy.url.strPort));
}
return deviceProxy.getDb_dev().get_property_list(wildcard);
}
// ==========================================================================
/**
* Query the database for a list of device properties for this device.
*
* @param propertyNames list of property names.
* @return properties in DbDatum objects.
*/
// ==========================================================================
public DbDatum[] get_property(final DeviceProxy deviceProxy,
final String[] propertyNames) throws DevFailed {
if (deviceProxy.url.protocol == TANGO) {
checkIfUseDb(deviceProxy, "get_property()");
if (deviceProxy.getDb_dev() == null) {
deviceProxy.setDb_dev(new DbDevice(deviceProxy.devname, deviceProxy.url.host,
deviceProxy.url.strPort));
}
return deviceProxy.getDb_dev().get_property(propertyNames);
} else {
if (deviceProxy.taco_device == null && deviceProxy.devname != null) {
build_connection(deviceProxy);
}
return deviceProxy.taco_device.get_property(propertyNames);
}
}
// ==========================================================================
/**
* Query the database for a device property for this device.
*
* @param propertyName property name.
* @return property in DbDatum objects.
*/
// ==========================================================================
public DbDatum get_property(final DeviceProxy deviceProxy,
final String propertyName) throws DevFailed {
if (deviceProxy.url.protocol == TANGO) {
checkIfUseDb(deviceProxy, "get_property()");
if (deviceProxy.getDb_dev() == null) {
deviceProxy.setDb_dev(new DbDevice(deviceProxy.devname, deviceProxy.url.host,
deviceProxy.url.strPort));
}
return deviceProxy.getDb_dev().get_property(propertyName);
} else {
final String[] propnames = { propertyName };
return deviceProxy.taco_device.get_property(propnames)[0];
}
}
// ==========================================================================
/**
* Query the database for a list of device properties for this device. The
* property names are specified by the DbDatum array objects.
*
* @param properties list of property DbDatum objects.
* @return properties in DbDatum objects.
*/
// ==========================================================================
public DbDatum[] get_property(final DeviceProxy deviceProxy,
final DbDatum[] properties) throws DevFailed {
checkIfUseDb(deviceProxy, "get_property()");
if (deviceProxy.getDb_dev() == null) {
deviceProxy.setDb_dev(new DbDevice(deviceProxy.devname, deviceProxy.url.host,
deviceProxy.url.strPort));
}
checkIfTango(deviceProxy, "get_property");
return deviceProxy.getDb_dev().get_property(properties);
}
// ==========================================================================
/**
* Insert or update a property for this device The property name and its
* value are specified by the DbDatum
*
* @param prop Property name and value
*/
// ==========================================================================
public void put_property(final DeviceProxy deviceProxy, final DbDatum prop) throws DevFailed {
checkIfUseDb(deviceProxy, "put_property()");
if (deviceProxy.getDb_dev() == null) {
deviceProxy.setDb_dev(new DbDevice(deviceProxy.devname, deviceProxy.url.host,
deviceProxy.url.strPort));
}
checkIfTango(deviceProxy, "put_property");
final DbDatum[] properties = new DbDatum[1];
properties[0] = prop;
put_property(deviceProxy, properties);
}
// ==========================================================================
/**
* Insert or update a list of properties for this device The property names
* and their values are specified by the DbDatum array.
*
* @param properties Properties names and values array.
*/
// ==========================================================================
public void put_property(final DeviceProxy deviceProxy,
final DbDatum[] properties) throws DevFailed {
checkIfUseDb(deviceProxy, "put_property()");
if (deviceProxy.getDb_dev() == null) {
deviceProxy.setDb_dev(new DbDevice(deviceProxy.devname, deviceProxy.url.host,
deviceProxy.url.strPort));
}
checkIfTango(deviceProxy, "put_property");
deviceProxy.getDb_dev().put_property(properties);
}
// ==========================================================================
/**
* Delete a list of properties for this device.
*
* @param propertyNames Property names.
*/
// ==========================================================================
public void delete_property(final DeviceProxy deviceProxy,
final String[] propertyNames) throws DevFailed {
checkIfUseDb(deviceProxy, "delete_property()");
if (deviceProxy.getDb_dev() == null) {
deviceProxy.setDb_dev(new DbDevice(deviceProxy.devname, deviceProxy.url.host,
deviceProxy.url.strPort));
}
checkIfTango(deviceProxy, "delete_property");
deviceProxy.getDb_dev().delete_property(propertyNames);
}
// ==========================================================================
/**
* Delete a property for this device.
*
* @param propertyName Property name.
*/
// ==========================================================================
public void delete_property(final DeviceProxy deviceProxy,
final String propertyName) throws DevFailed {
checkIfUseDb(deviceProxy, "delete_property()");
if (deviceProxy.getDb_dev() == null) {
deviceProxy.setDb_dev(new DbDevice(deviceProxy.devname, deviceProxy.url.host,
deviceProxy.url.strPort));
}
checkIfTango(deviceProxy, "delete_property");
deviceProxy.getDb_dev().delete_property(propertyName);
}
// ==========================================================================
/**
* Delete a list of properties for this device.
*
* @param properties Property DbDatum objects.
*/
// ==========================================================================
public void delete_property(final DeviceProxy deviceProxy,
final DbDatum[] properties) throws DevFailed {
checkIfUseDb(deviceProxy, "delete_property()");
if (deviceProxy.getDb_dev() == null) {
deviceProxy.setDb_dev(new DbDevice(deviceProxy.devname, deviceProxy.url.host,
deviceProxy.url.strPort));
}
checkIfTango(deviceProxy, "delete_property");
deviceProxy.getDb_dev().delete_property(properties);
}
// ============================================
// ATTRIBUTE PROPERTY MANAGEMENT
// ============================================
// ==========================================================================
/**
* Query the device for attribute config and returns names only.
*
* @return attributes list for specified device
*/
// ==========================================================================
public String[] get_attribute_list(final DeviceProxy deviceProxy) throws DevFailed {
build_connection(deviceProxy);
final int retries = deviceProxy.transparent_reconnection ? 2 : 1;
for (int oneTry=1 ; oneTry<=retries ; oneTry++) {
try {
if (deviceProxy.url.protocol == TANGO) {
// Read All attribute config
final String[] wildcard = new String[1];
if (deviceProxy.device_3 != null) {
wildcard[0] = TangoConst.Tango_AllAttr_3;
} else {
wildcard[0] = TangoConst.Tango_AllAttr;
}
final AttributeInfo[] ac = get_attribute_info(deviceProxy, wildcard);
final String[] result = new String[ac.length];
for (int i = 0; i < ac.length; i++) {
result[i] = ac[i].name;
}
return result;
} else {
return deviceProxy.taco_device.get_attribute_list();
}
}
catch (DevFailed e) {
if (oneTry>=retries)
throw e;
// else retry
}
}
return null; // cannot occur
}
// ==========================================================================
/**
* Insert or update a list of attribute properties for this device. The
* property names and their values are specified by the DbAttribute array.
*
* @param attr
* attribute names and properties (names and values) array.
*/
// ==========================================================================
public void put_attribute_property(final DeviceProxy deviceProxy,
final DbAttribute[] attr) throws DevFailed {
checkIfUseDb(deviceProxy, "put_attribute_property()");
if (deviceProxy.getDb_dev() == null) {
deviceProxy.setDb_dev(new DbDevice(deviceProxy.devname, deviceProxy.url.host,
deviceProxy.url.strPort));
}
checkIfTango(deviceProxy, "put_attribute_property");
deviceProxy.getDb_dev().put_attribute_property(attr);
}
// ==========================================================================
/**
* Insert or update an attribute properties for this device. The property
* names and their values are specified by the DbAttribute array.
*
* @param attr
* attribute name and properties (names and values).
*/
// ==========================================================================
public void put_attribute_property(final DeviceProxy deviceProxy,
final DbAttribute attr) throws DevFailed {
checkIfUseDb(deviceProxy, "put_attribute_property()");
if (deviceProxy.getDb_dev() == null) {
deviceProxy.setDb_dev(new DbDevice(deviceProxy.devname, deviceProxy.url.host,
deviceProxy.url.strPort));
}
checkIfTango(deviceProxy, "put_attribute_property");
deviceProxy.getDb_dev().put_attribute_property(attr);
}
// ==========================================================================
/**
* Delete a list of properties for this object.
*
* @param attributeName attribute name.
* @param propertyNames Property names.
*/
// ==========================================================================
public void delete_attribute_property(final DeviceProxy deviceProxy, final String attributeName,
final String[] propertyNames) throws DevFailed {
checkIfUseDb(deviceProxy, "delete_attribute_property()");
if (deviceProxy.getDb_dev() == null) {
deviceProxy.setDb_dev(new DbDevice(deviceProxy.devname, deviceProxy.url.host,
deviceProxy.url.strPort));
}
checkIfTango(deviceProxy, "delete_attribute_property");
deviceProxy.getDb_dev().delete_attribute_property(attributeName, propertyNames);
}
// ==========================================================================
/**
* Delete a property for this object.
*
* @param attributeName
* attribute name.
* @param propertyName
* Property name.
*/
// ==========================================================================
public void delete_attribute_property(final DeviceProxy deviceProxy, final String attributeName,
final String propertyName) throws DevFailed {
checkIfUseDb(deviceProxy, "delete_attribute_property()");
if (deviceProxy.getDb_dev() == null) {
deviceProxy.setDb_dev(new DbDevice(deviceProxy.devname, deviceProxy.url.host,
deviceProxy.url.strPort));
}
checkIfTango(deviceProxy, "delete_attribute_property");
deviceProxy.getDb_dev().delete_attribute_property(attributeName, propertyName);
}
// ==========================================================================
/**
* Delete a list of properties for this object.
*
* @param attr DbAttribute objects specifying attributes.
*/
// ==========================================================================
public void delete_attribute_property(final DeviceProxy deviceProxy,
final DbAttribute attr) throws DevFailed {
checkIfUseDb(deviceProxy, "delete_attribute_property()");
if (deviceProxy.getDb_dev() == null) {
deviceProxy.setDb_dev(new DbDevice(deviceProxy.devname, deviceProxy.url.host,
deviceProxy.url.strPort));
}
checkIfTango(deviceProxy, "delete_attribute_property");
deviceProxy.getDb_dev().delete_attribute_property(attr);
}
// ==========================================================================
/**
* Delete a list of properties for this object.
*
* @param attr DbAttribute objects array specifying attributes.
*/
// ==========================================================================
public void delete_attribute_property(final DeviceProxy deviceProxy,
final DbAttribute[] attr) throws DevFailed {
checkIfUseDb(deviceProxy, "delete_attribute_property()");
if (deviceProxy.getDb_dev() == null) {
deviceProxy.setDb_dev(new DbDevice(deviceProxy.devname, deviceProxy.url.host,
deviceProxy.url.strPort));
}
checkIfTango(deviceProxy, "delete_attribute_property");
deviceProxy.getDb_dev().delete_attribute_property(attr);
}
// ==========================================================================
/**
* Query the database for a list of device attribute properties for this
* device.
*
* @param attnames list of attribute names.
* @return properties in DbAttribute objects array.
*/
// ==========================================================================
public DbAttribute[] get_attribute_property(final DeviceProxy deviceProxy,
final String[] attnames) throws DevFailed {
checkIfUseDb(deviceProxy, "get_attribute_property()");
if (deviceProxy.getDb_dev() == null) {
deviceProxy.setDb_dev(new DbDevice(deviceProxy.devname, deviceProxy.url.host,
deviceProxy.url.strPort));
}
checkIfTango(deviceProxy, "get_attribute_property");
return deviceProxy.getDb_dev().get_attribute_property(attnames);
}
// ==========================================================================
/**
* Query the database for a device attribute property for this device.
*
* @param attributeName attribute name.
* @return property in DbAttribute object.
*/
// ==========================================================================
public DbAttribute get_attribute_property(final DeviceProxy deviceProxy,
final String attributeName) throws DevFailed {
checkIfUseDb(deviceProxy, "get_attribute_property()");
if (deviceProxy.getDb_dev() == null) {
deviceProxy.setDb_dev(new DbDevice(deviceProxy.devname, deviceProxy.url.host,
deviceProxy.url.strPort));
}
checkIfTango(deviceProxy, "get_attribute_property");
return deviceProxy.getDb_dev().get_attribute_property(attributeName);
}
// ==========================================================================
/**
* Delete an attribute for this object.
*
* @param attributeName attribute name.
*/
// ==========================================================================
public void delete_attribute(final DeviceProxy deviceProxy,
final String attributeName) throws DevFailed {
checkIfUseDb(deviceProxy, "delete_attribute()");
if (deviceProxy.getDb_dev() == null) {
deviceProxy.setDb_dev(new DbDevice(deviceProxy.devname, deviceProxy.url.host,
deviceProxy.url.strPort));
}
checkIfTango(deviceProxy, "delete_attribute");
deviceProxy.getDb_dev().delete_attribute(attributeName);
}
// ===========================================================
// Attribute Methods
// ===========================================================
// ==========================================================================
/**
* Get the attributes configuration for the specified device.
*
* @param attributeNames attribute names to request config.
* @return the attributes configuration.
*/
// ==========================================================================
public AttributeInfo[] get_attribute_info(final DeviceProxy deviceProxy,
final String[] attributeNames) throws DevFailed {
build_connection(deviceProxy);
final int retries = deviceProxy.transparent_reconnection ? 2 : 1;
for (int oneTry=1 ; oneTry<=retries ; oneTry++) {
try {
AttributeInfo[] result;
AttributeConfig[] ac = new AttributeConfig[0];
AttributeConfig_2[] ac_2 = null;
if (deviceProxy.url.protocol == TANGO) {
// Check IDL version
if (deviceProxy.device_2 != null) {
ac_2 = deviceProxy.device_2.get_attribute_config_2(attributeNames);
} else {
ac = deviceProxy.device.get_attribute_config(attributeNames);
}
} else {
ac = deviceProxy.taco_device.get_attribute_config(attributeNames);
}
// Convert AttributeConfig(_2) object to AttributeInfo object
final int size = ac_2 != null ? ac_2.length : ac.length;
result = new AttributeInfo[size];
for (int i = 0; i < size; i++) {
if (ac_2 != null) {
result[i] = new AttributeInfo(ac_2[i]);
} else {
result[i] = new AttributeInfo(ac[i]);
}
}
return result;
}
catch (final DevFailed e) {
if (oneTry>=retries) {
throw e;
}
}
catch (final Exception e) {
if (oneTry>=retries) {
ApiUtilDAODefaultImpl.removePendingRepliesOfDevice(deviceProxy);
throw_dev_failed(deviceProxy, e, "get_attribute_config", true);
}
}
}
return null;
}
// ==========================================================================
/**
* Get the extended attributes configuration for the specified device.
*
* @param attributeNames attribute names to request config.
* @return the attributes configuration.
*/
// ==========================================================================
public AttributeInfoEx[] get_attribute_info_ex(final DeviceProxy deviceProxy,
final String[] attributeNames) throws DevFailed {
build_connection(deviceProxy);
// try 2 times for reconnection if requested
AttributeInfoEx[] result = null;
boolean done = false;
final int retries = deviceProxy.transparent_reconnection ? 2 : 1;
for (int i=0 ; i TangoApi_READ_ONLY_MODE");
throwNotAuthorizedException(deviceProxy.devname + ".command_inout_asynch(" + cmdname + ")",
"Connection.command_inout_asynch()");
}
}
// Create the request object
// ----------------------------------
Request request;
if (deviceProxy.device_5 != null) {
request = deviceProxy.device_5._request("command_inout_4");
setRequestArgsForCmd(request, cmdname, data_in, get_source(deviceProxy), DevLockManager
.getInstance().getClntIdent());
}
else if (deviceProxy.device_4 != null) {
request = deviceProxy.device_4._request("command_inout_4");
setRequestArgsForCmd(request, cmdname, data_in, get_source(deviceProxy), DevLockManager
.getInstance().getClntIdent());
}
else if (deviceProxy.device_2 != null) {
request = deviceProxy.device_2._request("command_inout_2");
setRequestArgsForCmd(request, cmdname, data_in, get_source(deviceProxy), null);
}
else {
request = deviceProxy.device._request("command_inout");
setRequestArgsForCmd(request, cmdname, data_in, null, null);
}
// send it (deferred or just one way)
int id = 0;
// Else tango call
boolean done = false;
// try 2 times for reconnection if requested
final int retries = deviceProxy.transparent_reconnection ? 2 : 1;
for (int i = 0; i < retries && !done; i++) {
try {
if (forget) {
request.send_oneway();
} else {
request.send_deferred();
// store request reference to read reply later
final String[] names = new String[] { cmdname };
id = ApiUtil.put_async_request(
new AsyncCallObject(request, deviceProxy, CMD, names));
}
done = true;
} catch (final Exception e) {
manageExceptionReconnection(deviceProxy,
retries, i, e, this.getClass() + ".command_inout");
}
}
return id;
}
// ==========================================================================
/**
* Asynchronous command_inout using callback for reply.
*
* @param cmdname Command name.
* @param argin Input argument command.
* @param cb a CallBack object instance.
*/
// ==========================================================================
public void command_inout_asynch(final DeviceProxy deviceProxy, final String cmdname,
final DeviceData argin, final CallBack cb) throws DevFailed {
final int id = command_inout_asynch(deviceProxy, cmdname, argin, false);
ApiUtil.set_async_reply_model(id, CALLBACK);
ApiUtil.set_async_reply_cb(id, cb);
// if push callback, start a thread to do it
if (ApiUtil.get_asynch_cb_sub_model() == PUSH_CALLBACK) {
final AsyncCallObject aco = ApiUtil.get_async_object(id);
new CallbackThread(aco).start();
}
}
// ==========================================================================
/**
* Asynchronous command_inout using callback for reply.
*
* @param cmdname Command name.
* @param cb a CallBack object instance.
*/
// ==========================================================================
public void command_inout_asynch(final DeviceProxy deviceProxy, final String cmdname,
final CallBack cb) throws DevFailed {
command_inout_asynch(deviceProxy, cmdname, new DeviceData(), cb);
}
// ==========================================================================
/**
* Read Asynchronous command_inout reply.
*
* @param id asynchronous call id (returned by command_inout_asynch).
* @param timeout number of millisonds to wait reply before throw an excption.
*/
// ==========================================================================
public DeviceData command_inout_reply(final DeviceProxy deviceProxy,
final int id, final int timeout) throws DevFailed {
return command_inout_reply(deviceProxy, ApiUtil.get_async_object(id), timeout);
}
// ==========================================================================
/**
* Read Asynchronous command_inout reply.
*
* @param aco asynchronous call Request instance
* @param timeout number of milliseconds to wait reply before throw an exception.
*/
// ==========================================================================
public DeviceData command_inout_reply(final DeviceProxy deviceProxy,
final AsyncCallObject aco, final int timeout) throws DevFailed {
DeviceData argout = null;
final int ms_to_sleep = 10;
DevFailed except = null;
final long t0 = System.currentTimeMillis();
long t1 = t0;
while ((t1 - t0 < timeout || timeout == 0) && argout == null) {
try {
argout = command_inout_reply(deviceProxy, aco);
}
catch (final AsynReplyNotArrived na) {
except = na;
// Wait a bit before retry
try { Thread.sleep(ms_to_sleep); } catch (final InterruptedException e) { /* */ }
t1 = System.currentTimeMillis();
}
}
// If reply not arrived throw last exception
if (argout == null) {
ApiUtil.remove_async_request(aco.id);
throw except;
}
return argout;
}
// ==========================================================================
/**
* Read Asynchronous command_inout reply.
*
* @param id asynchronous call id (returned by command_inout_asynch).
*/
// ==========================================================================
public DeviceData command_inout_reply(final DeviceProxy deviceProxy, final int id)
throws DevFailed {
return command_inout_reply(deviceProxy, ApiUtil.get_async_object(id));
}
// ==========================================================================
/**
* Read Asynchronous command_inout reply.
*
* @param aco asynchronous call Request instance
*/
// ==========================================================================
public DeviceData command_inout_reply(final DeviceProxy deviceProxy,
final AsyncCallObject aco) throws DevFailed {
DeviceData data;
try {
if (deviceProxy.device_5 != null) {
check_asynch_reply(deviceProxy, aco.request, aco.id, "command_inout_4");
}
else if (deviceProxy.device_4 != null) {
check_asynch_reply(deviceProxy, aco.request, aco.id, "command_inout_4");
}
else if (deviceProxy.device_2 != null) {
check_asynch_reply(deviceProxy, aco.request, aco.id, "command_inout_2");
}
else {
check_asynch_reply(deviceProxy, aco.request, aco.id, "command_inout");
}
// If no exception, extract the any from return value,
final Any any = aco.request.return_value().extract_any();
// And put it in a DeviceData object
data = new DeviceData();
data.insert(any);
}
catch (ConnectionFailed e) {
try {
// If failed, retrieve data from request object
final NVList args = aco.request.arguments();
String command = args.item(0).value().extract_string(); // get command name
DeviceData argin = null;
if (args.count()>1) {
Any any = args.item(1).value().extract_any();
argin = new DeviceData(any);
}
//System.err.println(e.errors[0].desc);
// And do the synchronous command
if (argin==null)
data = deviceProxy.command_inout(command);
else
data = deviceProxy.command_inout(command, argin);
} catch (org.omg.CORBA.Bounds e1) {
System.err.println(e1);
throw e;
}
}
ApiUtil.remove_async_request(aco.id);
return data;
}
// ==========================================================================
/**
* add and set arguments to request for asynchronous attributes.
*/
// ==========================================================================
private void setRequestArgsForReadAttr(final Request request, final String[] names,
final DevSource src, final ClntIdent ident, final TypeCode return_type) {
Any any;
any = request.add_in_arg();
DevVarStringArrayHelper.insert(any, names);
// Add source if any
if (src != null) {
any = request.add_in_arg();
DevSourceHelper.insert(any, src);
}
if (ident != null) {
any = request.add_in_arg();
ClntIdentHelper.insert(any, ident);
}
request.set_return_type(return_type);
request.exceptions().add(DevFailedHelper.type());
}
// ==========================================================================
/**
* Asynchronous read_attribute.
*
* @param attname Attribute name.
*/
// ==========================================================================
public int read_attribute_asynch(final DeviceProxy deviceProxy, final String attname)
throws DevFailed {
final String[] attnames = new String[1];
attnames[0] = attname;
return read_attribute_asynch(deviceProxy, attnames);
}
// ==========================================================================
/**
* Asynchronous read_attribute.
*
* @param attnames Attribute names.
*/
// ==========================================================================
public int read_attribute_asynch(final DeviceProxy deviceProxy, final String[] attnames)
throws DevFailed {
checkIfTango(deviceProxy, "read_attributes_asynch");
build_connection(deviceProxy);
// Create the request object
// ----------------------------------
Request request;
if (deviceProxy.device_5 != null) {
request = deviceProxy.device_5._request("read_attributes_5");
setRequestArgsForReadAttr(request, attnames, get_source(deviceProxy),
DevLockManager.getInstance().getClntIdent(), AttributeValueList_5Helper.type());
request.exceptions().add(MultiDevFailedHelper.type());
}
else if (deviceProxy.device_4 != null) {
request = deviceProxy.device_4._request("read_attributes_4");
setRequestArgsForReadAttr(request, attnames, get_source(deviceProxy),
DevLockManager.getInstance().getClntIdent(), AttributeValueList_4Helper.type());
request.exceptions().add(MultiDevFailedHelper.type());
}
else if (deviceProxy.device_3 != null) {
request = deviceProxy.device_3._request("read_attributes_3");
setRequestArgsForReadAttr(request, attnames, get_source(deviceProxy), null,
AttributeValueList_3Helper.type());
}
else if (deviceProxy.device_2 != null) {
request = deviceProxy.device_2._request("read_attributes_2");
setRequestArgsForReadAttr(request, attnames, get_source(deviceProxy), null,
AttributeValueListHelper.type());
}
else {
request = deviceProxy.device._request("read_attributes");
setRequestArgsForReadAttr(request, attnames, null, null,
AttributeValueListHelper.type());
}
// send it (defered or just one way)
request.send_deferred();
// store request reference to read reply later
return ApiUtil.put_async_request(
new AsyncCallObject(request, deviceProxy, ATT_R, attnames));
}
// ==========================================================================
/**
* Retrieve the command/attribute arguments to build exception description.
*/
// ==========================================================================
public String get_asynch_idl_cmd(final DeviceProxy deviceProxy, final Request request,
final String idl_cmd) {
final NVList args = request.arguments();
final StringBuilder sb = new StringBuilder();
try {
if (idl_cmd.equals("command_inout")) {
return args.item(0).value().extract_string(); // get command name
} else {
// read_attribute, get attribute names
final String[] s_array = DevVarStringArrayHelper.extract(args.item(0).value());
for (int i = 0; i < s_array.length; i++) {
sb.append(s_array[i]);
if (i < s_array.length - 1) {
sb.append(", ");
}
}
}
} catch (final org.omg.CORBA.Bounds e) {
return "";
} catch (final Exception e) {
return "";
}
return sb.toString();
}
// ==========================================================================
/**
* Check Asynchronous call reply.
*
* @param id asynchronous call id (returned by read_attribute_asynch).
*/
// ==========================================================================
public void check_asynch_reply(final DeviceProxy deviceProxy, final Request request,
final int id, final String idl_cmd) throws DevFailed {
// Check if request object has been found
if (request == null) {
Except.throw_connection_failed("TangoApi_CommandFailed",
"Asynchronous call id not found", deviceProxy.getFull_class_name() + "."
+ idl_cmd + "_reply()");
} else {
if (!request.operation().equals(idl_cmd)) {
Except.throw_connection_failed("TangoApi_CommandFailed",
"Asynchronous call id not for " + idl_cmd, deviceProxy.getFull_class_name()
+ "." + idl_cmd + "_reply()");
}
// Reply arrived ? Throw exception if not yet arrived
if (!request.poll_response()) {
Except.throw_asyn_reply_not_arrived("API_AsynReplyNotArrived", "Device "
+ deviceProxy.devname + ": reply for asynchronous call (id = " + id
+ ") is not yet arrived", deviceProxy.getFull_class_name() + "." + idl_cmd
+ "_reply()");
} else {
// Check if an exception has been thrown
final Exception except = request.env().exception();
if (except != null) {
ApiUtil.remove_async_request(id);
if (except instanceof org.omg.CORBA.TRANSIENT) {
throw_dev_failed(deviceProxy, except,
deviceProxy.getFull_class_name()
+ "." + idl_cmd + "_reply("
+ get_asynch_idl_cmd(deviceProxy, request, idl_cmd) + ")", false);
}
else if (except instanceof org.omg.CORBA.TIMEOUT) {
throw_dev_failed(deviceProxy, except,
deviceProxy.getFull_class_name()
+ "." + idl_cmd + "_reply("
+ get_asynch_idl_cmd(deviceProxy, request, idl_cmd) + ")", false);
}
else
// Check if user exception (DevFailed).
if (except instanceof org.omg.CORBA.UnknownUserException) {
final Any any = ((org.omg.CORBA.UnknownUserException) except).except;
MultiDevFailed ex = null;
try {
//noinspection ThrowableResultOfMethodCallIgnored
ex = MultiDevFailedHelper.extract(any);
} catch (final Exception e) {
// not a MultiDevFailed, is a DevFailed
final DevFailed df = DevFailedHelper.extract(any);
Except.throw_connection_failed(df, "TangoApi_CommandFailed",
"Asynchronous command failed", deviceProxy.getFull_class_name()
+ "." + idl_cmd + "_reply("
+ get_asynch_idl_cmd(deviceProxy, request, idl_cmd)
+ ")");
}
if (ex!=null)
Except.throw_connection_failed(new DevFailed(
ex.errors[0].err_list),
"TangoApi_CommandFailed", "Asynchronous command failed",
deviceProxy.getFull_class_name() + "." + idl_cmd + "_reply("
+ get_asynch_idl_cmd(deviceProxy, request, idl_cmd) + ")");
} else {
except.printStackTrace();
// Another exception -> re-throw it as a DevFailed
System.err.println(deviceProxy.getFull_class_name() + "." + idl_cmd
+ "_reply(" + get_asynch_idl_cmd(deviceProxy, request, idl_cmd)
+ ")");
throw_dev_failed(deviceProxy, except, deviceProxy.getFull_class_name()
+ "." + idl_cmd + "_reply("
+ get_asynch_idl_cmd(deviceProxy, request, idl_cmd) + ")", false);
}
}
}
}
}
// ==========================================================================
/**
* Read Asynchronous read_attribute reply.
*
* @param id asynchronous call id (returned by read_attribute_asynch).
* @param timeout number of milliseconds to wait reply before throw an excption.
*/
// ==========================================================================
public DeviceAttribute[] read_attribute_reply(final DeviceProxy deviceProxy,
final int id, final int timeout) throws DevFailed {
DeviceAttribute[] argout = null;
final int ms_to_sleep = 10;
AsynReplyNotArrived except = null;
final long t0 = System.currentTimeMillis();
long t1 = t0;
while ((t1 - t0 < timeout || timeout == 0) && argout == null) {
try {
argout = read_attribute_reply(deviceProxy, id);
}
catch (final AsynReplyNotArrived na) {
except = na;
// Wait a bit before retry
try { Thread.sleep(ms_to_sleep); } catch (final InterruptedException e) { /* */ }
t1 = System.currentTimeMillis();
}
}
// If reply not arrived throw last exception
if (argout == null) {
ApiUtil.remove_async_request(id);
throw except;
}
return argout;
}
// ==========================================================================
/**
* Read Asynchronous read_attribute reply.
*
* @param id asynchronous call id (returned by read_attribute_asynch).
*/
// ==========================================================================
public DeviceAttribute[] read_attribute_reply(final DeviceProxy deviceProxy,
final int id) throws DevFailed {
DeviceAttribute[] data = new DeviceAttribute[0];
AttributeValue[] attributeValues;
AttributeValue_3[] attributeValues_3;
AttributeValue_4[] attributeValues_4;
AttributeValue_5[] attributeValues_5;
final Request request = ApiUtil.get_async_request(id);
// If no exception, extract the any from return value,
final Any any = request.return_value();
try {
if (deviceProxy.device_5 != null) {
check_asynch_reply(deviceProxy, request, id, "read_attributes_5");
attributeValues_5 = AttributeValueList_5Helper.extract(any);
data = new DeviceAttribute[attributeValues_5.length];
for (int i = 0; i < attributeValues_5.length; i++) {
data[i] = new DeviceAttribute(attributeValues_5[i]);
}
}
else if (deviceProxy.device_4 != null) {
check_asynch_reply(deviceProxy, request, id, "read_attributes_4");
attributeValues_4 = AttributeValueList_4Helper.extract(any);
data = new DeviceAttribute[attributeValues_4.length];
for (int i = 0; i < attributeValues_4.length; i++) {
data[i] = new DeviceAttribute(attributeValues_4[i]);
}
}
else if (deviceProxy.device_3 != null) {
check_asynch_reply(deviceProxy, request, id, "read_attributes_3");
attributeValues_3 = AttributeValueList_3Helper.extract(any);
data = new DeviceAttribute[attributeValues_3.length];
for (int i = 0; i < attributeValues_3.length; i++) {
data[i] = new DeviceAttribute(attributeValues_3[i]);
}
}
else if (deviceProxy.device_2 != null) {
check_asynch_reply(deviceProxy, request, id, "read_attributes_2");
attributeValues = AttributeValueListHelper.extract(any);
data = new DeviceAttribute[attributeValues.length];
for (int i = 0; i < attributeValues.length; i++) {
data[i] = new DeviceAttribute(attributeValues[i]);
}
}
else {
check_asynch_reply(deviceProxy, request, id, "read_attributes");
attributeValues = AttributeValueListHelper.extract(any);
data = new DeviceAttribute[attributeValues.length];
for (int i = 0; i < attributeValues.length; i++) {
data[i] = new DeviceAttribute(attributeValues[i]);
}
}
}
catch (Exception e) {
if (e instanceof AsynReplyNotArrived)
throw (AsynReplyNotArrived) e;
try {
// If failed, retrieve data from request object
final NVList args = request.arguments();
final String[] attributeNames =
DevVarStringArrayHelper.extract(args.item(0).value());
// And do the synchronous read_attributes
data = deviceProxy.read_attribute(attributeNames);
} catch (org.omg.CORBA.Bounds e1) {
System.err.println(e1);
if (e instanceof DevFailed)
throw (DevFailed)e;
else
Except.throw_exception(e.toString(), e.toString());
}
}
ApiUtil.remove_async_request(id);
return data;
}
// ==========================================================================
/**
* Asynchronous read_attribute using callback for reply.
*
* @param attname attribute name.
* @param cb a CallBack object instance.
*/
// ==========================================================================
public void read_attribute_asynch(final DeviceProxy deviceProxy, final String attname,
final CallBack cb) throws DevFailed {
final String[] attnames = new String[1];
attnames[0] = attname;
read_attribute_asynch(deviceProxy, attnames, cb);
}
// ==========================================================================
/**
* Asynchronous read_attribute using callback for reply.
*
* @param attnames attribute names.
* @param cb a CallBack object instance.
*/
// ==========================================================================
public void read_attribute_asynch(final DeviceProxy deviceProxy, final String[] attnames,
final CallBack cb) throws DevFailed {
final int id = read_attribute_asynch(deviceProxy, attnames);
ApiUtil.set_async_reply_model(id, CALLBACK);
ApiUtil.set_async_reply_cb(id, cb);
// if push callback, start a thread to do it
if (ApiUtil.get_asynch_cb_sub_model() == PUSH_CALLBACK) {
final AsyncCallObject aco = ApiUtil.get_async_object(id);
new CallbackThread(aco).start();
}
}
// ==========================================================================
/**
* Asynchronous write_attribute.
*
* @param attr Attribute value (name, writing value...)
*/
// ==========================================================================
public int write_attribute_asynch(final DeviceProxy deviceProxy, final DeviceAttribute attr)
throws DevFailed {
return write_attribute_asynch(deviceProxy, attr, false);
}
// ==========================================================================
/**
* Asynchronous write_attribute.
*
* @param attr Attribute value (name, writing value...)
* @param forget forget the response if true
*/
// ==========================================================================
public int write_attribute_asynch(final DeviceProxy deviceProxy, final DeviceAttribute attr,
final boolean forget) throws DevFailed {
final DeviceAttribute[] attribs = new DeviceAttribute[1];
attribs[0] = attr;
return write_attribute_asynch(deviceProxy, attribs);
}
// ==========================================================================
/**
* Asynchronous write_attribute.
*
* @param attribs Attribute values (name, writing value...)
*/
// ==========================================================================
public int write_attribute_asynch(final DeviceProxy deviceProxy, final DeviceAttribute[] attribs)
throws DevFailed {
return write_attribute_asynch(deviceProxy, attribs, false);
}
// ==========================================================================
/**
* Asynchronous write_attribute.
*
* @param attribs Attribute values (name, writing value...)
* @param forget forget the response if true
*/
// ==========================================================================
public int write_attribute_asynch(final DeviceProxy deviceProxy,
final DeviceAttribute[] attribs, final boolean forget) throws DevFailed {
build_connection(deviceProxy);
//
// Manage Access control
//
if (deviceProxy.access == TangoConst.ACCESS_READ) {
// pind the device to throw execption
// if failed (for reconnection)
ping(deviceProxy);
throwNotAuthorizedException(deviceProxy.devname + ".write_attribute_asynch()",
"DeviceProxy.write_attribute_asynch()");
}
// Build idl argin object
Request request;
final String[] attributeNames = new String[attribs.length];
Any any;
if (deviceProxy.device_5 != null || deviceProxy.device_4 != null) {
final AttributeValue_4[] attributeValues_4 = new AttributeValue_4[attribs.length];
for (int i=0; i=4) {
AttributeValue_4[] attributeValues_4 =
AttributeValueList_4Helper.extract(any);
deviceAttributes = new DeviceAttribute[attributeValues_4.length];
for (int i=0 ; i=3) {
AttributeValue[] attributeValues =
AttributeValueListHelper.extract(any);
deviceAttributes = new DeviceAttribute[attributeValues.length];
for (int i=0 ; iDevice name.
* Class name Device type Device server name Host name
*/
// ==========================================================================
public String[] dev_inform(final DeviceProxy deviceProxy) throws DevFailed {
checkIfTaco(deviceProxy, "dev_inform");
if (deviceProxy.taco_device == null && deviceProxy.devname != null) {
build_connection(deviceProxy);
}
return deviceProxy.taco_device.dev_inform();
}
// ==========================================================================
/**
* Execute the dev_rpc_protocol TACO command to change RPC protocol mode.
*
* @param mode RPC protocol mode to be seted
* (TangoApi.TacoDevice.D_TCP or
* TangoApi.TacoDevice.D_UDP).
*/
// ==========================================================================
public void set_rpc_protocol(final DeviceProxy deviceProxy, final int mode) throws DevFailed {
checkIfTaco(deviceProxy, "dev_rpc_protocol");
build_connection(deviceProxy);
deviceProxy.taco_device.set_rpc_protocol(mode);
}
// ==========================================================================
/**
* @return mode RPC protocol mode used (TangoApi.TacoDevice.D_TCP or
* TangoApi.TacoDevice.D_UDP).
*/
// ==========================================================================
public int get_rpc_protocol(final DeviceProxy deviceProxy) throws DevFailed {
checkIfTaco(deviceProxy, "get_rpc_protocol");
build_connection(deviceProxy);
return deviceProxy.taco_device.get_rpc_protocol();
}
// ===================================================================
/*
* ToDo Pipe related methods
*/
// ===================================================================
// ===================================================================
/**
* Query device for pipe configuration list
* @return pipe configuration list
* @throws DevFailed if device connection failed
*/
// ===================================================================
public List getPipeConfig(DeviceProxy deviceProxy) throws DevFailed {
build_connection(deviceProxy);
if (deviceProxy.idl_version<5)
Except.throw_exception("TangoApi_NOT_SUPPORTED",
"Pipe not supported in IDL " + deviceProxy.idl_version);
ArrayList infoList = new ArrayList();
boolean done = false;
final int retries = deviceProxy.transparent_reconnection ? 2 : 1;
for (int tr=0; tr getPipeConfig(DeviceProxy deviceProxy, List pipeNames) throws DevFailed {
build_connection(deviceProxy);
if (deviceProxy.idl_version<5)
Except.throw_exception("TangoApi_NOT_SUPPORTED",
"Pipe not supported in IDL " + deviceProxy.idl_version);
ArrayList infoList = new ArrayList();
boolean done = false;
final int retries = deviceProxy.transparent_reconnection ? 2 : 1;
for (int tr=0 ; tr pipeInfoList) throws DevFailed {
build_connection(deviceProxy);
if (deviceProxy.idl_version<5)
Except.throw_exception("TangoApi_NOT_SUPPORTED",
"Pipe not supported in IDL " + deviceProxy.idl_version);
boolean done = false;
final int retries = deviceProxy.transparent_reconnection ? 2 : 1;
for (int tr=0 ; tr
© 2015 - 2025 Weber Informatics LLC | Privacy Policy