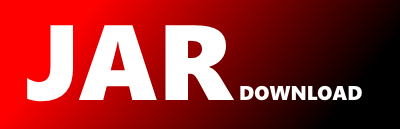
org.tap4j.ext.jmeter.model.AbstractSample Maven / Gradle / Ivy
Show all versions of tap4j-ext Show documentation
//
// This file was generated by the JavaTM Architecture for XML Binding(JAXB) Reference Implementation, v2.2.7
// See http://java.sun.com/xml/jaxb
// Any modifications to this file will be lost upon recompilation of the source schema.
// Generated on: 2018.05.19 at 08:25:57 PM NZST
//
package org.tap4j.ext.jmeter.model;
import java.util.ArrayList;
import java.util.List;
import javax.xml.bind.annotation.XmlAccessType;
import javax.xml.bind.annotation.XmlAccessorType;
import javax.xml.bind.annotation.XmlAttribute;
import javax.xml.bind.annotation.XmlSeeAlso;
import javax.xml.bind.annotation.XmlType;
/**
* Java class for AbstractSample complex type.
*
*
The following schema fragment specifies the expected content contained within this class.
*
*
* <complexType name="AbstractSample">
* <complexContent>
* <restriction base="{http://www.w3.org/2001/XMLSchema}anyType">
* <sequence>
* <element name="assertionResult" type="{}AssertionResult" maxOccurs="unbounded" minOccurs="0"/>
* </sequence>
* <attribute name="t" use="required" type="{http://www.w3.org/2001/XMLSchema}string" />
* <attribute name="it" type="{http://www.w3.org/2001/XMLSchema}string" />
* <attribute name="lt" type="{http://www.w3.org/2001/XMLSchema}string" />
* <attribute name="ts" type="{http://www.w3.org/2001/XMLSchema}string" />
* <attribute name="s" type="{http://www.w3.org/2001/XMLSchema}boolean" />
* <attribute name="lb" type="{http://www.w3.org/2001/XMLSchema}string" />
* <attribute name="rc" type="{http://www.w3.org/2001/XMLSchema}string" />
* <attribute name="rm" type="{http://www.w3.org/2001/XMLSchema}string" />
* <attribute name="tn" type="{http://www.w3.org/2001/XMLSchema}string" />
* <attribute name="dt" type="{http://www.w3.org/2001/XMLSchema}string" />
* <attribute name="de" type="{http://www.w3.org/2001/XMLSchema}string" />
* <attribute name="by" type="{http://www.w3.org/2001/XMLSchema}string" />
* <attribute name="sc" type="{http://www.w3.org/2001/XMLSchema}string" />
* <attribute name="ec" type="{http://www.w3.org/2001/XMLSchema}string" />
* <attribute name="ng" type="{http://www.w3.org/2001/XMLSchema}string" />
* <attribute name="na" type="{http://www.w3.org/2001/XMLSchema}string" />
* <attribute name="hn" type="{http://www.w3.org/2001/XMLSchema}string" />
* </restriction>
* </complexContent>
* </complexType>
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "AbstractSample", propOrder = {
"assertionResult"
})
@XmlSeeAlso({
HttpSample.class,
Sample.class
})
public class AbstractSample {
protected List assertionResult;
@XmlAttribute(name = "t", required = true)
protected String t;
@XmlAttribute(name = "it")
protected String it;
@XmlAttribute(name = "lt")
protected String lt;
@XmlAttribute(name = "ts")
protected String ts;
@XmlAttribute(name = "s")
protected Boolean s;
@XmlAttribute(name = "lb")
protected String lb;
@XmlAttribute(name = "rc")
protected String rc;
@XmlAttribute(name = "rm")
protected String rm;
@XmlAttribute(name = "tn")
protected String tn;
@XmlAttribute(name = "dt")
protected String dt;
@XmlAttribute(name = "de")
protected String de;
@XmlAttribute(name = "by")
protected String by;
@XmlAttribute(name = "sc")
protected String sc;
@XmlAttribute(name = "ec")
protected String ec;
@XmlAttribute(name = "ng")
protected String ng;
@XmlAttribute(name = "na")
protected String na;
@XmlAttribute(name = "hn")
protected String hn;
/**
* Gets the value of the assertionResult property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the JAXB object.
* This is why there is not a set
method for the assertionResult property.
*
*
* For example, to add a new item, do as follows:
*
* getAssertionResult().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link AssertionResult }
*
*
*/
public List getAssertionResult() {
if (assertionResult == null) {
assertionResult = new ArrayList();
}
return this.assertionResult;
}
/**
* Gets the value of the t property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getT() {
return t;
}
/**
* Sets the value of the t property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setT(String value) {
this.t = value;
}
/**
* Gets the value of the it property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getIt() {
return it;
}
/**
* Sets the value of the it property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setIt(String value) {
this.it = value;
}
/**
* Gets the value of the lt property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getLt() {
return lt;
}
/**
* Sets the value of the lt property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setLt(String value) {
this.lt = value;
}
/**
* Gets the value of the ts property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getTs() {
return ts;
}
/**
* Sets the value of the ts property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setTs(String value) {
this.ts = value;
}
/**
* Gets the value of the s property.
*
* @return
* possible object is
* {@link Boolean }
*
*/
public Boolean isS() {
return s;
}
/**
* Sets the value of the s property.
*
* @param value
* allowed object is
* {@link Boolean }
*
*/
public void setS(Boolean value) {
this.s = value;
}
/**
* Gets the value of the lb property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getLb() {
return lb;
}
/**
* Sets the value of the lb property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setLb(String value) {
this.lb = value;
}
/**
* Gets the value of the rc property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getRc() {
return rc;
}
/**
* Sets the value of the rc property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setRc(String value) {
this.rc = value;
}
/**
* Gets the value of the rm property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getRm() {
return rm;
}
/**
* Sets the value of the rm property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setRm(String value) {
this.rm = value;
}
/**
* Gets the value of the tn property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getTn() {
return tn;
}
/**
* Sets the value of the tn property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setTn(String value) {
this.tn = value;
}
/**
* Gets the value of the dt property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getDt() {
return dt;
}
/**
* Sets the value of the dt property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setDt(String value) {
this.dt = value;
}
/**
* Gets the value of the de property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getDe() {
return de;
}
/**
* Sets the value of the de property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setDe(String value) {
this.de = value;
}
/**
* Gets the value of the by property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getBy() {
return by;
}
/**
* Sets the value of the by property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setBy(String value) {
this.by = value;
}
/**
* Gets the value of the sc property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getSc() {
return sc;
}
/**
* Sets the value of the sc property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setSc(String value) {
this.sc = value;
}
/**
* Gets the value of the ec property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getEc() {
return ec;
}
/**
* Sets the value of the ec property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setEc(String value) {
this.ec = value;
}
/**
* Gets the value of the ng property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getNg() {
return ng;
}
/**
* Sets the value of the ng property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setNg(String value) {
this.ng = value;
}
/**
* Gets the value of the na property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getNa() {
return na;
}
/**
* Sets the value of the na property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setNa(String value) {
this.na = value;
}
/**
* Gets the value of the hn property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getHn() {
return hn;
}
/**
* Sets the value of the hn property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setHn(String value) {
this.hn = value;
}
}