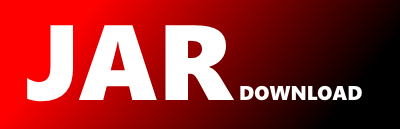
org.teamapps.dto.UiInfiniteItemView Maven / Gradle / Ivy
The newest version!
package org.teamapps.dto;
import java.util.ArrayList;
import java.util.Iterator;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Set;
import java.util.function.Supplier;
import java.util.stream.Collectors;
import com.fasterxml.jackson.databind.annotation.JsonDeserialize;
import com.fasterxml.jackson.databind.annotation.JsonSerialize;
import com.fasterxml.jackson.annotation.JsonTypeInfo;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.databind.annotation.JsonTypeIdResolver;
/**
* THIS IS GENERATED CODE!
* PLEASE DO NOT MODIFY - ALL YOUR WORK WOULD BE LOST!
*/
@JsonTypeInfo(use = JsonTypeInfo.Id.CUSTOM, property = "_type", defaultImpl = UiInfiniteItemView.class)
public class UiInfiniteItemView extends UiComponent implements UiObject {
protected UiTemplate itemTemplate;
protected float itemWidth = 0;
protected int rowHeight;
protected List data;
protected int totalNumberOfRecords = 0;
protected int horizontalItemMargin = 0;
protected boolean autoHeight = false;
protected UiItemJustification itemJustification = UiItemJustification.LEFT;
protected UiVerticalItemAlignment verticalItemAlignment = UiVerticalItemAlignment.STRETCH;
protected boolean contextMenuEnabled = false;
protected boolean selectionEnabled;
/**
* @deprecated Only for Jackson deserialization. Use the other constructor instead.
*/
@Deprecated
public UiInfiniteItemView() {
// default constructor for Jackson
}
public UiInfiniteItemView(UiTemplate itemTemplate, int rowHeight) {
super();
this.itemTemplate = itemTemplate;
this.rowHeight = rowHeight;
}
@com.fasterxml.jackson.annotation.JsonIgnore
public UiObjectType getUiObjectType() {
return UiObjectType.UI_INFINITE_ITEM_VIEW;
}
@SuppressWarnings("unchecked")
public String toString() {
return new StringBuilder(getClass().getSimpleName()).append(": ")
.append("id=" + id).append(", ")
.append("debuggingId=" + debuggingId).append(", ")
.append("classNamesBySelector=" + classNamesBySelector).append(", ")
.append("visible=" + visible).append(", ")
.append("stylesBySelector=" + stylesBySelector).append(", ")
.append("attributesBySelector=" + attributesBySelector).append(", ")
.append(itemTemplate != null ? "itemTemplate={" + itemTemplate.toString() + "}" : "").append(", ")
.append("itemWidth=" + itemWidth).append(", ")
.append("rowHeight=" + rowHeight).append(", ")
.append("totalNumberOfRecords=" + totalNumberOfRecords).append(", ")
.append("horizontalItemMargin=" + horizontalItemMargin).append(", ")
.append("autoHeight=" + autoHeight).append(", ")
.append("itemJustification=" + itemJustification).append(", ")
.append("verticalItemAlignment=" + verticalItemAlignment).append(", ")
.append("contextMenuEnabled=" + contextMenuEnabled).append(", ")
.append("selectionEnabled=" + selectionEnabled).append(", ")
.append(data != null ? "data={" + data.toString() + "}" : "")
.toString();
}
@com.fasterxml.jackson.annotation.JsonGetter("itemTemplate")
public UiTemplate getItemTemplate() {
return itemTemplate;
}
@com.fasterxml.jackson.annotation.JsonGetter("itemWidth")
public float getItemWidth() {
return itemWidth;
}
@com.fasterxml.jackson.annotation.JsonGetter("rowHeight")
public int getRowHeight() {
return rowHeight;
}
@com.fasterxml.jackson.annotation.JsonGetter("data")
public List getData() {
return data;
}
@com.fasterxml.jackson.annotation.JsonGetter("totalNumberOfRecords")
public int getTotalNumberOfRecords() {
return totalNumberOfRecords;
}
@com.fasterxml.jackson.annotation.JsonGetter("horizontalItemMargin")
public int getHorizontalItemMargin() {
return horizontalItemMargin;
}
@com.fasterxml.jackson.annotation.JsonGetter("autoHeight")
public boolean getAutoHeight() {
return autoHeight;
}
@com.fasterxml.jackson.annotation.JsonGetter("itemJustification")
public UiItemJustification getItemJustification() {
return itemJustification;
}
@com.fasterxml.jackson.annotation.JsonGetter("verticalItemAlignment")
public UiVerticalItemAlignment getVerticalItemAlignment() {
return verticalItemAlignment;
}
@com.fasterxml.jackson.annotation.JsonGetter("contextMenuEnabled")
public boolean getContextMenuEnabled() {
return contextMenuEnabled;
}
@com.fasterxml.jackson.annotation.JsonGetter("selectionEnabled")
public boolean getSelectionEnabled() {
return selectionEnabled;
}
@com.fasterxml.jackson.annotation.JsonSetter("id")
public UiInfiniteItemView setId(String id) {
this.id = id;
return this;
}
@com.fasterxml.jackson.annotation.JsonSetter("debuggingId")
public UiInfiniteItemView setDebuggingId(String debuggingId) {
this.debuggingId = debuggingId;
return this;
}
@com.fasterxml.jackson.annotation.JsonSetter("visible")
public UiInfiniteItemView setVisible(boolean visible) {
this.visible = visible;
return this;
}
@com.fasterxml.jackson.annotation.JsonSetter("stylesBySelector")
public UiInfiniteItemView setStylesBySelector(Map> stylesBySelector) {
this.stylesBySelector = stylesBySelector;
return this;
}
@com.fasterxml.jackson.annotation.JsonSetter("classNamesBySelector")
public UiInfiniteItemView setClassNamesBySelector(Map> classNamesBySelector) {
this.classNamesBySelector = classNamesBySelector;
return this;
}
@com.fasterxml.jackson.annotation.JsonSetter("attributesBySelector")
public UiInfiniteItemView setAttributesBySelector(Map> attributesBySelector) {
this.attributesBySelector = attributesBySelector;
return this;
}
@com.fasterxml.jackson.annotation.JsonSetter("itemWidth")
public UiInfiniteItemView setItemWidth(float itemWidth) {
this.itemWidth = itemWidth;
return this;
}
@com.fasterxml.jackson.annotation.JsonSetter("data")
public UiInfiniteItemView setData(List data) {
this.data = data;
return this;
}
@com.fasterxml.jackson.annotation.JsonSetter("totalNumberOfRecords")
public UiInfiniteItemView setTotalNumberOfRecords(int totalNumberOfRecords) {
this.totalNumberOfRecords = totalNumberOfRecords;
return this;
}
@com.fasterxml.jackson.annotation.JsonSetter("horizontalItemMargin")
public UiInfiniteItemView setHorizontalItemMargin(int horizontalItemMargin) {
this.horizontalItemMargin = horizontalItemMargin;
return this;
}
@com.fasterxml.jackson.annotation.JsonSetter("autoHeight")
public UiInfiniteItemView setAutoHeight(boolean autoHeight) {
this.autoHeight = autoHeight;
return this;
}
@com.fasterxml.jackson.annotation.JsonSetter("itemJustification")
public UiInfiniteItemView setItemJustification(UiItemJustification itemJustification) {
this.itemJustification = itemJustification;
return this;
}
@com.fasterxml.jackson.annotation.JsonSetter("verticalItemAlignment")
public UiInfiniteItemView setVerticalItemAlignment(UiVerticalItemAlignment verticalItemAlignment) {
this.verticalItemAlignment = verticalItemAlignment;
return this;
}
@com.fasterxml.jackson.annotation.JsonSetter("contextMenuEnabled")
public UiInfiniteItemView setContextMenuEnabled(boolean contextMenuEnabled) {
this.contextMenuEnabled = contextMenuEnabled;
return this;
}
@com.fasterxml.jackson.annotation.JsonSetter("selectionEnabled")
public UiInfiniteItemView setSelectionEnabled(boolean selectionEnabled) {
this.selectionEnabled = selectionEnabled;
return this;
}
public static class DisplayedRangeChangedEvent implements UiEvent {
@UiComponentId protected String componentId;
protected int startIndex;
protected int length;
protected List displayedRecordIds;
protected UiInfiniteItemViewDataRequest dataRequest;
/**
* @deprecated Only for Jackson deserialization. Use the other constructor instead.
*/
@Deprecated
public DisplayedRangeChangedEvent() {
// default constructor for Jackson
}
public DisplayedRangeChangedEvent(String componentId, int startIndex, int length, List displayedRecordIds, UiInfiniteItemViewDataRequest dataRequest) {
this.componentId = componentId;
this.startIndex = startIndex;
this.length = length;
this.displayedRecordIds = displayedRecordIds;
this.dataRequest = dataRequest;
}
public UiEventType getUiEventType() {
return UiEventType.UI_INFINITE_ITEM_VIEW_DISPLAYED_RANGE_CHANGED;
}
@SuppressWarnings("unchecked")
public String toString() {
return new StringBuilder(getClass().getSimpleName()).append(": ")
.append("componentId=" + componentId).append(", ")
.append("displayedRecordIds=" + displayedRecordIds).append(", ")
.append("startIndex=" + startIndex).append(", ")
.append("length=" + length).append(", ")
.append(dataRequest != null ? "dataRequest={" + dataRequest.toString() + "}" : "")
.toString();
}
@com.fasterxml.jackson.annotation.JsonGetter("componentId")
public String getComponentId() {
return componentId;
}
@com.fasterxml.jackson.annotation.JsonGetter("startIndex")
public int getStartIndex() {
return startIndex;
}
@com.fasterxml.jackson.annotation.JsonGetter("length")
public int getLength() {
return length;
}
@com.fasterxml.jackson.annotation.JsonGetter("displayedRecordIds")
public List getDisplayedRecordIds() {
return displayedRecordIds;
}
@com.fasterxml.jackson.annotation.JsonGetter("dataRequest")
public UiInfiniteItemViewDataRequest getDataRequest() {
return dataRequest;
}
}
public static class ItemClickedEvent implements UiEvent {
@UiComponentId protected String componentId;
protected int recordId;
protected boolean isDoubleClick;
/**
* @deprecated Only for Jackson deserialization. Use the other constructor instead.
*/
@Deprecated
public ItemClickedEvent() {
// default constructor for Jackson
}
public ItemClickedEvent(String componentId, int recordId, boolean isDoubleClick) {
this.componentId = componentId;
this.recordId = recordId;
this.isDoubleClick = isDoubleClick;
}
public UiEventType getUiEventType() {
return UiEventType.UI_INFINITE_ITEM_VIEW_ITEM_CLICKED;
}
@SuppressWarnings("unchecked")
public String toString() {
return new StringBuilder(getClass().getSimpleName()).append(": ")
.append("componentId=" + componentId).append(", ")
.append("recordId=" + recordId).append(", ")
.append("isDoubleClick=" + isDoubleClick)
.toString();
}
@com.fasterxml.jackson.annotation.JsonGetter("componentId")
public String getComponentId() {
return componentId;
}
@com.fasterxml.jackson.annotation.JsonGetter("recordId")
public int getRecordId() {
return recordId;
}
@com.fasterxml.jackson.annotation.JsonGetter("isDoubleClick")
public boolean getIsDoubleClick() {
return isDoubleClick;
}
}
public static class ContextMenuRequestedEvent implements UiEvent {
@UiComponentId protected String componentId;
protected int requestId;
protected int recordId;
/**
* @deprecated Only for Jackson deserialization. Use the other constructor instead.
*/
@Deprecated
public ContextMenuRequestedEvent() {
// default constructor for Jackson
}
public ContextMenuRequestedEvent(String componentId, int requestId, int recordId) {
this.componentId = componentId;
this.requestId = requestId;
this.recordId = recordId;
}
public UiEventType getUiEventType() {
return UiEventType.UI_INFINITE_ITEM_VIEW_CONTEXT_MENU_REQUESTED;
}
@SuppressWarnings("unchecked")
public String toString() {
return new StringBuilder(getClass().getSimpleName()).append(": ")
.append("componentId=" + componentId).append(", ")
.append("requestId=" + requestId).append(", ")
.append("recordId=" + recordId)
.toString();
}
@com.fasterxml.jackson.annotation.JsonGetter("componentId")
public String getComponentId() {
return componentId;
}
@com.fasterxml.jackson.annotation.JsonGetter("requestId")
public int getRequestId() {
return requestId;
}
@com.fasterxml.jackson.annotation.JsonGetter("recordId")
public int getRecordId() {
return recordId;
}
}
public static class AddDataCommand implements UiCommand {
@UiComponentId protected String componentId;
protected int startIndex;
protected List data;
protected int totalNumberOfRecords;
protected boolean clear;
/**
* @deprecated Only for Jackson deserialization. Use the other constructor instead.
*/
@Deprecated
public AddDataCommand() {
// default constructor for Jackson
}
public AddDataCommand(String componentId, int startIndex, List data, int totalNumberOfRecords, boolean clear) {
this.componentId = componentId;
this.startIndex = startIndex;
this.data = data;
this.totalNumberOfRecords = totalNumberOfRecords;
this.clear = clear;
}
@SuppressWarnings("unchecked")
public String toString() {
return new StringBuilder(getClass().getSimpleName()).append(": ")
.append("componentId=" + componentId).append(", ")
.append("startIndex=" + startIndex).append(", ")
.append("totalNumberOfRecords=" + totalNumberOfRecords).append(", ")
.append("clear=" + clear).append(", ")
.append(data != null ? "data={" + data.toString() + "}" : "")
.toString();
}
@com.fasterxml.jackson.annotation.JsonGetter("componentId")
public String getComponentId() {
return componentId;
}
@com.fasterxml.jackson.annotation.JsonGetter("startIndex")
public int getStartIndex() {
return startIndex;
}
@com.fasterxml.jackson.annotation.JsonGetter("data")
public List getData() {
return data;
}
@com.fasterxml.jackson.annotation.JsonGetter("totalNumberOfRecords")
public int getTotalNumberOfRecords() {
return totalNumberOfRecords;
}
@com.fasterxml.jackson.annotation.JsonGetter("clear")
public boolean getClear() {
return clear;
}
}
public static class RemoveDataCommand implements UiCommand {
@UiComponentId protected String componentId;
protected List ids;
/**
* @deprecated Only for Jackson deserialization. Use the other constructor instead.
*/
@Deprecated
public RemoveDataCommand() {
// default constructor for Jackson
}
public RemoveDataCommand(String componentId, List ids) {
this.componentId = componentId;
this.ids = ids;
}
@SuppressWarnings("unchecked")
public String toString() {
return new StringBuilder(getClass().getSimpleName()).append(": ")
.append("componentId=" + componentId).append(", ")
.append("ids=" + ids)
.toString();
}
@com.fasterxml.jackson.annotation.JsonGetter("componentId")
public String getComponentId() {
return componentId;
}
@com.fasterxml.jackson.annotation.JsonGetter("ids")
public List getIds() {
return ids;
}
}
public static class SetItemTemplateCommand implements UiCommand {
@UiComponentId protected String componentId;
protected UiTemplate itemTemplate;
/**
* @deprecated Only for Jackson deserialization. Use the other constructor instead.
*/
@Deprecated
public SetItemTemplateCommand() {
// default constructor for Jackson
}
public SetItemTemplateCommand(String componentId, UiTemplate itemTemplate) {
this.componentId = componentId;
this.itemTemplate = itemTemplate;
}
@SuppressWarnings("unchecked")
public String toString() {
return new StringBuilder(getClass().getSimpleName()).append(": ")
.append("componentId=" + componentId).append(", ")
.append(itemTemplate != null ? "itemTemplate={" + itemTemplate.toString() + "}" : "")
.toString();
}
@com.fasterxml.jackson.annotation.JsonGetter("componentId")
public String getComponentId() {
return componentId;
}
@com.fasterxml.jackson.annotation.JsonGetter("itemTemplate")
public UiTemplate getItemTemplate() {
return itemTemplate;
}
}
public static class SetItemWidthCommand implements UiCommand {
@UiComponentId protected String componentId;
protected float itemWidth;
/**
* @deprecated Only for Jackson deserialization. Use the other constructor instead.
*/
@Deprecated
public SetItemWidthCommand() {
// default constructor for Jackson
}
public SetItemWidthCommand(String componentId, float itemWidth) {
this.componentId = componentId;
this.itemWidth = itemWidth;
}
@SuppressWarnings("unchecked")
public String toString() {
return new StringBuilder(getClass().getSimpleName()).append(": ")
.append("componentId=" + componentId).append(", ")
.append("itemWidth=" + itemWidth)
.toString();
}
@com.fasterxml.jackson.annotation.JsonGetter("componentId")
public String getComponentId() {
return componentId;
}
@com.fasterxml.jackson.annotation.JsonGetter("itemWidth")
public float getItemWidth() {
return itemWidth;
}
}
public static class SetHorizontalItemMarginCommand implements UiCommand {
@UiComponentId protected String componentId;
protected int horizontalItemMargin;
/**
* @deprecated Only for Jackson deserialization. Use the other constructor instead.
*/
@Deprecated
public SetHorizontalItemMarginCommand() {
// default constructor for Jackson
}
public SetHorizontalItemMarginCommand(String componentId, int horizontalItemMargin) {
this.componentId = componentId;
this.horizontalItemMargin = horizontalItemMargin;
}
@SuppressWarnings("unchecked")
public String toString() {
return new StringBuilder(getClass().getSimpleName()).append(": ")
.append("componentId=" + componentId).append(", ")
.append("horizontalItemMargin=" + horizontalItemMargin)
.toString();
}
@com.fasterxml.jackson.annotation.JsonGetter("componentId")
public String getComponentId() {
return componentId;
}
@com.fasterxml.jackson.annotation.JsonGetter("horizontalItemMargin")
public int getHorizontalItemMargin() {
return horizontalItemMargin;
}
}
public static class SetItemJustificationCommand implements UiCommand {
@UiComponentId protected String componentId;
protected UiItemJustification itemJustification;
/**
* @deprecated Only for Jackson deserialization. Use the other constructor instead.
*/
@Deprecated
public SetItemJustificationCommand() {
// default constructor for Jackson
}
public SetItemJustificationCommand(String componentId, UiItemJustification itemJustification) {
this.componentId = componentId;
this.itemJustification = itemJustification;
}
@SuppressWarnings("unchecked")
public String toString() {
return new StringBuilder(getClass().getSimpleName()).append(": ")
.append("componentId=" + componentId).append(", ")
.append("itemJustification=" + itemJustification)
.toString();
}
@com.fasterxml.jackson.annotation.JsonGetter("componentId")
public String getComponentId() {
return componentId;
}
@com.fasterxml.jackson.annotation.JsonGetter("itemJustification")
public UiItemJustification getItemJustification() {
return itemJustification;
}
}
public static class SetVerticalItemAlignmentCommand implements UiCommand {
@UiComponentId protected String componentId;
protected UiVerticalItemAlignment verticalItemAlignment;
/**
* @deprecated Only for Jackson deserialization. Use the other constructor instead.
*/
@Deprecated
public SetVerticalItemAlignmentCommand() {
// default constructor for Jackson
}
public SetVerticalItemAlignmentCommand(String componentId, UiVerticalItemAlignment verticalItemAlignment) {
this.componentId = componentId;
this.verticalItemAlignment = verticalItemAlignment;
}
@SuppressWarnings("unchecked")
public String toString() {
return new StringBuilder(getClass().getSimpleName()).append(": ")
.append("componentId=" + componentId).append(", ")
.append("verticalItemAlignment=" + verticalItemAlignment)
.toString();
}
@com.fasterxml.jackson.annotation.JsonGetter("componentId")
public String getComponentId() {
return componentId;
}
@com.fasterxml.jackson.annotation.JsonGetter("verticalItemAlignment")
public UiVerticalItemAlignment getVerticalItemAlignment() {
return verticalItemAlignment;
}
}
public static class SetContextMenuContentCommand implements UiCommand {
@UiComponentId protected String componentId;
protected int requestId;
protected UiClientObjectReference component;
/**
* @deprecated Only for Jackson deserialization. Use the other constructor instead.
*/
@Deprecated
public SetContextMenuContentCommand() {
// default constructor for Jackson
}
public SetContextMenuContentCommand(String componentId, int requestId, UiClientObjectReference component) {
this.componentId = componentId;
this.requestId = requestId;
this.component = component;
}
@SuppressWarnings("unchecked")
public String toString() {
return new StringBuilder(getClass().getSimpleName()).append(": ")
.append("componentId=" + componentId).append(", ")
.append("requestId=" + requestId).append(", ")
.append(component != null ? "component={" + component.toString() + "}" : "")
.toString();
}
@com.fasterxml.jackson.annotation.JsonGetter("componentId")
public String getComponentId() {
return componentId;
}
@com.fasterxml.jackson.annotation.JsonGetter("requestId")
public int getRequestId() {
return requestId;
}
@com.fasterxml.jackson.annotation.JsonGetter("component")
public UiClientObjectReference getComponent() {
return component;
}
}
public static class CloseContextMenuCommand implements UiCommand {
@UiComponentId protected String componentId;
protected int requestId;
/**
* @deprecated Only for Jackson deserialization. Use the other constructor instead.
*/
@Deprecated
public CloseContextMenuCommand() {
// default constructor for Jackson
}
public CloseContextMenuCommand(String componentId, int requestId) {
this.componentId = componentId;
this.requestId = requestId;
}
@SuppressWarnings("unchecked")
public String toString() {
return new StringBuilder(getClass().getSimpleName()).append(": ")
.append("componentId=" + componentId).append(", ")
.append("requestId=" + requestId)
.toString();
}
@com.fasterxml.jackson.annotation.JsonGetter("componentId")
public String getComponentId() {
return componentId;
}
@com.fasterxml.jackson.annotation.JsonGetter("requestId")
public int getRequestId() {
return requestId;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy