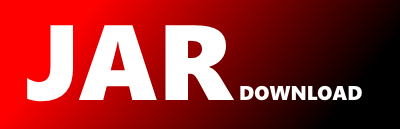
fr.esrf.TangoApi.DatabaseDAODefaultImpl Maven / Gradle / Ivy
//+======================================================================
// $Source$
//
// Project: Tango
//
// Description: java source code for the TANGO client/server API.
//
// $Author: pascal_verdier $
//
// Copyright (C) : 2004,2005,2006,2007,2008,2009,2010,2011,2012,2013,2014,
// European Synchrotron Radiation Facility
// BP 220, Grenoble 38043
// FRANCE
//
// This file is part of Tango.
//
// Tango is free software: you can redistribute it and/or modify
// it under the terms of the GNU Lesser General Public License as published by
// the Free Software Foundation, either version 3 of the License, or
// (at your option) any later version.
//
// Tango is distributed in the hope that it will be useful,
// but WITHOUT ANY WARRANTY; without even the implied warranty of
// MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
// GNU Lesser General Public License for more details.
//
// You should have received a copy of the GNU Lesser General Public License
// along with Tango. If not, see .
//
// $Revision: 30265 $
//
//-======================================================================
package fr.esrf.TangoApi;
import fr.esrf.Tango.DevFailed;
import fr.esrf.Tango.DevVarLongStringArray;
import fr.esrf.TangoApi.events.DbEventImportInfo;
import fr.esrf.TangoDs.Except;
import fr.esrf.TangoDs.TangoConst;
import java.util.*;
/**
* Class Description:
* This class is the main class for TANGO database API.
* The TANGO database is implemented as a TANGO device server.
* To access it, the user has the CORBA interface command_inout().
* This expects and returns all parameters as ascii strings thereby making
* the database laborious to use for retreing device properties and information.
* In order to simplify this access, a high-level API has been implemented
* which hides the low-level formatting necessary to convert the
* command_inout() return values into binary values and all CORBA aspects
* of the TANGO.
* All data types are native java types e.g. simple types an arrays.
*
* @author verdier
* @version $Revision: 30265 $
*/
public class DatabaseDAODefaultImpl extends ConnectionDAODefaultImpl implements IDatabaseDAO {
//===================================================================
/**
* Database access constructor.
*/
//===================================================================
public DatabaseDAODefaultImpl() {
super();
}
//===================================================================
/**
* Database access init method.
*
* @throws DevFailed in case of environment not corectly set.
*/
//===================================================================
public void init(Database database) throws DevFailed {
super.init(database);
}
//===================================================================
/**
* Database access constructor.
*
* @throws DevFailed in case of host or port not available
* @param host host where database is running.
* @param port port for database connection.
*/
//===================================================================
public void init(Database database, String host, String port) throws DevFailed {
super.init(database, host, port);
}
//==========================================================================
//==========================================================================
/* (non-Javadoc)
* @see fr.esrf.TangoApi.IDatabaseDAO#toString()
*/
public String toString(Database database) {
return database.url.host + ":" + database.url.port;
}
//==========================================================================
/**
* Convert a String array to a sting.
*
* @param array String array to be converted
* @return string after conversion.
*/
//==========================================================================
private String stringArray2String(String[] array) {
StringBuilder sb = new StringBuilder("");
for (int i = 0 ; i " +
// ((database.access==TangoConst.ACCESS_READ)? "Read" : "Write"));
}
}
//**************************************
// MISCELLANEOUS MANAGEMENT
//**************************************
//==========================================================================
/* (non-Javadoc)
* @see fr.esrf.TangoApi.IDatabaseDAO#get_info()
*/
//==========================================================================
public String get_info(Database database) throws DevFailed {
if (!database.isAccess_checked()) checkAccess(database);
// Query info from database
DeviceData argout = command_inout(database, "DbInfo");
String[] info = argout.extractStringArray();
// format result as string
return stringArray2String(info);
}
//==========================================================================
/* (non-Javadoc)
* @see fr.esrf.TangoApi.IDatabaseDAO#get_host_list()
*/
//==========================================================================
public String[] get_host_list(Database database) throws DevFailed {
if (!database.isAccess_checked()) checkAccess(database);
// Query info from database
DeviceData argin = new DeviceData();
argin.insert("*");
DeviceData argout = command_inout(database, "DbGetHostList", argin);
return argout.extractStringArray();
}
//==========================================================================
/* (non-Javadoc)
* @see fr.esrf.TangoApi.IDatabaseDAO#get_host_list(java.lang.String)
*/
//==========================================================================
public String[] get_host_list(Database database, String wildcard) throws DevFailed {
if (!database.isAccess_checked()) checkAccess(database);
// Query info from database
DeviceData argin = new DeviceData();
argin.insert(wildcard);
DeviceData argout = command_inout(database, "DbGetHostList", argin);
return argout.extractStringArray();
}
//**************************************
// SERVERS MANAGEMENT
//**************************************
//==========================================================================
/* (non-Javadoc)
* @see fr.esrf.TangoApi.IDatabaseDAO#get_server_class_list(java.lang.String)
*/
//==========================================================================
public String[] get_server_class_list(Database database, String servname) throws DevFailed {
if (!database.isAccess_checked()) checkAccess(database);
DeviceData argin = new DeviceData();
argin.insert(servname);
DeviceData argout = command_inout(database, "DbGetDeviceServerClassList", argin);
String[] list = argout.extractStringArray();
// Extract DServer class
int nb_classes;
if (list.length==0)
nb_classes = 0;
else
nb_classes = list.length - 1;
String[] classes = new String[nb_classes];
for (int i = 0, j = 0 ; i0)
properties[pnum++] = new DbDatum(strprop[i],
strprop, start_val, end_val);
else {
// no property --> fields do not exist
properties[pnum++] = new DbDatum(strprop[i]);
// If nb property is zero there is a property
// set to space char (!!!)
// if Object is device it is true but false for a class !!!
//----------------------------------------------------------
if (start_val + 1 " + array[i]);
System.out.println("cmd -> " + cmd);
*********/
// Send it to Database
//------------------------------
DeviceData argin = new DeviceData();
argin.insert(array);
command_inout(database, cmd, argin);
}
//==========================================================================
/* (non-Javadoc)
* @see fr.esrf.TangoApi.IDatabaseDAO#get_object_list(java.lang.String)
*/
//==========================================================================
public String[] get_object_list(Database database, String wildcard)
throws DevFailed {
if (!database.isAccess_checked()) checkAccess(database);
DeviceData argin = new DeviceData();
argin.insert(wildcard);
DeviceData argout = command_inout(database, "DbGetObjectList", argin);
return argout.extractStringArray();
}
//==========================================================================
/* (non-Javadoc)
* @see fr.esrf.TangoApi.IDatabaseDAO#get_object_property_list(java.lang.String, java.lang.String)
*/
//==========================================================================
public String[] get_object_property_list(Database database, String objname, String wildcard)
throws DevFailed {
if (!database.isAccess_checked()) checkAccess(database);
String[] array = new String[2];
array[0] = objname;
array[1] = wildcard;
DeviceData argin = new DeviceData();
argin.insert(array);
DeviceData argout = command_inout(database, "DbGetPropertyList", argin);
return argout.extractStringArray();
}
//==========================================================================
/* (non-Javadoc)
* @see fr.esrf.TangoApi.IDatabaseDAO#get_property(java.lang.String, java.lang.String[])
*/
//==========================================================================
public DbDatum[] get_property(Database database, String name, String[] propnames)
throws DevFailed {
String type = ""; // No object type
return get_obj_property(database, name, type, propnames);
}
//==========================================================================
/* (non-Javadoc)
* @see fr.esrf.TangoApi.IDatabaseDAO#get_property(java.lang.String, java.lang.String)
*/
//==========================================================================
public DbDatum get_property(Database database, String name, String propname)
throws DevFailed {
String type = ""; // No object type
return get_obj_property(database, name, type, propname);
}
//==========================================================================
/**
* Query the database for an object (ie non-device)
* property for the pecified object without access check (initilizing phase).
*
* @param name Object name.
* @param propname list of property names.
* @return property in DbDatum object.
*/
//==========================================================================
public DbDatum get_property(Database database, String name, String propname, boolean forced)
throws DevFailed {
int tmp_access = database.access;
if (forced)
database.access = TangoConst.ACCESS_WRITE;
DbDatum datum;
try {
// Format input parameters as string array
//--------------------------------------------
String[] array;
array = new String[2];
array[0] = name;
array[1] = propname;
// Read Database
//---------------------
DeviceData argin = new DeviceData();
argin.insert(array);
DeviceData argout = command_inout(database, "DbGetProperty", argin);
String[] result = argout.extractStringArray();
// And convert to DbDatum array before returning
datum = stringArray2DbDatum(result)[0];
database.access = tmp_access;
} catch (DevFailed e) {
database.access = tmp_access;
throw e;
}
return datum;
}
//==========================================================================
/* (non-Javadoc)
* @see fr.esrf.TangoApi.IDatabaseDAO#get_property(java.lang.String, fr.esrf.TangoApi.DbDatum[])
*/
//==========================================================================
public DbDatum[] get_property(Database database, String name, DbDatum[] properties)
throws DevFailed {
String type = ""; // No object type
return get_obj_property(database, name, type, properties);
}
//==========================================================================
/* (non-Javadoc)
* @see fr.esrf.TangoApi.IDatabaseDAO#put_property(java.lang.String, fr.esrf.TangoApi.DbDatum[])
*/
//==========================================================================
public void put_property(Database database, String name, DbDatum[] properties)
throws DevFailed {
if (!database.isAccess_checked()) checkAccess(database);
String[] array = dbdatum2StringArray(name, properties);
DeviceData argin = new DeviceData();
argin.insert(array);
command_inout(database, "DbPutProperty", argin);
}
//==========================================================================
/* (non-Javadoc)
* @see fr.esrf.TangoApi.IDatabaseDAO#delete_property(java.lang.String, java.lang.String[])
*/
//==========================================================================
public void delete_property(Database database, String name, String[] propnames)
throws DevFailed {
String type = "";
delete_obj_property(database, name, type, propnames);
}
//==========================================================================
/* (non-Javadoc)
* @see fr.esrf.TangoApi.IDatabaseDAO#delete_property(java.lang.String, java.lang.String)
*/
//==========================================================================
public void delete_property(Database database, String name, String propname)
throws DevFailed {
String type = "";
delete_obj_property(database, name, type, propname);
}
//==========================================================================
/* (non-Javadoc)
* @see fr.esrf.TangoApi.IDatabaseDAO#delete_property(java.lang.String, fr.esrf.TangoApi.DbDatum[])
*/
//==========================================================================
public void delete_property(Database database, String name, DbDatum[] properties)
throws DevFailed {
String type = "";
delete_obj_property(database, name, type, properties);
}
//==========================================================================
/* (non-Javadoc)
* @see fr.esrf.TangoApi.IDatabaseDAO#get_class_property_list(java.lang.String, java.lang.String)
*/
//==========================================================================
public String[] get_class_property_list(Database database, String classname, String wildcard)
throws DevFailed {
if (!database.isAccess_checked()) checkAccess(database);
DeviceData argin = new DeviceData();
argin.insert(classname);
DeviceData argout = command_inout(database, "DbGetClassPropertyList", argin);
return argout.extractStringArray();
}
//==========================================================================
/* (non-Javadoc)
* @see fr.esrf.TangoApi.IDatabaseDAO#get_device_property_list(java.lang.String, java.lang.String)
*/
//==========================================================================
public String[] get_device_property_list(Database database, String devname, String wildcard)
throws DevFailed {
if (!database.isAccess_checked()) checkAccess(database);
String[] array = new String[2];
array[0] = devname;
array[1] = wildcard;
DeviceData argin = new DeviceData();
argin.insert(array);
DeviceData argout = command_inout(database, "DbGetDevicePropertyList", argin);
return argout.extractStringArray();
}
//==========================================================================
//==========================================================================
/* (non-Javadoc)
* @see fr.esrf.TangoApi.IDatabaseDAO#get_class_for_device(java.lang.String)
*/
public String get_class_for_device(Database database, String devname)
throws DevFailed {
if (!database.isAccess_checked()) checkAccess(database);
DeviceData argin = new DeviceData();
argin.insert(devname);
DeviceData argout = command_inout(database, "DbGetClassForDevice", argin);
return argout.extractString();
}
//==========================================================================
//==========================================================================
/* (non-Javadoc)
* @see fr.esrf.TangoApi.IDatabaseDAO#get_class_inheritance_for_device(java.lang.String)
*/
public String[] get_class_inheritance_for_device(Database database, String devname)
throws DevFailed {
if (!database.isAccess_checked()) checkAccess(database);
String[] result = {"Device_3Impl"};
try {
DeviceData argin = new DeviceData();
argin.insert(devname);
DeviceData argout = command_inout(database, "DbGetClassInheritanceForDevice", argin);
result = argout.extractStringArray();
} catch (DevFailed e) {
// Check if an old API else re-throw
if (!e.errors[0].reason.equals("API_CommandNotFound"))
throw e;
}
return result;
}
//**************************************
// DEVICE PROPERTIES MANAGEMENT
//**************************************
//==========================================================================
/* (non-Javadoc)
* @see fr.esrf.TangoApi.IDatabaseDAO#get_device_property(java.lang.String, java.lang.String[])
*/
//==========================================================================
public DbDatum[] get_device_property(Database database, String name, String[] propnames)
throws DevFailed {
String type = "Device"; // Device object type
return get_obj_property(database, name, type, propnames);
}
//==========================================================================
/* (non-Javadoc)
* @see fr.esrf.TangoApi.IDatabaseDAO#get_device_property(java.lang.String, java.lang.String)
*/
//==========================================================================
public DbDatum get_device_property(Database database, String name, String propname)
throws DevFailed {
String type = "Device"; // Device object type
return get_obj_property(database, name, type, propname);
}
//==========================================================================
/* (non-Javadoc)
* @see fr.esrf.TangoApi.IDatabaseDAO#get_device_property(java.lang.String, fr.esrf.TangoApi.DbDatum[])
*/
//==========================================================================
public DbDatum[] get_device_property(Database database, String name, DbDatum[] properties)
throws DevFailed {
String type = "Device"; // Device object type
return get_obj_property(database, name, type, properties);
}
//==========================================================================
/* (non-Javadoc)
* @see fr.esrf.TangoApi.IDatabaseDAO#put_device_property(java.lang.String, fr.esrf.TangoApi.DbDatum[])
*/
//==========================================================================
public void put_device_property(Database database, String name, DbDatum[] properties)
throws DevFailed {
if (!database.isAccess_checked()) checkAccess(database);
String[] array = dbdatum2StringArray(name, properties);
DeviceData argin = new DeviceData();
argin.insert(array);
command_inout(database, "DbPutDeviceProperty", argin);
}
//==========================================================================
/* (non-Javadoc)
* @see fr.esrf.TangoApi.IDatabaseDAO#delete_device_property(java.lang.String, java.lang.String[])
*/
//==========================================================================
public void delete_device_property(Database database, String name, String[] propnames)
throws DevFailed {
String type = "Device";
delete_obj_property(database, name, type, propnames);
}
//==========================================================================
/* (non-Javadoc)
* @see fr.esrf.TangoApi.IDatabaseDAO#delete_device_property(java.lang.String, java.lang.String)
*/
//==========================================================================
public void delete_device_property(Database database, String name, String propname)
throws DevFailed {
String type = "Device";
delete_obj_property(database, name, type, propname);
}
//==========================================================================
/* (non-Javadoc)
* @see fr.esrf.TangoApi.IDatabaseDAO#delete_device_property(java.lang.String, fr.esrf.TangoApi.DbDatum[])
*/
//==========================================================================
public void delete_device_property(Database database, String name, DbDatum[] properties)
throws DevFailed {
String type = "Device";
delete_obj_property(database, name, type, properties);
}
//**************************************
// ATTRIBUTE PROPERTIES MANAGEMENT
//**************************************
//==========================================================================
/* (non-Javadoc)
* @see fr.esrf.TangoApi.IDatabaseDAO#get_device_attribute_list(java.lang.String)
*/
//==========================================================================
public String[] get_device_attribute_list(Database database, String devname)
throws DevFailed {
if (!database.isAccess_checked()) checkAccess(database);
DeviceData argin = new DeviceData();
argin.insert(new String[]{devname, "*"});
DeviceData argout = command_inout(database, "DbGetDeviceAttributeList", argin);
return argout.extractStringArray();
}
//==========================================================================
/* (non-Javadoc)
* @see fr.esrf.TangoApi.IDatabaseDAO#get_device_attribute_property(java.lang.String, java.lang.String[])
*/
//==========================================================================
public DbAttribute[] get_device_attribute_property(Database database, String devname, String[] attnames)
throws DevFailed {
if (!database.isAccess_checked()) checkAccess(database);
DeviceData argin = new DeviceData();
DeviceData argout;
int mode = 2;
try {
// value is an array
argin.insert(ApiUtil.toStringArray(devname, attnames));
argout = command_inout(database, "DbGetDeviceAttributeProperty2", argin);
} catch (DevFailed e) {
if (e.errors[0].reason.equals("API_CommandNotFound")) {
// Value is just one element
argout = command_inout(database, "DbGetDeviceAttributeProperty", argin);
mode = 1;
} else
throw e;
}
return ApiUtil.toDbAttributeArray(argout.extractStringArray(), mode);
}
//==========================================================================
/* (non-Javadoc)
* @see fr.esrf.TangoApi.IDatabaseDAO#get_device_attribute_property(java.lang.String, java.lang.String)
*/
//==========================================================================
public DbAttribute get_device_attribute_property(Database database, String devname, String attname)
throws DevFailed {
String[] attnames = new String[1];
attnames[0] = attname;
return get_device_attribute_property(database, devname, attnames)[0];
}
//==========================================================================
/* (non-Javadoc)
* @see fr.esrf.TangoApi.IDatabaseDAO#put_device_attribute_property(java.lang.String, fr.esrf.TangoApi.DbAttribute[])
*/
//==========================================================================
public void put_device_attribute_property(Database database, String devname, DbAttribute[] attr)
throws DevFailed {
if (!database.isAccess_checked()) checkAccess(database);
DeviceData argin = new DeviceData();
try {
// value is an array
argin.insert(ApiUtil.toStringArray(devname, attr, 2));
command_inout(database, "DbPutDeviceAttributeProperty2", argin);
} catch (DevFailed e) {
if (e.errors[0].reason.equals("API_CommandNotFound")) {
// Value is just one element
argin.insert(ApiUtil.toStringArray(devname, attr, 1));
command_inout(database, "DbPutDeviceAttributeProperty", argin);
} else
throw e;
}
}
//==========================================================================
/* (non-Javadoc)
* @see fr.esrf.TangoApi.IDatabaseDAO#put_device_attribute_property(java.lang.String, fr.esrf.TangoApi.DbAttribute)
*/
//==========================================================================
public void put_device_attribute_property(Database database, String devname, DbAttribute attr)
throws DevFailed {
DbAttribute[] da = new DbAttribute[1];
da[0] = attr;
put_device_attribute_property(database, devname, da);
}
//==========================================================================
/* (non-Javadoc)
* @see fr.esrf.TangoApi.IDatabaseDAO#delete_device_attribute_property(java.lang.String, fr.esrf.TangoApi.DbAttribute)
*/
//==========================================================================
public void delete_device_attribute_property(Database database, String devname, DbAttribute attr)
throws DevFailed {
delete_device_attribute_property(database, devname,
attr.name, attr.get_property_list());
}
//==========================================================================
/* (non-Javadoc)
* @see fr.esrf.TangoApi.IDatabaseDAO#delete_device_attribute_property(java.lang.String, fr.esrf.TangoApi.DbAttribute[])
*/
//==========================================================================
public void delete_device_attribute_property(Database database, String devname, DbAttribute[] attribute)
throws DevFailed {
for (DbAttribute att : attribute)
delete_device_attribute_property(database, devname,
att.name, att.get_property_list());
}
//==========================================================================
/* (non-Javadoc)
* @see fr.esrf.TangoApi.IDatabaseDAO#delete_device_attribute_property(java.lang.String, java.lang.String, java.lang.String[])
*/
//==========================================================================
public void delete_device_attribute_property(Database database, String devname, String attname, String[] propnames)
throws DevFailed {
if (propnames.length==0)
return;
if (!database.isAccess_checked()) checkAccess(database);
// Build a String array before command
String[] array = new String[2 + propnames.length];
array[0] = devname;
array[1] = attname;
System.arraycopy(propnames, 0, array, 2, propnames.length);
DeviceData argin = new DeviceData();
argin.insert(array);
command_inout(database, "DbDeleteDeviceAttributeProperty", argin);
}
//==========================================================================
/* (non-Javadoc)
* @see fr.esrf.TangoApi.IDatabaseDAO#delete_device_attribute_property(java.lang.String, java.lang.String, java.lang.String)
*/
//==========================================================================
public void delete_device_attribute_property(Database database, String devname, String attname, String propname)
throws DevFailed {
String[] array = new String[1];
array[0] = propname;
delete_device_attribute_property(database, devname, attname, array);
}
//==========================================================================
/* (non-Javadoc)
* @see fr.esrf.TangoApi.IDatabaseDAO#delete_device_attribute(java.lang.String, java.lang.String)
*/
//==========================================================================
public void delete_device_attribute(Database database, String devname, String attname) throws DevFailed {
if (!database.isAccess_checked()) checkAccess(database);
String[] array = new String[2];
array[0] = devname;
array[1] = attname;
DeviceData argin = new DeviceData();
argin.insert(array);
command_inout(database, "DbDeleteDeviceAttribute", argin);
}
//**************************************
// CLASS PROPERTIES MANAGEMENT
//**************************************
//==========================================================================
/* (non-Javadoc)
* @see fr.esrf.TangoApi.IDatabaseDAO#get_class_list(java.lang.String)
*/
//==========================================================================
public String[] get_class_list(Database database, String servname) throws DevFailed {
if (!database.isAccess_checked()) checkAccess(database);
// Query info from database
DeviceData argin = new DeviceData();
argin.insert(servname);
DeviceData argout = command_inout(database, "DbGetClassList", argin);
return argout.extractStringArray();
}
//==========================================================================
/* (non-Javadoc)
* @see fr.esrf.TangoApi.IDatabaseDAO#get_class_property(java.lang.String, java.lang.String[])
*/
//==========================================================================
public DbDatum[] get_class_property(Database database, String name, String[] propnames)
throws DevFailed {
String type = "Class"; // class object type
return get_obj_property(database, name, type, propnames);
}
//==========================================================================
/* (non-Javadoc)
* @see fr.esrf.TangoApi.IDatabaseDAO#get_class_property(java.lang.String, java.lang.String)
*/
//==========================================================================
public DbDatum get_class_property(Database database, String name, String propname)
throws DevFailed {
String type = "Class"; // class object type
return get_obj_property(database, name, type, propname);
}
//==========================================================================
/* (non-Javadoc)
* @see fr.esrf.TangoApi.IDatabaseDAO#get_class_property(java.lang.String, fr.esrf.TangoApi.DbDatum[])
*/
//==========================================================================
public DbDatum[] get_class_property(Database database, String name, DbDatum[] properties)
throws DevFailed {
String type = "Class"; // Device object type
return get_obj_property(database, name, type, properties);
}
//==========================================================================
/* (non-Javadoc)
* @see fr.esrf.TangoApi.IDatabaseDAO#put_class_property(java.lang.String, fr.esrf.TangoApi.DbDatum[])
*/
//==========================================================================
public void put_class_property(Database database, String name, DbDatum[] properties)
throws DevFailed {
if (!database.isAccess_checked()) checkAccess(database);
String[] array = dbdatum2StringArray(name, properties);
DeviceData argin = new DeviceData();
argin.insert(array);
command_inout(database, "DbPutClassProperty", argin);
}
//==========================================================================
/* (non-Javadoc)
* @see fr.esrf.TangoApi.IDatabaseDAO#delete_class_property(java.lang.String, java.lang.String[])
*/
//==========================================================================
public void delete_class_property(Database database, String name, String[] propnames)
throws DevFailed {
String type = "Class";
delete_obj_property(database, name, type, propnames);
}
//==========================================================================
/* (non-Javadoc)
* @see fr.esrf.TangoApi.IDatabaseDAO#delete_class_property(java.lang.String, java.lang.String)
*/
//==========================================================================
public void delete_class_property(Database database, String name, String propname)
throws DevFailed {
String type = "Class";
delete_obj_property(database, name, type, propname);
}
//==========================================================================
/* (non-Javadoc)
* @see fr.esrf.TangoApi.IDatabaseDAO#delete_class_property(java.lang.String, fr.esrf.TangoApi.DbDatum[])
*/
//==========================================================================
public void delete_class_property(Database database, String name, DbDatum[] properties)
throws DevFailed {
String type = "Class";
delete_obj_property(database, name, type, properties);
}
//**************************************
// CLASS Attribute PROPERTIES MANAGEMENT
//**************************************
//==========================================================================
/* (non-Javadoc)
* @see fr.esrf.TangoApi.IDatabaseDAO#get_class_attribute_list(java.lang.String, java.lang.String)
*/
//==========================================================================
public String[] get_class_attribute_list(Database database, String classname, String wildcard) throws DevFailed {
if (!database.isAccess_checked()) checkAccess(database);
DeviceData argin = new DeviceData();
argin.insert(ApiUtil.toStringArray(classname, wildcard));
DeviceData argout = command_inout(database, "DbGetClassAttributeList", argin);
return argout.extractStringArray();
}
//==========================================================================
/* (non-Javadoc)
* @see fr.esrf.TangoApi.IDatabaseDAO#get_class_attribute_property(java.lang.String, java.lang.String)
*/
//==========================================================================
public DbAttribute get_class_attribute_property(Database database, String classname, String attname) throws DevFailed {
String[] attnames = new String[1];
attnames[0] = attname;
return get_class_attribute_property(database, classname, attnames)[0];
}
//==========================================================================
/* (non-Javadoc)
* @see fr.esrf.TangoApi.IDatabaseDAO#get_class_attribute_property(java.lang.String, java.lang.String[])
*/
//==========================================================================
public DbAttribute[] get_class_attribute_property(Database database, String classname, String[] attnames)
throws DevFailed {
if (!database.isAccess_checked()) checkAccess(database);
DeviceData argin = new DeviceData();
DeviceData argout;
int mode = 2;
try {
// value is an array
argin.insert(ApiUtil.toStringArray(classname, attnames));
argout = command_inout(database, "DbGetClassAttributeProperty2", argin);
} catch (DevFailed e) {
if (e.errors[0].reason.equals("API_CommandNotFound")) {
// Value is just one element
argout = command_inout(database, "DbGetClassAttributeProperty", argin);
mode = 1;
} else
throw e;
}
return ApiUtil.toDbAttributeArray(argout.extractStringArray(), mode);
}
//==========================================================================
/* (non-Javadoc)
* @see fr.esrf.TangoApi.IDatabaseDAO#put_class_attribute_property(java.lang.String, fr.esrf.TangoApi.DbAttribute[])
*/
//==========================================================================
public void put_class_attribute_property(Database database, String classname, DbAttribute[] attr)
throws DevFailed {
if (!database.isAccess_checked()) checkAccess(database);
DeviceData argin = new DeviceData();
argin.insert(ApiUtil.toStringArray(classname, attr, 2));
command_inout(database, "DbPutClassAttributeProperty2", argin);
}
//==========================================================================
/* (non-Javadoc)
* @see fr.esrf.TangoApi.IDatabaseDAO#put_class_attribute_property(java.lang.String, fr.esrf.TangoApi.DbAttribute)
*/
//==========================================================================
public void put_class_attribute_property(Database database, String classname, DbAttribute attr)
throws DevFailed {
DbAttribute[] da = new DbAttribute[1];
da[0] = attr;
put_class_attribute_property(database, classname, da);
}
//==========================================================================
/* (non-Javadoc)
* @see fr.esrf.TangoApi.IDatabaseDAO#delete_class_attribute_property(java.lang.String, java.lang.String, java.lang.String)
*/
//==========================================================================
public void delete_class_attribute_property(Database database, String name, String attname, String propname)
throws DevFailed {
String[] array = new String[1];
array[0] = propname;
delete_class_attribute_property(database, name, attname, array);
}
//==========================================================================
/* (non-Javadoc)
* @see fr.esrf.TangoApi.IDatabaseDAO#delete_class_attribute_property(java.lang.String, java.lang.String, java.lang.String[])
*/
//==========================================================================
public void delete_class_attribute_property(Database database, String name, String attname, String[] propnames)
throws DevFailed {
if (!database.isAccess_checked()) checkAccess(database);
String[] array = new String[2 + propnames.length];
array[0] = name;
array[1] = attname;
System.arraycopy(propnames, 0, array, 2, propnames.length);
DeviceData argin = new DeviceData();
argin.insert(array);
command_inout(database, "DbDeleteClassAttributeProperty", argin);
}
//==========================================================================
/* (non-Javadoc)
* @see fr.esrf.TangoApi.IDatabaseDAO#get_device_exported(java.lang.String)
*/
//==========================================================================
public String[] get_device_exported(Database database, String wildcard)
throws DevFailed {
if (!database.isAccess_checked()) checkAccess(database);
DeviceData argin = new DeviceData();
argin.insert(wildcard);
DeviceData argout = command_inout(database, "DbGetDeviceExportedList", argin);
return argout.extractStringArray();
}
//==========================================================================
/* (non-Javadoc)
* @see fr.esrf.TangoApi.IDatabaseDAO#get_device_exported_for_class(java.lang.String)
*/
//==========================================================================
public String[] get_device_exported_for_class(Database database, String classname)
throws DevFailed {
if (!database.isAccess_checked()) checkAccess(database);
DeviceData argin = new DeviceData();
argin.insert(classname);
DeviceData argout = command_inout(database, "DbGetExportdDeviceListForClass", argin);
return argout.extractStringArray();
}
//==========================================================================
// Aliases management
//==========================================================================
// ==========================================================================
/**
* Query the database for an alias for the specified device.
*
* @param database specified database object.
* @param deviceName device's name.
* @return the device alias found.
* @throws DevFailed in case of database access failed
*/
// ==========================================================================
public String getAliasFromDevice(Database database, String deviceName) throws DevFailed {
DeviceData argin = new DeviceData();
argin.insert(deviceName);
DeviceData argout = command_inout(database, "DbGetDeviceAlias", argin);
return argout.extractString();
}
// ==========================================================================
/**
* Query the database for a device name for the specified alias.
*
* @param database specified database object.
* @param alias alias name.
* @return the device name found.
* @throws DevFailed in case of database access failed
*/
// ==========================================================================
public String getDeviceFromAlias(Database database, String alias) throws DevFailed {
DeviceData argin = new DeviceData();
argin.insert(alias);
DeviceData argout = command_inout(database, "DbGetAliasDevice", argin);
return argout.extractString();
}
// ==========================================================================
/**
* Query the database for an alias for the specified attribute.
*
* @param database specified database object.
* @param attName attribute name.
* @return the alias found.
* @throws DevFailed in case of database access failed
*/
// ==========================================================================
public String getAliasFromAttribute(Database database, String attName) throws DevFailed {
DeviceData argin = new DeviceData();
argin.insert(attName);
DeviceData argout = command_inout(database, "DbGetAttributeAlias2", argin);
return argout.extractString();
}
// ==========================================================================
/**
* Query the database for an attribute name for the specified alias.
*
* @param database specified database object.
* @param alias alias name.
* @return the attribute found.
* @throws DevFailed in case of database access failed
*/
// ==========================================================================
public String getAttributeFromAlias(Database database, String alias) throws DevFailed {
DeviceData argin = new DeviceData();
argin.insert(alias);
DeviceData argout = command_inout(database, "DbGetAliasAttribute", argin);
return argout.extractString();
}
//==========================================================================
/* (non-Javadoc)
* @see fr.esrf.TangoApi.IDatabaseDAO#get_device_alias_list(java.lang.String)
*/
//==========================================================================
public String[] get_device_alias_list(Database database, String wildcard)
throws DevFailed {
DeviceData argin = new DeviceData();
argin.insert(wildcard);
DeviceData argout = command_inout(database, "DbGetDeviceAliasList", argin);
return argout.extractStringArray();
}
//==========================================================================
/* (non-Javadoc)
* @see fr.esrf.TangoApi.IDatabaseDAO#get_device_alias(java.lang.String)
*/
//==========================================================================
public String get_device_alias(Database database, String devname)
throws DevFailed {
if (!database.isAccess_checked()) checkAccess(database);
DeviceData argin = new DeviceData();
argin.insert(devname);
DeviceData argout = command_inout(database, "DbGetDeviceAlias", argin);
return argout.extractString();
}
//==========================================================================
/* (non-Javadoc)
* @see fr.esrf.TangoApi.IDatabaseDAO#get_alias_device(java.lang.String)
*/
//==========================================================================
public String get_alias_device(Database database, String alias)
throws DevFailed {
if (!database.isAccess_checked()) checkAccess(database);
DeviceData argin = new DeviceData();
argin.insert(alias);
DeviceData argout = command_inout(database, "DbGetAliasDevice", argin);
return argout.extractString();
}
//==========================================================================
/* (non-Javadoc)
* @see fr.esrf.TangoApi.IDatabaseDAO#put_device_alias(java.lang.String, java.lang.String)
*/
//==========================================================================
public void put_device_alias(Database database, String devname, String aliasname)
throws DevFailed {
String[] array = new String[2];
array[0] = devname;
array[1] = aliasname;
DeviceData argin = new DeviceData();
argin.insert(array);
command_inout(database, "DbPutDeviceAlias", argin);
}
//==========================================================================
/* (non-Javadoc)
* @see fr.esrf.TangoApi.IDatabaseDAO#delete_device_alias(java.lang.String)
*/
//==========================================================================
public void delete_device_alias(Database database, String alias)
throws DevFailed {
if (!database.isAccess_checked()) checkAccess(database);
DeviceData argin = new DeviceData();
argin.insert(alias);
command_inout(database, "DbDeleteDeviceAlias", argin);
}
//==========================================================================
/* (non-Javadoc)
* @see fr.esrf.TangoApi.IDatabaseDAO#get_attribute_alias_list(java.lang.String)
*/
//==========================================================================
public String[] get_attribute_alias_list(Database database, String wildcard)
throws DevFailed {
DeviceData argin = new DeviceData();
argin.insert(wildcard);
DeviceData argout = command_inout(database, "DbGetAttributeAliasList", argin);
return argout.extractStringArray();
}
//==========================================================================
/* (non-Javadoc)
* @see fr.esrf.TangoApi.IDatabaseDAO#get_attribute_alias(java.lang.String)
*/
//==========================================================================
public String get_attribute_alias(Database database, String attname)
throws DevFailed {
if (!database.isAccess_checked()) checkAccess(database);
DeviceData argin = new DeviceData();
argin.insert(attname);
DeviceData argout = command_inout(database, "DbGetAttributeAlias", argin);
return argout.extractString();
}
//==========================================================================
/* (non-Javadoc)
* @see fr.esrf.TangoApi.IDatabaseDAO#put_attribute_alias(java.lang.String, java.lang.String)
*/
//==========================================================================
public void put_attribute_alias(Database database, String attname, String aliasname)
throws DevFailed {
String[] array = new String[2];
array[0] = attname;
array[1] = aliasname;
DeviceData argin = new DeviceData();
argin.insert(array);
command_inout(database, "DbPutAttributeAlias", argin);
}
//==========================================================================
/* (non-Javadoc)
* @see fr.esrf.TangoApi.IDatabaseDAO#delete_attribute_alias(java.lang.String)
*/
//==========================================================================
public void delete_attribute_alias(Database database, String alias)
throws DevFailed {
if (!database.isAccess_checked()) checkAccess(database);
DeviceData argin = new DeviceData();
argin.insert(alias);
command_inout(database, "DbDeleteAttributeAlias", argin);
}
//==========================================================================
//==========================================================================
/* (non-Javadoc)
* @see fr.esrf.TangoApi.IDatabaseDAO#getDevices(java.lang.String)
*/
public String[] getDevices(Database database, String wildcard) throws DevFailed {
// Get each field of device name
StringTokenizer stk = new StringTokenizer(wildcard, "/");
Vector vector = new Vector();
while (stk.hasMoreTokens())
vector.add(stk.nextToken());
if (vector.size()<3)
Except.throw_exception("TangoApi_DeviceNameNotValid",
"Device name not valid", "ATangoApi.Database.getDevices()");
String domain = vector.elementAt(0);
String family = vector.elementAt(1);
String member = vector.elementAt(2);
vector.clear();
// Check for specifieddomain
String[] domains = get_device_domain(database, domain);
if (domains.length==0)
domains = new String[]{domain};
// Check for all domains found
for (String domain_1 : domains) {
String domain_header = domain_1 + "/";
// Get families
String[] families = get_device_family(database, domain_header + family);
if (families.length==0)
families = new String[]{family};
// Check for all falilies found
for (String family_1 : families) {
String family_header = domain_header + family_1 + "/";
String[] members = get_device_member(database, family_header + member);
// Add all members found
for (String member_1 : members)
vector.add(family_header + member_1);
}
}
// Copy all from vector to String array
String[] devices = new String[vector.size()];
for (int i = 0 ; i convertPropertyHistory(String[] ret, boolean isAttribute)
throws DevFailed {
ArrayList list = new ArrayList();
int i = 0;
int count = 0;
int offset;
String aName = "";
String pName;
String pDate;
String pCount;
while (i dbHistories = convertPropertyHistory(argout.extractStringArray(), false);
DbHistory[] array = new DbHistory[dbHistories.size()];
for (int i=0 ; i dbHistories = convertPropertyHistory(argout.extractStringArray(), true);
DbHistory[] array = new DbHistory[dbHistories.size()];
for (int i=0 ; i dbHistories = convertPropertyHistory(argout.extractStringArray(), false);
DbHistory[] array = new DbHistory[dbHistories.size()];
for (int i=0 ; i dbHistories = convertPropertyHistory(argout.extractStringArray(), true);
DbHistory[] array = new DbHistory[dbHistories.size()];
for (int i=0 ; i dbHistories = convertPropertyHistory(argout.extractStringArray(), false);
DbHistory[] array = new DbHistory[dbHistories.size()];
for (int i=0 ; i v = new Vector();
char separ;
// Read Service property
DbDatum datum = get_property(database, TangoConst.CONTROL_SYSTEM,
TangoConst.SERVICE_PROP_NAME, true);
if (!datum.is_empty()) {
String[] services = datum.extractStringArray();
// Build filter
String target = servicename.toLowerCase();
if (!instname.equals("*")) {
target += "/" + instname.toLowerCase();
separ = ':';
} else
separ = '/';
// Search with filter
int start;
for (String service : services) {
start = service.indexOf(separ);
if (start>0) {
String startLine =
service.substring(0, start).toLowerCase();
if (startLine.equals(target))
v.add(service.substring(
service.indexOf(':') + 1));
}
}
}
String[] result = new String[v.size()];
for (int i = 0 ; i v = new Vector();
for (String service : services) {
String line = service.toLowerCase();
int idx = line.indexOf(':');
if (idx>0)
line = line.substring(0, idx);
if (line.equals(target)) {
// Found -> replace existing by new one
exists = true;
v.add(new_line);
} else
v.add(service);
}
if (!exists)
v.add(new_line);
// Copy vector to String array
services = new String[v.size()];
for (int i = 0 ; i v = new Vector();
for (String service : services) {
String line = service.toLowerCase();
int idx = line.indexOf(':');
if (idx>0)
line = line.substring(0, idx);
if (line.equals(target)) // Found
exists = true;
else
v.add(service);
}
if (exists) {
// Copy vector to String array
services = new String[v.size()];
for (int i = 0 ; i0)
access_devname = services[0];
else {
// if not set --> No check
//System.out.println("No Access Service Found !");
database.check_access = false;
access_service_read = true;
return TangoConst.ACCESS_WRITE;
}
}
// If tango_host not from env -> add tango_host as header for access device name
// Check if header not already in access_devname :-)
if (!devUrl.fromEnv && !access_devname.startsWith("tango://"))
access_devname = "tango://" + devUrl.host + ":" + devUrl.port + "/" + access_devname;
// Then build Tango Access Control Proxy
database.setAccess_proxy(new AccessProxy(access_devname));
}
if (database.getAccess_proxy()!=null) {
access = database.getAccess_proxy().checkAccessControl(devname);
}
// if database access not already checked, and not first import -> do it now
if (!database.isAccess_checked())
if (!devname.equals(database.device.name()))
checkAccess(database);
} catch (DevFailed e) {
// In case of failure, returns always TangoConst.ACCESS_READ
access = TangoConst.ACCESS_READ;
// if cannot import AccessProxy
// -> change description to be more explicit
if (e.errors.length>1)
if (e.errors[1].reason.equals("TangoApi_CANNOT_IMPORT_DEVICE"))
e.errors[0].desc +=
"\nControlled access service defined in Db but unreachable --> Read Only access given to all devices...";
database.setAccess_devfailed(e);
//Except.print_exception(e);
}
}
return access;
}
//===================================================================
/**
* Check for specified device, the specified command is allowed.
*
* @param classname Specified class name.
* @param cmd Specified command name.
*/
//===================================================================
public boolean isCommandAllowed(Database database, String classname, String cmd)
throws DevFailed {
if (database.getAccess_proxy()==null) {
if (!database.isAccess_checked())
checkAccess(database);
return !database.check_access;
} else
return database.getAccess_proxy().isCommandAllowed(classname, cmd);
}
//===================================================================
//===================================================================
public String[] getPossibleTangoHosts(Database database) {
String[] tangoHosts = null;
try {
DeviceData deviceData = database.command_inout("DbGetCSDbServerList");
tangoHosts = deviceData.extractStringArray();
} catch (DevFailed e) {
String desc = e.errors[0].desc.toLowerCase();
try {
if (desc.startsWith("command ") && desc.endsWith("not found")) {
tangoHosts = new String[]{database.getFullTangoHost()};
} else
System.err.println(e.errors[0].desc);
} catch (DevFailed e2) {
System.err.println(e2.errors[0].desc);
}
}
return tangoHosts;
}
//===================================================================
//===================================================================
//===================================================================
/**
* Pipe related methods
*/
//===================================================================
// ===================================================================
/**
* Query the database for a list of device pipe properties
* for the specified pipe.
* @param database Database object.
* @param deviceName specified device.
* @return a list of device pipe properties.
* @throws DevFailed in case of database access failed
*/
// ===================================================================
public DbPipe getDevicePipeProperties(Database database, String deviceName, String pipeName) throws DevFailed {
DeviceData argIn = new DeviceData();
argIn.insert(new String[]{deviceName, pipeName});
DeviceData argOut = database.command_inout("DbGetDevicePipeProperty", argIn);
return ApiUtil.toDbPipe(pipeName, argOut.extractStringArray());
}
// ===================================================================
/**
* Query the database for a list of class pipe properties
* for the specified pipe.
* @param database Database object.
* @param className specified class.
* @param pipeName specified pipe.
* @return a list of class pipe properties.
* @throws DevFailed in case of database access failed
*/
// ===================================================================
public DbPipe getClassPipeProperties(Database database, String className, String pipeName) throws DevFailed {
DeviceData argIn = new DeviceData();
argIn.insert(new String[]{className, pipeName});
DeviceData argOut = database.command_inout("DbGetClassPipeProperty", argIn);
return ApiUtil.toDbPipe(pipeName, argOut.extractStringArray());
}
// ==========================================================================
/**
* Insert or update a list of pipe properties for the specified device.
* The property names and their values are specified by the DbAPipe.
*
* @param database Database object.
* @param deviceName device name.
* @param dbPipe pipe name, and properties (names and values).
* @throws DevFailed in case of database access failed
*/
// ==========================================================================
public void putDevicePipeProperty(Database database, String deviceName, DbPipe dbPipe) throws DevFailed {
DeviceData argIn = new DeviceData();
String[] array = ApiUtil.toStringArray(deviceName, dbPipe);
argIn.insert(array);
database.command_inout("DbPutDevicePipeProperty", argIn);
}
// ==========================================================================
/**
* Insert or update a list of pipe properties for the specified class.
* The property names and their values are specified by the DbAPipe.
*
* @param database Database object.
* @param className class name.
* @param dbPipe pipe name, and properties (names and values).
* @throws DevFailed in case of database access failed
*/
// ==========================================================================
public void putClassPipeProperty(Database database, String className, DbPipe dbPipe) throws DevFailed {
DeviceData argIn = new DeviceData();
String[] array = ApiUtil.toStringArray(className, dbPipe);
argIn.insert(array);
database.command_inout("DbPutClassPipeProperty", argIn);
}
// ===================================================================
/**
* Query database for a list of pipes for specified device and specified wildcard.
*
* @param deviceName specified device name.
* @param wildcard specified wildcard.
* @return a list of pipes defined in database for specified device and specified wildcard.
* @throws DevFailed in case of database access failed
*/
// ===================================================================
public List getDevicePipeList(Database database, String deviceName, String wildcard) throws DevFailed {
DeviceData argIn = new DeviceData();
argIn.insert(new String[]{deviceName, wildcard});
DeviceData argOut = database.command_inout("DbGetDevicePipeList", argIn);
//System.out.println("database.command_inout(\"DbGetDevicePipeList\", argIn)");
String[] array = argOut.extractStringArray();
ArrayList list = new ArrayList();
Collections.addAll(list, array);
return list;
}
// ===================================================================
/**
* Query database for a list of pipes for specified class and specified wildcard.
*
* @param className specified class name.
* @param wildcard specified wildcard.
* @return a list of pipes defined in database for specified class and specified wildcard.
* @throws DevFailed in case of database access failed
*/
// ===================================================================
public List getClassPipeList(Database database, String className, String wildcard) throws DevFailed {
DeviceData argIn = new DeviceData();
argIn.insert(new String[]{className, wildcard});
DeviceData argOut = database.command_inout("DbGetDevicePipeList", argIn);
String[] array = argOut.extractStringArray();
ArrayList list = new ArrayList();
Collections.addAll(list, array);
return list;
}
// ==========================================================================
/**
* Delete a pipe property for the specified device.
*
* @param database Database object.
* @param deviceName Device name.
* @param pipeName pipe name
* @param propertyNames property names
* @throws DevFailed in case of database access failed
*/
// ==========================================================================
public void deleteDevicePipeProperties(Database database, String deviceName,
String pipeName, List propertyNames) throws DevFailed {
String[] array = new String[propertyNames.size()+2];
int i=0;
array[i++] = deviceName;
array[i++] = pipeName;
for (String propertyName : propertyNames)
array[i++] = propertyName;
DeviceData argIn = new DeviceData();
argIn.insert(array);
database.command_inout("DbDeleteDevicePipeProperty", argIn);
}
// ==========================================================================
/**
* Delete a pipe property for the specified class.
*
* @param database Database object.
* @param className class name.
* @param pipeName pipe name
* @param propertyNames property names
* @throws DevFailed in case of database access failed
*/
// ==========================================================================
public void deleteClassPipeProperties(Database database, String className,
String pipeName, List propertyNames) throws DevFailed {
String[] array = new String[propertyNames.size()+2];
int i=0;
array[i++] = className;
array[i++] = pipeName;
for (String propertyName : propertyNames)
array[i++] = propertyName;
DeviceData argIn = new DeviceData();
argIn.insert(array);
database.command_inout("DbDeleteClassPipeProperty", argIn);
}
// ===================================================================
/**
* Delete specified pipe for specified device.
* @param database Database object.
* @param deviceName device name
* @param pipeName pipe name
* @throws DevFailed in case of database access failed
*/
// ===================================================================
public void deleteDevicePipe(Database database, String deviceName, String pipeName) throws DevFailed {
String[] array = new String[] { deviceName, pipeName };
DeviceData argIn = new DeviceData();
argIn.insert(array);
database.command_inout("DbDeleteDevicePipe", argIn);
}
// ===================================================================
/**
* Delete specified pipe for specified class.
* @param database Database object.
* @param className class name
* @param pipeName pipe name
* @throws DevFailed in case of database access failed
*/
// ===================================================================
public void deleteClassPipe(Database database, String className, String pipeName) throws DevFailed {
String[] array = new String[] { className, pipeName };
DeviceData argIn = new DeviceData();
argIn.insert(array);
database.command_inout("DbDeleteClassPipe", argIn);
}
// ===================================================================
/**
* Delete all properties for specified pipes
* @param database Database object.
* @param deviceName device name
* @param pipeNames pipe names
* @throws DevFailed in case of database access failed
*/
// ===================================================================
public void deleteAllDevicePipeProperty(Database database, String deviceName,
List pipeNames) throws DevFailed {
String[] array = new String[1+pipeNames.size()];
int i=0;
array[i++] = deviceName;
for (String pipeName : pipeNames)
array[i++] = pipeName;
DeviceData argIn = new DeviceData();
argIn.insert(array);
database.command_inout("DbDeleteAllDevicePipeProperty", argIn);
}
// ===================================================================
/**
* Returns the property history for specified pipe.
* @param database Database object.
* @param deviceName device name
* @param pipeName pipe name
* @param propertyName property Name
* @return the property history for specified pipe.
* @throws DevFailed in case of database access failed
*/
// ===================================================================
public List getDevicePipePropertyHistory(Database database, String deviceName,
String pipeName, String propertyName) throws DevFailed {
String[] array = new String[] { deviceName, pipeName, propertyName };
DeviceData argIn = new DeviceData();
argIn.insert(array);
DeviceData argOut = database.command_inout("DbGetDevicePipePropertyHist", argIn);
return convertPropertyHistory(argOut.extractStringArray(), true);
}
// ===================================================================
/**
* Returns the property history for specified pipe.
* @param database Database object.
* @param className class name
* @param pipeName pipe name
* @param propertyName property Name
* @return the property history for specified pipe.
* @throws DevFailed in case of database access failed
*/
// ===================================================================
public List getClassPipePropertyHistory(Database database, String className,
String pipeName, String propertyName) throws DevFailed {
String[] array = new String[] { className, pipeName, propertyName };
DeviceData argIn = new DeviceData();
argIn.insert(array);
DeviceData argOut = database.command_inout("DbGetClassPipePropertyHist", argIn);
return convertPropertyHistory(argOut.extractStringArray(), true);
}
// ===================================================================
/**
* Query database to get a list of device using the specified device as
* as root for forwarded attributes
* @param deviceName the specified device
* @return a list of device using the specified device as as root for forwarded attributes
* @throws DevFailed
*/
// ===================================================================
public List getForwardedAttributeDataForDevice(Database database, String deviceName) throws DevFailed {
DeviceData argIn = new DeviceData();
argIn.insert(deviceName);
DeviceData argOut = database.command_inout("DbGetForwardedAttributeListForDevice", argIn);
String[] array = argOut.extractStringArray();
ArrayList list = new ArrayList();
for (int i=0 ; i
© 2015 - 2025 Weber Informatics LLC | Privacy Policy