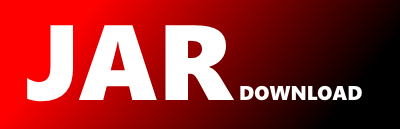
org.teamapps.dto.UiInfiniteItemView2 Maven / Gradle / Ivy
The newest version!
package org.teamapps.dto;
import java.util.ArrayList;
import java.util.Iterator;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Set;
import java.util.function.Supplier;
import java.util.stream.Collectors;
import com.fasterxml.jackson.databind.annotation.JsonDeserialize;
import com.fasterxml.jackson.databind.annotation.JsonSerialize;
import com.fasterxml.jackson.annotation.JsonTypeInfo;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.databind.annotation.JsonTypeIdResolver;
/**
* THIS IS GENERATED CODE!
* PLEASE DO NOT MODIFY - ALL YOUR WORK WOULD BE LOST!
*/
@JsonTypeInfo(use = JsonTypeInfo.Id.CUSTOM, property = "_type", defaultImpl = UiInfiniteItemView2.class)
public class UiInfiniteItemView2 extends UiComponent implements UiObject {
protected UiTemplate itemTemplate;
protected float itemWidth = 0;
protected float itemHeight = 100;
protected float horizontalSpacing;
protected float verticalSpacing;
protected float itemPositionAnimationTime;
protected UiHorizontalElementAlignment itemContentHorizontalAlignment = UiHorizontalElementAlignment.CENTER;
protected UiVerticalElementAlignment itemContentVerticalAlignment = UiVerticalElementAlignment.STRETCH;
protected UiItemJustification rowHorizontalAlignment = UiItemJustification.SPACE_AROUND;
protected boolean contextMenuEnabled;
protected boolean selectionEnabled;
/**
* @deprecated Only for Jackson deserialization. Use the other constructor instead.
*/
@Deprecated
public UiInfiniteItemView2() {
// default constructor for Jackson
}
public UiInfiniteItemView2(UiTemplate itemTemplate) {
super();
this.itemTemplate = itemTemplate;
}
@com.fasterxml.jackson.annotation.JsonIgnore
public UiObjectType getUiObjectType() {
return UiObjectType.UI_INFINITE_ITEM_VIEW2;
}
@SuppressWarnings("unchecked")
public String toString() {
return new StringBuilder(getClass().getSimpleName()).append(": ")
.append("id=" + id).append(", ")
.append("debuggingId=" + debuggingId).append(", ")
.append("classNamesBySelector=" + classNamesBySelector).append(", ")
.append("visible=" + visible).append(", ")
.append("stylesBySelector=" + stylesBySelector).append(", ")
.append("attributesBySelector=" + attributesBySelector).append(", ")
.append(itemTemplate != null ? "itemTemplate={" + itemTemplate.toString() + "}" : "").append(", ")
.append("itemWidth=" + itemWidth).append(", ")
.append("itemHeight=" + itemHeight).append(", ")
.append("horizontalSpacing=" + horizontalSpacing).append(", ")
.append("verticalSpacing=" + verticalSpacing).append(", ")
.append("itemPositionAnimationTime=" + itemPositionAnimationTime).append(", ")
.append("itemContentHorizontalAlignment=" + itemContentHorizontalAlignment).append(", ")
.append("itemContentVerticalAlignment=" + itemContentVerticalAlignment).append(", ")
.append("rowHorizontalAlignment=" + rowHorizontalAlignment).append(", ")
.append("contextMenuEnabled=" + contextMenuEnabled).append(", ")
.append("selectionEnabled=" + selectionEnabled)
.toString();
}
@com.fasterxml.jackson.annotation.JsonGetter("itemTemplate")
public UiTemplate getItemTemplate() {
return itemTemplate;
}
@com.fasterxml.jackson.annotation.JsonGetter("itemWidth")
public float getItemWidth() {
return itemWidth;
}
@com.fasterxml.jackson.annotation.JsonGetter("itemHeight")
public float getItemHeight() {
return itemHeight;
}
@com.fasterxml.jackson.annotation.JsonGetter("horizontalSpacing")
public float getHorizontalSpacing() {
return horizontalSpacing;
}
@com.fasterxml.jackson.annotation.JsonGetter("verticalSpacing")
public float getVerticalSpacing() {
return verticalSpacing;
}
@com.fasterxml.jackson.annotation.JsonGetter("itemPositionAnimationTime")
public float getItemPositionAnimationTime() {
return itemPositionAnimationTime;
}
@com.fasterxml.jackson.annotation.JsonGetter("itemContentHorizontalAlignment")
public UiHorizontalElementAlignment getItemContentHorizontalAlignment() {
return itemContentHorizontalAlignment;
}
@com.fasterxml.jackson.annotation.JsonGetter("itemContentVerticalAlignment")
public UiVerticalElementAlignment getItemContentVerticalAlignment() {
return itemContentVerticalAlignment;
}
@com.fasterxml.jackson.annotation.JsonGetter("rowHorizontalAlignment")
public UiItemJustification getRowHorizontalAlignment() {
return rowHorizontalAlignment;
}
@com.fasterxml.jackson.annotation.JsonGetter("contextMenuEnabled")
public boolean getContextMenuEnabled() {
return contextMenuEnabled;
}
@com.fasterxml.jackson.annotation.JsonGetter("selectionEnabled")
public boolean getSelectionEnabled() {
return selectionEnabled;
}
@com.fasterxml.jackson.annotation.JsonSetter("id")
public UiInfiniteItemView2 setId(String id) {
this.id = id;
return this;
}
@com.fasterxml.jackson.annotation.JsonSetter("debuggingId")
public UiInfiniteItemView2 setDebuggingId(String debuggingId) {
this.debuggingId = debuggingId;
return this;
}
@com.fasterxml.jackson.annotation.JsonSetter("visible")
public UiInfiniteItemView2 setVisible(boolean visible) {
this.visible = visible;
return this;
}
@com.fasterxml.jackson.annotation.JsonSetter("stylesBySelector")
public UiInfiniteItemView2 setStylesBySelector(Map> stylesBySelector) {
this.stylesBySelector = stylesBySelector;
return this;
}
@com.fasterxml.jackson.annotation.JsonSetter("classNamesBySelector")
public UiInfiniteItemView2 setClassNamesBySelector(Map> classNamesBySelector) {
this.classNamesBySelector = classNamesBySelector;
return this;
}
@com.fasterxml.jackson.annotation.JsonSetter("attributesBySelector")
public UiInfiniteItemView2 setAttributesBySelector(Map> attributesBySelector) {
this.attributesBySelector = attributesBySelector;
return this;
}
@com.fasterxml.jackson.annotation.JsonSetter("itemWidth")
public UiInfiniteItemView2 setItemWidth(float itemWidth) {
this.itemWidth = itemWidth;
return this;
}
@com.fasterxml.jackson.annotation.JsonSetter("itemHeight")
public UiInfiniteItemView2 setItemHeight(float itemHeight) {
this.itemHeight = itemHeight;
return this;
}
@com.fasterxml.jackson.annotation.JsonSetter("horizontalSpacing")
public UiInfiniteItemView2 setHorizontalSpacing(float horizontalSpacing) {
this.horizontalSpacing = horizontalSpacing;
return this;
}
@com.fasterxml.jackson.annotation.JsonSetter("verticalSpacing")
public UiInfiniteItemView2 setVerticalSpacing(float verticalSpacing) {
this.verticalSpacing = verticalSpacing;
return this;
}
@com.fasterxml.jackson.annotation.JsonSetter("itemPositionAnimationTime")
public UiInfiniteItemView2 setItemPositionAnimationTime(float itemPositionAnimationTime) {
this.itemPositionAnimationTime = itemPositionAnimationTime;
return this;
}
@com.fasterxml.jackson.annotation.JsonSetter("itemContentHorizontalAlignment")
public UiInfiniteItemView2 setItemContentHorizontalAlignment(UiHorizontalElementAlignment itemContentHorizontalAlignment) {
this.itemContentHorizontalAlignment = itemContentHorizontalAlignment;
return this;
}
@com.fasterxml.jackson.annotation.JsonSetter("itemContentVerticalAlignment")
public UiInfiniteItemView2 setItemContentVerticalAlignment(UiVerticalElementAlignment itemContentVerticalAlignment) {
this.itemContentVerticalAlignment = itemContentVerticalAlignment;
return this;
}
@com.fasterxml.jackson.annotation.JsonSetter("rowHorizontalAlignment")
public UiInfiniteItemView2 setRowHorizontalAlignment(UiItemJustification rowHorizontalAlignment) {
this.rowHorizontalAlignment = rowHorizontalAlignment;
return this;
}
@com.fasterxml.jackson.annotation.JsonSetter("contextMenuEnabled")
public UiInfiniteItemView2 setContextMenuEnabled(boolean contextMenuEnabled) {
this.contextMenuEnabled = contextMenuEnabled;
return this;
}
@com.fasterxml.jackson.annotation.JsonSetter("selectionEnabled")
public UiInfiniteItemView2 setSelectionEnabled(boolean selectionEnabled) {
this.selectionEnabled = selectionEnabled;
return this;
}
public static class DisplayedRangeChangedEvent implements UiEvent {
@UiComponentId protected String componentId;
protected int startIndex;
protected int length;
/**
* @deprecated Only for Jackson deserialization. Use the other constructor instead.
*/
@Deprecated
public DisplayedRangeChangedEvent() {
// default constructor for Jackson
}
public DisplayedRangeChangedEvent(String componentId, int startIndex, int length) {
this.componentId = componentId;
this.startIndex = startIndex;
this.length = length;
}
public UiEventType getUiEventType() {
return UiEventType.UI_INFINITE_ITEM_VIEW2_DISPLAYED_RANGE_CHANGED;
}
@SuppressWarnings("unchecked")
public String toString() {
return new StringBuilder(getClass().getSimpleName()).append(": ")
.append("componentId=" + componentId).append(", ")
.append("startIndex=" + startIndex).append(", ")
.append("length=" + length)
.toString();
}
@com.fasterxml.jackson.annotation.JsonGetter("componentId")
public String getComponentId() {
return componentId;
}
@com.fasterxml.jackson.annotation.JsonGetter("startIndex")
public int getStartIndex() {
return startIndex;
}
@com.fasterxml.jackson.annotation.JsonGetter("length")
public int getLength() {
return length;
}
}
public static class ItemClickedEvent implements UiEvent {
@UiComponentId protected String componentId;
protected int recordId;
protected boolean isDoubleClick;
/**
* @deprecated Only for Jackson deserialization. Use the other constructor instead.
*/
@Deprecated
public ItemClickedEvent() {
// default constructor for Jackson
}
public ItemClickedEvent(String componentId, int recordId, boolean isDoubleClick) {
this.componentId = componentId;
this.recordId = recordId;
this.isDoubleClick = isDoubleClick;
}
public UiEventType getUiEventType() {
return UiEventType.UI_INFINITE_ITEM_VIEW2_ITEM_CLICKED;
}
@SuppressWarnings("unchecked")
public String toString() {
return new StringBuilder(getClass().getSimpleName()).append(": ")
.append("componentId=" + componentId).append(", ")
.append("recordId=" + recordId).append(", ")
.append("isDoubleClick=" + isDoubleClick)
.toString();
}
@com.fasterxml.jackson.annotation.JsonGetter("componentId")
public String getComponentId() {
return componentId;
}
@com.fasterxml.jackson.annotation.JsonGetter("recordId")
public int getRecordId() {
return recordId;
}
@com.fasterxml.jackson.annotation.JsonGetter("isDoubleClick")
public boolean getIsDoubleClick() {
return isDoubleClick;
}
}
public static class ContextMenuRequestedEvent implements UiEvent {
@UiComponentId protected String componentId;
protected int requestId;
protected int recordId;
/**
* @deprecated Only for Jackson deserialization. Use the other constructor instead.
*/
@Deprecated
public ContextMenuRequestedEvent() {
// default constructor for Jackson
}
public ContextMenuRequestedEvent(String componentId, int requestId, int recordId) {
this.componentId = componentId;
this.requestId = requestId;
this.recordId = recordId;
}
public UiEventType getUiEventType() {
return UiEventType.UI_INFINITE_ITEM_VIEW2_CONTEXT_MENU_REQUESTED;
}
@SuppressWarnings("unchecked")
public String toString() {
return new StringBuilder(getClass().getSimpleName()).append(": ")
.append("componentId=" + componentId).append(", ")
.append("requestId=" + requestId).append(", ")
.append("recordId=" + recordId)
.toString();
}
@com.fasterxml.jackson.annotation.JsonGetter("componentId")
public String getComponentId() {
return componentId;
}
@com.fasterxml.jackson.annotation.JsonGetter("requestId")
public int getRequestId() {
return requestId;
}
@com.fasterxml.jackson.annotation.JsonGetter("recordId")
public int getRecordId() {
return recordId;
}
}
public static class SetDataCommand implements UiCommand {
@UiComponentId protected String componentId;
protected int startIndex;
protected List recordIds;
protected List newRecords;
protected int totalNumberOfRecords;
/**
* @deprecated Only for Jackson deserialization. Use the other constructor instead.
*/
@Deprecated
public SetDataCommand() {
// default constructor for Jackson
}
public SetDataCommand(String componentId, int startIndex, List recordIds, List newRecords, int totalNumberOfRecords) {
this.componentId = componentId;
this.startIndex = startIndex;
this.recordIds = recordIds;
this.newRecords = newRecords;
this.totalNumberOfRecords = totalNumberOfRecords;
}
@SuppressWarnings("unchecked")
public String toString() {
return new StringBuilder(getClass().getSimpleName()).append(": ")
.append("componentId=" + componentId).append(", ")
.append("recordIds=" + recordIds).append(", ")
.append("startIndex=" + startIndex).append(", ")
.append("totalNumberOfRecords=" + totalNumberOfRecords).append(", ")
.append(newRecords != null ? "newRecords={" + newRecords.toString() + "}" : "")
.toString();
}
@com.fasterxml.jackson.annotation.JsonGetter("componentId")
public String getComponentId() {
return componentId;
}
@com.fasterxml.jackson.annotation.JsonGetter("startIndex")
public int getStartIndex() {
return startIndex;
}
@com.fasterxml.jackson.annotation.JsonGetter("recordIds")
public List getRecordIds() {
return recordIds;
}
@com.fasterxml.jackson.annotation.JsonGetter("newRecords")
public List getNewRecords() {
return newRecords;
}
@com.fasterxml.jackson.annotation.JsonGetter("totalNumberOfRecords")
public int getTotalNumberOfRecords() {
return totalNumberOfRecords;
}
}
public static class SetItemTemplateCommand implements UiCommand {
@UiComponentId protected String componentId;
protected UiTemplate itemTemplate;
/**
* @deprecated Only for Jackson deserialization. Use the other constructor instead.
*/
@Deprecated
public SetItemTemplateCommand() {
// default constructor for Jackson
}
public SetItemTemplateCommand(String componentId, UiTemplate itemTemplate) {
this.componentId = componentId;
this.itemTemplate = itemTemplate;
}
@SuppressWarnings("unchecked")
public String toString() {
return new StringBuilder(getClass().getSimpleName()).append(": ")
.append("componentId=" + componentId).append(", ")
.append(itemTemplate != null ? "itemTemplate={" + itemTemplate.toString() + "}" : "")
.toString();
}
@com.fasterxml.jackson.annotation.JsonGetter("componentId")
public String getComponentId() {
return componentId;
}
@com.fasterxml.jackson.annotation.JsonGetter("itemTemplate")
public UiTemplate getItemTemplate() {
return itemTemplate;
}
}
public static class SetItemWidthCommand implements UiCommand {
@UiComponentId protected String componentId;
protected float itemWidth;
/**
* @deprecated Only for Jackson deserialization. Use the other constructor instead.
*/
@Deprecated
public SetItemWidthCommand() {
// default constructor for Jackson
}
public SetItemWidthCommand(String componentId, float itemWidth) {
this.componentId = componentId;
this.itemWidth = itemWidth;
}
@SuppressWarnings("unchecked")
public String toString() {
return new StringBuilder(getClass().getSimpleName()).append(": ")
.append("componentId=" + componentId).append(", ")
.append("itemWidth=" + itemWidth)
.toString();
}
@com.fasterxml.jackson.annotation.JsonGetter("componentId")
public String getComponentId() {
return componentId;
}
@com.fasterxml.jackson.annotation.JsonGetter("itemWidth")
public float getItemWidth() {
return itemWidth;
}
}
public static class SetItemHeightCommand implements UiCommand {
@UiComponentId protected String componentId;
protected float itemHeight;
/**
* @deprecated Only for Jackson deserialization. Use the other constructor instead.
*/
@Deprecated
public SetItemHeightCommand() {
// default constructor for Jackson
}
public SetItemHeightCommand(String componentId, float itemHeight) {
this.componentId = componentId;
this.itemHeight = itemHeight;
}
@SuppressWarnings("unchecked")
public String toString() {
return new StringBuilder(getClass().getSimpleName()).append(": ")
.append("componentId=" + componentId).append(", ")
.append("itemHeight=" + itemHeight)
.toString();
}
@com.fasterxml.jackson.annotation.JsonGetter("componentId")
public String getComponentId() {
return componentId;
}
@com.fasterxml.jackson.annotation.JsonGetter("itemHeight")
public float getItemHeight() {
return itemHeight;
}
}
public static class SetHorizontalSpacingCommand implements UiCommand {
@UiComponentId protected String componentId;
protected float horizontalSpacing;
/**
* @deprecated Only for Jackson deserialization. Use the other constructor instead.
*/
@Deprecated
public SetHorizontalSpacingCommand() {
// default constructor for Jackson
}
public SetHorizontalSpacingCommand(String componentId, float horizontalSpacing) {
this.componentId = componentId;
this.horizontalSpacing = horizontalSpacing;
}
@SuppressWarnings("unchecked")
public String toString() {
return new StringBuilder(getClass().getSimpleName()).append(": ")
.append("componentId=" + componentId).append(", ")
.append("horizontalSpacing=" + horizontalSpacing)
.toString();
}
@com.fasterxml.jackson.annotation.JsonGetter("componentId")
public String getComponentId() {
return componentId;
}
@com.fasterxml.jackson.annotation.JsonGetter("horizontalSpacing")
public float getHorizontalSpacing() {
return horizontalSpacing;
}
}
public static class SetVerticalSpacingCommand implements UiCommand {
@UiComponentId protected String componentId;
protected float verticalSpacing;
/**
* @deprecated Only for Jackson deserialization. Use the other constructor instead.
*/
@Deprecated
public SetVerticalSpacingCommand() {
// default constructor for Jackson
}
public SetVerticalSpacingCommand(String componentId, float verticalSpacing) {
this.componentId = componentId;
this.verticalSpacing = verticalSpacing;
}
@SuppressWarnings("unchecked")
public String toString() {
return new StringBuilder(getClass().getSimpleName()).append(": ")
.append("componentId=" + componentId).append(", ")
.append("verticalSpacing=" + verticalSpacing)
.toString();
}
@com.fasterxml.jackson.annotation.JsonGetter("componentId")
public String getComponentId() {
return componentId;
}
@com.fasterxml.jackson.annotation.JsonGetter("verticalSpacing")
public float getVerticalSpacing() {
return verticalSpacing;
}
}
public static class SetItemContentHorizontalAlignmentCommand implements UiCommand {
@UiComponentId protected String componentId;
protected UiHorizontalElementAlignment itemContentHorizontalAlignment;
/**
* @deprecated Only for Jackson deserialization. Use the other constructor instead.
*/
@Deprecated
public SetItemContentHorizontalAlignmentCommand() {
// default constructor for Jackson
}
public SetItemContentHorizontalAlignmentCommand(String componentId, UiHorizontalElementAlignment itemContentHorizontalAlignment) {
this.componentId = componentId;
this.itemContentHorizontalAlignment = itemContentHorizontalAlignment;
}
@SuppressWarnings("unchecked")
public String toString() {
return new StringBuilder(getClass().getSimpleName()).append(": ")
.append("componentId=" + componentId).append(", ")
.append("itemContentHorizontalAlignment=" + itemContentHorizontalAlignment)
.toString();
}
@com.fasterxml.jackson.annotation.JsonGetter("componentId")
public String getComponentId() {
return componentId;
}
@com.fasterxml.jackson.annotation.JsonGetter("itemContentHorizontalAlignment")
public UiHorizontalElementAlignment getItemContentHorizontalAlignment() {
return itemContentHorizontalAlignment;
}
}
public static class SetItemContentVerticalAlignmentCommand implements UiCommand {
@UiComponentId protected String componentId;
protected UiVerticalElementAlignment itemContentVerticalAlignment;
/**
* @deprecated Only for Jackson deserialization. Use the other constructor instead.
*/
@Deprecated
public SetItemContentVerticalAlignmentCommand() {
// default constructor for Jackson
}
public SetItemContentVerticalAlignmentCommand(String componentId, UiVerticalElementAlignment itemContentVerticalAlignment) {
this.componentId = componentId;
this.itemContentVerticalAlignment = itemContentVerticalAlignment;
}
@SuppressWarnings("unchecked")
public String toString() {
return new StringBuilder(getClass().getSimpleName()).append(": ")
.append("componentId=" + componentId).append(", ")
.append("itemContentVerticalAlignment=" + itemContentVerticalAlignment)
.toString();
}
@com.fasterxml.jackson.annotation.JsonGetter("componentId")
public String getComponentId() {
return componentId;
}
@com.fasterxml.jackson.annotation.JsonGetter("itemContentVerticalAlignment")
public UiVerticalElementAlignment getItemContentVerticalAlignment() {
return itemContentVerticalAlignment;
}
}
public static class SetRowHorizontalAlignmentCommand implements UiCommand {
@UiComponentId protected String componentId;
protected UiItemJustification rowHorizontalAlignment;
/**
* @deprecated Only for Jackson deserialization. Use the other constructor instead.
*/
@Deprecated
public SetRowHorizontalAlignmentCommand() {
// default constructor for Jackson
}
public SetRowHorizontalAlignmentCommand(String componentId, UiItemJustification rowHorizontalAlignment) {
this.componentId = componentId;
this.rowHorizontalAlignment = rowHorizontalAlignment;
}
@SuppressWarnings("unchecked")
public String toString() {
return new StringBuilder(getClass().getSimpleName()).append(": ")
.append("componentId=" + componentId).append(", ")
.append("rowHorizontalAlignment=" + rowHorizontalAlignment)
.toString();
}
@com.fasterxml.jackson.annotation.JsonGetter("componentId")
public String getComponentId() {
return componentId;
}
@com.fasterxml.jackson.annotation.JsonGetter("rowHorizontalAlignment")
public UiItemJustification getRowHorizontalAlignment() {
return rowHorizontalAlignment;
}
}
public static class SetItemPositionAnimationTimeCommand implements UiCommand {
@UiComponentId protected String componentId;
protected int animationMillis;
/**
* @deprecated Only for Jackson deserialization. Use the other constructor instead.
*/
@Deprecated
public SetItemPositionAnimationTimeCommand() {
// default constructor for Jackson
}
public SetItemPositionAnimationTimeCommand(String componentId, int animationMillis) {
this.componentId = componentId;
this.animationMillis = animationMillis;
}
@SuppressWarnings("unchecked")
public String toString() {
return new StringBuilder(getClass().getSimpleName()).append(": ")
.append("componentId=" + componentId).append(", ")
.append("animationMillis=" + animationMillis)
.toString();
}
@com.fasterxml.jackson.annotation.JsonGetter("componentId")
public String getComponentId() {
return componentId;
}
@com.fasterxml.jackson.annotation.JsonGetter("animationMillis")
public int getAnimationMillis() {
return animationMillis;
}
}
public static class SetSelectionEnabledCommand implements UiCommand {
@UiComponentId protected String componentId;
protected boolean selectionEnabled;
/**
* @deprecated Only for Jackson deserialization. Use the other constructor instead.
*/
@Deprecated
public SetSelectionEnabledCommand() {
// default constructor for Jackson
}
public SetSelectionEnabledCommand(String componentId, boolean selectionEnabled) {
this.componentId = componentId;
this.selectionEnabled = selectionEnabled;
}
@SuppressWarnings("unchecked")
public String toString() {
return new StringBuilder(getClass().getSimpleName()).append(": ")
.append("componentId=" + componentId).append(", ")
.append("selectionEnabled=" + selectionEnabled)
.toString();
}
@com.fasterxml.jackson.annotation.JsonGetter("componentId")
public String getComponentId() {
return componentId;
}
@com.fasterxml.jackson.annotation.JsonGetter("selectionEnabled")
public boolean getSelectionEnabled() {
return selectionEnabled;
}
}
public static class SetSelectedRecordCommand implements UiCommand {
@UiComponentId protected String componentId;
protected Integer uiRecordId;
/**
* @deprecated Only for Jackson deserialization. Use the other constructor instead.
*/
@Deprecated
public SetSelectedRecordCommand() {
// default constructor for Jackson
}
public SetSelectedRecordCommand(String componentId, Integer uiRecordId) {
this.componentId = componentId;
this.uiRecordId = uiRecordId;
}
@SuppressWarnings("unchecked")
public String toString() {
return new StringBuilder(getClass().getSimpleName()).append(": ")
.append("componentId=" + componentId).append(", ")
.append("uiRecordId=" + uiRecordId)
.toString();
}
@com.fasterxml.jackson.annotation.JsonGetter("componentId")
public String getComponentId() {
return componentId;
}
@com.fasterxml.jackson.annotation.JsonGetter("uiRecordId")
public Integer getUiRecordId() {
return uiRecordId;
}
}
public static class SetContextMenuContentCommand implements UiCommand {
@UiComponentId protected String componentId;
protected int requestId;
protected UiClientObjectReference component;
/**
* @deprecated Only for Jackson deserialization. Use the other constructor instead.
*/
@Deprecated
public SetContextMenuContentCommand() {
// default constructor for Jackson
}
public SetContextMenuContentCommand(String componentId, int requestId, UiClientObjectReference component) {
this.componentId = componentId;
this.requestId = requestId;
this.component = component;
}
@SuppressWarnings("unchecked")
public String toString() {
return new StringBuilder(getClass().getSimpleName()).append(": ")
.append("componentId=" + componentId).append(", ")
.append("requestId=" + requestId).append(", ")
.append(component != null ? "component={" + component.toString() + "}" : "")
.toString();
}
@com.fasterxml.jackson.annotation.JsonGetter("componentId")
public String getComponentId() {
return componentId;
}
@com.fasterxml.jackson.annotation.JsonGetter("requestId")
public int getRequestId() {
return requestId;
}
@com.fasterxml.jackson.annotation.JsonGetter("component")
public UiClientObjectReference getComponent() {
return component;
}
}
public static class CloseContextMenuCommand implements UiCommand {
@UiComponentId protected String componentId;
protected int requestId;
/**
* @deprecated Only for Jackson deserialization. Use the other constructor instead.
*/
@Deprecated
public CloseContextMenuCommand() {
// default constructor for Jackson
}
public CloseContextMenuCommand(String componentId, int requestId) {
this.componentId = componentId;
this.requestId = requestId;
}
@SuppressWarnings("unchecked")
public String toString() {
return new StringBuilder(getClass().getSimpleName()).append(": ")
.append("componentId=" + componentId).append(", ")
.append("requestId=" + requestId)
.toString();
}
@com.fasterxml.jackson.annotation.JsonGetter("componentId")
public String getComponentId() {
return componentId;
}
@com.fasterxml.jackson.annotation.JsonGetter("requestId")
public int getRequestId() {
return requestId;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy